A C++ class constructor is a special member function that is automatically called when an object of the class is created, allowing you to initialize its properties.
class MyClass {
public:
MyClass(int value) {
this->value = value; // constructor initializes the 'value' member
}
private:
int value;
};
What is a Constructor in C++?
A C++ class constructor is a special member function that is executed automatically whenever an object of the class is created. Constructors are primarily responsible for initializing an object’s attributes and allocating resources as necessary. One of the defining characteristics of constructors is that they share the same name as the class they belong to and do not have a return type.
Difference Between Constructors and Regular Member Functions
Unlike regular member functions which can be called explicitly, constructors cannot be called explicitly. They are called by the compiler when an object is created. Regular member functions can return values, while constructors do not return anything, not even `void`. This distinct nature makes constructors vital for object-oriented programming in C++.
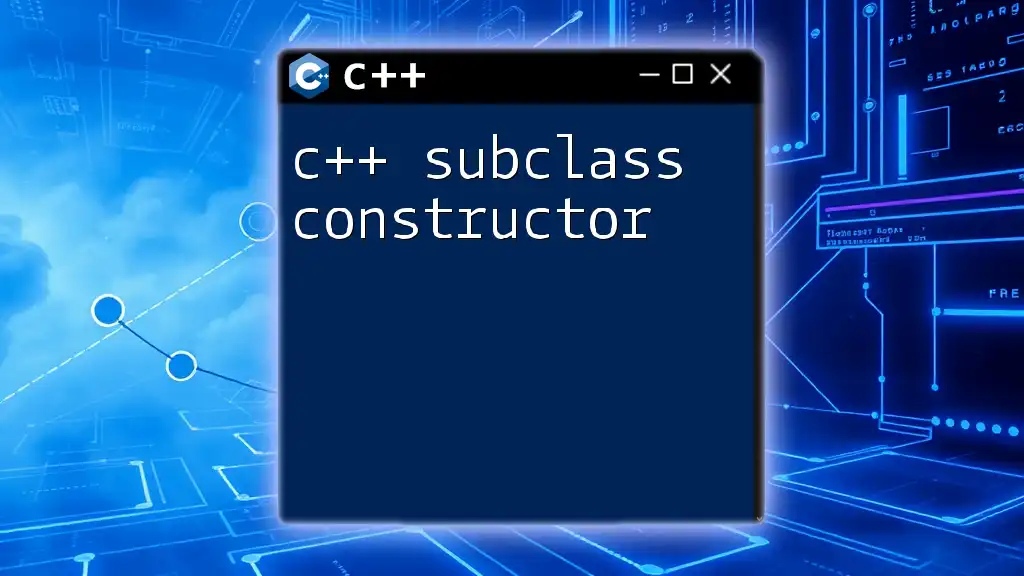
Why Use Constructors in C++?
Using constructors is essential for ensuring that your objects are in a valid state before they are used. Here are some key reasons to utilize constructors:
-
Importance of Initialization: Constructors ensure that all member variables of a class are initialized correctly, protecting against undefined behavior which can occur from using uninitialized variables.
-
Automatic Memory Management: In C++, particularly with dynamic memory, constructors can manage resource allocation efficiently, reducing the risk of memory leaks by pairing them with destructors to free resources.
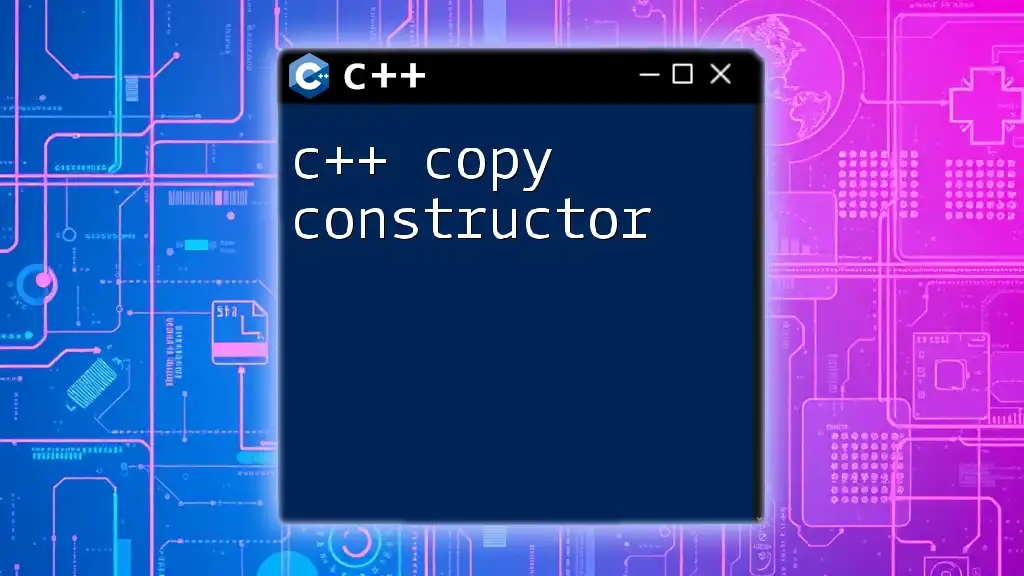
Types of Constructors in C++
Default Constructor
A default constructor is a constructor that takes no arguments or all of its parameters have default values. It’s useful for creating objects without needing to provide explicit initialization parameters.
class Example {
public:
Example() {
// initialization code
}
};
Parameterized Constructor
A parameterized constructor allows you to initialize an object with specific values provided as parameters. This is useful when you want to create an object with customized properties from the outset.
class Example {
public:
int x;
Example(int val) {
x = val;
}
};
Copy Constructor
A copy constructor is used to create a new object as a copy of an existing object. This is particularly important for managing resources in a class that involves dynamic memory allocation.
class Example {
public:
int x;
Example(const Example &obj) {
x = obj.x;
}
};
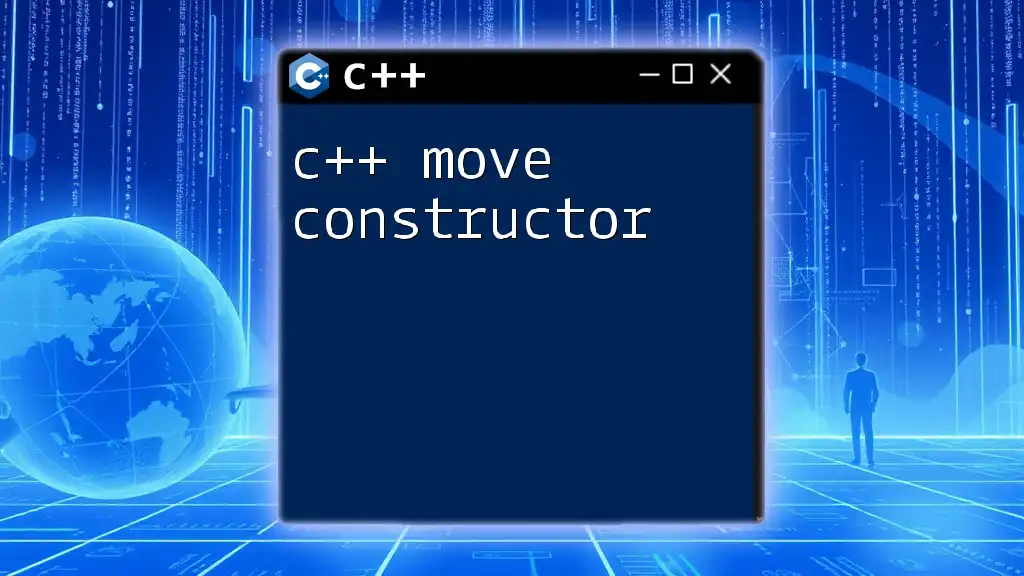
How Constructors Work in C++
Constructor Overloading
Constructor overloading allows you to define multiple constructors with different parameter lists in the same class. This enables flexible object initialization.
class Example {
public:
Example() { /* no params */ }
Example(int val) { /* param */ }
};
Initialization Lists
Initialization lists are used to initialize member variables before the constructor body executes. This is particularly effective for constants and reference types.
class Example {
public:
int a, b;
Example(int x, int y) : a(x), b(y) { }
};
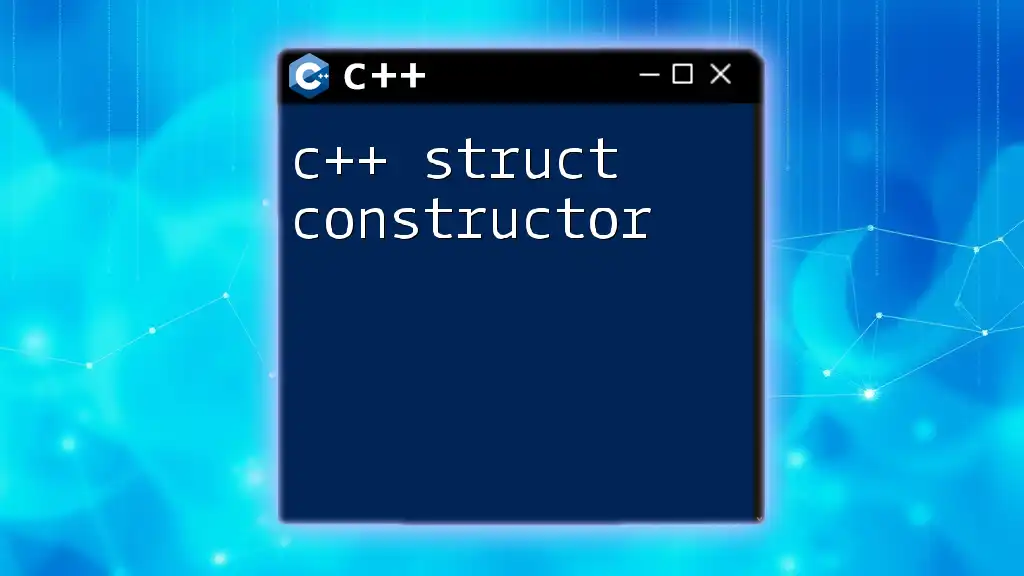
Practical Examples of C++ Class Constructors
Basic C++ Constructor Example
Here’s a straightforward example to illustrate the usage of a C++ class constructor:
class Point {
public:
int x, y;
Point(int a, int b) : x(a), y(b) {}
};
int main() {
Point p(10, 20); // Object p is initialized with x=10, y=20
return 0;
}
Using Constructors in Resource Management
In C++, constructors play a crucial role in resource management. They help implement the RAII (Resource Acquisition Is Initialization) principle effectively, ensuring resources are allocated and deallocated properly.
class Array {
public:
int* arr;
int size;
Array(int s) : size(s), arr(new int[s]) {} // Constructor allocating memory
~Array() { delete[] arr; } // Destructor freeing memory
};
int main() {
Array myArray(10); // Creates an array of size 10
return 0; // Automatically cleans up memory when myArray goes out of scope
}
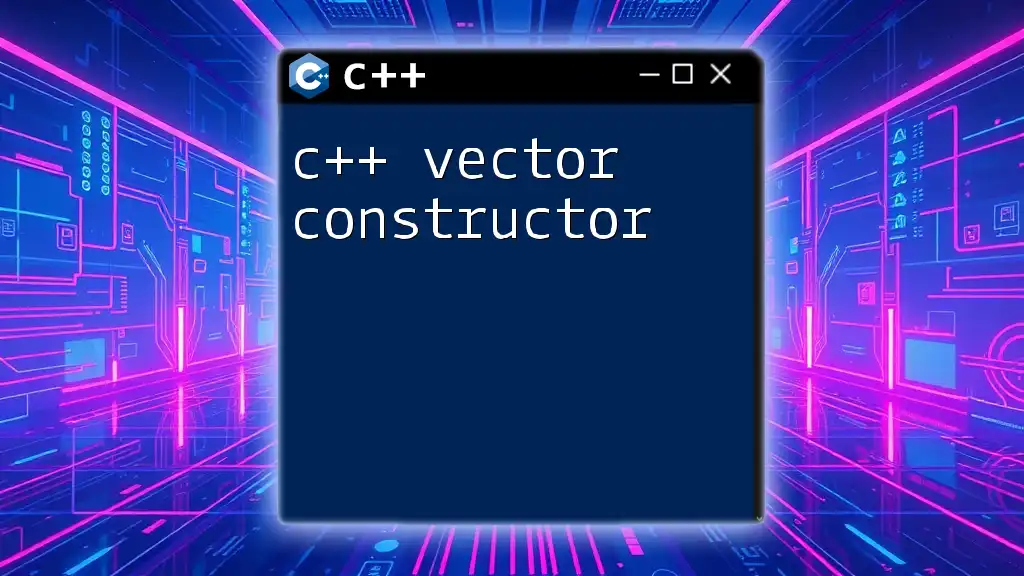
Best Practices for Using Constructors in C++
Avoiding Memory Leaks
To prevent memory leaks, always ensure that you pair resource allocation in the constructor with corresponding deallocation in the destructor. If you allocate memory in a constructor, deallocate it in the destructor.
Const Correctness
Using `const` in constructors and member functions ensures that they do not modify the state of the object. This practice enhances the safety and predictability of your code.
class Example {
public:
int value;
Example(int val) : value(val) {}
int getValue() const { return value; } // This function cannot modify the object state
};
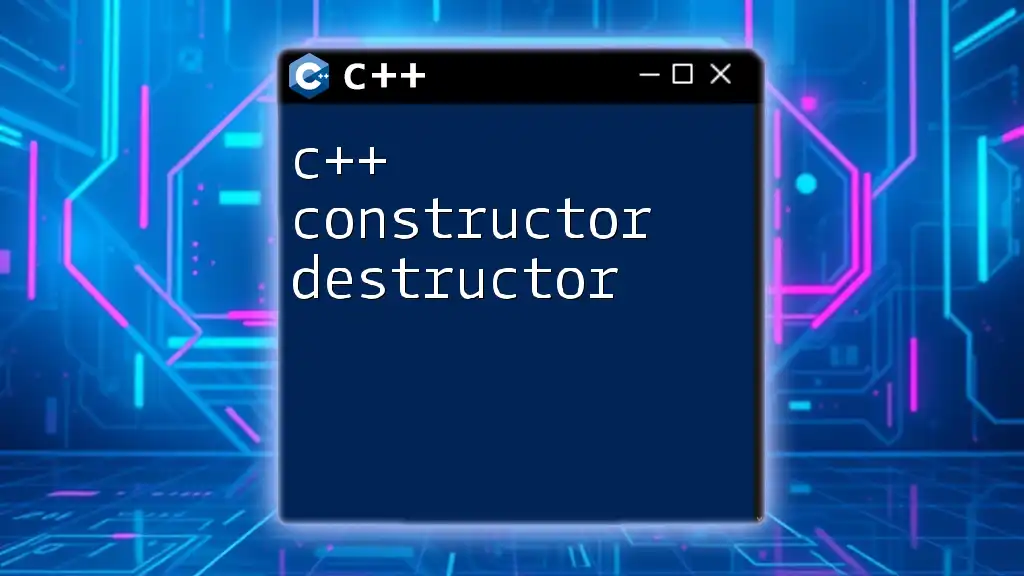
Common Mistakes to Avoid
Forgotten Initializations
One common mistake is failing to initialize member variables. Accessing uninitialized variables can lead to unexpected behavior and hard-to-track bugs.
Incorrect Overloading
Ambiguous constructor calls can arise from incorrect overloading. Ensure that constructors’ parameter types distinguish them clearly.
Copy Elision and RVO (Return Value Optimization)
Understanding copy elision and RVO can significantly optimize your code. Many compilers optimize away unnecessary copying of objects, making operations faster.
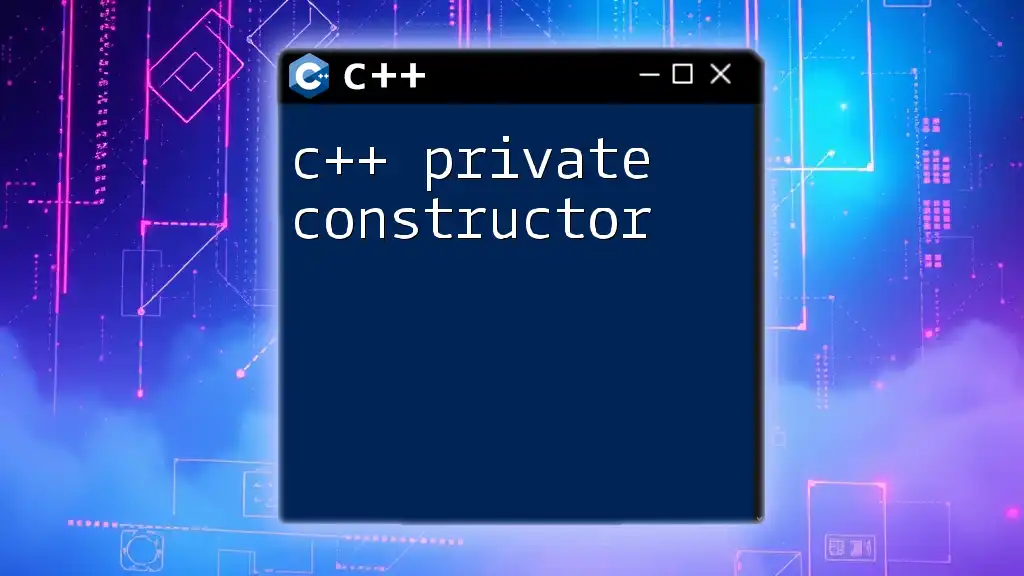
Conclusion
In summary, the C++ class constructor is a fundamental concept that provides automatic initialization of objects. Understanding the types of constructors and best practices for their use enables developers to write robust and efficient C++ programs. It’s essential to practice these concepts in real-world scenarios, ensuring the development of well-structured and maintainable code. Embrace the design principles that constructors offer, and continue exploring resources to deepen your knowledge of C++ programming!
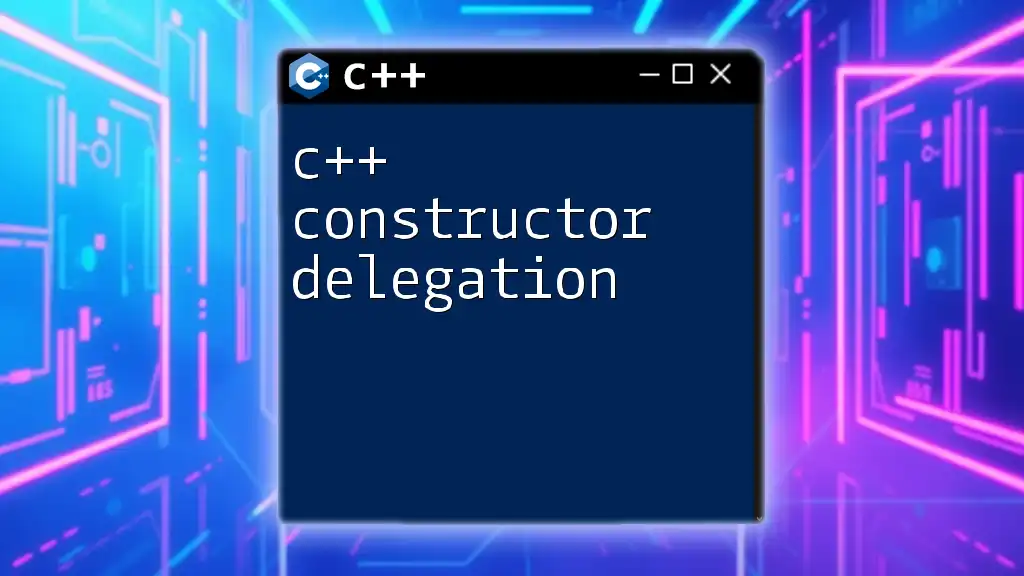
Additional Resources
For further learning, consider exploring books and tutorials specific to C++, engaging in online communities, or examining sample code repositories to see practical implementations of C++ class constructors and other advanced features.