To initialize a vector in C++ with all elements set to 0, you can use the following syntax:
#include <vector>
std::vector<int> myVector(10, 0); // Initializes a vector of size 10 with all elements set to 0
What is a Vector in C++?
Definition of a Vector
A vector in C++ is a part of the Standard Template Library (STL), functioning as a dynamic array. Unlike static arrays, vectors can grow and shrink in size automatically, providing flexibility in memory management. This dynamic nature allows you to easily add or remove elements without the need to manually handle memory allocation.
Importance of Vectors in C++
Vectors are highly favored over traditional arrays for several reasons:
- Dynamic Sizing: You can add elements without worrying about the initial size, which is especially useful in scenarios where the number of elements is unknown at compile time.
- Random Access: Vectors allow for direct access to elements using indices, making data retrieval efficient.
- Ease of Use: Vectors come with built-in functions that simplify operations such as insertion, deletion, and sorting.
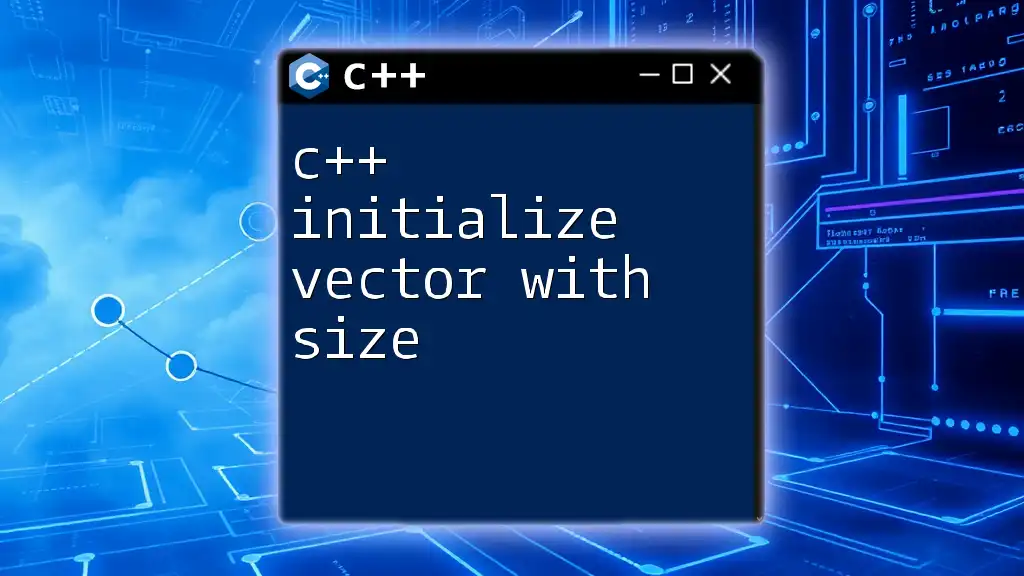
Initializing Vectors in C++
Methods to Initialize Vectors
When it comes to vectors, C++ provides multiple methods for initialization, which enables developers to choose the most appropriate approach based on their needs.
Initializing a Vector with Zeros
Using Constructor
One of the simplest ways to initialize a vector with zeros is by utilizing the constructor. You can specify the size and the initial value directly:
#include <vector>
std::vector<int> myVector(10, 0); // Initializes a vector with 10 elements, all set to 0
In the example above, `myVector` has 10 elements, and each one is initialized to zero. This method is efficient and straightforward, making it a common choice for developers.
Using `assign()` Method
Another method to initialize a vector is the `assign()` function, which allows you to set a specified number of elements to a specific value after the vector has been declared:
std::vector<int> myVector;
myVector.assign(10, 0); // Assigns 10 zeros to the vector
In this example, the vector is first created and then populated with 10 zeros using the `assign()` method. This is particularly helpful if the vector was previously initialized and you need to reset its contents.
Using `resize()` Method
The `resize()` method provides a way to change the size of the vector while also specifying a value to initialize new elements:
std::vector<int> myVector;
myVector.resize(10, 0); // Resizes and initializes the vector with zeros
Here, `resize()` not only increases the size of `myVector` to 10 but also initializes new elements to zero. If `myVector` already contained elements, the existing values beyond the new size would be retained or removed.
Initializing a Vector of Different Data Types with Zeros
For Floating Points
Vectors can store various types of data, including floating-point numbers. An example of initializing a vector containing `double` values set to zero is as follows:
std::vector<double> myDoubleVector(10, 0.0); // Initializes a vector of doubles with zeros
Using `0.0` ensures that the vector is correctly initialized with floating-point zeros, which is essential for type safety.
For Custom Objects
If you have a custom class and wish to initialize a vector of that class with default values, ensure that your class has a constructor that initializes its attributes to 0 or any default value. Here’s an example:
class MyClass {
public:
int value;
MyClass() : value(0) {} // Default constructor initializing value to 0
};
std::vector<MyClass> myClassVector(10); // Initializes a vector of MyClass objects
In this case, `myClassVector` contains 10 instances of `MyClass`, each of which is initialized to have a member variable `value` set to 0.
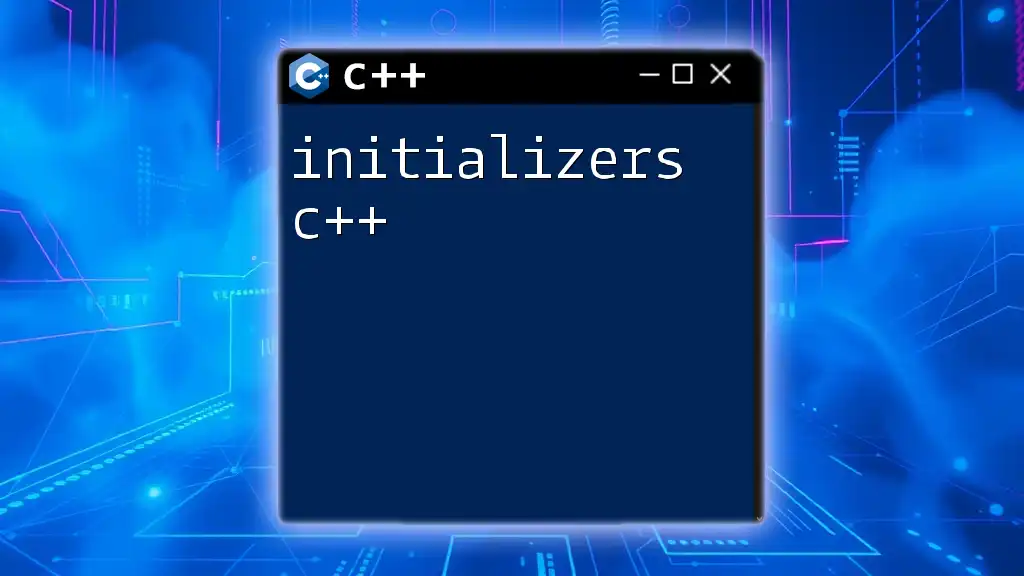
Common Use Cases for Initializing a Vector with Zeros
Default Initialization for Data Storage
A zero-initialized vector can be incredibly useful as a placeholder for future data input. For instance, if you’re aggregating results from different calculations, starting with zeros ensures that the accumulation will be accurate.
Algorithms and Computations
Zero-initialized vectors are often used in algorithms, particularly in scenarios involving mathematical computations. For example, when calculating the sum or average of a set of numbers, initializing the vector to zero can provide a clean starting point for your calculations.
Consider the following accumulation example:
#include <vector>
#include <numeric>
std::vector<int> myVector(10, 0); // Initializes a vector with 10 zeros
int sum = std::accumulate(myVector.begin(), myVector.end(), 0); // Sum = 0
The use of a zero-initialized vector here ensures the sum starts at zero.
Performance and Memory Considerations
When initializing vectors with zeros, it's critical to consider performance implications. Using a constructor to initialize with zeros is generally more memory-efficient, as it avoids unnecessary reallocations. Carefully choosing the method of initialization based on your application's needs can lead to optimized performance.
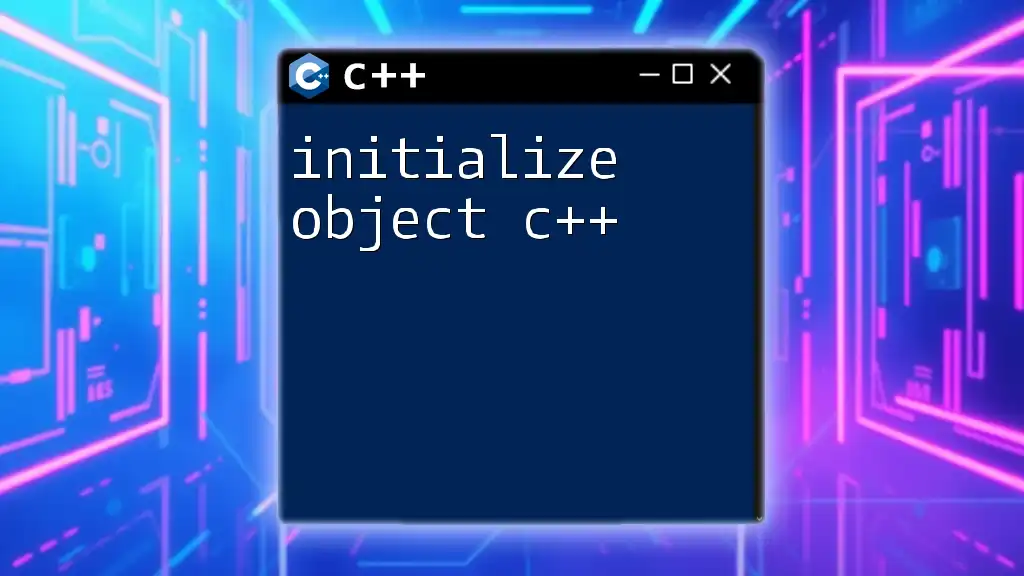
Error Handling and Best Practices
Avoiding Common Pitfalls
Common mistakes when initializing vectors include failing to specify the size, which can lead to uninitialized elements, or confusing the syntax of different initialization methods. Always ensure clarity in your code.
Best Practices for Initialization
- Choose the right method: Use constructors when the size is known at compile time and `resize()` when modifying existing vectors.
- Prefer clarity: Write clear code that clearly indicates the intention of initialization, making it easier for others to understand.
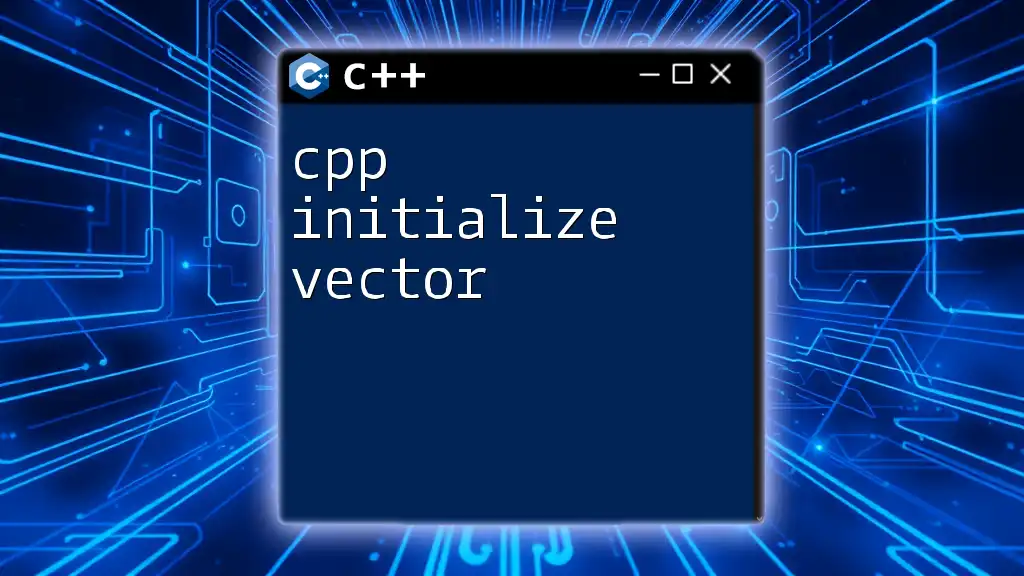
Conclusion
In sum, learning how to initialize vector C++ with 0 can significantly improve your programming efficiency and clarity. Whether you utilize constructors, the `assign()` method, or `resize()`, understanding these techniques lays a solid foundation for effective vector usage in C++. We encourage you to practice these concepts in various coding scenarios to enhance your skills further.
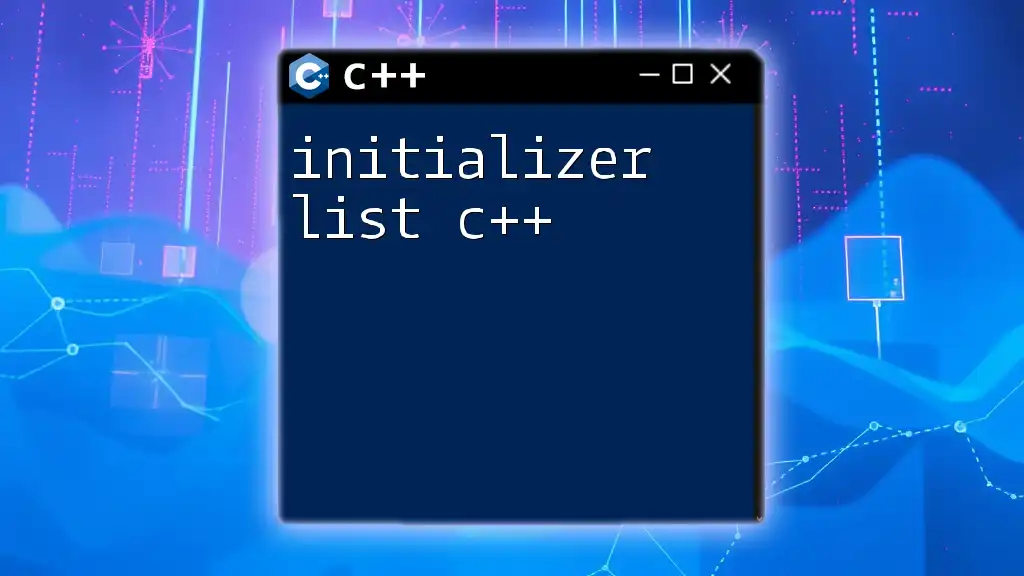
Additional Resources
Recommended Reading Materials
Explore books, articles, and documentation on C++ vectors for deeper insights and advanced techniques.
Online Tutorials and Tools
Utilize various online platforms for practice and further learning about vector manipulation and best practices in C++.