In C++, multiple constructors allow a class to have more than one constructor method to initialize objects in different ways depending on the parameters provided.
Here’s an example of multiple constructors:
class Box {
public:
Box() { // Default constructor
width = 1;
height = 1;
}
Box(int w, int h) { // Parameterized constructor
width = w;
height = h;
}
void display() {
std::cout << "Width: " << width << ", Height: " << height << std::endl;
}
private:
int width, height;
};
int main() {
Box box1; // Calls default constructor
Box box2(5, 10); // Calls parameterized constructor
box1.display();
box2.display();
return 0;
}
Understanding the Basics of Constructors
Default Constructor
A default constructor is a special member function that is automatically invoked when an object of the class is created. It initializes the members of the class to default values, which are typically zero for built-in types or equivalent to calling the default constructor for user-defined types.
Here's a simple code snippet to illustrate a default constructor:
class Example {
public:
Example() {
// Initialization code.
}
};
Parameterized Constructor
A parameterized constructor takes arguments and allows users to provide specific values for initializing object members. This provides flexibility in creating objects with different initial states.
For example:
class Example {
public:
Example(int parameter) {
// Initialization code with parameter.
}
};
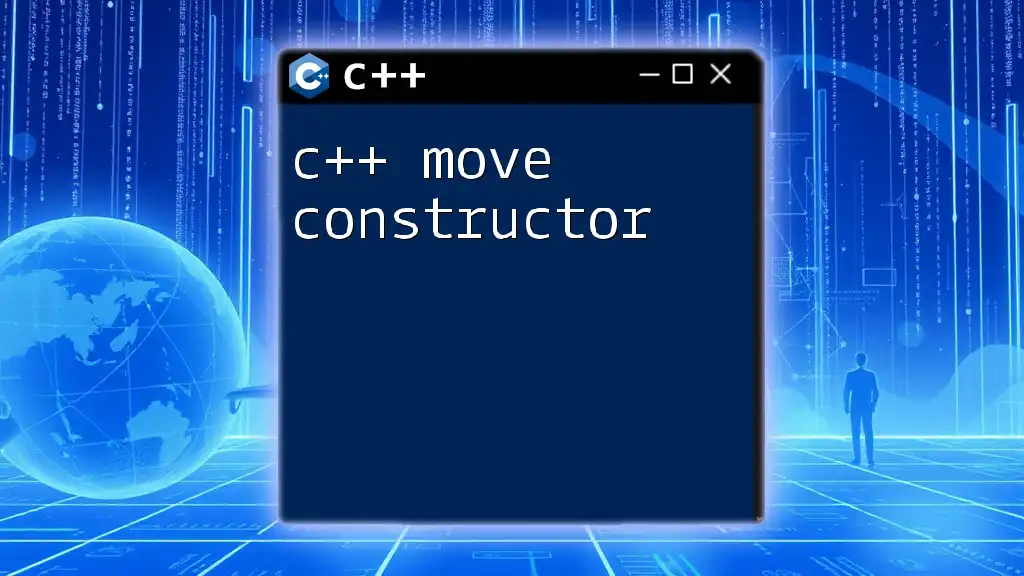
Exploring Multiple Constructors
Constructor Overloading
Constructor overloading enables a class to have more than one constructor, distinguished by the number or type of parameters. This is a powerful feature that allows for various initializations of objects depending on the context in which they are created.
When you overload constructors, you offer various ways to create an object, giving users the flexibility to use the constructor best suited for their needs.
Example of Constructor Overloading
Consider the class `Box`, which can be initialized in different ways to represent various box shapes:
class Box {
public:
Box() { /* Default constructor */ }
Box(double width, double height) { /* Parameterized constructor */ }
Box(double side) { /* Constructor for square box */ }
};
In this example, the class `Box` can be instantiated with no arguments (default), with two arguments (width and height), or with one argument (for a square).
Use Cases of Multiple Constructors
Multiple constructors are beneficial in various contexts, such as creating objects from different sets of information. Different instances can represent various aspects of a domain model. For example, an `Employee` might be created with just a name, or a name and an ID, depending on how much information is available at that point.
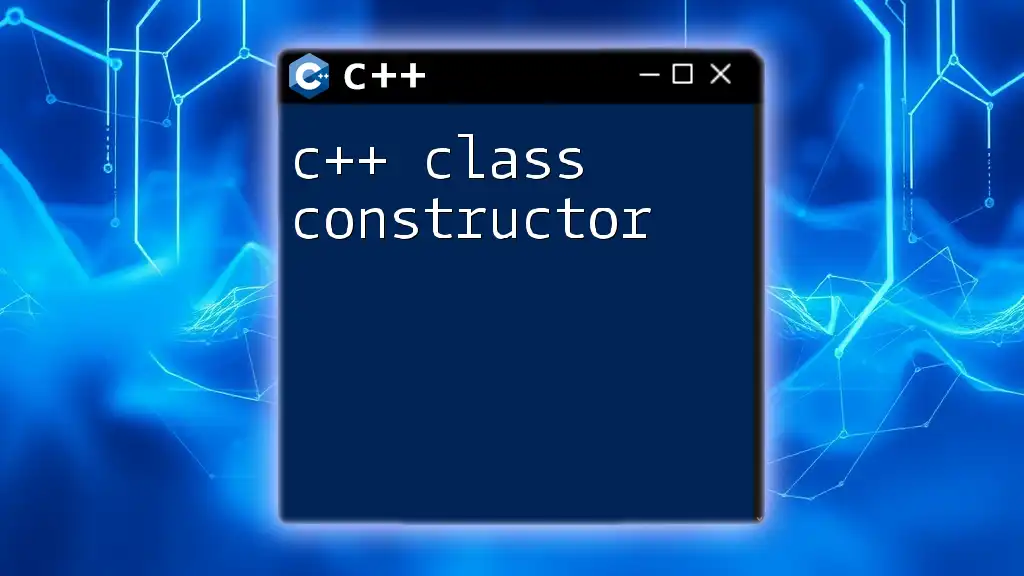
Detailed Code Examples
Example 1: Using Default and Parameterized Constructors
Let’s explore an example involving an `Employee` class:
class Employee {
private:
std::string name;
int id;
public:
Employee() : name("Unknown"), id(0) {} // Default constructor
Employee(std::string empName, int empId) : name(empName), id(empId) {} // Parameterized constructor
};
In this example, we have defined a default constructor that initializes the name to "Unknown" and the ID to 0. In contrast, the parameterized constructor lets you create an `Employee` object with a specific name and ID.
Example 2: Constructor Overloading in Action
Consider the `Circle` class as another example:
class Circle {
private:
double radius;
public:
Circle() : radius(1.0) {} // Default constructor
Circle(double r) : radius(r) {} // Parameterized constructor
Circle(int diameter) : radius(diameter / 2.0) {} // Constructor with diameter as parameter
};
This class features multiple constructors: a default constructor that gives a circle a default radius of 1.0, a parameterized constructor that accepts a radius, and another constructor that accepts a diameter, calculating the radius from it.
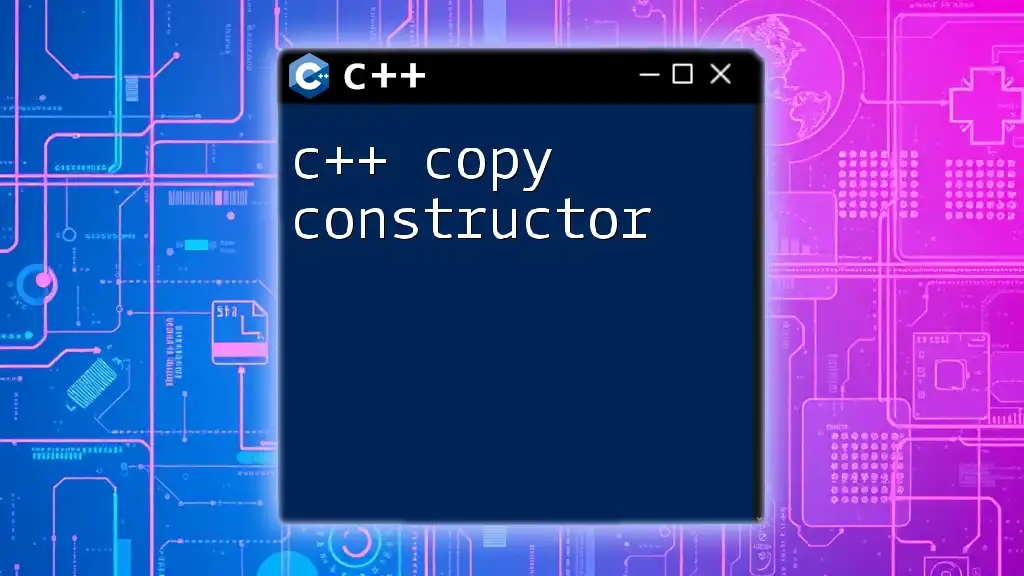
Best Practices for Using Multiple Constructors
Keep Constructors Concise
When designing constructors, it’s essential to keep them clear and concise. Avoid unnecessary complexity. Constructors should clearly express their purpose without excessive logic.
Avoid Code Duplication
To enhance maintainability, avoid duplicating code across multiple constructors. You can call one constructor from another using an initializer list. For example, if you have a parameterized constructor, use it in the default constructor to ensure consistency:
Employee() : Employee("Unknown", 0) {} // Calls parameterized constructor
Use Descriptive Names for Parameters
When declaring parameters in constructors, always use descriptive names. This practice improves code readability and helps other developers understand the purpose of each parameter.
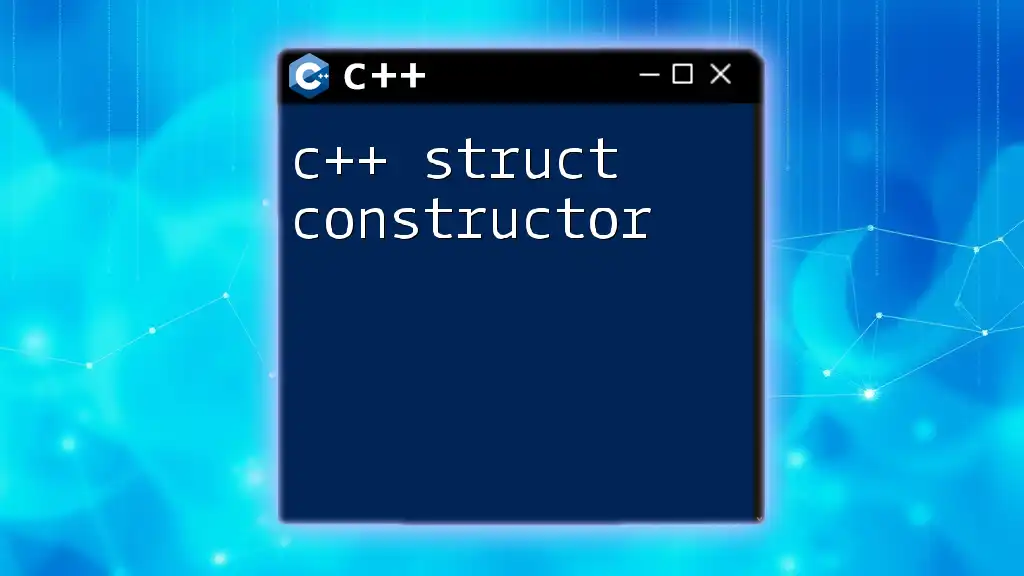
Common Mistakes and How to Avoid Them
Initializing Members Incorrectly
One common error involves incorrectly initializing members, leading to unexpected behaviors in objects. Ensure you initialize all members properly to avoid undefined behavior.
Improper Use of 'this' Pointer
New C++ programmers might mistakenly misuse the `this` pointer within constructors. The `this` pointer refers to the invoking instance, but it should be used judiciously to avoid logical errors or confusion.
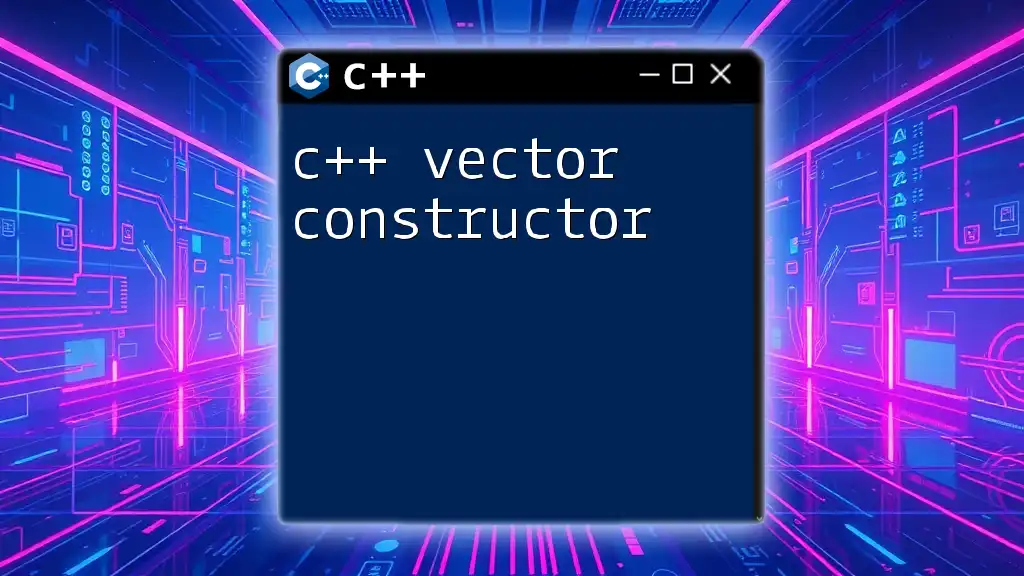
Conclusion
In summary, C++ multiple constructors provide flexibility and control over object initialization, which is essential in object-oriented programming. Constructors allow for different ways to create and initialize objects, facilitating the development process.
Utilizing constructor overloading can significantly reduce redundancy while enhancing code maintainability. As you practice and implement multiple constructors, you'll deepen your understanding of C++ and efficient class design.
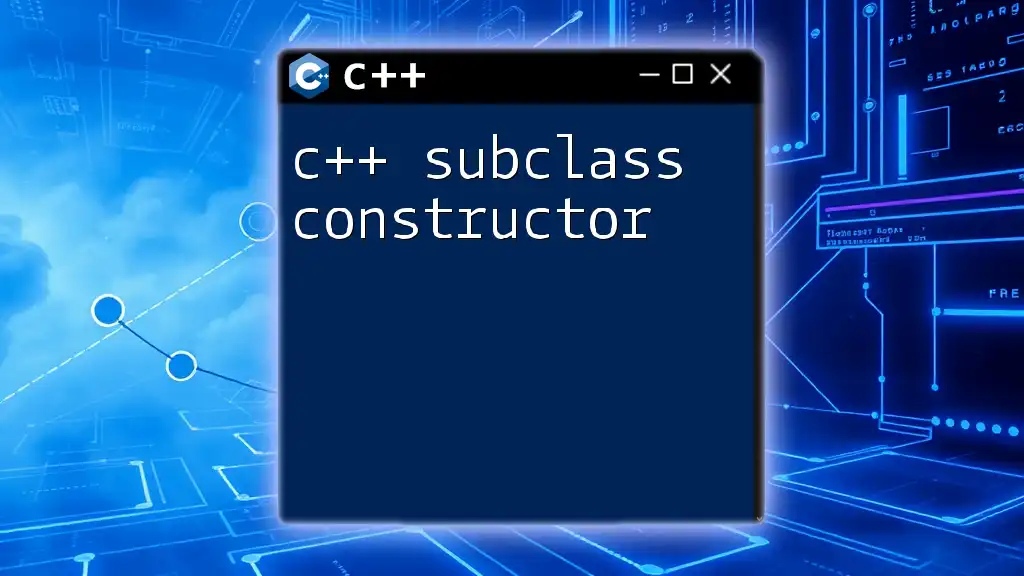
Additional Resources
To further enhance your knowledge, consult books on C++ programming, take online courses, and engage with online forums or communities. Engaging with others can provide valuable insights and foster a collaborative learning environment.