In C++, a virtual constructor is a design pattern used to emulate constructor behavior in polymorphic classes, as constructors cannot be virtual, but can be simulated using a clone method or factory function to create instances of derived classes.
Here's a simple example using a factory method:
#include <iostream>
class Base {
public:
virtual Base* clone() const = 0; // Pure virtual clone method
virtual void info() const = 0; // Pure virtual method
};
class Derived : public Base {
public:
Base* clone() const override { return new Derived(*this); }
void info() const override { std::cout << "This is a Derived object." << std::endl; }
};
int main() {
Base* obj = new Derived();
Base* objClone = obj->clone(); // Create a cloned object
objClone->info(); // Output the type of object
delete obj;
delete objClone;
return 0;
}
Understanding Constructors in C++
What is a Constructor?
In C++, a constructor is a special member function that gets called when an object of a class is instantiated. Its primary purpose is to initialize the object's attributes and prepare it for use.
There are several types of constructors:
- Default Constructor: A constructor that takes no parameters. It initializes attributes to default values.
- Parameterized Constructor: A constructor that takes parameters to initialize an object with specific values.
- Copy Constructor: A constructor that initializes a new object as a copy of an existing object.
The Role of Virtual Functions
Virtual functions enable polymorphism in C++. These functions allow derived classes to define their behaviors while maintaining the interface of the base class. This is essential for allowing dynamic binding, where the method called is determined at runtime based on the actual object type, rather than the type of the pointer or reference.
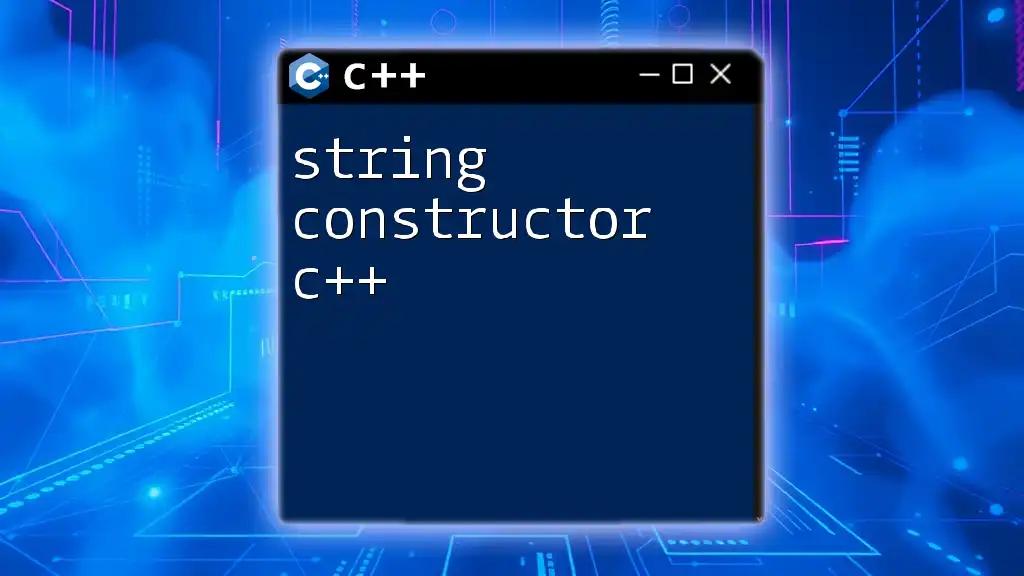
What is a Virtual Constructor?
Definition of Virtual Constructor
The term "virtual constructor" is often misunderstood in C++. It suggests a constructor that can behave polymorphically, which isn’t possible because constructors cannot be declared as virtual in C++. Instead, what is generally meant by a virtual constructor is a design pattern that simulates the behavior typically associated with a constructor through factory methods or cloning.
Why Can't Constructors be Virtual?
In C++, the rules governing constructors dictate that they cannot be virtual. When a class is instantiated, memory is allocated, and the constructor initializes that memory. Since the type of the object being created is not known until it is fully constructed, virtual constructors cannot exist in the traditional sense.
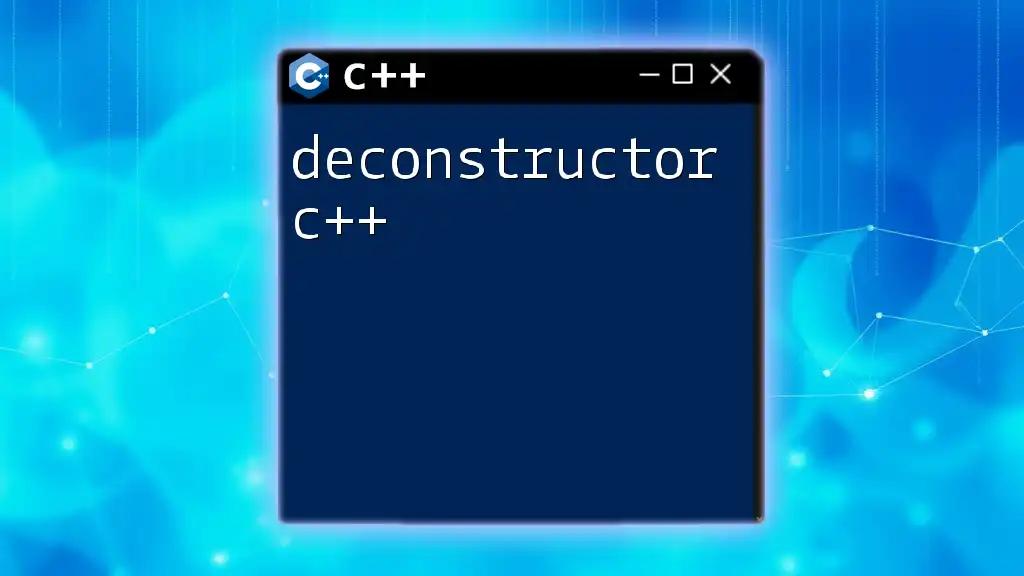
Simulating a Virtual Constructor
Using Factory Methods
To simulate the behavior of a virtual constructor, we often utilize a factory method pattern. This design encapsulates the object creation process and allows derived classes to be instantiated without the consumer needing to know their exact types.
How it Works
A factory method provides a way to create objects while allowing the specific class to be instantiated at runtime based on input or other logical conditions.
Code Example:
class Base {
public:
virtual Base* clone() const = 0; // Clone method
virtual ~Base() {}
};
class Derived : public Base {
public:
Derived* clone() const override { return new Derived(*this); }
};
Base* createObject(const std::string& type) {
if (type == "derived") {
return new Derived();
}
return nullptr;
}
In this example, the `createObject` function demonstrates how we can dynamically create an instance of `Derived` based on a string input. This allows for flexibility without having to explicitly know the derived class details.
Using Cloning
Another effective approach to simulate virtual constructors in C++ is via cloning, which involves defining a virtual `clone` function that allows an object to create a copy of itself.
Code Example:
class Animal {
public:
virtual Animal* clone() const = 0;
virtual ~Animal() {}
};
class Dog : public Animal {
public:
Dog* clone() const override { return new Dog(*this); }
};
Animal* original = new Dog();
Animal* copy = original->clone();
In this example, the `Dog` class implements the `clone` method, allowing one to create a new instance of `Dog` based on an existing instance. This is a powerful technique that leverages polymorphism effectively for object creation.
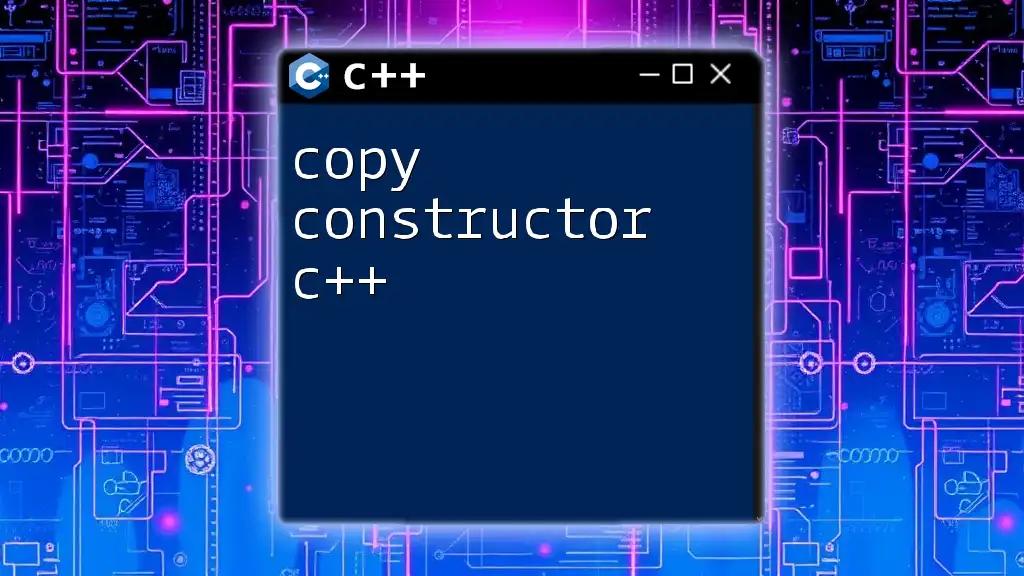
Use Cases for Virtual Constructor Alternatives
When to Use Factory Methods vs. Cloning
The choice between factory methods and cloning often depends on the specific requirements of the application:
-
Factory Methods: Opt for factory methods when you need to create instances of multiple derived classes or when the instantiation logic is based on parameters or configuration.
-
Cloning: Use cloning when the creation of an object is based on an existing instance, and you want to duplicate the object’s state and behavior effectively.
Real-world Applications
Several design patterns showcase how these concepts can be effectively utilized, such as:
- Singleton Pattern: Where a single instance is created and managed globally.
- Prototype Pattern: Which emphasizes cloning existing instances rather than creating new ones from scratch.
Understanding factory patterns and cloning can significantly enhance your design skills as they offer flexibility and reusability in your code.

Common Mistakes and Misunderstandings
Understanding Object Slicing
A common issue that arises in C++ with classes and inheritance is object slicing. This occurs when an object of a derived class is assigned to a variable of a base class type leading to the loss of the derived part of the object.
Example:
Derived derived;
Base base = derived; // This leads to object slicing
In this case, the `Base` class object will only retain its attributes and methods while the derived attributes are lost. It’s crucial to be aware of this when working with inherited classes to avoid unintended behavior.
Misuse of Factory Functions
Another pitfall is the misuse of factory functions. Common mistakes include:
- Not implementing proper memory management, potentially leading to memory leaks.
- Creating factory methods that expose too much of the underlying class structure, compromising encapsulation.
To avoid these pitfalls, ensure that your factory methods are designed with clarity and separation of concerns in mind.
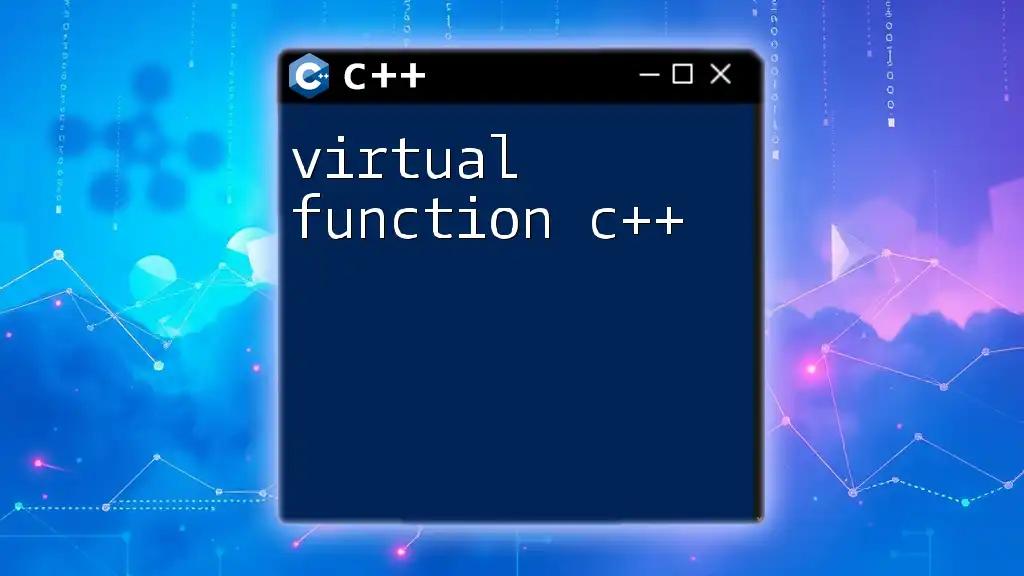
Conclusion
Understanding the concept of a virtual constructor in C++ is essential for mastering object-oriented programming. By leveraging factory methods and cloning, developers can effectively simulate a constructor’s polymorphic behavior, enabling flexible and maintainable code.
As you deepen your understanding of C++ design patterns, consider exploring additional resources and tools that can further enhance your capabilities and improve your software design skills. The journey to mastering C++ is a continuous one, filled with opportunities to learn and innovate.