In C++, an initializing constructor is a special type of constructor that initializes member variables using an initialization list, allowing for efficient and direct assignment of values when an object is created.
class MyClass {
public:
int x;
MyClass(int val) : x(val) {} // Initializing constructor
};
MyClass obj(10); // Creates an object 'obj' with x initialized to 10
Understanding Constructor Initialization
Constructors are special member functions in C++ designed to initialize objects when they are created. The principle behind constructor initialization is to set up the initial state of the class objects, ensuring they are ready to use right after their creation. This initial setup is crucial in resource management, providing stability and predictability in object-oriented programming.
Types of Constructors
There are three main types of constructors in C++:
-
Default Constructor: A constructor that can be called without any arguments. It usually initializes member variables to default values.
-
Parameterized Constructor: A constructor that takes arguments to initialize member variables based on the values provided during object creation.
-
Copy Constructor: A constructor that creates a new object as a copy of an existing object, which is essential for managing resources properly.
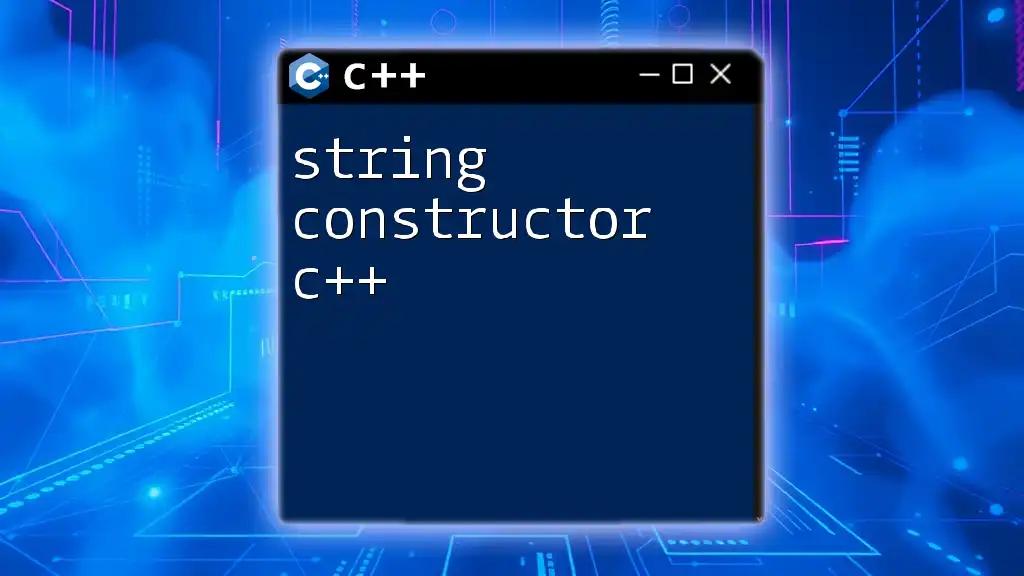
C++ Constructor Initialization: The Basics
Constructor initialization is the process through which a class instance is set up when an object is instantiated. It plays a pivotal role in ensuring that class members hold meaningful values, improving code reliability. For instance, if you create an object of a class representing a `Rectangle`, the constructor can accept length and width as parameters, initializing these members right at the object’s birth.
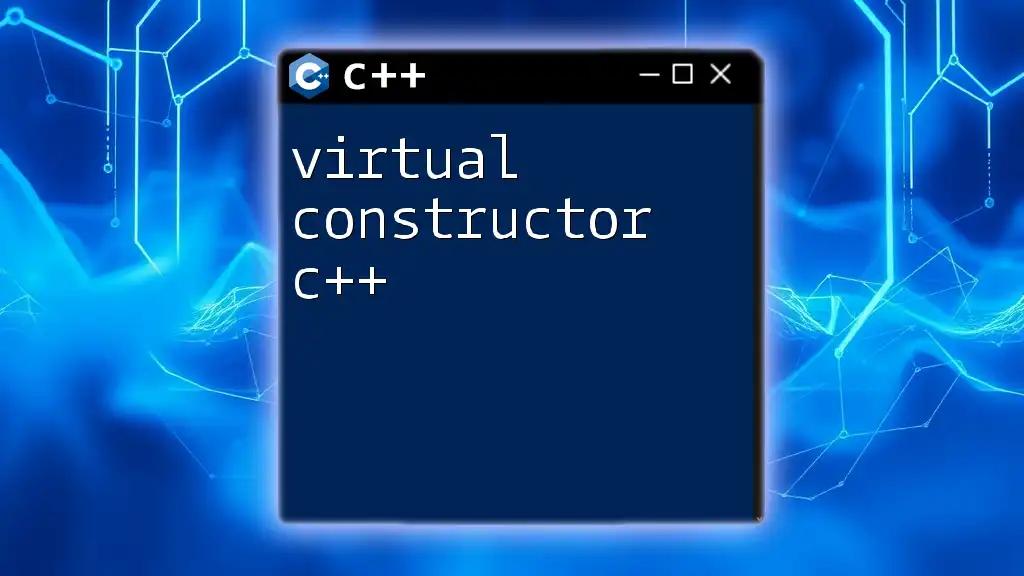
The Constructor Initialization List
What is a Constructor Initialization List?
The constructor initialization list is a mechanism in C++ that allows for the initialization of class members before the constructor's body executes. This is particularly beneficial because it can enhance performance and ensure that all member variables are initialized correctly.
Syntax and Structure
The syntax for using an initialization list is as follows:
ClassName::ClassName(parameters) : initializer1(value1), initializer2(value2) {
// Constructor Body
}
Example of Constructor Initialization List
Consider the following example of a class `Point`:
class Point {
private:
int x, y;
public:
Point(int x_value, int y_value) : x(x_value), y(y_value) {
// No additional tasks in constructor body
}
};
In this example, `x` and `y` are initialized before the constructor body executes, ensuring that the `Point` object starts with defined values.

Benefits of Using Initialization Lists
Efficiency and Performance
One of the key advantages of using initialization lists is their efficiency. When member variables are initialized directly in the list, it can minimize the overhead associated with assignment operations that occur within the constructor body. This is particularly evident in cases where constructors of complex types or containers are involved.
Enforcing Invariants in Class Initialization
By relying on initialization lists, you can enforce invariants—rules that must always hold true for the consistent state of the object. For instance, initializing a class's member variables in an initializer list guarantees that the constructor body won’t execute unless all members are set correctly.
Handling Constant and Reference Members
When working with `const` members or references, initialization lists are essential. Since `const` members cannot be assigned new values after they have been created, they must be initialized when the constructor is called. Here's an example:
class Example {
const int id;
int& ref;
public:
Example(int idValue, int& refValue) : id(idValue), ref(refValue) {}
};
In this code, `id` is a `const` member that must be initialized through the constructor’s initialization list, alongside `ref`, demonstrating the necessity of this practice.

Constructor Initialization in C++
How to Properly Initialize Members
Properly initializing members within a class involves leveraging both parameterized constructors and initializer lists. This strategy not only ensures that all members receive valid input values but also enhances code maintainability. By following clear and consistent patterns, developers can avoid linking errors and introduce fewer bugs.
Best Practices for Constructor Initialization
To maximize effectiveness in constructor initialization, consider the following best practices:
-
Always prefer initialization lists over assignment within the constructor body. This makes initialization explicit and predictable.
-
Use `const` members whenever possible to ensure the integrity of an object’s state.
-
Avoid unnecessary computations or side effects in the initializer list. The purpose is solely to assign values during object creation.

Class Initializer in C++
Using Default and Delegating Constructors
C++11 introduced the concept of delegating constructors, which allows one constructor to call another, effectively reducing redundancy. Here’s how you can implement this:
class Employee {
public:
Employee() : Employee("Default", 0) {}
Employee(std::string n, int a) : name(n), age(a) {}
};
In this example, the default constructor invokes the parameterized constructor, setting default values for a `name` and `age`. This not only prevents code duplication but also enhances readability.
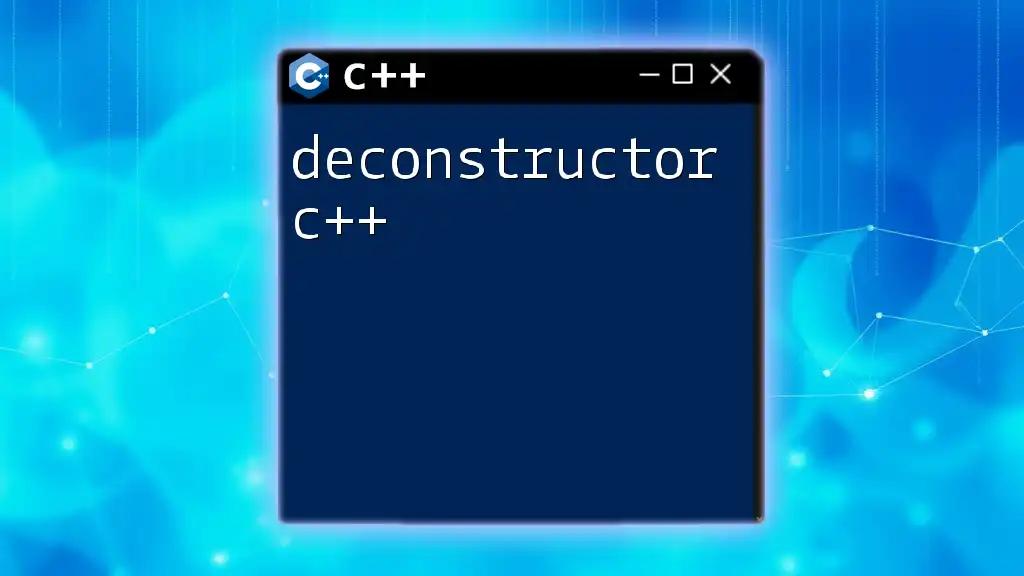
C++ Class Initialization Techniques
Using Smart Pointers in Initialization
Smart pointers are a robust feature in C++ used for dynamic memory management. They ensure that memory is adequately managed through automatic deallocation when the pointer goes out of scope. Here's an example of using a smart pointer in a class constructor:
class Resource {
std::shared_ptr<int> value;
public:
Resource(int val) : value(std::make_shared<int>(val)) {}
};
In this scenario, `value` is initialized using `std::make_shared`, providing a secure way of managing memory and avoiding memory leaks.

Common Mistakes in C++ Constructor Initialization
Overlooking Member Initialization
One common mistake developers encounter is overlooking member initialization. Failing to initialize members can lead to undefined behavior, resulting in difficult-to-debug issues. It’s essential to ensure every member variable receives a valid state when an object is constructed.
Misusing Initialization Lists
Additional errors arise from misuse of initialization lists. For example, using them to perform complex calculations or calls to non-const member functions can lead to surprising behavior. Always keep the initialization list focused on direct member assignments.
Not Following Best Practices
Ignoring established best practices can result in unreliable code. Developers should strive to maintain high code standards to enhance collaboration and minimize errors. Following consistent patterns simplifies other developers’ understanding of the code.
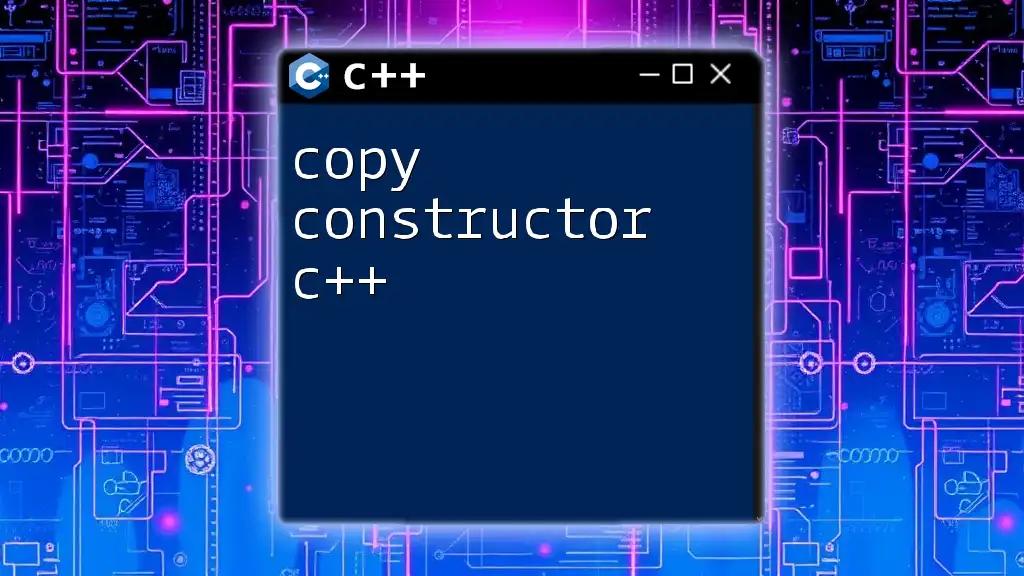
Conclusion
Mastering the art of initializing constructors in C++ is a vital skill for programming excellence. A thorough understanding of initialization lists, best practices, and common pitfalls will empower developers to write efficient, reliable code that stands the test of time. The journey to becoming proficient in this area not only enhances your personal skillset but also builds more robust software solutions.

Additional Resources
As you continue your journey with C++, consider exploring recommended books and online courses. Consult the robust documentation available online to deepen your understanding and practice your skills continually. This proactive approach will ensure your abilities remain sharp as you progress in the fascinating world of C++.