The `reserve` function in C++ is used to preallocate memory for a vector, which can improve performance by reducing the number of reallocations when adding elements.
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec;
vec.reserve(10); // reserves space for 10 elements
std::cout << "Capacity after reserve: " << vec.capacity() << std::endl; // Outputs: Capacity after reserve: 10
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A vector in C++ is a sequence container representing an array that can change in size dynamically. Unlike static arrays, vectors can easily resize themselves when elements are added or removed. This feature is particularly useful when the number of elements is not known at compile time.
For example, you can declare and initialize a vector of integers as follows:
#include <vector>
std::vector<int> numbers; // Declares an empty vector of integers
Benefits of Using C++ Vectors
C++ vectors offer several key advantages:
- Automatic Memory Management: Vectors manage their own memory allocation, minimizing the risk of memory leaks that can occur with manual memory management.
- Random Access: Vectors allow access to elements using an index, much like arrays. This makes retrieving and modifying data efficient.
- Utility Functions: Vectors provide a variety of member functions such as `push_back`, `pop_back`, and `size`, enhancing usability.
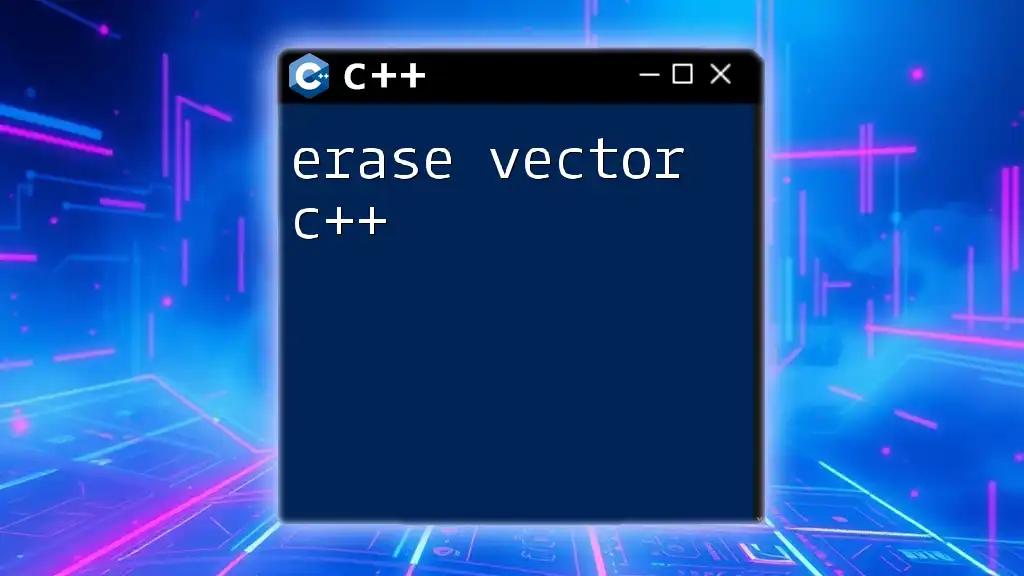
The `reserve` Method
What is `reserve` in C++?
The `reserve` function is a member of the vector class that allows you to preallocate memory for a specified number of elements. This method is especially potent when you have an estimate of the number of elements that will be stored in the vector to avoid frequent reallocations.
The syntax for `reserve` is:
void reserve(size_type n);
Why Use `reserve`?
Using `reserve` has several benefits:
- Performance Improvements: Reserving space in advance helps to limit the number of reallocations required as the vector grows. Frequent reallocations can lead to increased time complexity, as elements need to be copied over to a new memory location.
- Heap Fragmentation: By reserving a larger block of memory, you reduce the chances of heap fragmentation, which can occur when memory is allocated in small chunks.
When you invoke `reserve`, you are indicating to the vector that it should allocate enough memory to store n elements. However, it does not change the current size of the vector.

How to Use `reserve` in C++
Basic Example
Using `reserve` is straightforward. Here’s a simple example to illustrate its use:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.reserve(10); // Reserve space for 10 integers
std::cout << "Capacity after reserve: " << numbers.capacity() << std::endl;
return 0;
}
In this example, we reserve capacity for 10 integers. After the call to `reserve`, the capacity of the vector will be adjusted to hold at least 10 elements without reallocating memory again until the capacity exceeds this value.
Advanced Usage Scenarios
Pre-populating Vectors
When you know the number of elements in advance, you can efficiently utilize `reserve` to prevent multiple reallocations while filling the vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers;
numbers.reserve(10); // Reserve space for 10 integers
for (int i = 0; i < 10; ++i) {
numbers.push_back(i); // Fill the vector
}
std::cout << "Size after populating: " << numbers.size() << std::endl;
std::cout << "Capacity after populating: " << numbers.capacity() << std::endl;
return 0;
}
After reserving space, you can efficiently fill the vector without worrying about multiple reallocations affecting performance.
Managing Large Data Sets
When dealing with large datasets, reserving the necessary memory can be crucial. For example:
#include <vector>
#include <string>
#include <iostream>
int main() {
std::vector<std::string> largeDataSet;
largeDataSet.reserve(100000); // Reserve space for 100,000 strings
// Example of filling the vector with sample data
for (int i = 0; i < 100000; ++i) {
largeDataSet.push_back("Sample string " + std::to_string(i));
}
std::cout << "Size of dataset: " << largeDataSet.size() << std::endl;
std::cout << "Capacity of dataset: " << largeDataSet.capacity() << std::endl;
return 0;
}
In this case, by preallocating memory for 100,000 strings, you avoid the performance hit caused by multiple reallocation as the vector grows.
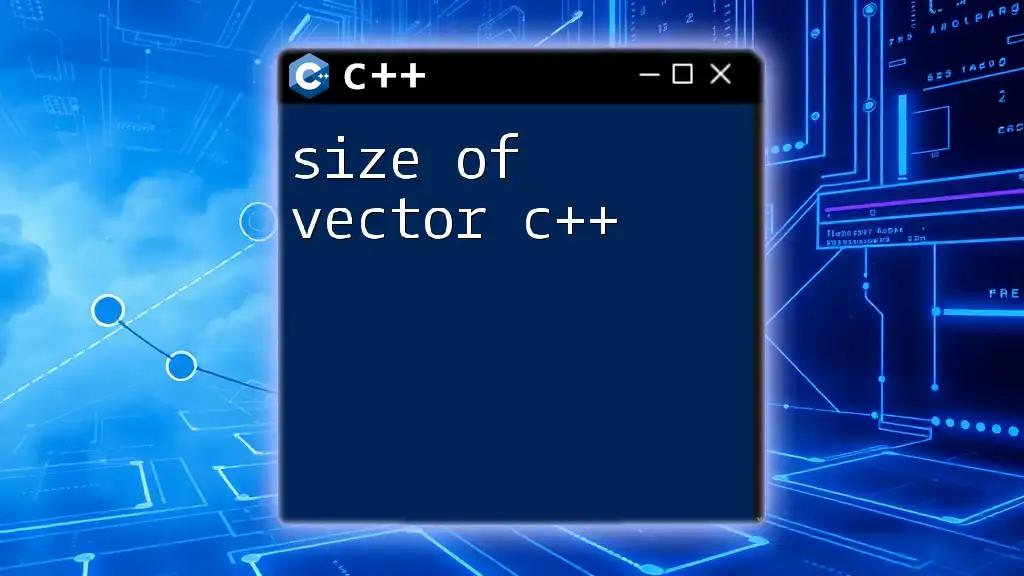
Common Misconceptions About `reserve`
`reserve` vs. `resize`
It's essential to understand the distinction between `reserve` and `resize`. While both adjust the capacity of a vector, they serve different purposes:
-
`reserve`: Changes the capacity but does not affect the size or initialize existing elements. For instance:
std::vector<int> numbers; numbers.reserve(10); // Capacity becomes at least 10, size remains 0
-
`resize`: Modifies both the size and the capacity of the vector. It can also initialize new elements to a default value. For example:
std::vector<int> numbers; numbers.resize(10); // Size is now 10, and elements are default-initialized
What `reserve` Does Not Do
It is a common misunderstanding that `reserve` initializes new elements in the vector. In contrast, it merely allocates the required memory. After reserving, the size of the vector remains unchanged until you add elements using methods such as `push_back`.
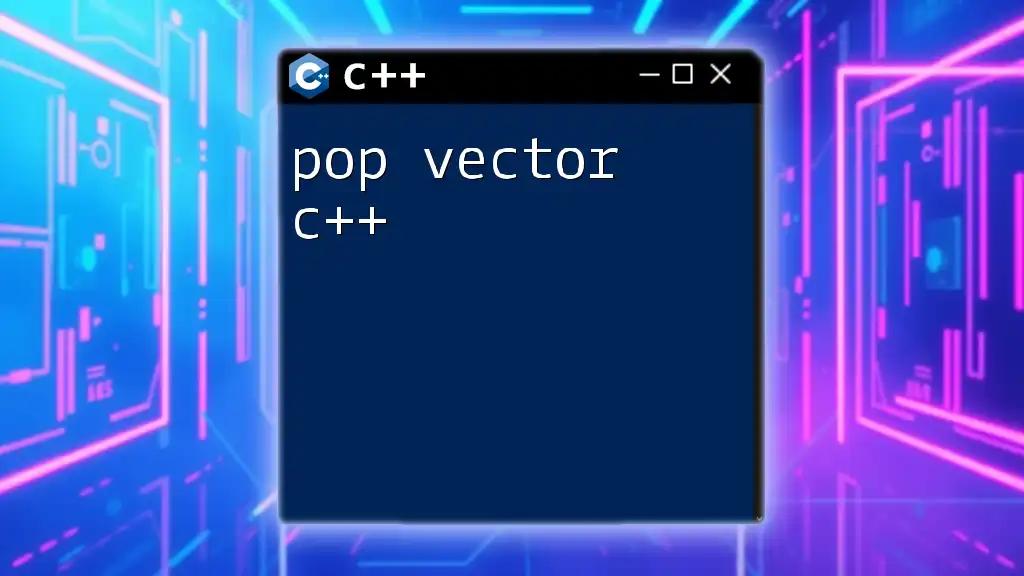
Performance Insights
Measuring Performance Improvements
Using `reserve` can lead to marked performance improvements, especially in loops or when handling bulk data. Consider running benchmarks to compare the performance of an operation using `reserve` versus without it, especially with large collections.
Best Practices for Using `reserve`
When working with vectors in C++, here are some guidelines for effectively using `reserve`:
- Estimate Required Capacity: If you have prior knowledge about the number of elements, always use `reserve` to minimize multiple allocations.
- Benchmark Performance: In performance-sensitive applications, utilize tools to measure the impact of `reserve` on memory usage and runtime.
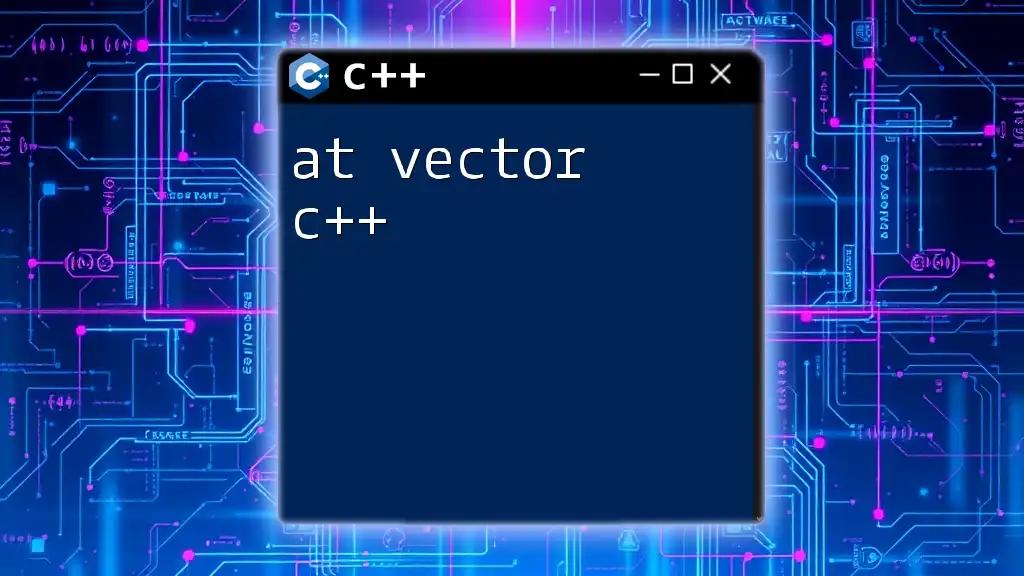
Conclusion
Understanding the mechanics of `reserve vector c++` is crucial for efficient memory management and performance optimization in your C++ programs. By reserving space promptly, you can significantly enhance your vector operations. With this knowledge, experiment with vectors in your code to see firsthand the benefits that `reserve` offers.
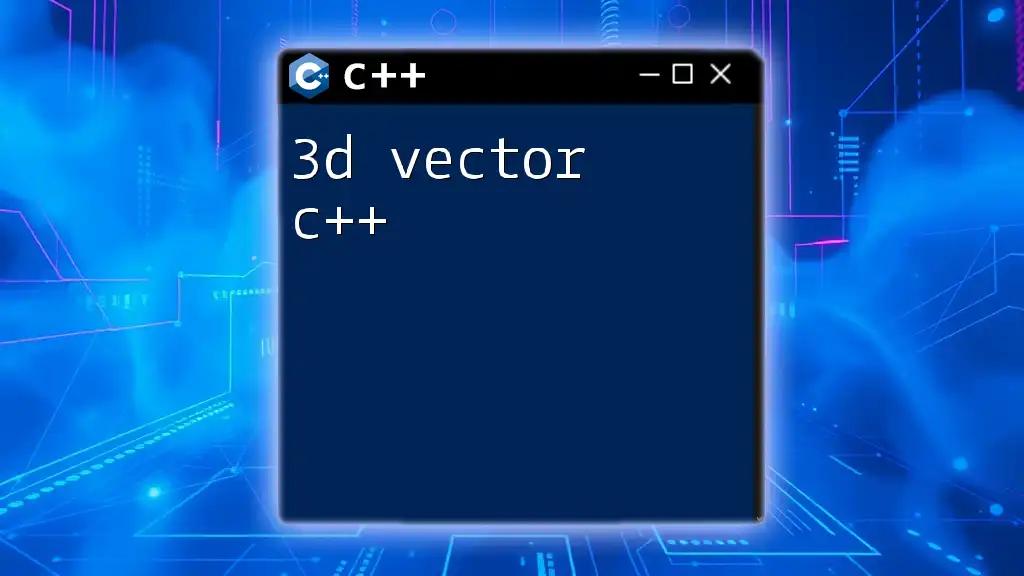
Additional Resources
For further reading, consider referring to the official C++ documentation on vectors to deepen your understanding, as well as tutorials that explore advanced topics in vector management.
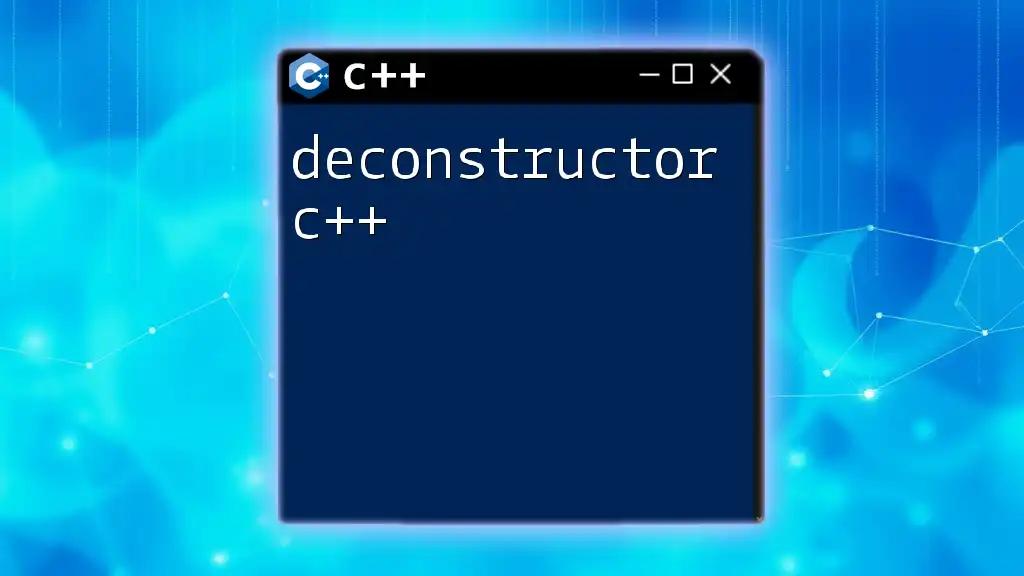
FAQs about `reserve` in C++
If you have specific questions regarding the use of `reserve`, don’t hesitate to explore forums or community boards. Engaging with fellow developers can shed light on additional use cases and best practices tailored to your unique projects. Remember, practical experience is the best teacher!