A copy constructor in C++ is a special constructor that initializes a new object as a copy of an existing object.
#include <iostream>
using namespace std;
class Example {
public:
int value;
// Copy constructor
Example(const Example &obj) {
value = obj.value;
}
Example(int val) : value(val) {}
};
int main() {
Example obj1(42); // Create an object
Example obj2 = obj1; // Use copy constructor
cout << obj2.value; // Output: 42
return 0;
}
Understanding the Basics of Copy Constructors
Definition of a Copy Constructor
In C++, a copy constructor is a special constructor that initializes a new object as a copy of an existing object. The syntax for defining a copy constructor is as follows:
ClassName(const ClassName &obj);
The parameter is a reference to an object of the same class type. This allows you to create an instance of a class based on another instance.
Two types of copy constructors exist:
-
Default Copy Constructor: C++ provides a compiler-generated copy constructor when you do not provide your own. It performs a member-wise copy, which might not be suitable for classes that manage dynamic resources.
-
User-defined Copy Constructor: You define this type of constructor to tailor the copying mechanism, especially when the class includes pointers or dynamically allocated memory.
Characteristics of Copy Constructors
Understanding how copy constructors work in different scenarios is crucial.
-
Shallow Copy vs. Deep Copy: A shallow copy duplicates the object's data members as they are. In scenarios where data members are pointers, both the original and the copied object will point to the same memory address. A deep copy, on the other hand, allocates new memory and copies the value to ensure that changes to one object do not affect the other.
-
Copy Constructor Overloading: You can have multiple copy constructors that accept different types or numbers of parameters, but this is rare and may lead to confusion. Ensure the class semantics remain clear.
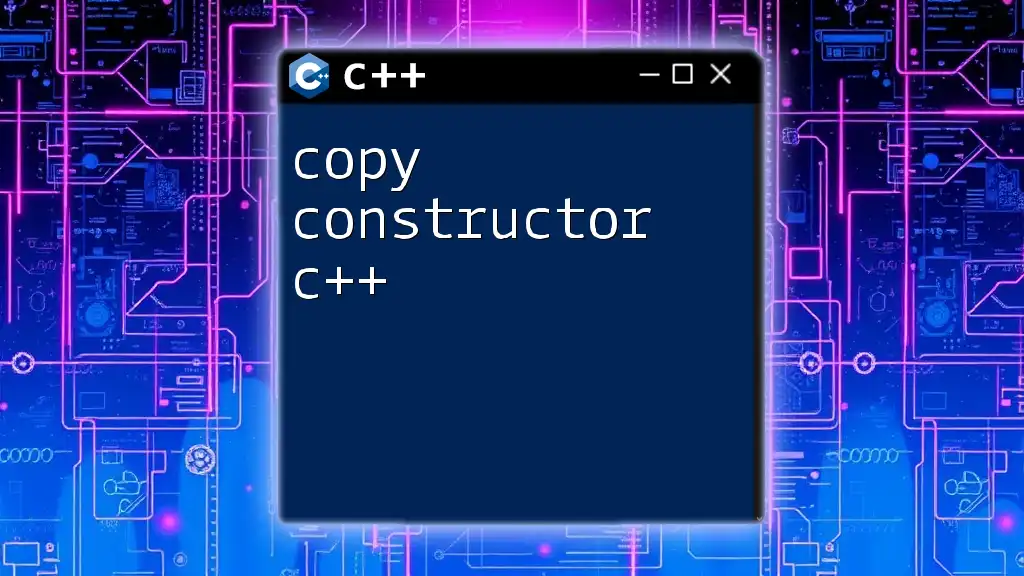
Example of Copy Constructor in C++
Simple Copy Constructor Example
Here’s a basic implementation of a copy constructor in a class:
class MyClass {
public:
int data;
// Copy Constructor
MyClass(const MyClass &obj) {
data = obj.data; // Copy data
}
};
Explanation
In the above example, the `MyClass` class has one data member, `data`. The copy constructor enables cloning of an instance of `MyClass`. When a new object is created from an existing object, the copy constructor is invoked. The member `data` of the original object is copied to the new instance:
MyClass obj1;
obj1.data = 42;
// Object obj2 is created using the copy constructor
MyClass obj2 = obj1; // obj2.data now equals 42
This demonstrates a simple copy operation using the copy constructor.
Copy Constructor with Dynamic Memory Allocation
When dealing with dynamic resources, it is vital to implement a deep copy. This ensures that each instance manages its own separate memory. Here’s an advanced implementation:
class MyDynamicClass {
public:
int* arr;
int size;
// Constructor
MyDynamicClass(int s) {
size = s;
arr = new int[size]; // Dynamic allocation
}
// Copy Constructor
MyDynamicClass(const MyDynamicClass &obj) {
size = obj.size;
arr = new int[size]; // Allocate new memory
for (int i = 0; i < size; i++) {
arr[i] = obj.arr[i]; // Deep Copy
}
}
// Destructor
~MyDynamicClass() {
delete[] arr; // Free allocated memory
}
};
Explanation
In this example, the `MyDynamicClass` class manages an integer array. The constructor allocates memory for `arr` based on the specified size. In the copy constructor, we perform a deep copy by allocating new memory for `arr` and copying the contents of the original array. This is essential to prevent both objects from pointing to the same memory address, avoiding accidental data corruption and memory leaks.
The destructor ensures that memory allocated to `arr` is released when the object is destroyed.
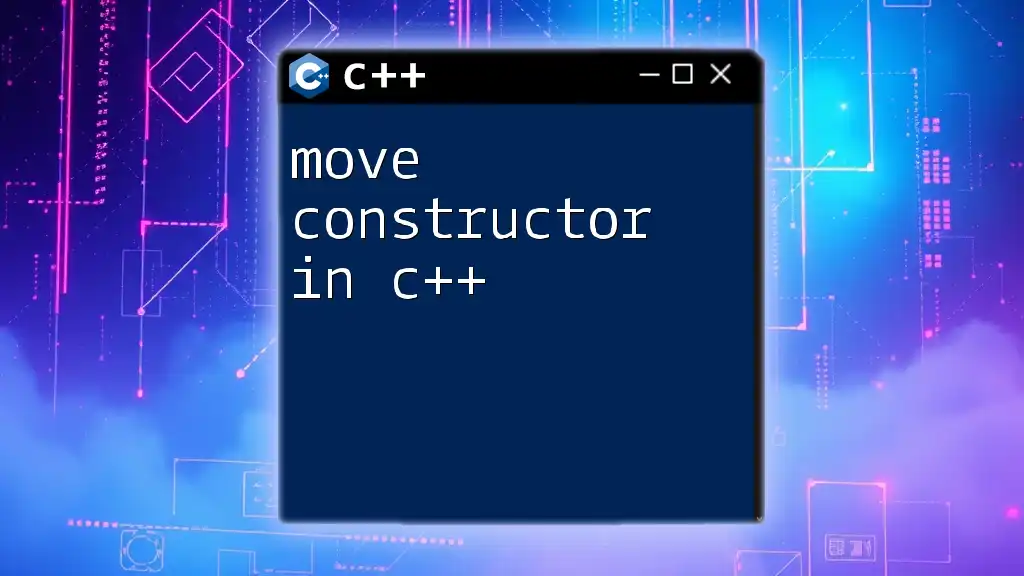
Common Mistakes with Copy Constructors in C++
Forgetting to Implement a Copy Constructor
If a class does not explicitly define a copy constructor, the C++ compiler automatically generates one. However, this might not suffice when the class handles raw pointers or external resources. Failing to implement a custom copy constructor can lead to shallow copies and potential data issues.
Copying Resources Incorrectly
Incorrect implementations can occur when copying resources, especially if managing dynamic memory. Shallow copies can lead to unexpected behavior, where changes to one object reflect in another, resulting in data inconsistency and crashes.
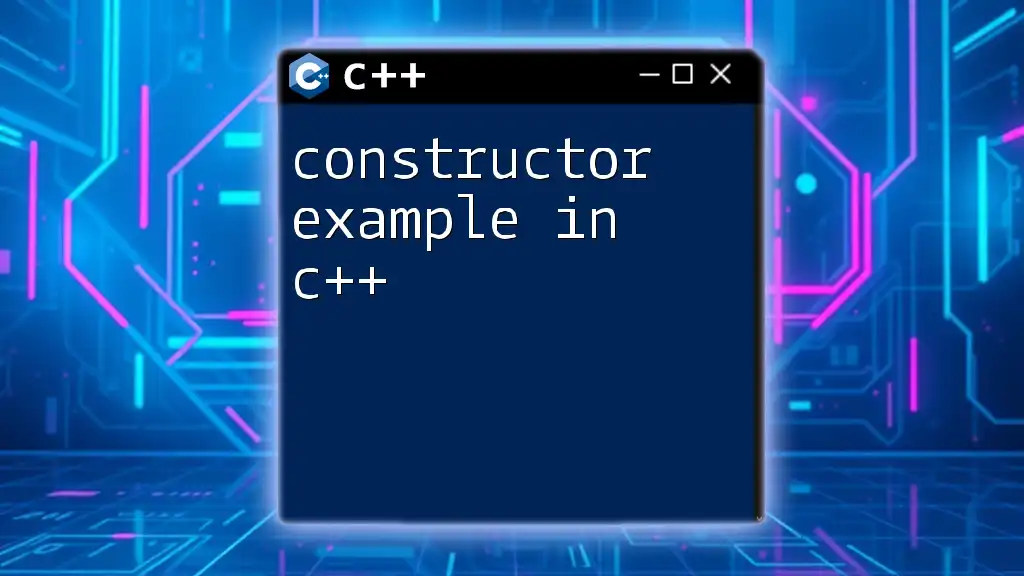
Best Practices for Using Copy Constructors in C++
When to Use a Copy Constructor
- Use when a class contains pointers, references, or resources that require deep copying.
- If you have complex classes that need custom initialization upon copying, a copy constructor is essential.
Key Takeaways for Effective Copy Constructor Implementation
- Always ensure to implement a copy constructor if your class contains dynamic memory or is managing resources.
- Make use of deep copies to prevent unintended side effects when copying objects.
- Thoroughly test your copy constructor to confirm that it correctly replicates the intended object's state without causing memory leaks or data corruption.
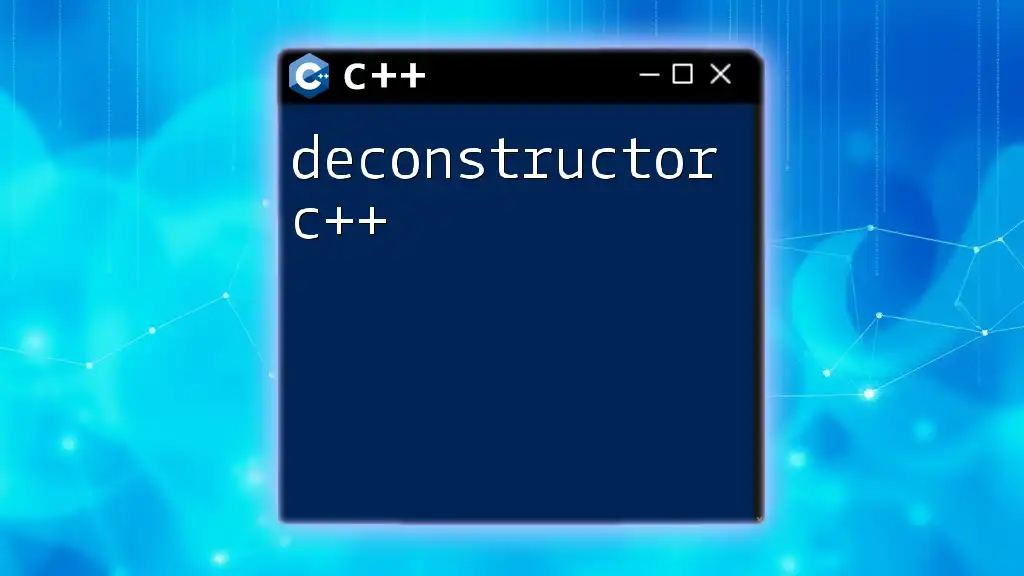
Conclusion
Understanding the intricacies of the copy constructor in C++ example is pivotal for any developer dealing with object-oriented programming. A good grasp of copy constructors helps in creating robust and efficient C++ applications, managing resources smartly, and ensuring that your program behaves as expected. Continuous learning and practice will contribute to refining your skills in effectively utilizing copy constructors and object management in C++.
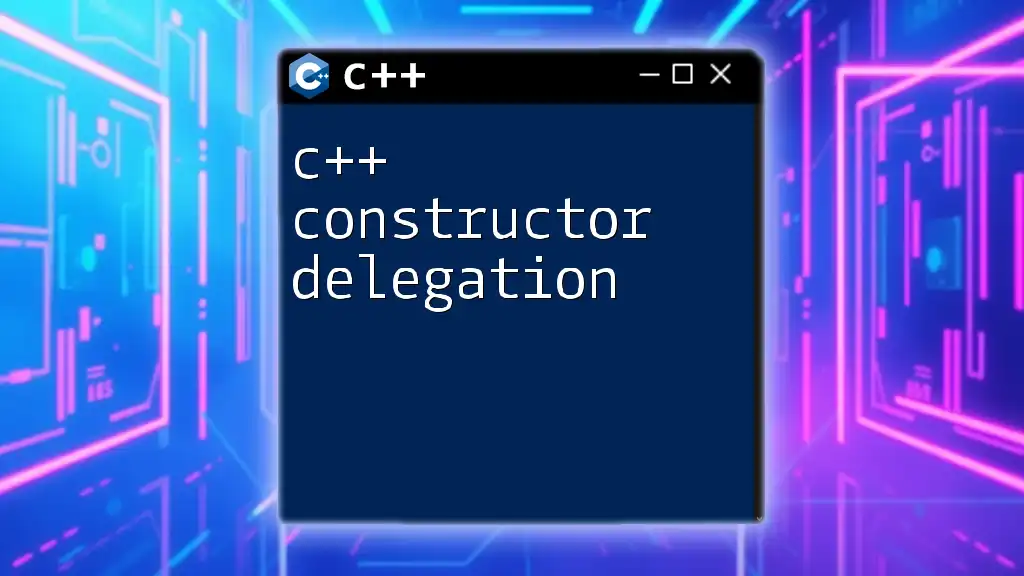
Additional Resources
Recommended Books and Websites
- Books on C++ Programming, such as "Effective C++" by Scott Meyers.
- Online courses and platforms like Codecademy and Coursera for in-depth tutorials.
Practice Problems
Seek out suggested problems that involve implementing and testing copy constructors, and leverage coding platforms like LeetCode or HackerRank for hands-on experience.