Overloading constructors in C++ allows a class to have multiple constructors with different parameters, enabling the creation of objects in various ways.
Here’s an example:
class Point {
public:
int x, y;
// Default constructor
Point() : x(0), y(0) {}
// Parameterized constructor
Point(int xCoord, int yCoord) : x(xCoord), y(yCoord) {}
};
// Usage
Point p1; // Calls default constructor
Point p2(5, 10); // Calls parameterized constructor
What is C++ Constructor Overloading?
Constructor overloading is a powerful feature in C++ that allows a class to have multiple constructors, each with different parameter lists. By defining multiple constructors, developers can create objects in various ways, providing flexibility and functionality tailored to different initialization needs.
The main advantage of constructor overloading is that it allows for clearer, more concise object creation. Instead of writing numerous setter methods or creating different types of objects, a single class can encapsulate various initialization patterns, enhancing code readability and usability.
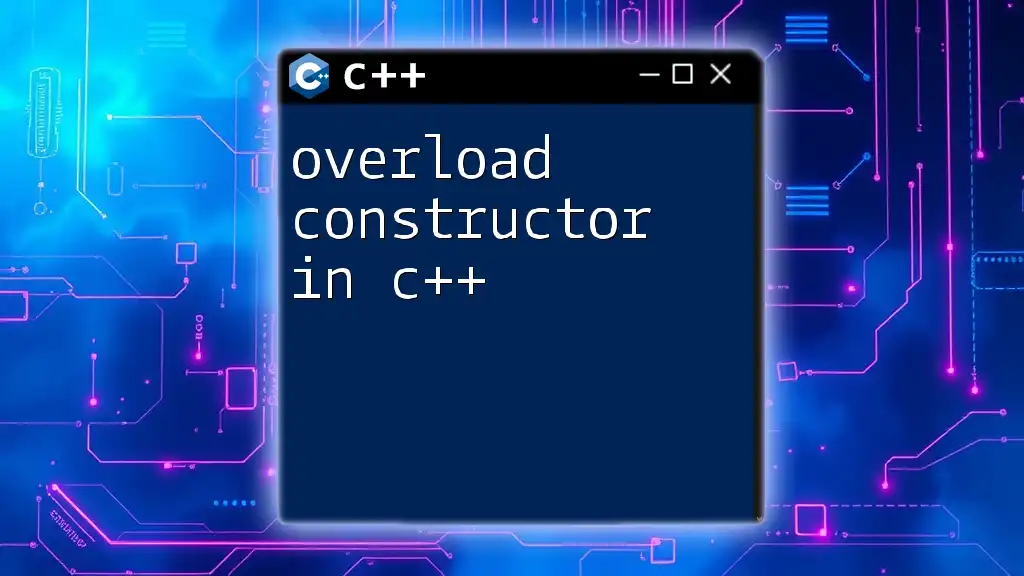
Syntax of Overloaded Constructors
The syntax for defining overloaded constructors is fairly straightforward. Each constructor must have a unique parameter list but can otherwise have the same name. Here’s the general layout:
class ClassName {
public:
ClassName(); // Default constructor
ClassName(Type1 param1); // Overloaded constructor with one parameter
ClassName(Type1 param1, Type2 param2); // Overloaded constructor with two parameters
};
In the above example, we define a class named `MyClass` with three constructors. Their unique parameter lists allow C++ to distinguish between them at compile time.
Example
class MyClass {
public:
MyClass(); // Default constructor
MyClass(int a); // Overloaded constructor with one parameter
MyClass(int a, int b); // Overloaded constructor with two parameters
};
Here, `MyClass` can be instantiated in three different ways depending on whether the user provides no arguments, one integer, or two integers.
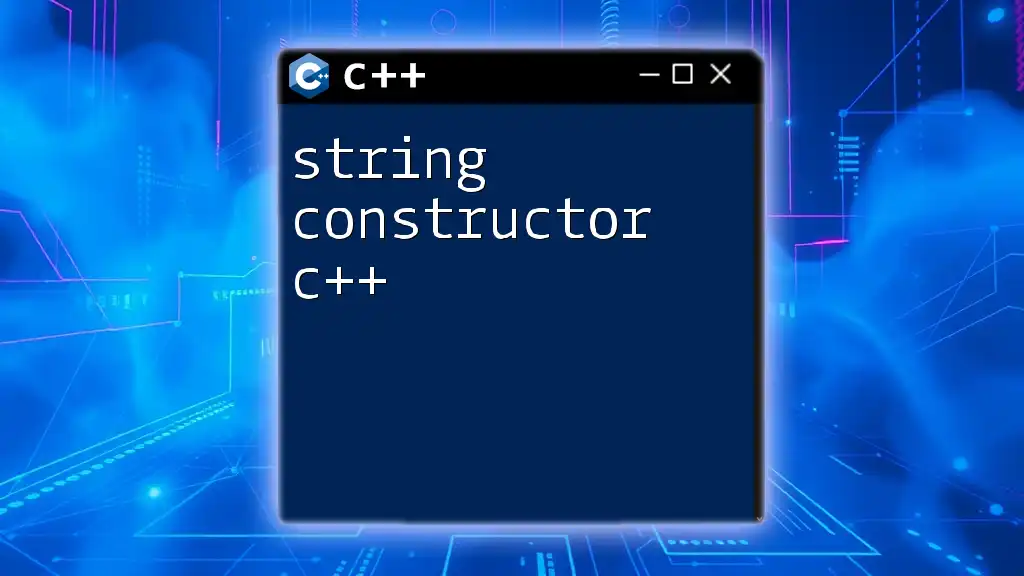
Reasons to Use Constructor Overloading in CPP
Enhanced Flexibility
Overloading constructors provides significant flexibility when creating objects. Different situations might require different initial setups. For example, if you're creating a `Rectangle` class, you might want the option to define a rectangle with only width (creating a square), or with width and height separately.
Example:
class Rectangle {
public:
int width, height;
Rectangle() : width(0), height(0) {} // Default constructor
Rectangle(int w) : width(w), height(w) {} // Square constructor
Rectangle(int w, int h) : width(w), height(h) {} // Rectangular constructor
};
Improved Readability
Constructor overloading can improve the readability of your code. By making object instantiation self-explanatory, developers can instantly recognize the intended object type from its construction.
For instance:
Rectangle defaultRect; // Default constructor sets dimensions to (0, 0)
Rectangle square(5); // Clear intention of creating a square
Rectangle rect(5, 10); // Instantly clear that a rectangle with specific dimensions is created
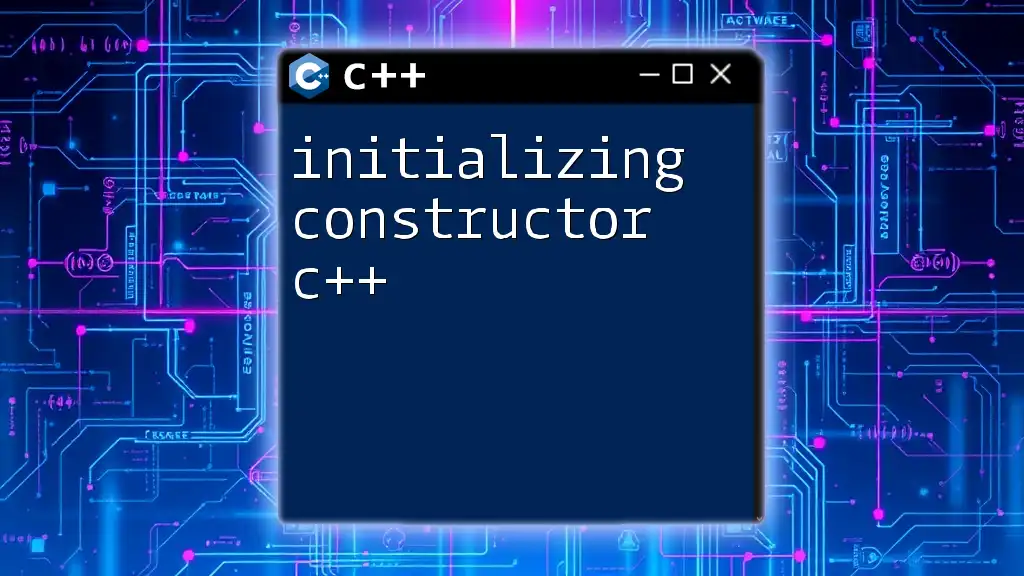
How to Implement Constructor Overloading in C++
Step-by-Step Implementation
Creating a class with overloaded constructors involves defining multiple constructors with distinct parameter lists.
- Define the Class: Start by creating the class and declaring its members.
- Implement Overloaded Constructors: Write constructors for different initialization scenarios.
Here’s a simple implementation:
class Circle {
private:
double radius;
public:
Circle() : radius(1.0) {} // Default constructor
Circle(double r) : radius(r) {} // Parameterized constructor
};
In this example, `Circle` can either be instantiated with a default radius of 1.0 or custom-defined radius.
Common Scenarios for Constructor Overloading
Constructor overloading is most beneficial when initializing objects with varying levels of detail. It allows for options based on user input or default values, which mirrors many real-world object creations.
Circle defaultCircle; // Uses default constructor, radius = 1.0
Circle customCircle(2.5); // Radius is set to 2.5
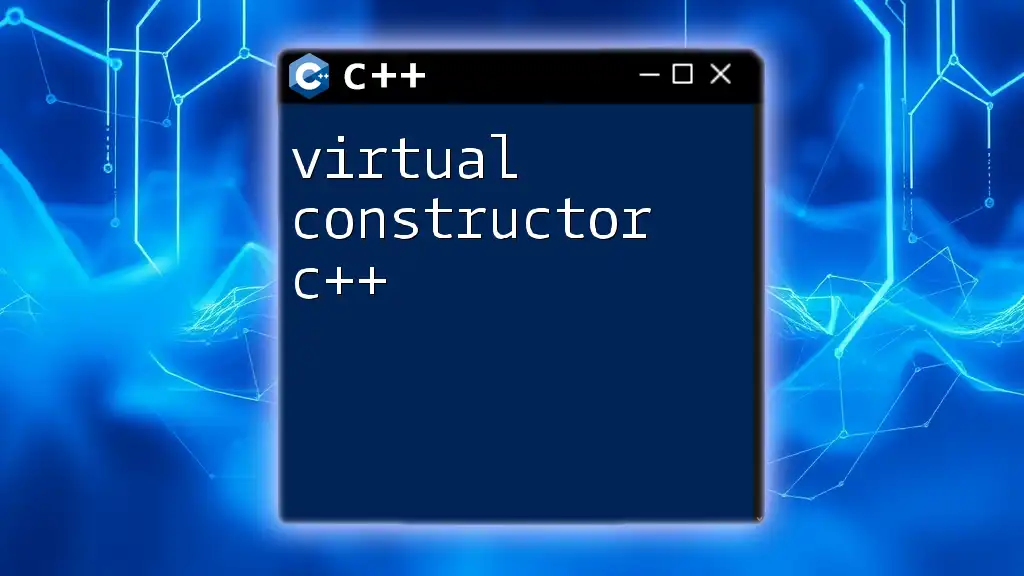
Differences Between Constructor Overloading and Other Forms of Overloading
It’s essential to clarify the distinction between constructor overloading and other forms of overloading, such as method overloading.
-
Constructor Overloading: Applies specifically to constructors within a class, allowing multiple ways to instantiate an object based on argument differences.
-
Method Overloading: Involves defining multiple methods with the same name but different parameter lists within a class. This is useful when a method can be enacted in different contexts.
Deciding whether to use constructor overloading or method overloading depends on the specific needs of your class design and object instantiation.
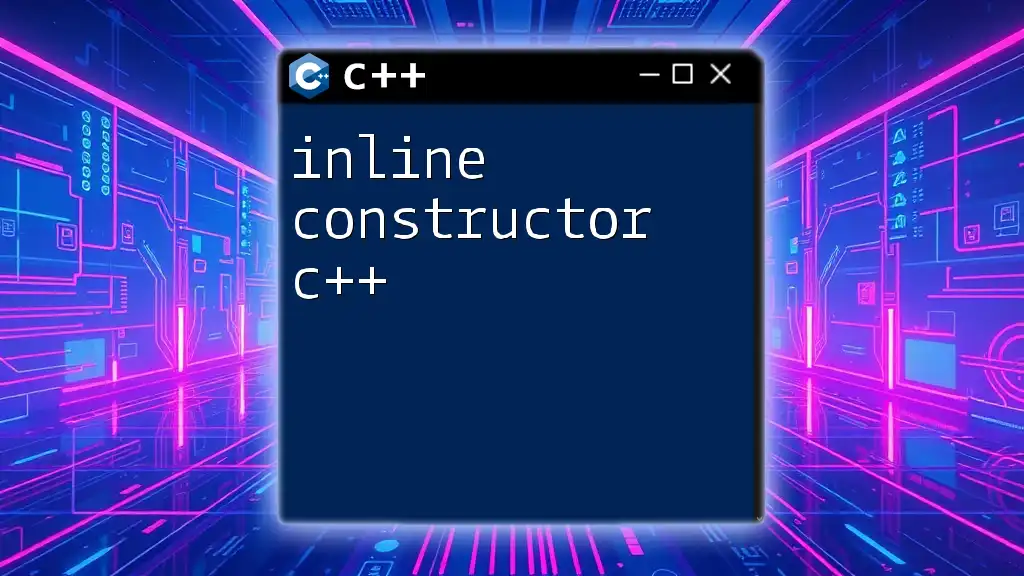
Best Practices for Overloading Constructors in C++
Keeping Constructors Simple
While overloading constructors, strive for simplicity. Constructors should primarily focus on initializing object state, not performing complex calculations or conditional logic. If initialization becomes complex, consider utilizing factory methods or dedicated initialization functions outside the constructor.
Using Delegating Constructors (C++11 and beyond)
Introduced in C++11, delegating constructors are a powerful feature that allows one constructor to call another directly, enhancing code reusability and minimizing redundancy.
Example:
class Point {
private:
int x, y;
public:
Point(int xVal) : Point(xVal, 0) {} // Delegates to two-parameter constructor
Point(int xVal, int yVal) : x(xVal), y(yVal) {}
};
This example demonstrates how the one-parameter constructor delegates the task of setting both `x` and `y` to the two-parameter constructor.
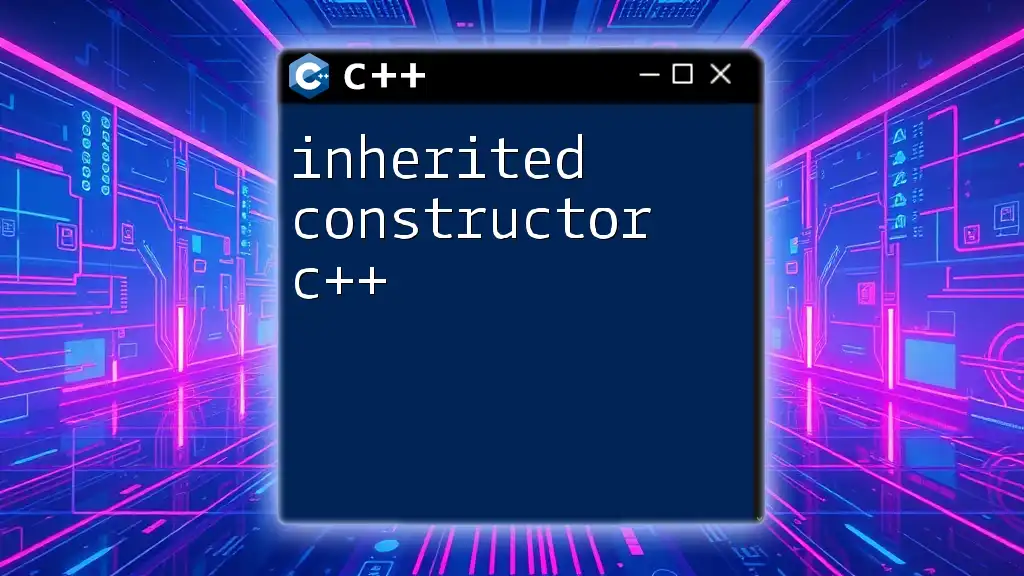
Common Mistakes to Avoid When Overloading Constructors
Avoiding Ambiguity
One common mistake when overloading constructors is creating ambiguity due to similar parameter lists. For example:
class Test {
public:
Test(int a, double b);
Test(double a, int b); // This may cause ambiguity if values passed are convertible
};
Inserting values that can match multiple constructors can lead to confusion. Always ensure that your parameter lists are distinct enough to avoid this situation.
Ensuring Clarity
Make constructors self-explanatory and intuitive—inform potential users about what each constructor intends to achieve through well-chosen parameter names and documentation.
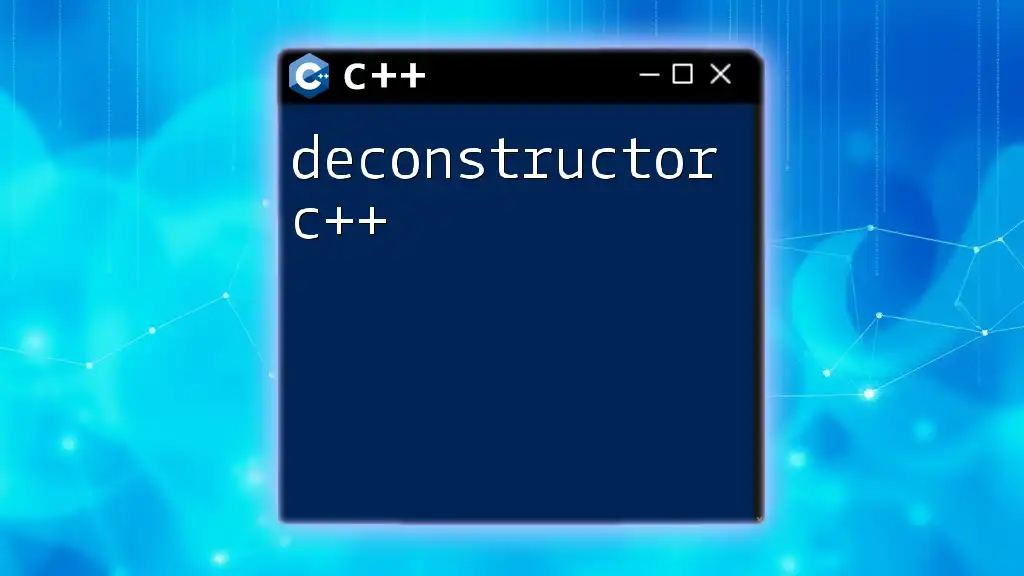
Conclusion
In summary, overloading constructors in C++ not only enhances code flexibility and readability but also provides a structured way to create complex objects. By understanding the syntax and implementation nuances of constructor overloading, developers can leverage C++’s capabilities to write cleaner, more maintainable code. The practice of using overloaded constructors should be coupled with good design principles, ensuring that your classes remain comprehensible and efficient. As you implement and master constructor overloading, you’ll find your C++ programs can efficiently handle a myriad of object creation scenarios.
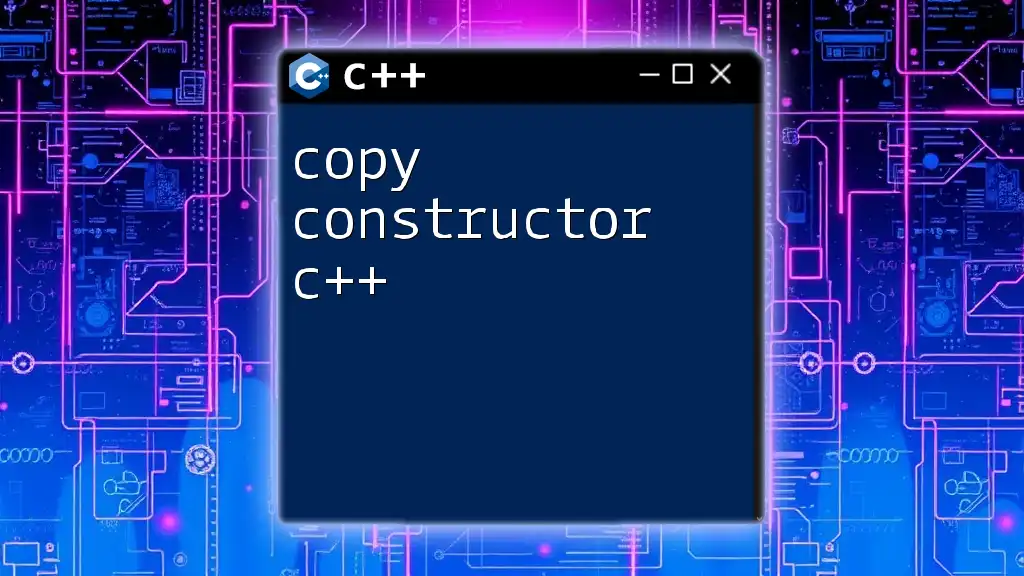
Additional Resources
For further exploration of constructor overloading and general C++ programming concepts, consider diving into recommended books, online courses, and engaging with developer communities. Exploring additional resources will help solidify your understanding and proficiency in using C++ effectively.