The Qt C++ framework is a powerful, cross-platform toolkit that simplifies the development of GUI applications and allows for the creation of rich user interfaces with integrated functionality.
Here’s a simple example of creating a basic Qt application window:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Qt C++ Example");
window.show();
return app.exec();
}
What is the Qt Framework?
The Qt Framework is an open-source, cross-platform development framework primarily used for creating graphical user interfaces (GUIs) and multi-platform applications. It has evolved significantly since its inception in the mid-1990s, becoming a powerful toolkit that allows developers to build applications for desktops, mobile devices, and embedded systems.
The framework consists of several core components, including:
- Core Modules: Basic functionality required for all applications, such as data types and event handling.
- GUI Modules: Essential for building user interfaces, providing widgets and UI elements.
- Network Modules: Facilitate network communication capabilities.
- Multimedia Modules: Handle audio and video content in applications.
The Qt Framework encapsulates vast functionalities that streamline the development process, making it a favorite among developers globally.
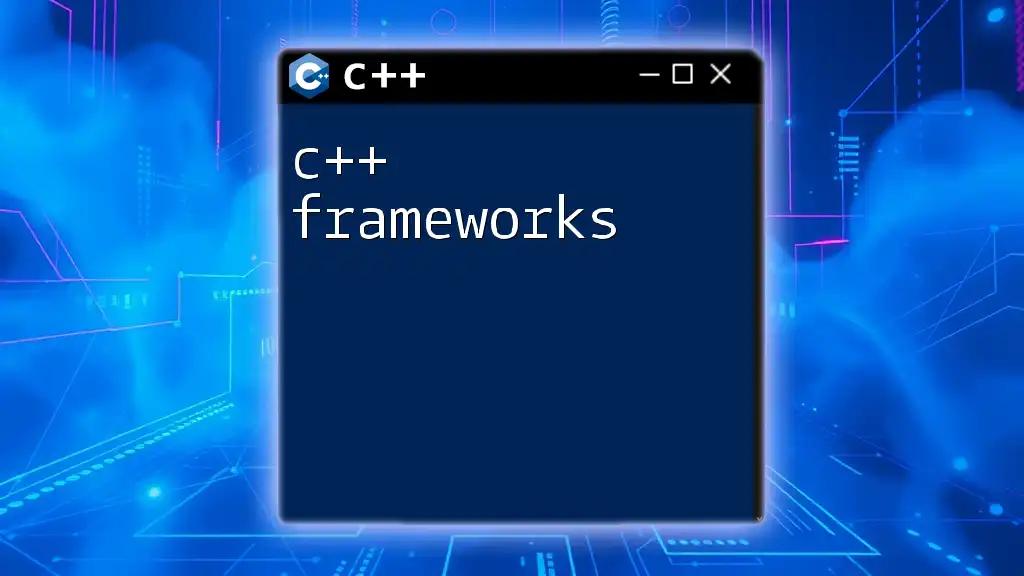
Why Choose Qt for C++?
When it comes to developing applications, choosing the right framework can make a significant difference. The Qt C++ Framework stands out due to its:
- Cross-Platform Capabilities: Write your code once and deploy it across multiple platforms (Windows, macOS, Linux, Android, iOS) with minimal modifications.
- Rich User Interface Features: Provides intuitive APIs for creating dynamic user interfaces, supporting various graphics and animation effects.
- Integrated Development Environment (IDE): Qt Creator offers advanced features such as code completion, debugging, and seamless project management.
With its wide array of features, Qt is an excellent choice for developers looking to create applications efficiently while ensuring high quality and functionality.

Setting Up Qt for C++ Development
Installing Qt Framework
Getting started with the Qt C++ Framework begins with installation. To install Qt:
- Visit the [Qt download page](https://www.qt.io/download) and download the Qt installer suitable for your operating system.
- Run the installer and follow the prompts to choose components. It’s recommended to install the Qt Creator IDE for a more integrated experience.
- After installation, set up the environment to ensure everything works smoothly.
Setting up your environment with an IDE, like Qt Creator, can greatly enhance your coding experience by providing valuable tools to streamline development.
Creating Your First Qt C++ Project
Once the installation is complete, you can start a new project:
- Open Qt Creator and navigate to "File" > "New File or Project…" to create a new project.
- Select "Qt Widgets Application" from the project types available and click "Choose…".
- Specify the project name and location, and configure various settings as required.
After completing these steps, you will see a well-structured directory containing key files such as `main.cpp`, `mainwindow.ui`, and others, which together form your application.
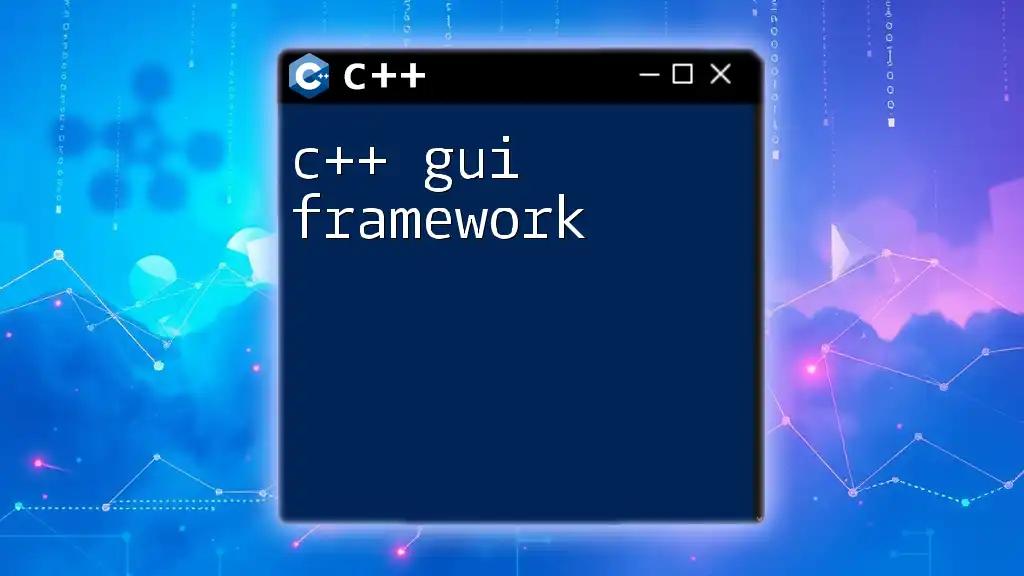
Understanding the Core Components of Qt Framework
Qt Modules Overview
The Qt Framework is modular, providing various modules to address different programming needs. Some important modules include:
- Core Modules: Include foundational classes such as `QCoreApplication` for handling application control flow and `QObject`, the base class for all Qt objects which enables signals and slots functionality.
- GUI Modules: Offer classes like `QWidget` (base class for all UI objects) and `QMainWindow` (provides a main application window) that aid in constructing engaging interfaces.
- Network Module: Essential for building networked applications, featuring classes like `QNetworkAccessManager` for handling HTTP requests and `QNetworkReply` for processing responses.
- Multimedia Module: Classes like `QMediaPlayer` and `QVideoWidget` are provided to facilitate media playback within your applications.
Key Classes in Qt
The heart of the Qt C++ Framework can be found in its classes. A prime example is:
- QObject: The foundational class that provides functionalities to manage object lifecycles, memory management, signals, and slots.
One of the distinctive features of Qt is its signals and slots mechanism, which allows communication between objects. For instance, connecting a signal emitted by a button to a slot that defines what happens when the button is clicked:
QObject::connect(sender, SIGNAL(signalName()), receiver, SLOT(slotName()));
This concise syntax encapsulates the elegance of Qt’s event-driven programming model.

Building User Interfaces with Qt
Introduction to Qt Widgets
Creating user interfaces with Qt is as simple as incorporating various Qt Widgets. Some commonly used widgets include:
- QPushButton: A clickable button that can trigger actions.
- QLabel: Displays static text or images.
- QLineEdit: Allows users to input text.
To create a basic QPushButton, you can initialize it in your code as follows:
QPushButton *button = new QPushButton("Click Me!");
This simplicity makes constructing UIs straightforward while maintaining functionality.
Layout Management in Qt
Proper layout management is crucial for building responsive and aesthetically pleasing interfaces. Qt offers several layout managers to arrange widgets effectively:
- QHBoxLayout: Lays out widgets horizontally.
- QVBoxLayout: Stacks widgets vertically.
- QGridLayout: Positions widgets in a grid format.
Using `QVBoxLayout` as an example, you can create a vertical layout as follows:
QVBoxLayout *layout = new QVBoxLayout();
layout->addWidget(button);
Leveraging these layout managers allows for dynamic scaling of your UI, accommodating various screen sizes effortlessly.

Event Handling in Qt C++
Understanding Events and Event Loop
At its core, Qt operates on an event-driven basis. An event is any significant interaction that your application can respond to, such as mouse clicks or keyboard input. Managing these events is facilitated by the event loop, which continually checks for incoming events to process within your application.
Writing Event Handlers
Creating custom responses to events is straightforward in Qt. For instance, handling a button click event can be done with the following example:
connect(button, &QPushButton::clicked, []() {
qDebug() << "Button clicked!";
});
In this case, when the button is clicked, the message "Button clicked!" is printed to the debug output, showcasing how easy it is to respond to events in the Qt C++ Framework.
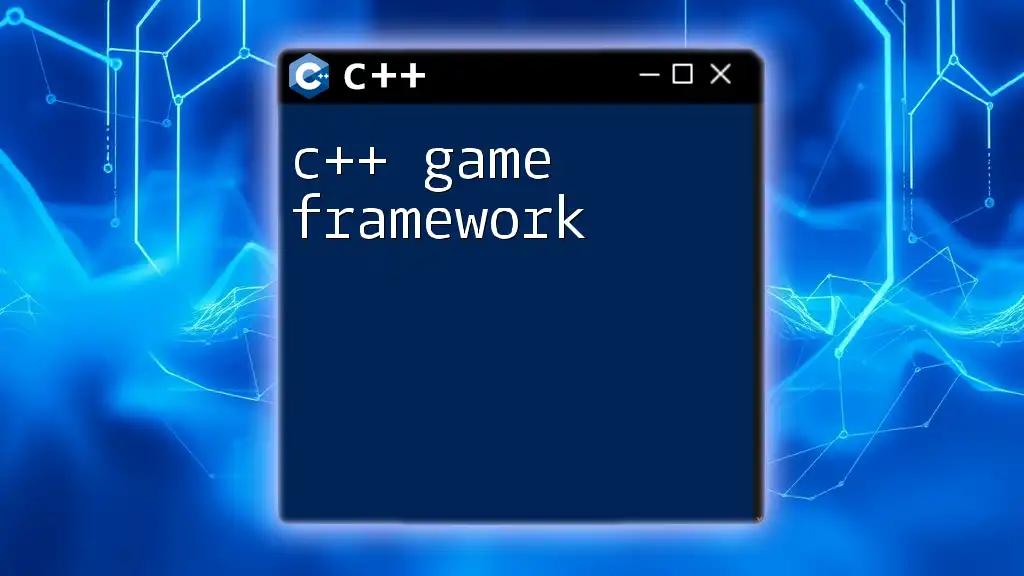
Advanced Features of Qt
Working with Qt QML
QML (Qt Modeling Language) is a declarative language that allows for quick and easy UI creation, often used in conjunction with C++. QML simplifies user interface design, allowing developers to create dynamic and fluid interfaces by defining UI components in a straightforward syntax.
For example, a simple QML file could look like this:
import QtQuick 2.0
Rectangle {
width: 200; height: 200
color: "lightblue"
Text {
text: "Hello, Qt!"
}
}
Utilizing QML can lead to highly interactive applications with less code, allowing developers to harness both the power of C++ and the flexibility of QML.
Internationalization in Qt
To make applications more accessible, organizing internationalization (i18n) support within your Qt C++ Framework project is vital. With Qt's extensive support for translations, developers can easily adapt their applications for various languages.
The following code demonstrates how to set up translations:
QApplication::setApplicationName("MyApp");
QTranslator translator;
translator.load(":/translations/myapp_fr.qm");
app.installTranslator(&translator);
With just a few steps, your application can cater to a global audience, providing a better user experience for non-English speakers.
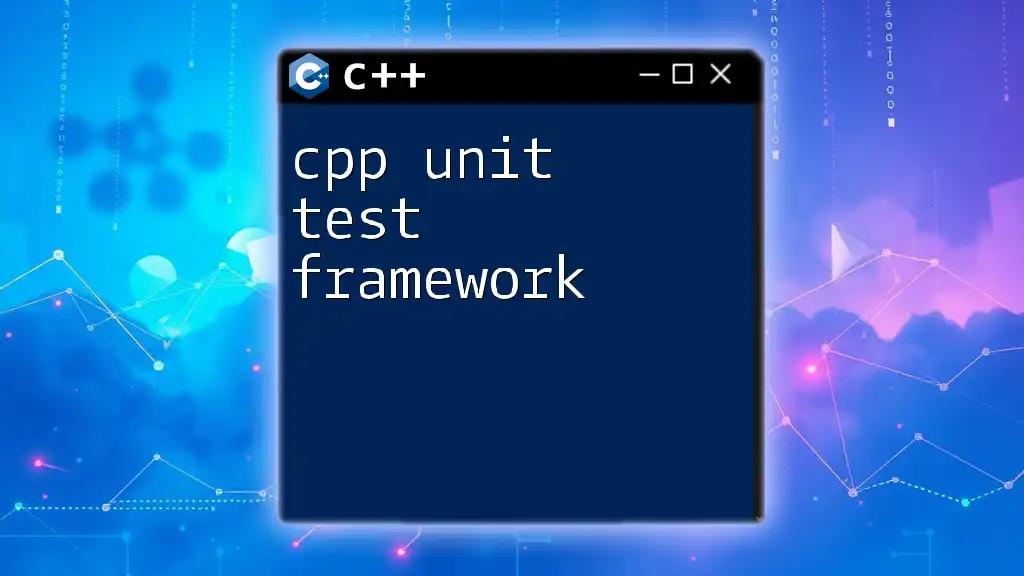
Debugging and Testing Qt Applications
Debugging Techniques
Debugging is essential in software development, and Qt Creator provides an integrated debugger that simplifies this process. You can set breakpoints, inspect variables, and analyze the call stack, providing a comprehensive toolkit to identify and fix issues within your application.
Writing Tests for Qt Applications
Ensuring that your code is reliable through testing is crucial. The Qt Test framework provides a simple and effective way to create unit tests. Here's a brief example:
#include <QtTest/QtTest>
class TestMyClass : public QObject {
Q_OBJECT
private slots:
void testCase1() {
QVERIFY(true);
}
};
By incorporating tests into your development cycle, you can maintain high-quality standards and ensure that your application behaves as expected.

Conclusion
As we have explored, the Qt C++ Framework is a robust, versatile toolkit for application development. From installing the framework to building rich user interfaces and managing complex functionality, Qt empowers developers with the tools necessary for creating high-quality applications.
For those eager to dive deeper into Qt and expand their skills, countless resources are available, including books, online tutorials, and community forums. By engaging with these resources, you can enhance your knowledge and further elevate your coding proficiency in the invigorating world of C++ and the Qt Framework.