C++ constructors are special member functions that are automatically called when an object of a class is created, allowing for the initialization of objects with specific values.
class MyClass {
public:
int value;
MyClass(int v) : value(v) {} // Constructor initializes 'value'
};
MyClass obj(10); // Creates an object 'obj' with 'value' initialized to 10
Understanding C++ Constructors
What is a Constructor in C++?
A constructor in C++ is a special member function that is automatically called when an object of a class is created. The primary purpose of a constructor is to initialize the object's attributes with valid values. Unlike regular member functions, constructors have the same name as the class and do not have a return type. This distinctive feature allows the compiler to identify them quickly during the object creation process.
Syntax for Constructor in C++
The basic syntax for declaring a constructor is straightforward. It follows the class name and does not require a return type. Here’s a simple example of the syntax:
class MyClass {
public:
MyClass() {
// constructor body
}
};
In this example, `MyClass` has a constructor named `MyClass`, clearly indicating that it initializes `MyClass` instances.
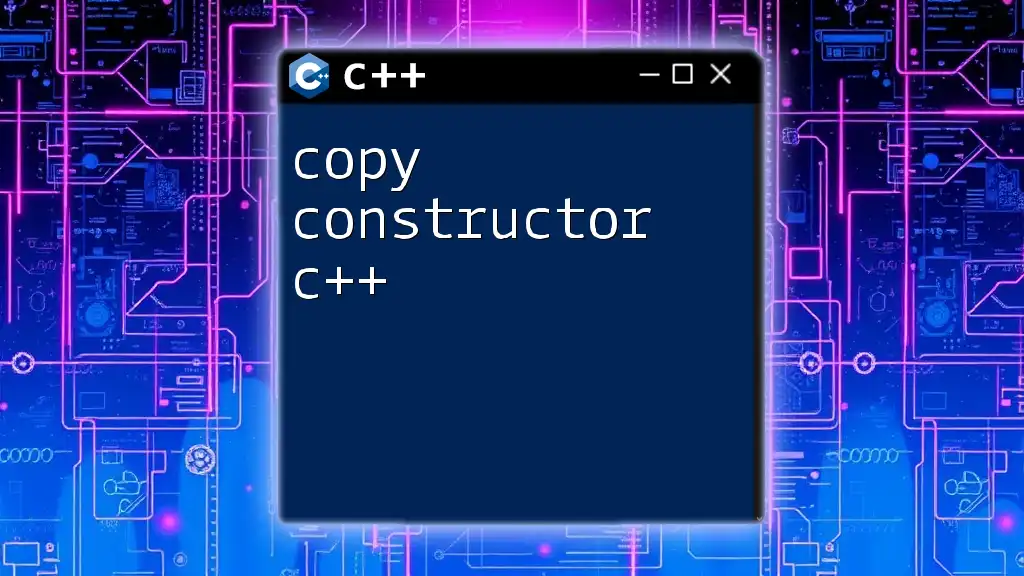
Types of Constructors in C++
Default Constructor
A default constructor is a constructor that can be called with no arguments. If no constructor is explicitly defined, the compiler provides a default constructor that initializes member variables to default values (0, null, etc.).
When you implement a default constructor, you can set up specific default values for your object's attributes. Here’s an example:
class Point {
public:
int x, y;
Point() { // Default constructor
x = 0;
y = 0;
}
};
In this code snippet, every instance of `Point` will start with x and y initialized to zero.
Parameterized Constructor
Parameterized constructors allow you to pass arguments to the constructor at the time of object creation. This feature enables you to initialize an object with specific values right away. Here's how you can implement a parameterized constructor:
class Point {
public:
int x, y;
Point(int xVal, int yVal) { // Parameterized constructor
x = xVal;
y = yVal;
}
};
Point p(10, 20); // Creates a Point object with x = 10, y = 20
In this code, the `Point` class can now create objects with any x and y values you choose, enhancing flexibility.
Copy Constructor
A copy constructor is used to create a new object as a copy of an existing object. If you do not provide a copy constructor, C++ automatically generates one for you that performs a shallow copy (bitwise copy of the object's memory). However, a shallow copy may not be sufficient for some classes using pointers for dynamic memory allocation. Here’s an example:
class Point {
public:
int* x;
Point(int val) {
x = new int(val);
}
// Custom copy constructor
Point(const Point &p) {
x = new int(*p.x); // deep copy
}
~Point() {
delete x; // destructor to free resources
}
};
In this example, the custom copy constructor ensures deep copying of the dynamic memory rather than just copying the pointer.
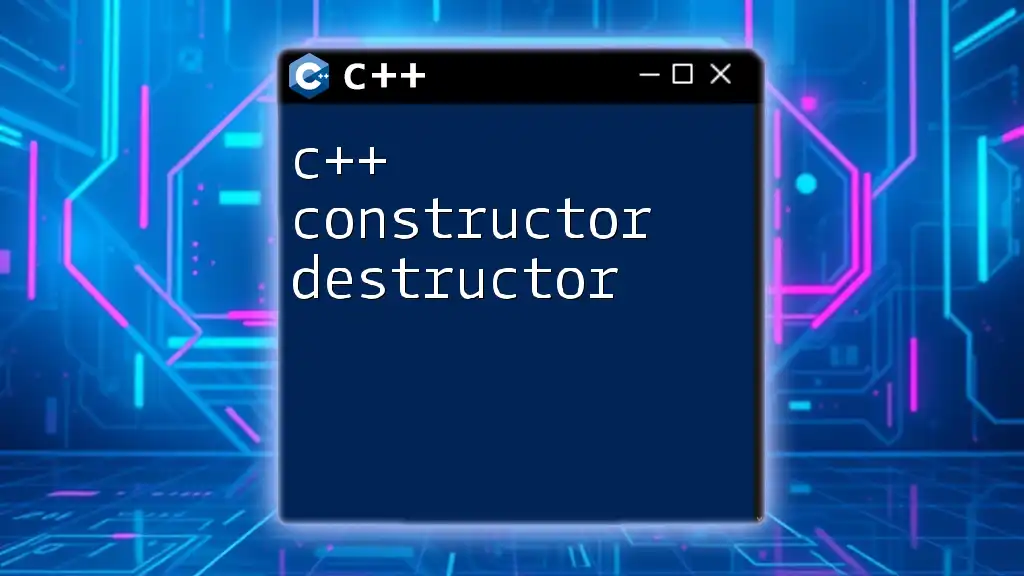
Special Characteristics of Constructors
Constructor Overloading
Constructor overloading is the ability to create multiple constructors within the same class, each with different signatures (parameter lists). This feature gives flexibility in object creation. Here’s an example of overloaded constructors:
class Rectangle {
public:
int length, width;
Rectangle() { // Default constructor
length = 1;
width = 1;
}
Rectangle(int l, int w) { // Parameterized constructor
length = l;
width = w;
}
};
Rectangle r1; // Calls default constructor
Rectangle r2(10, 5); // Calls parameterized constructor
In this case, `Rectangle` can be initialized using either the default constructor or the parameterized constructor based on the requirements.
Constructor Initialization Lists
Using initialization lists is a preferred way to initialize class members, especially when those members are constants or references. An initialization list improves performance by preventing multiple assignments. Here's how you can do it:
class Circle {
public:
const double pi;
double radius;
Circle(double r) : pi(3.14), radius(r) { // Initialization list
}
};
In this example, `pi` is initialized directly with the value `3.14`, showcasing the efficiency and clarity of initialization lists.
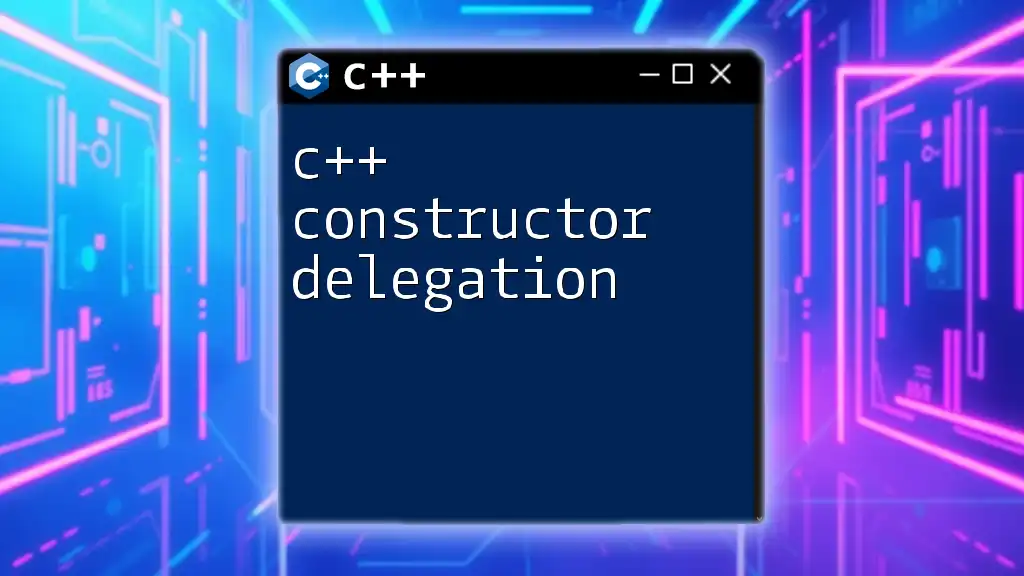
Special Cases in Constructors
Constructors and Dynamic Memory
When using dynamic memory within a constructor, it’s essential to manage that memory properly to prevent memory leaks. This typically involves allocating memory in the constructor and freeing it in the destructor. See the following example:
class Array {
public:
int* arr;
int size;
Array(int s) {
size = s;
arr = new int[size]; // allocating memory
}
~Array() {
delete[] arr; // freeing memory
}
};
In this case, the `Array` constructor allocates memory for an array of integers, and the destructor ensures the memory is released, preventing memory leaks.
Destructor vs. Constructor
While constructors initialize an object, destructors are called when an object goes out of scope or is deleted, serving to clean up. It’s crucial to implement both to manage resources effectively. Here’s a simple example:
class MyClass {
public:
MyClass() {
// constructor actions
}
~MyClass() {
// cleanup actions
}
};
In this example, `MyClass` uses a destructor to perform necessary cleanup when an instance is destroyed.
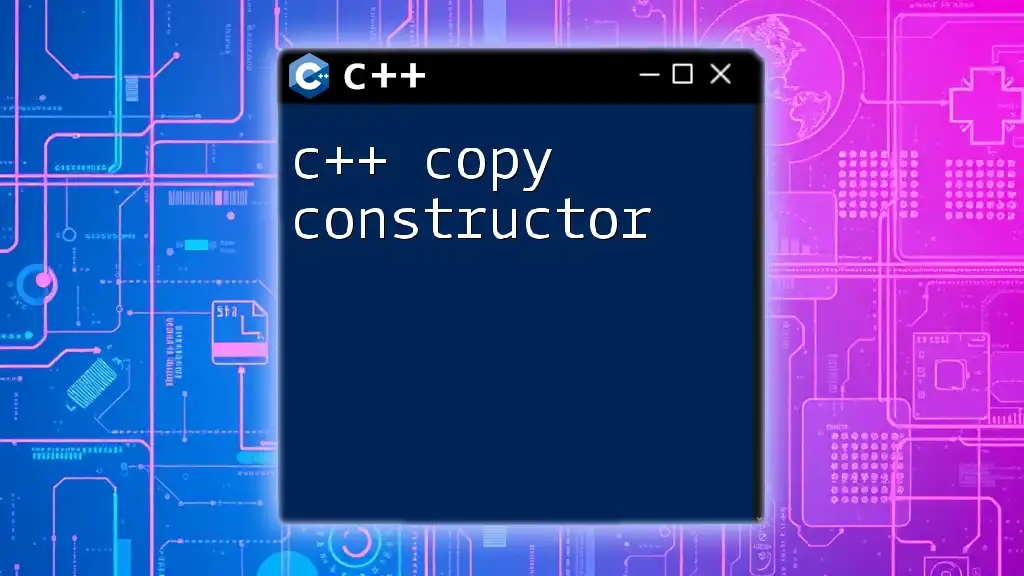
Common Errors Related to C++ Constructors
Forgetting to Define a Constructor
If you create a class without defining a constructor and attempt to form an object, C++ will generate a compiler-defined default constructor. However, without understanding what the compiler does, you may unintentionally create objects with uninitialized member variables. Always define constructors intentionally to avoid surprises.
Copy Constructor Issues
Another common mistake arises from the automatic shallow copy behavior of the default copy constructor. If you have dynamically allocated memory within your class, not providing your own copy constructor can lead to serious issues like double deletion, where two objects attempt to delete the same memory allocation.
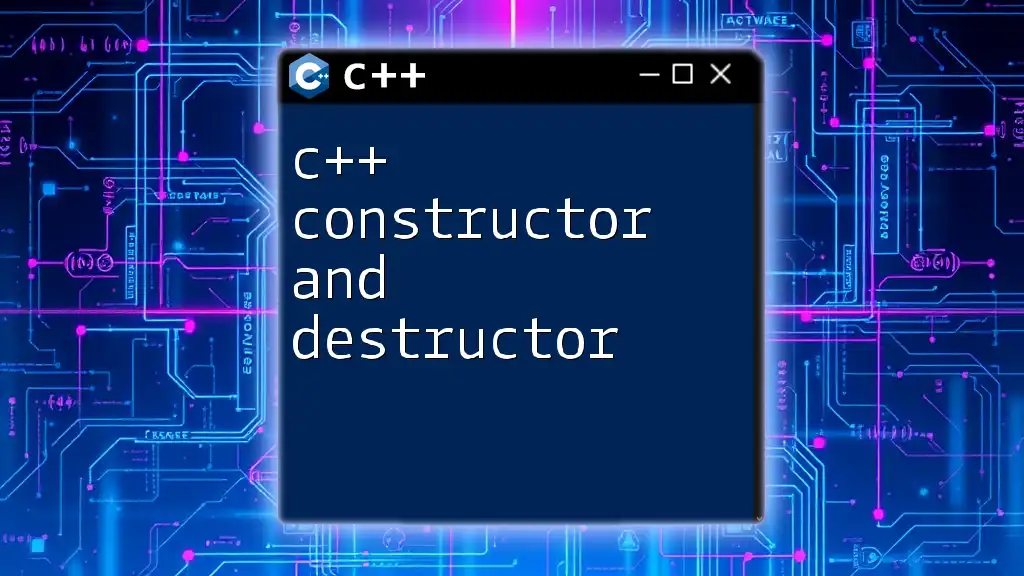
Best Practices for Using Constructors in C++
- Define constructors explicitly: Always define what your constructor should do to initialize your class objects effectively.
- Use initialization lists: When possible, prefer initialization lists over assignment in the constructor body.
- Implement a destructor: If your constructor allocates memory dynamically, always provide a corresponding destructor to free that memory.
- Be cautious with copies: Implement custom copy constructors and assignment operators as needed to prevent shallow copies, especially for classes involving dynamically allocated resources.
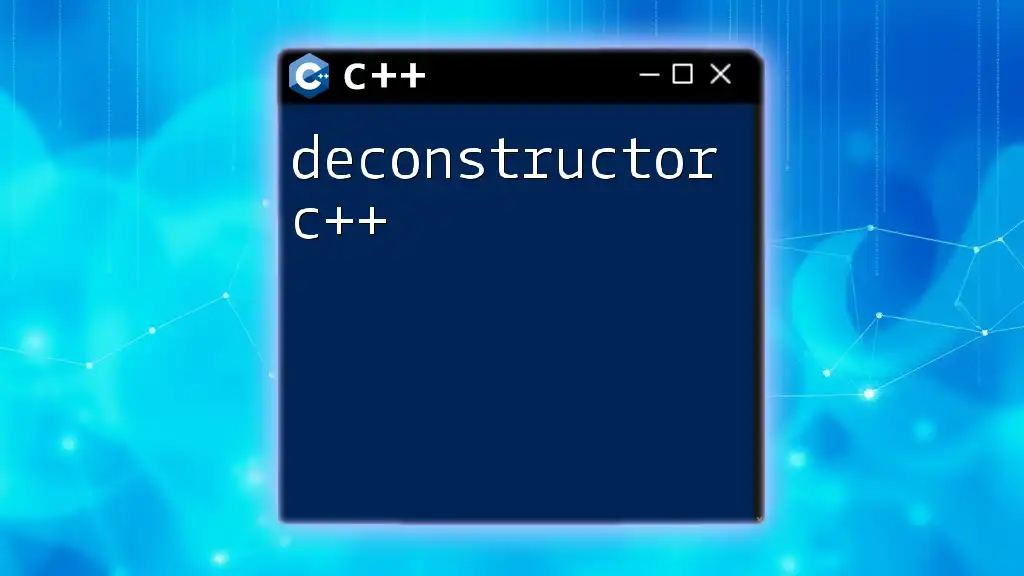
Conclusion
C++ constructors are an essential feature that helps developers manage the initialization of class objects. By understanding and mastering default, parameterized, and copy constructors, along with their special characteristics, you can significantly enhance your C++ programming skills. Practice is key—try creating various classes using different types of constructors to grasp their full potential. For those looking to dive deeper, there are numerous resources available to explore the breadth of C++ programming.
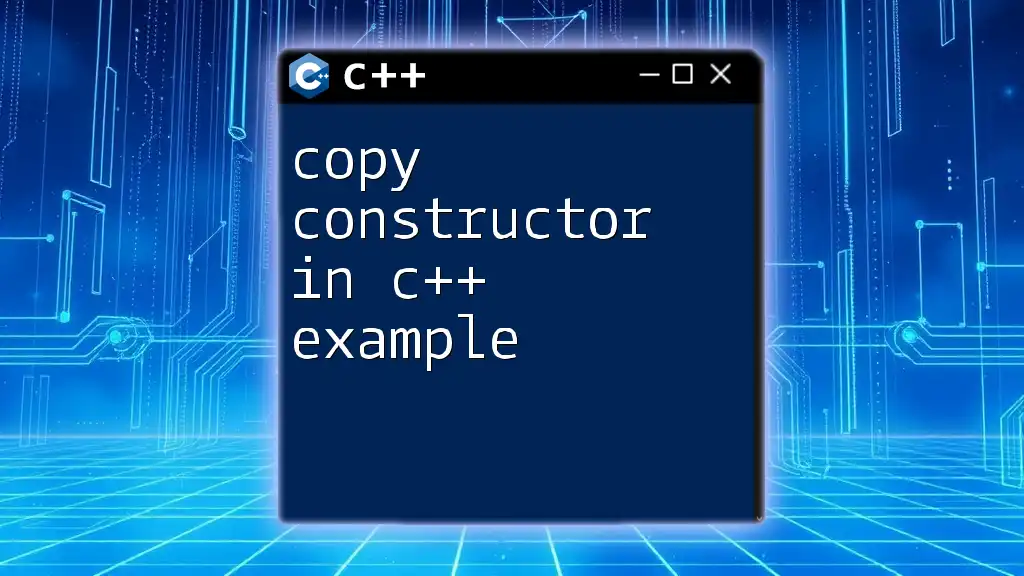
Additional Resources
To further enhance your understanding of C++ and its constructors, consider looking up the official C++ documentation. Books like "C++ Primer" or online courses can also provide in-depth knowledge and additional examples.
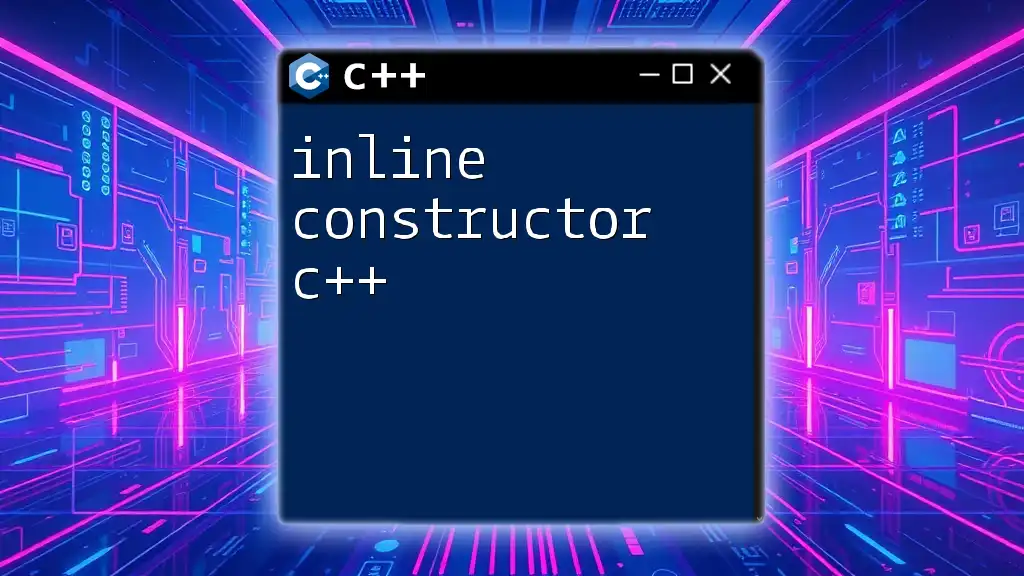
Call to Action
If you have any questions or comments about C++ constructors, feel free to leave them below! Don't forget to share this article with others interested in learning about C++ programming.