A C++ move constructor allows for the transfer of resources from a temporary object to a new object, significantly improving performance by avoiding unnecessary deep copies.
Here’s a simple example:
#include <iostream>
#include <utility> // for std::move
class MyClass {
public:
MyClass(int value) : data(new int(value)) {
std::cout << "Constructed with value: " << *data << std::endl;
}
// Move constructor
MyClass(MyClass&& other) noexcept : data(other.data) {
other.data = nullptr; // Leave other in a valid state
std::cout << "Moved value: " << *data << std::endl;
}
~MyClass() {
delete data; // Clean up
}
private:
int* data;
};
int main() {
MyClass obj1(42); // Regular construction
MyClass obj2(std::move(obj1)); // Move construction
return 0;
}
Understanding the Move Constructor in C++
A move constructor is a special constructor in C++ that allows the resources of an object to be moved from one instance to another. This is particularly useful when working with temporary objects, as it can significantly improve performance by eliminating unnecessary copying.
The primary difference between a copy constructor and a move constructor lies in how they handle resources. The copy constructor duplicates the content of the source object, while the move constructor transfers ownership of the resources without creating a copy. By using a move constructor, you can optimize resource management in classes that manage dynamic resources, such as heap-allocated memory.
The syntax for a move constructor typically looks like this:
ClassName(ClassName&& other) noexcept {
// Implementation
}
Here, `ClassName&&` signifies an rvalue reference, which allows the function to accept temporary objects that can safely relinquish their resources.

When to Use Move Constructor
Using a move constructor is highly beneficial in certain scenarios, particularly when dealing with large objects or resource-intensive operations. In situations where temporary objects are created and destroyed frequently, such as in functions that return large data structures, using a move constructor can drastically reduce overhead.
Performance Benefits: Move constructors help prevent multiple allocations and deallocations by allowing resources to be transferred directly. This can lead to significant performance improvements, particularly in applications requiring high efficiency, such as games or real-time simulations.
However, it is essential to be aware of common misconceptions. For instance, some developers may believe that move constructors are only applicable to standard library containers. In reality, any class can benefit from implementing a move constructor, especially when it handles resources like memory, file handles, or network connections.

Implementing a Move Constructor in C++
Implementing a move constructor is straightforward but requires careful consideration of resource management. Here's a basic implementation example:
class MyClass {
public:
int* data;
size_t size;
MyClass(size_t s) : size(s) {
data = new int[s]; // Resource allocation
}
// Move Constructor
MyClass(MyClass&& other) noexcept
: data(other.data), size(other.size) {
other.data = nullptr; // Nullifying the source object's pointer
other.size = 0; // Resetting size to avoid double free
}
~MyClass() {
delete[] data; // Resource deallocation
}
};
In this example, `MyClass` has a dynamic resource `data`. The move constructor transfers ownership of `data` from the source object (`other`) to the current object. After the transfer, `other.data` is set to `nullptr` to ensure that the destructor of the original object does not attempt to delete the same memory again.
Detailed Breakdown of the Implementation
- Moving Resources: When moving an object, you should transfer the resource pointers from the source object to the target object.
- Nullifying Source Pointers: It is crucial to set the source object’s pointers to `nullptr`. This prevents the resource from being freed multiple times, leading to potential crashes or undefined behavior.
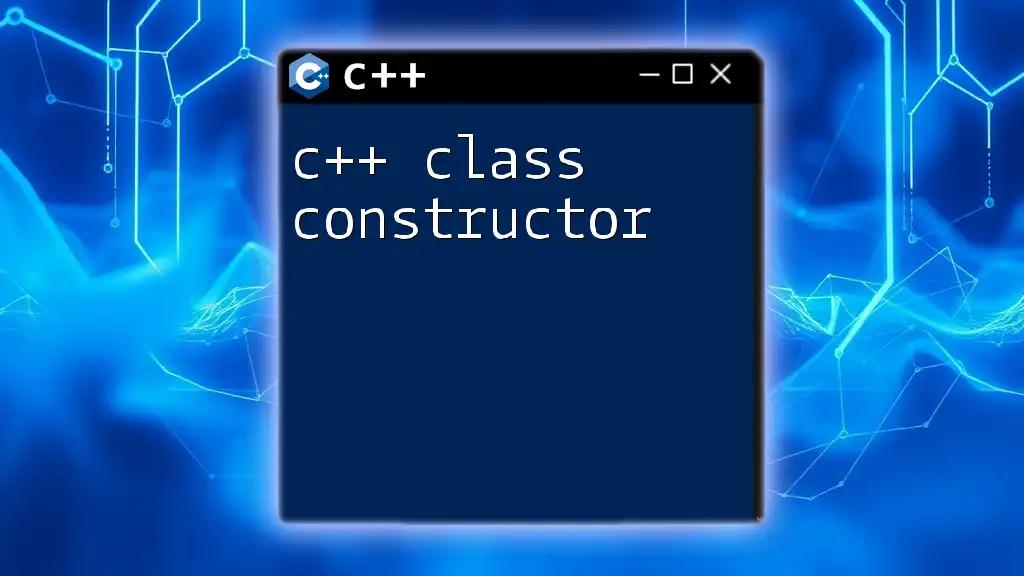
Practical Examples of Move Constructor
Example 1: Implementing Move Constructor for a Custom Class
Consider a custom class that manages a dynamic array:
class DynamicArray {
private:
int* data;
size_t size;
public:
DynamicArray(size_t s) : size(s) {
data = new int[s]; // Allocate dynamic memory
}
// Move Constructor
DynamicArray(DynamicArray&& other) noexcept
: data(other.data), size(other.size) {
other.data = nullptr; // Prevent double free
other.size = 0;
}
~DynamicArray() {
delete[] data; // Clean up
}
};
In this code snippet, `DynamicArray` implements a move constructor which enables efficient movement of its members while avoiding unnecessary allocations.
Example 2: Using std::vector with Move Constructor
The standard library container `std::vector` is designed to leverage move semantics. When a vector is moved, the internal pointers are switched instead of copying all elements:
#include <vector>
std::vector<int> vec1 = {1, 2, 3, 4, 5};
std::vector<int> vec2 = std::move(vec1); // Move semantics in action
In this example, `vec1` is moved into `vec2`. After the move, `vec1` is in a valid but unspecified state, where it holds no elements and cannot be safely relied upon.

Best Practices for Implementing Move Constructors
To ensure successful and efficient implementation of move constructors, consider these practices:
-
Avoid Self-Assignment: Always check for self-assignment within your move constructor to prevent issues. Although common in copy constructors, it's typically less relevant for move constructors. However, establishing a habit of writing robust code is advisable.
-
Resource Safety and Correctness: Always ensure that resources are correctly managed. Utilizing smart pointers like `std::unique_ptr` can simplify this, automatically managing memory and preventing leaks.
-
Copy-and-Swap Idiom: The copy-and-swap idiom combines the copy constructor and destructor to create a robust move constructor. This technique allows for strong exception safety guarantees.
class CopySwap {
private:
std::string str;
public:
CopySwap(std::string s) : str(std::move(s)) {}
CopySwap(CopySwap other) : str(std::move(other.str)) {} // Copy Constructor
CopySwap& operator=(CopySwap other) { // Copy-and-Swap
std::swap(str, other.str);
return *this;
}
};
In this example, even if an exception occurs during copying, the original object remains unchanged due to the swap.

Real-World Application of Move Constructors
In large data structures, move constructors are invaluable. For instance, in game development, often times high-performance buffers are created. Moving the large objects used in game states minimizes performance hits, ensuring smoother operations.
Additionally, frameworks that involve returning large objects can utilize move constructors to minimize resource duplication. For instance, returning complex data structures from functions can significantly profit from move semantics, leading to more efficient code execution.
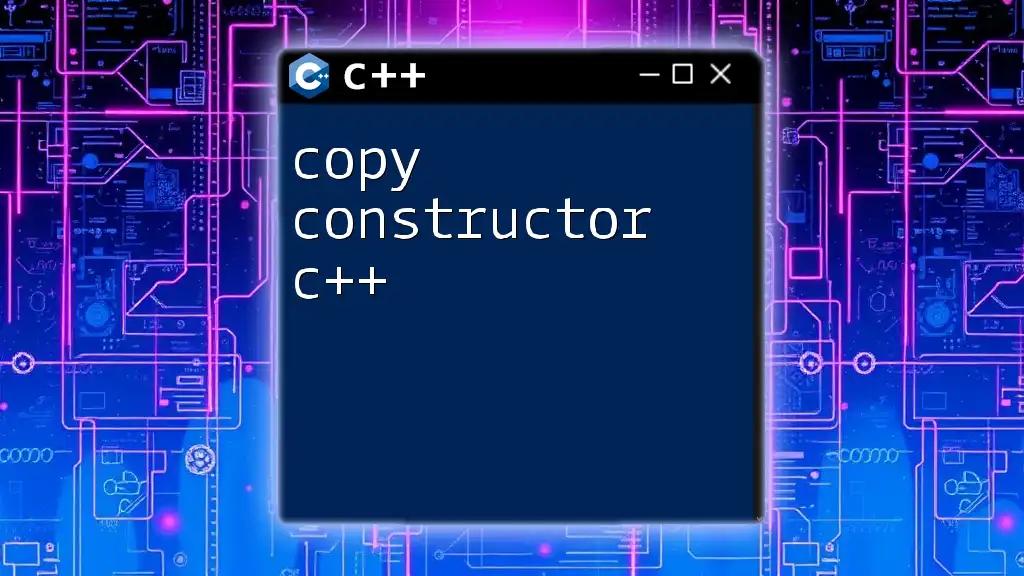
Troubleshooting Common Issues with Move Constructors
C++ move constructors can introduce complications, especially when errors arise. Common issues include:
- Failed Moves: If a move operation fails (e.g., during resource allocation), the state of the objects involved may become inconsistent. Implementing exception safety is critical.
- Debugging Tips: Use tools such as Valgrind or AddressSanitizer to uncover memory-related errors, which may indicate misuse of move constructors or incorrect resource management.

Conclusion
In summary, the C++ move constructor plays a crucial role in resource management and performance optimization in modern C++. Understanding its proper implementation and operation can lead to significant improvements, especially in applications that require efficient data handling. By using move semantics, you can create robust, performant classes that interact efficiently with both the C++ standard library and your custom resource managing classes.
The future of move semantics in C++ is vital to high-performance applications, encouraging developers to fully embrace these powerful tools to write cleaner, faster, and more efficient code.