The `std::remove` function in C++ is used to remove elements from a container based on a specified value, effectively shifting remaining elements to fill in the gap.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 2, 5};
vec.erase(std::remove(vec.begin(), vec.end(), 2), vec.end());
for (int num : vec) {
std::cout << num << " "; // Output: 1 3 4 5
}
return 0;
}
What is the `remove` Function?
The `remove` function is a powerful tool in C++ that allows developers to efficiently eliminate elements from sequences. It is particularly useful in the context of the Standard Template Library (STL), helping manage collections of items without ever needing to explicitly "erase" them from the data structure. Instead, `remove` rearranges the elements to push unwanted values towards the end of the collection.
Syntax of `remove`
The syntax of the `remove` function can be expressed as follows:
std::remove(iterator first, iterator last, const value_type& val);
- Parameters:
- `first`: The starting iterator of the range.
- `last`: The ending iterator (one past the last element).
- `val`: The value to be removed from the range.
Return Value
The function returns an iterator that points to the new end of the range after the specified value has been removed, providing the ability to further manipulate the container easily.
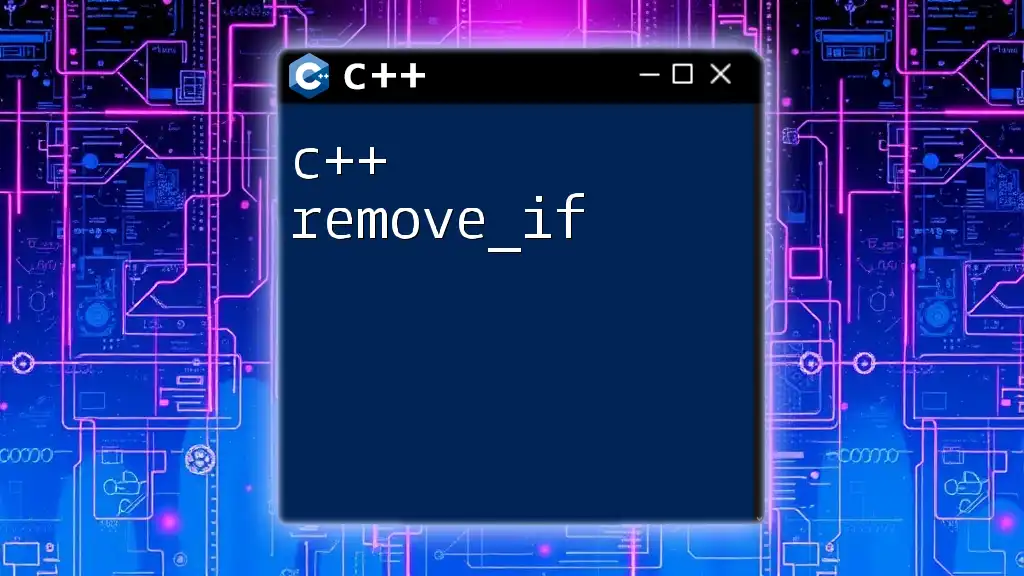
Context of Usage
The `remove` Function in STL
Located within the `<algorithm>` header, the `remove` function is part of the STL, which is a crucial component in modern C++ programming. The STL provides a robust collection of algorithms and data structures that streamline coding processes, enabling developers to focus on core logic rather than implementation details.
Common Use Cases
The `remove` function is commonly applied to various data structures, including vectors and lists. Scenarios where one might find it useful include removing duplicates from an array or eliminating specific elements from a collection efficiently.
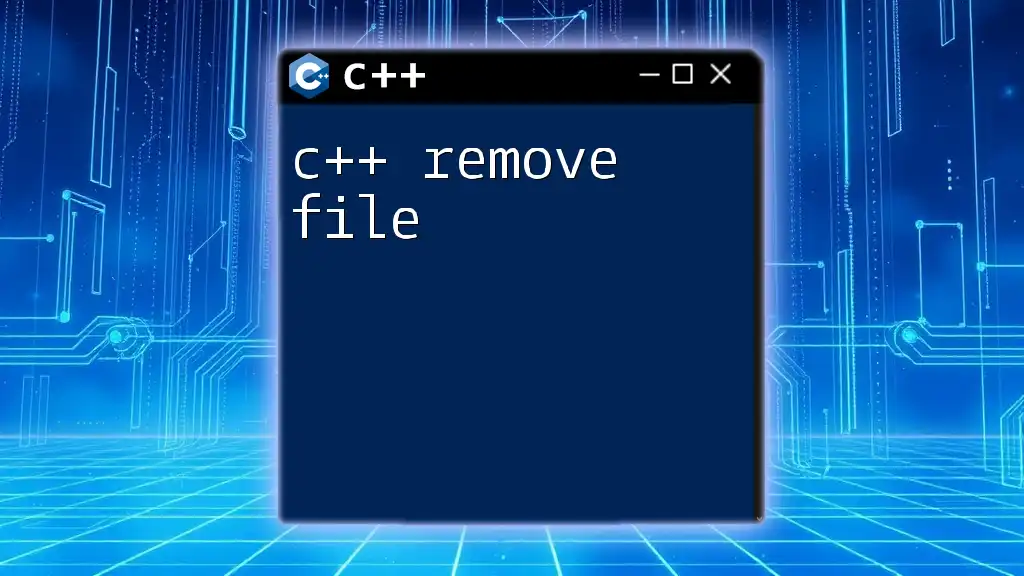
How to Use the `remove` Function
Step-by-Step Guide
Step 1: Include Necessary Headers
To use the `remove` function, make sure to include the necessary headers in your C++ program:
#include <iostream>
#include <vector>
#include <algorithm>
Step 2: Create a Data Structure
Next, you need to create a data structure that will hold the values. For example:
std::vector<int> vec = {1, 2, 3, 4, 2, 5};
Step 3: Call the `remove` Function
Now, you can invoke the `remove` function to eliminate elements from your vector:
auto new_end = std::remove(vec.begin(), vec.end(), 2);
Step 4: Resize the Container
One key aspect of using `remove` is recognizing that it only rearranges elements but does not physically reduce the container's size. Thus, you need to resize the vector:
vec.erase(new_end, vec.end());
Complete Example
Here’s a complete sample program that illustrates these steps in action:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 2, 5};
auto new_end = std::remove(vec.begin(), vec.end(), 2);
vec.erase(new_end, vec.end());
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
In this example, the controversial instances of `2` are removed, and the vector then contains: `1, 3, 4, 5`.
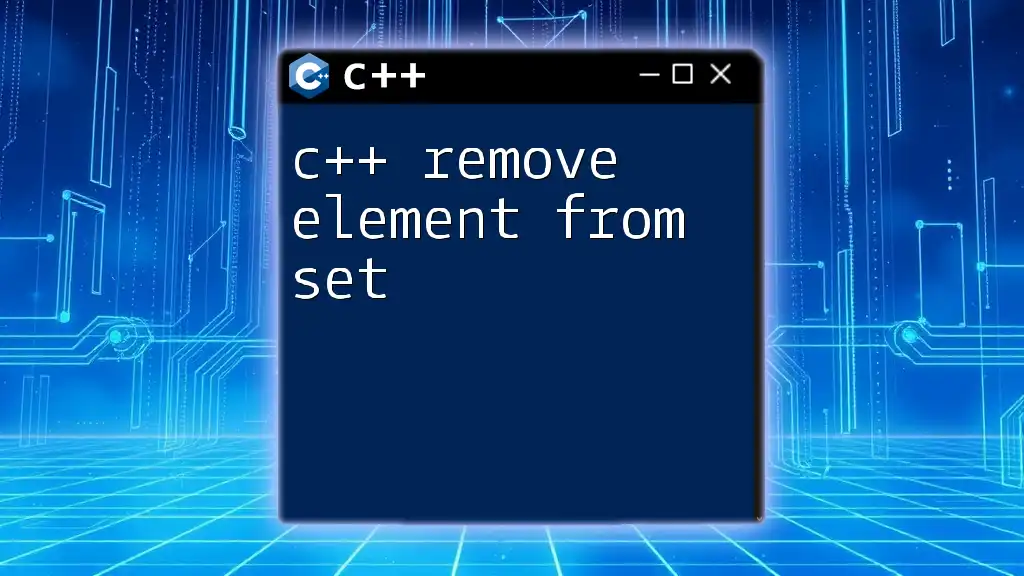
Understanding the Logical Operation of `remove`
How `remove` Works Internally
The `remove` function operates by iterating through the range specified, shifting elements to overwrite target values. As it does this, it creates a new logical state for the range. This means that all instances of the specified value are moved to the end of the container, while the valid entries remain at the beginning.
Performance Considerations
In terms of performance, the `remove` function is efficient with a time complexity of O(n) since it goes through the elements in a linear fashion. It’s essential to ensure that you choose `remove` when you need to eliminate specific elements, as opposed to implementing more complex methods like nested loops.
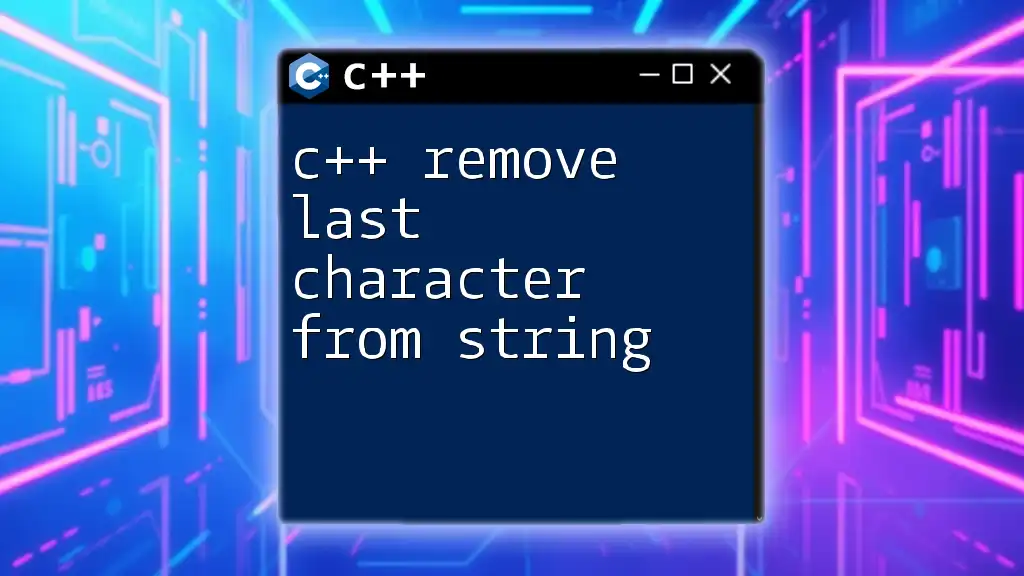
Successful Patterns and Common Pitfalls
Patterns to Follow
When using `remove`, it's beneficial to recognize patterns that can simplify coding tasks:
- Using `remove` with different types of containers, such as lists and arrays, can yield consistent results if the syntax is accurately followed.
- Combining `remove` with other STL algorithms, such as `sort` and `unique`, can enhance functionality and ensure efficient data management.
Common Mistakes
-
Forgetting to resize: One of the most common mistakes is neglecting to adjust the container’s size after using `remove`. Always remember to `erase` after a `remove`.
-
Misunderstanding operation: The `remove` function does not actually shrink the container; it only reorders the elements.
-
Confusing `remove` with `erase`: While they sound similar, `remove` only repositions elements and doesn’t erase them from the container.
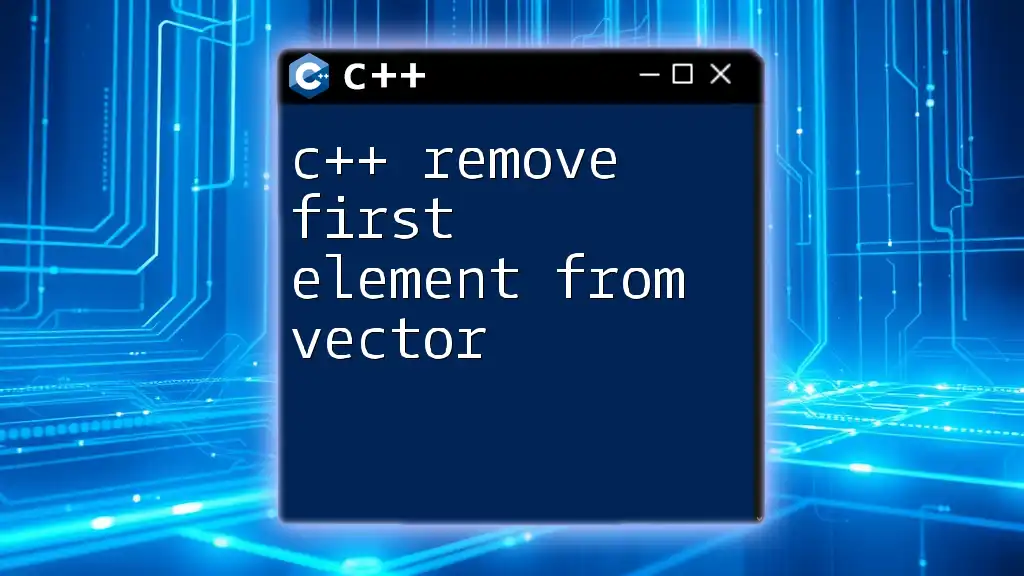
Alternatives to `remove`
`remove_if`
For cases where you need to remove elements based on a specific condition (rather than a single value), you can use the `remove_if` function. The syntax for `remove_if` is as follows:
std::remove_if(iterator first, iterator last, predicate);
How to Use `remove_if`
To illustrate how it works, consider the example below where we use `remove_if` to eliminate all even numbers from a vector:
std::vector<int> vec = {1, 2, 3, 4, 5};
auto new_end = std::remove_if(vec.begin(), vec.end(), [](int x) { return x % 2 == 0; });
vec.erase(new_end, vec.end());
Performance and Use Cases of `remove_if`
Just like `remove`, the `remove_if` function also operates in linear time O(n). It is particularly useful when you have a more complex condition for removal or when you need to eliminate multiple values.
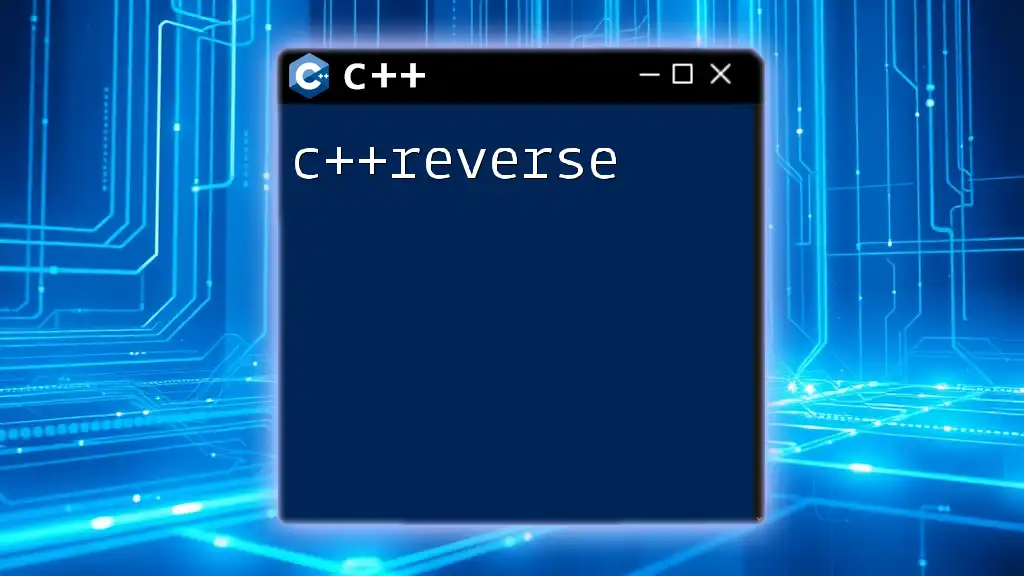
Conclusion
In conclusion, mastering the C++ `remove` function is essential for efficient data manipulation. Its ability to rearrange elements in a sequence without explicitly deleting them contributes to cleaner and more minimal code. Understanding both its use cases and how it operates internally can significantly enhance your ability to work with collections in C++.
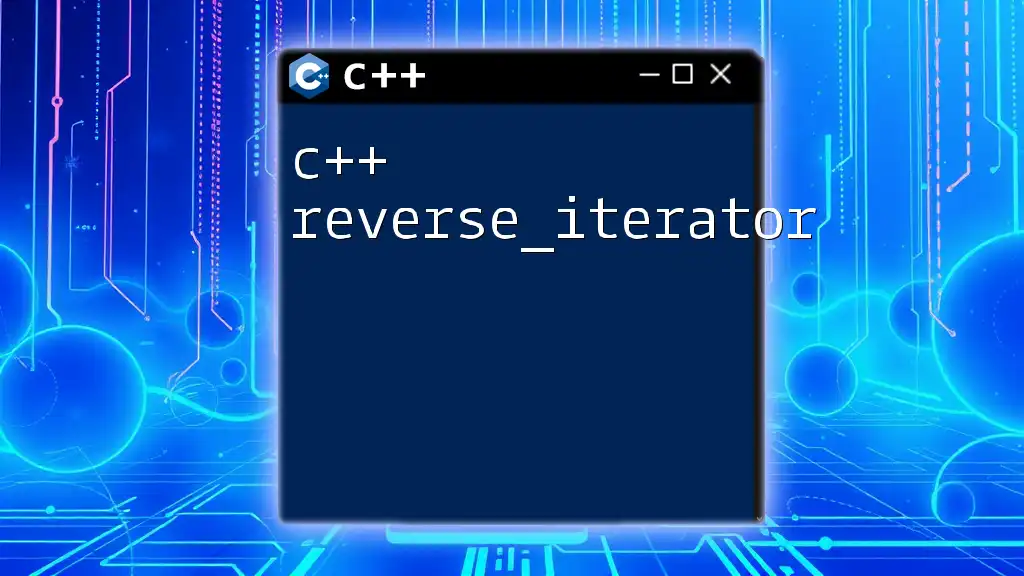
Additional Resources
For further learning, refer to the official C++ documentation. Engage in practice exercises to solidify your understanding, and consider building projects that implement the `remove` function to deepen your practical knowledge.
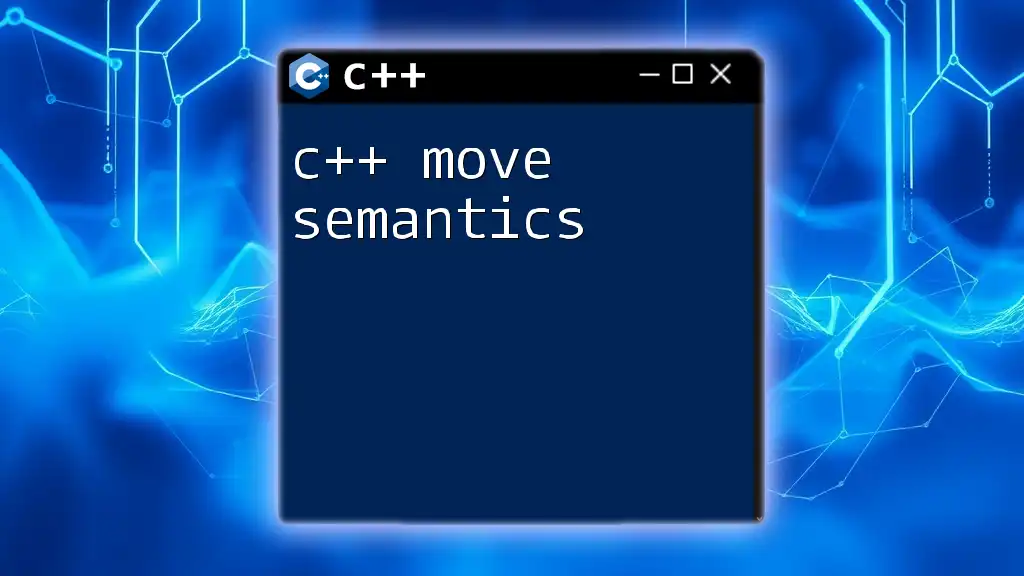
Call to Action
Take the time to implement the `remove` function in your own projects. Experiment with its mechanics, and dive deeper into combining it with other STL functionalities. With practice, you'll find it becomes an invaluable part of your C++ toolkit.