A move constructor in C++ allows the transfer of resources from one object to another, enabling efficient management of dynamically allocated memory.
class MyClass {
public:
int* data;
// Constructor
MyClass(int value) {
data = new int(value);
}
// Move Constructor
MyClass(MyClass&& other) noexcept : data(other.data) {
other.data = nullptr; // Leave the moved-from object in a valid state
}
// Destructor
~MyClass() {
delete data;
}
};
What is a Move Constructor?
Definition and Purpose
A move constructor in C++ is a special type of constructor that enables the transfer of resources from one object to another. This is particularly useful for managing dynamic memory, allowing an object to "steal" the resources of a temporary object (an rvalue) rather than making a copy. The primary purpose of a move constructor is to improve performance and resource management by eliminating unnecessary deep copies.
Importance in Modern C++
Move constructors are crucial in modern C++ programming, primarily introduced with C++11. They allow developers to write more efficient code by utilizing move semantics, which can significantly reduce the overhead associated with object copying. Unlike copy constructors, which create a copy of an object and allocate new resources, move constructors transfer ownership of existing resources. This leads to enhanced performance—especially in cases involving complex data structures and large objects.
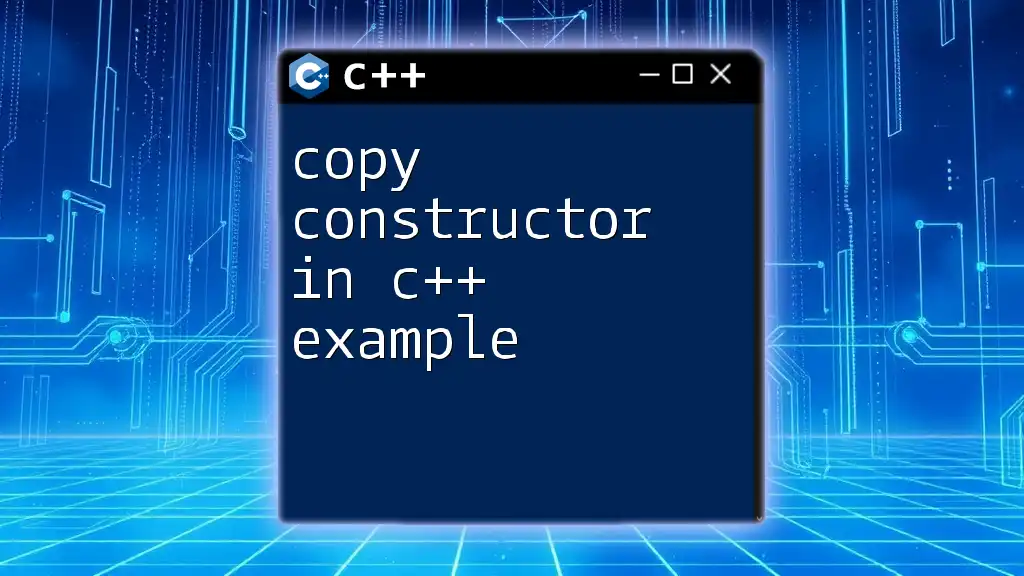
Move Constructor Syntax
Basic Structure
The basic syntax for defining a move constructor uses rvalue references, which are indicated with `&&`. Here's how the syntax looks:
ClassName(ClassName&& other);
Example: Implementing a Move Constructor
To illustrate, consider a simple class that implements a move constructor:
class MyString {
public:
MyString(char* data); // Constructor
MyString(MyString&& other); // Move constructor
// Other members...
};
In this example, `MyString` contains a dynamically allocated string. The move constructor will allow this class to efficiently transfer ownership of that string when a temporary instance is involved.
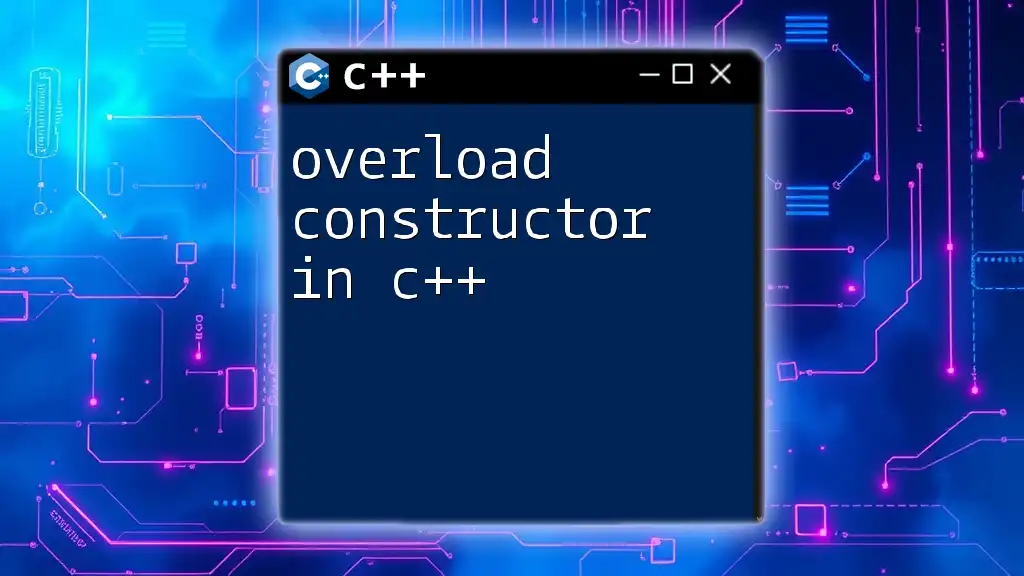
How Move Constructors Work
The Mechanics of Moving
During a move operation, the move constructor takes an rvalue reference to another object. It transfers the resource ownership by moving the pointers (or references) instead of copying the data, which is generally a more efficient operation. This is achieved by implementing the following:
- The member variables of the object being moved (the `other` object) are assigned to the current object (the new instance).
- The member variables of the `other` object are then set to a safe state, preventing them from being destructed when the `other` object is destroyed.
The Concept of "Stealing" Resources
When using a move constructor, we can think of the operation as stealing resources. Moving effectively vacates the source object, allowing the destination object to take control of the original resources without duplicating them. This is not only more memory efficient but also time efficient, as allocation and deallocation operations are reduced.
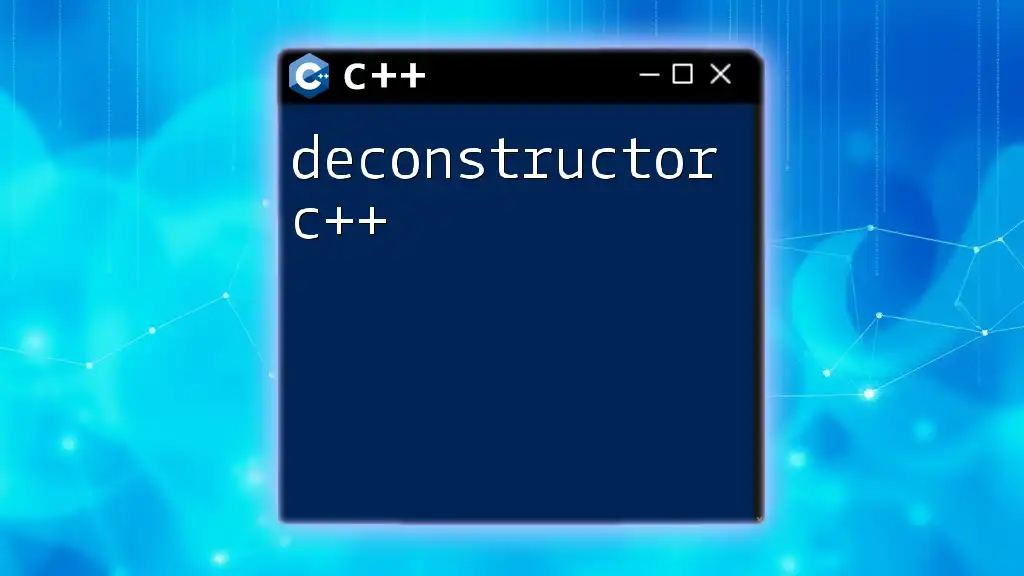
Implementing a Move Constructor
Step-by-Step Guide
The implementation of a move constructor is straightforward. Let’s create a more detailed example below, which explains each part:
class MyString {
private:
char* data; // Pointer to the string data
public:
// Constructor
MyString(char* data) : data(data) {}
// Move constructor
MyString(MyString&& other) noexcept {
// Steal the resources
this->data = other.data;
other.data = nullptr; // Leave the moved-from object in a valid state
}
// Destructor
~MyString() {
delete[] data; // Clean up the allocated memory
}
// Other members...
};
In this implementation, the move constructor takes an rvalue reference to `other`. The `data` pointer is transferred, and the `other.data` is set to `nullptr`, which ensures that when `other` is destroyed, it won't attempt to delete the same memory twice.
Best Practices
It is essential to leave the moved-from object in a valid state after transferring its resources. This helps avoid undefined behavior during subsequent operations on the moved-from object. Notably, handling self-assignment correctly is vital—although it's less of a concern for move constructors than for copy constructors.
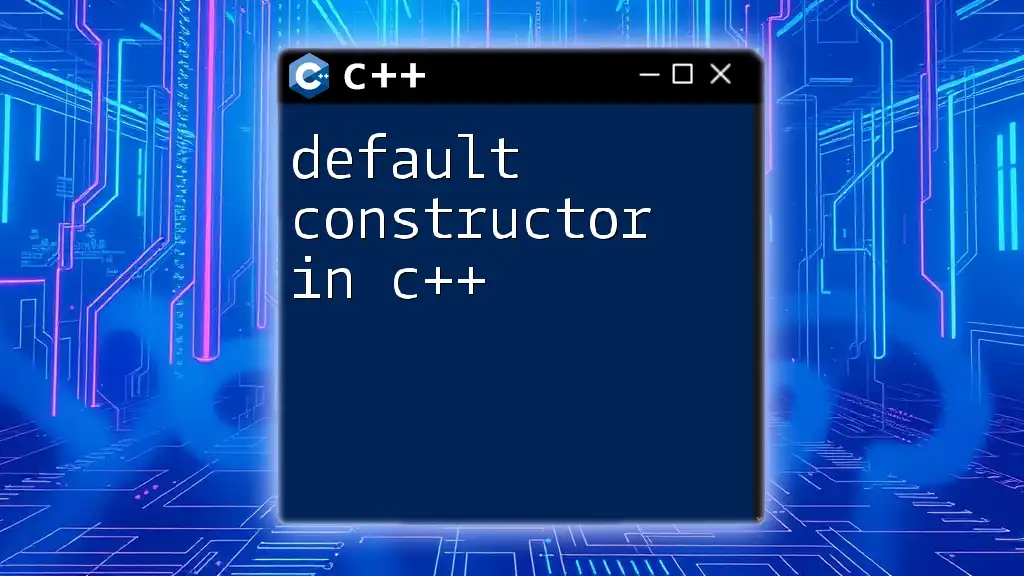
Advantages of Move Constructors
Performance Improvements
Move constructors offer significant performance benefits by reducing the number of copies made in resource management. For instance, when using containers like `std::vector`, instead of copying an object when resizing or transferring, a move constructor can be invoked, allowing the original data to be moved effortlessly and quickly.
Memory Management
With move semantics, we gain better control over dynamic memory. By efficiently transferring ownership, we minimize the risk of memory bloat and improve resource allocation, leading to a more efficient and faster application.
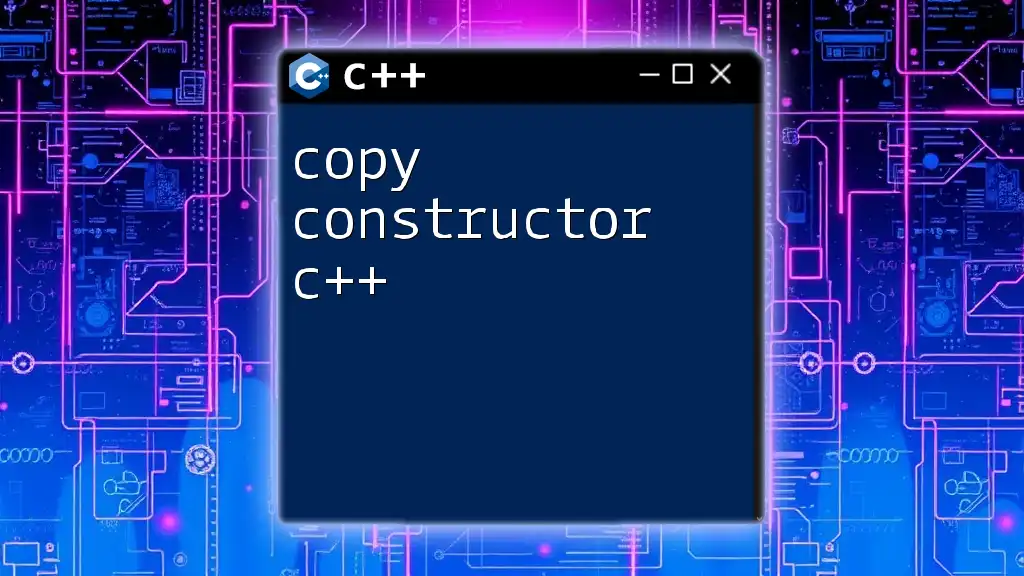
When to Use Move Constructors
Ideal Use Cases
Move constructors are particularly beneficial in scenarios that involve:
- Temporary objects: When an object is defined and immediately passed to a context where it can be consumed (such as returning objects from functions).
- Standard library containers: Using standard templates like `std::vector` or `std::map`, which often involve reallocating or restructuring internal data, makes move semantics ideal.
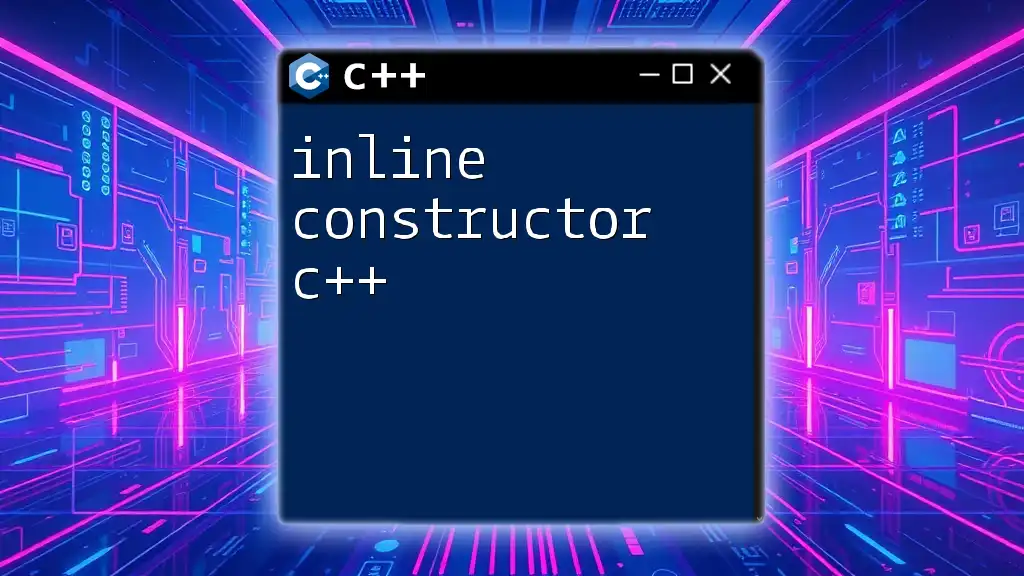
Common Mistakes and Pitfalls
Common Errors to Avoid
When implementing move constructors, some common pitfalls include:
- Not nullifying moved-from pointers: This can lead to double deletion errors if the destructor is called on the moved-from object.
- Forgetting the `noexcept` specifier: Declaring the move constructor as `noexcept` can optimize performance, as it allows the compiler to make additional guarantees about its behavior.
Debugging Move Constructors
If you encounter issues with resource management, consider verifying the state of both the moved-from and the moved-to objects. Utilizing logging or assertions can help confirm that resources are being managed correctly and provide insights into any unexpected behaviors.
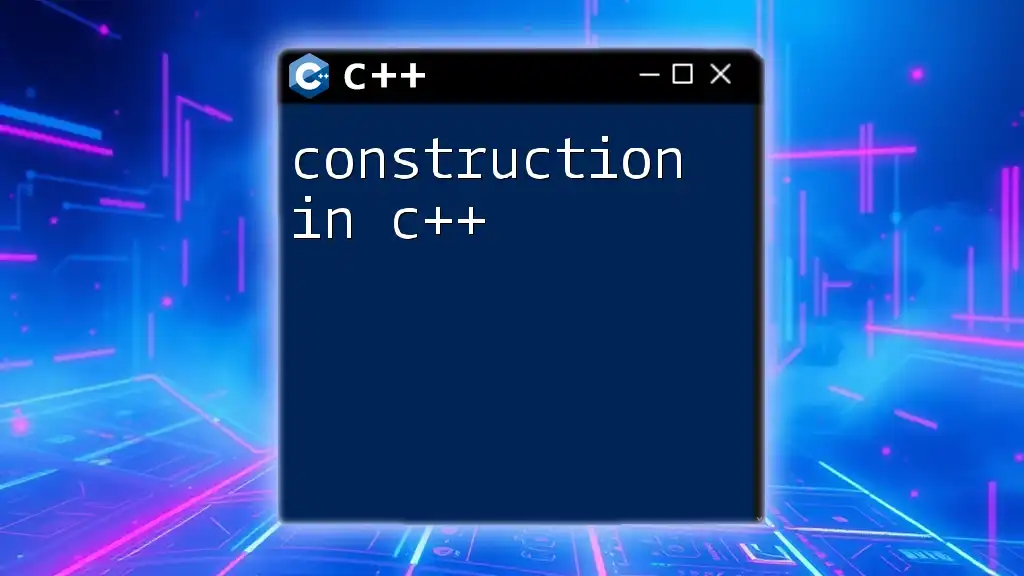
Conclusion
Recap of Key Points
Move constructors in C++ are a powerful feature introduced with C++11 that allow for efficient resource management through move semantics. By transferring ownership of resources, they enhance performance and streamline memory management.
Encouragement to Implement
If you’re looking to develop more efficient applications, leverage move constructors in your projects. By utilizing them, you can ensure your applications run faster and manage memory more effectively.
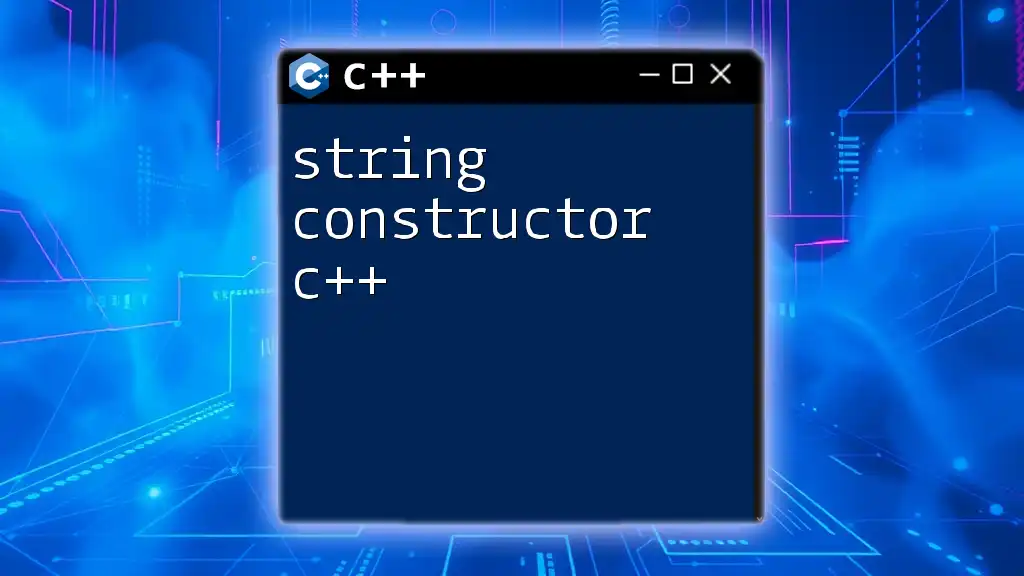
Additional Resources
Learning and Reference Materials
For those interested in diving deeper into move semantics, consider exploring the following resources:
- Books: Look for C++ programming books that focus on C++11 and beyond.
- Online Tutorials: Websites and courses dedicated to advanced C++ programming.
- Documentation: Refer to the official C++ documentation for a comprehensive understanding of move constructors and their proper implementation.