C++ frameworks provide structured environments and libraries that streamline the development process by offering reusable components and tools for building applications more efficiently.
Here's a simple example of using the Qt framework to create a basic window application:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, C++ Framework!");
button.resize(200, 100);
button.show();
return app.exec();
}
What is a Framework in C++?
A C++ framework can be defined as a collection of pre-written code that provides a foundation to help developers build applications efficiently. Unlike libraries, which provide a set of functions that can be called directly, frameworks dictate the architecture of your application and come with a structure that your code must adhere to. This can significantly speed up the development process by minimizing the amount of repetitive coding required.

Why Use C++ Frameworks?
Utilizing C++ frameworks offers several compelling benefits:
- Increased Productivity: Frameworks encapsulate common patterns and best practices, allowing developers to work more efficiently.
- Standardized Practices: Frameworks enforce coding standards and patterns that can improve code maintainability.
- Robust Community Support: Many frameworks come with extensive documentation and active communities, which can provide assistance and resources for common challenges.
For example, the rise of frameworks like Qt and POCO has enabled rapid development in both GUI and networking applications, giving developers the tools they need to produce quality software without reinventing the wheel.
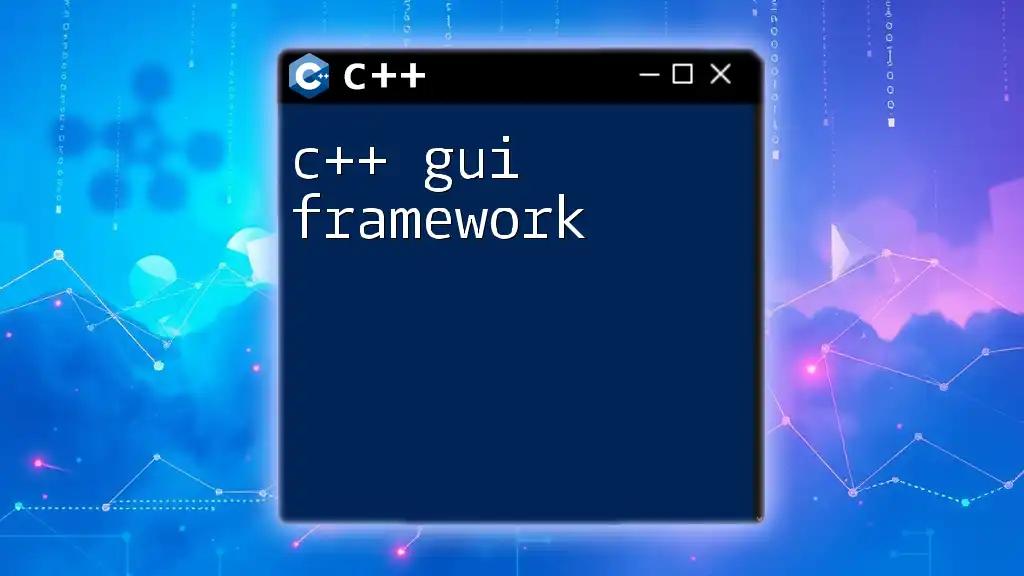
Popular C++ Frameworks
Qt Framework
Overview of Qt
Qt is one of the most well-known C++ frameworks, designed primarily for developing graphical user interfaces (GUIs) and cross-platform applications. It has a rich set of libraries, tools, and APIs that allow developers to create apps that run seamlessly across different platforms, such as Windows, macOS, and Linux.
Getting Started with Qt
To get started with Qt, you first need to install the Qt framework on your machine. The official Qt website provides an installer where you can select the components you wish to install. Once installation is complete, you can create a new project in the Qt Creator IDE.
Example: Creating a Simple GUI Application with Qt
Here’s a small code snippet that demonstrates how to create a basic window using Qt:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
In this example, we include the necessary Qt headers, create a `QApplication` instance, and then a `QPushButton`. The button displays the text "Hello, World!" and is resized accordingly. When the button is shown, the application is executed, providing a window interface.
Boost
What is Boost?
Boost is a collection of C++ libraries that extend the functionality of C++ by providing additional modules to streamline coding tasks. It's highly regarded within the C++ community for its ability to enhance both standard libraries and the language itself.
Key Libraries and Functions
Boost includes a plethora of libraries that can help with various programming needs:
- Boost.Asio: For network programming
- Boost.Filesystem: For file system manipulation
- Boost.Regex: For regular expressions handling
Example: File Handling with Boost.Filesystem
Here’s a simple example of how to use Boost.Filesystem to handle file operations:
#include <boost/filesystem.hpp>
#include <iostream>
int main() {
boost::filesystem::path filePath("example.txt");
// Check if file exists
if (boost::filesystem::exists(filePath)) {
std::cout << "File exists." << std::endl;
} else {
std::cout << "File does not exist. Creating the file." << std::endl;
std::ofstream file(filePath.string());
file << "This is a new file created with Boost.Filesystem." << std::endl;
file.close();
}
return 0;
}
In this example, we check if a file named `example.txt` exists in the current directory. If it does not exist, the program creates it and writes a line of text to it. The `boost::filesystem` library simplifies file existence checks and operations.
POCO C++ Libraries
Introduction to POCO Libraries
POCO (C++ Portable Components) is an open-source framework that provides a collection of C++ libraries to build network-centric applications and services. It’s modular and portable, making it suitable for both large enterprises and small projects.
Key Features of POCO Libraries
Some of the capabilities included in POCO libraries are:
- Networking support for creating HTTP servers and clients.
- File system access for managing files and directories.
- Threading support for building multi-threaded applications.
Example: Building a REST API with POCO
Here’s a basic example demonstrating how to create a simple REST API using POCO:
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPServerRequest.h>
#include <Poco/Net/HTTPServerResponse.h>
#include <Poco/Net/HTTPRequestHandler.h>
#include <Poco/Net/HTTPRequestHandlerFactory.h>
#include <Poco/Util/ServerApplication.h>
class HelloWorldRequestHandler : public Poco::Net::HTTPRequestHandler {
public:
void handleRequest(Poco::Net::HTTPServerRequest& request, Poco::Net::HTTPServerResponse& response) override {
response.setContentType("text/plain");
std::ostream& out = response.send();
out << "Hello, World from POCO!";
}
};
class HelloWorldRequestHandlerFactory : public Poco::Net::HTTPRequestHandlerFactory {
public:
Poco::Net::HTTPRequestHandler* createRequestHandler(const Poco::Net::HTTPServerRequest& request) override {
return new HelloWorldRequestHandler();
}
};
class HelloWorldServer : public Poco::Util::ServerApplication {
protected:
int main(const std::vector<std::string>& args) override {
Poco::Net::HTTPServer server(new HelloWorldRequestHandlerFactory, Poco::Net::ServerSocket(8080), new Poco::Net::HTTPServerParams);
server.start();
waitForTerminationRequest();
server.stop();
return 0;
}
};
int main(int argc, char** argv) {
HelloWorldServer app;
return app.run(argc, argv);
}
This example sets up a simple HTTP server that listens on port 8080. When accessed, it responds with "Hello, World from POCO!" This showcases the simplicity of building web services using the POCO libraries.
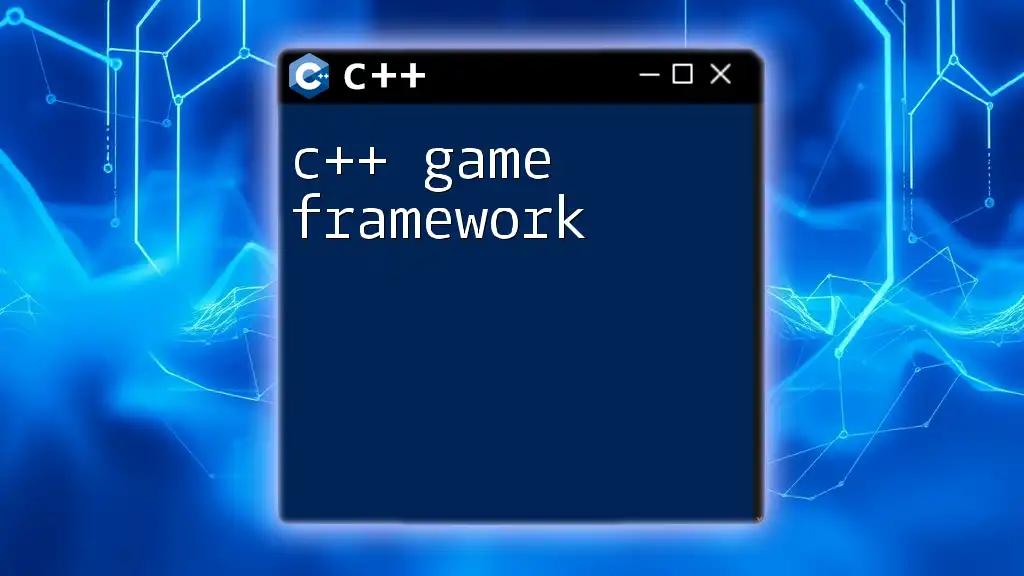
Other Noteworthy C++ Frameworks
Cinder
What is Cinder?
Cinder is an open-source C++ framework designed primarily for creative coding, providing tools for interactive media, visual art, and motion graphics. Its focus on graphics and computational design makes it a favorite among artists and designers.
Basic Concepts and Tools in Cinder
Cinder includes support for advanced graphics, including 2D and 3D rendering, audio, and even video manipulation. The framework offers a structured interface that allows for creativity and flexibility in designing applications.
Example: A Simple Graphics Application
Here’s an example of using Cinder to create a simple graphics application:
#include <cinder/app/App.h>
#include <cinder/app/RendererGl.h>
#include <cinder/gl/gl.h>
class SimpleApp : public ci::app::App {
public:
void setup() override {
ci::gl::clear(ci::Color(0, 0, 0)); // Clear screen with black
}
void draw() override {
ci::gl::clear(ci::Color(0, 0, 0));
ci::gl::color(1, 0, 0); // Set color to red
ci::gl::drawSolidCircle(getWindowCenter(), 50); // Draw a solid circle
}
};
CINDER_APP(SimpleApp, ci::app::RendererGl)
This snippet creates an application that clears the screen and draws a red solid circle in the center. Cinder’s simplicity in handling graphics makes it ideal for artists looking to integrate coding with their creative expressions.
wxWidgets
Overview of wxWidgets
wxWidgets is a C++ framework that allows developers to create native GUI applications on various platforms, including Windows, macOS, and Linux. It provides a rich set of classes that wrap native controls, ensuring a native look-and-feel while maintaining a single codebase.
Creating Cross-Platform Applications
To begin with wxWidgets, you need to download the framework and set it up within your development environment. Once set up, you can create a new wxWidgets application using its project templates.
Example: Building a Basic GUI with wxWidgets
Here’s an example code snippet to set up a simple wxWidgets application:
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame(const wxString& title);
private:
void OnHello(wxCommandEvent& event);
void OnExit(wxCommandEvent& event);
};
enum {
ID_Hello = 1
};
wxBEGIN_EVENT_TABLE(MyFrame, wxFrame)
EVT_MENU(ID_Hello, MyFrame::OnHello)
EVT_MENU(wxID_EXIT, MyFrame::OnExit)
wxEND_EVENT_TABLE()
wxIMPLEMENT_APP(MyApp);
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame("Hello wxWidgets");
frame->Show(true);
return true;
}
MyFrame::MyFrame(const wxString& title)
: wxFrame(NULL, wxID_ANY, title) {
wxMenu *menuFile = new wxMenu;
menuFile->Append(ID_Hello, "&Hello\tCtrl-H", "Help string shown in status bar");
menuFile->AppendSeparator();
menuFile->Append(wxID_EXIT, "E&xit\tCtrl-Q", "Quit this program");
wxMenuBar *menuBar = new wxMenuBar;
menuBar->Append(menuFile, "&File");
SetMenuBar(menuBar);
}
void MyFrame::OnHello(wxCommandEvent& event) {
wxLogMessage("Hello wxWidgets!");
}
void MyFrame::OnExit(wxCommandEvent& event) {
Close(true);
}
This wxWidgets example sets up a basic application with a menu that includes options to display a message ("Hello wxWidgets!") and exit the application. The framework is straightforward, making it easy to create cross-platform GUI applications.
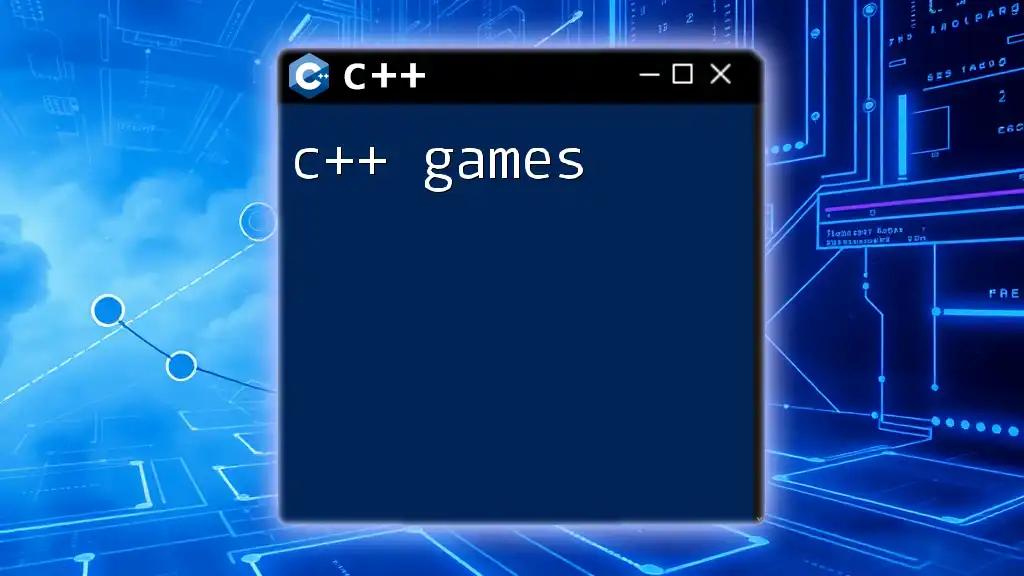
Key Factors to Consider
Project Requirements
When choosing a C++ framework, it’s essential to evaluate your project requirements. For instance, if your project revolves around GUI development, frameworks like Qt or wxWidgets may be more appropriate. On the other hand, if you’re working on network services, Boost or POCO might be the better options.
Community and Support
Another crucial factor is the community and support surrounding the framework. A strong community means access to tutorials, forums, and open-source contributions, which can significantly speed up your learning curve and troubleshooting efforts.
Performance Considerations
Framework choices can also impact performance. Some frameworks might introduce overhead due to abstraction, while others are optimized for specific tasks. Understanding these trade-offs can help make an informed decision.
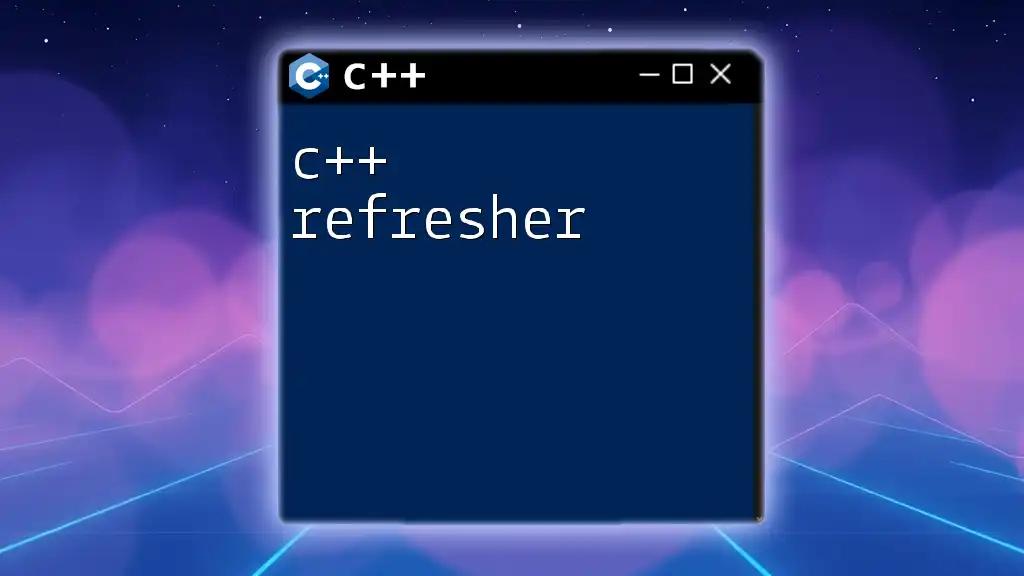
Framework Comparisons
Qt vs. Boost vs. POCO
When comparing Qt, Boost, and POCO, each has its strengths and weaknesses. Qt excels in creating rich GUI applications with cross-platform capabilities, while Boost offers a vast library for diverse tasks. POCO stands out in network-centric development, providing a powerful toolkit for building web services.
When to Choose Each Framework
When deciding which framework to choose, consider your specific project goals, the features offered by each framework, and the learning curve associated with that framework. Trying out small sample projects in each can also facilitate a hands-on understanding of their capabilities.

Recap of C++ Framework Benefits
In summary, C++ frameworks simplify the development process by providing a structured approach to application design. They encourage best practices and reusable components, leading to more maintainable and scalable software solutions.
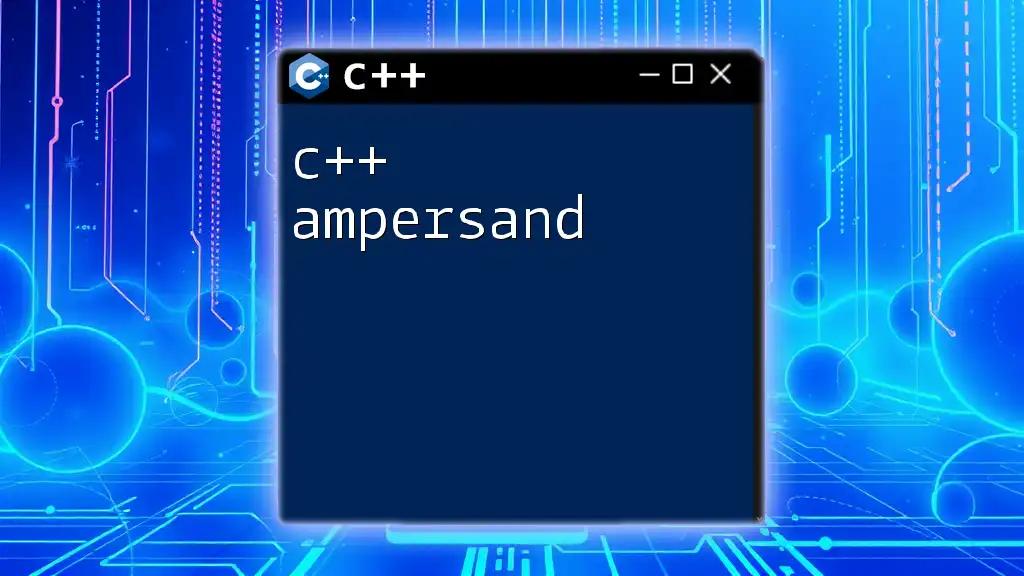
Encouragement to Explore Frameworks
As you start your journey into C++ development, I encourage you to explore various frameworks and see how they can enhance your coding efficiency. Embrace the power of frameworks to unlock new possibilities in your projects.
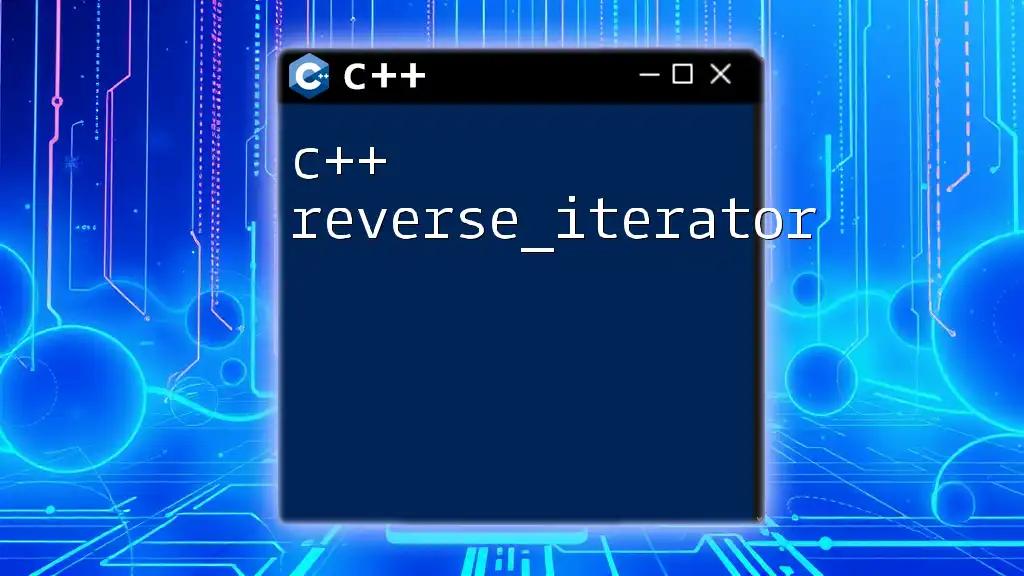
Resources for Further Learning
For those keen on diving deeper, consider reviewing documentation and tutorials from each framework's official website or engaging with the community in forums and open-source projects. From Qt to Boost, the resources available are abundant and invaluable for mastering C++ frameworks.