An iterator in C++ is an object that enables traversal through the elements of a container, such as a vector or list, without exposing the underlying structure of the container.
Here's a simple example demonstrating how to use an iterator with a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
What is an Iterator in C++?
An iterator in C++ is essentially a generalized pointer that allows traversing through elements of a collection, such as arrays, vectors, lists, and other containers. Unlike regular pointers, iterators provide a clean abstraction that allows programmers to interact with various data structures consistently.
Key Characteristics:
- Generalized Pointers: For instance, while you can use pointers to access array elements, iterators enable you to seamlessly work with data structures without worrying about their specific implementations.
- Abstraction: Because iterators abstract away the underlying data structure, they provide a uniform interface, making it easier to write generic algorithms.
Relevance: Iterators are critical in modern C++ programming. They simplify the process of traversing and manipulating collections while providing additional features such as insertion and deletion of elements.
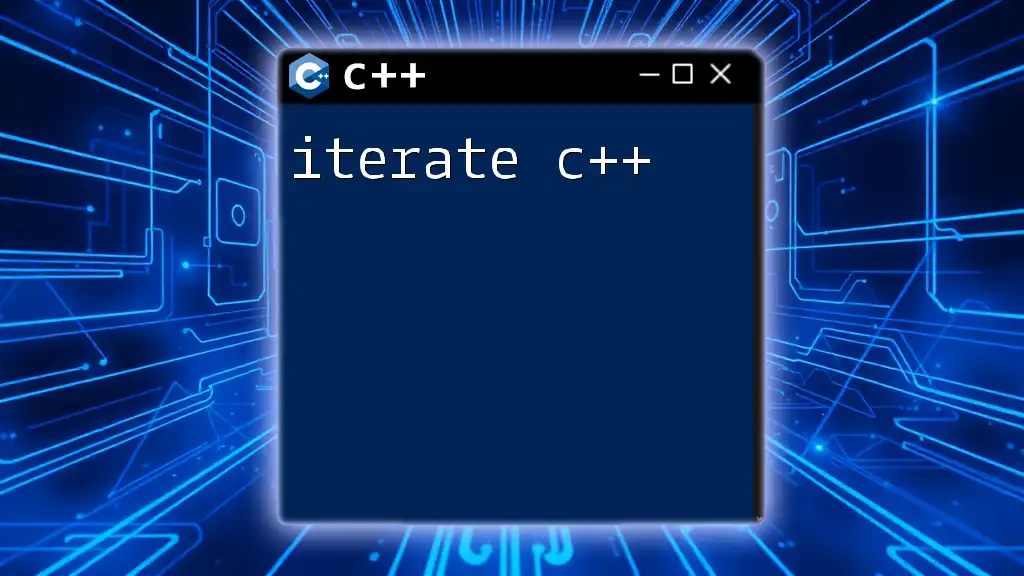
Types of Iterators in C++
Input Iterators
An input iterator allows reading elements from a container without modifying them. These are essential for input operations.
Example and Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::iterator it = vec.begin();
while (it != vec.end()) {
std::cout << *it << " ";
++it;
}
return 0;
}
In this example, we demonstrate how to traverse a vector using an input iterator. The code initializes an iterator `it` that points to the beginning of the vector and increments it until it reaches the end.
Use Cases and Limitations: Input iterators are crucial for algorithms that need to read data, but they typically have limited functionality since they can only move forward and don't support writing.
Output Iterators
On the other hand, output iterators allow modifying or writing elements in a container.
Example and Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::iterator it = vec.begin();
for (auto i = 0; i < vec.size(); ++i) {
std::cout << vec[i] << " ";
}
return 0;
}
In this example, we iterate over a vector using an output iterator. It's important to note that while we utilize iterators for reading, their main purpose is to write or output values to a container.
Applications: Output iterators are valuable when you need to write to a data structure, but once again, they have limitations in terms of access patterns.
Forward Iterators
Forward iterators allow traversing a container in a single direction but can be read multiple times. These iterators are useful for collections where repeated reading is required.
Example and Code Snippet:
#include <iostream>
#include <list>
int main() {
std::list<int> lst = {10, 20, 30};
std::list<int>::iterator it = lst.begin();
std::advance(it, 1); // Move to the second element
std::cout << *it << std::endl; // 20
return 0;
}
In this example, we use `std::advance` to move the iterator `it` to point to the second element of the list.
Use Cases and Advantages: Forward iterators allow for multiple passes over the same collection; they are more flexible for reading and slightly more versatile than output iterators.
Bidirectional Iterators
Bidirectional iterators extend the functionality of forward iterators by allowing movement in both directions.
Example and Code Snippet:
#include <iostream>
#include <set>
int main() {
std::set<int> s = {20, 10, 40};
std::set<int>::iterator it = s.end();
--it; // Move to last element
std::cout << *it << std::endl; // 40
return 0;
}
This example moves the iterator `it` back to the last element in a set, showcasing the capability of bidirectional iterators to travel both forwards and backwards.
Efficiency and Application Areas: Bidirectional iterators are handy when you need to traverse both forwards and backwards, making them particularly useful in data structures like `std::list` and `std::set`.
Random Access Iterators
The most powerful of iterators, random access iterators, allow direct access to any element in a container. They can move freely within the collection, offering the same capabilities as pointers.
Example and Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << vec[2] << std::endl; // Accessing the third element
return 0;
}
This code example demonstrates how a random access iterator can be used to access elements directly via indexing.
Advantages and When to Use Them: Random access iterators are essential for optimizing performance when random access is required, making them ideal for containers like `std::vector` and `std::array`.
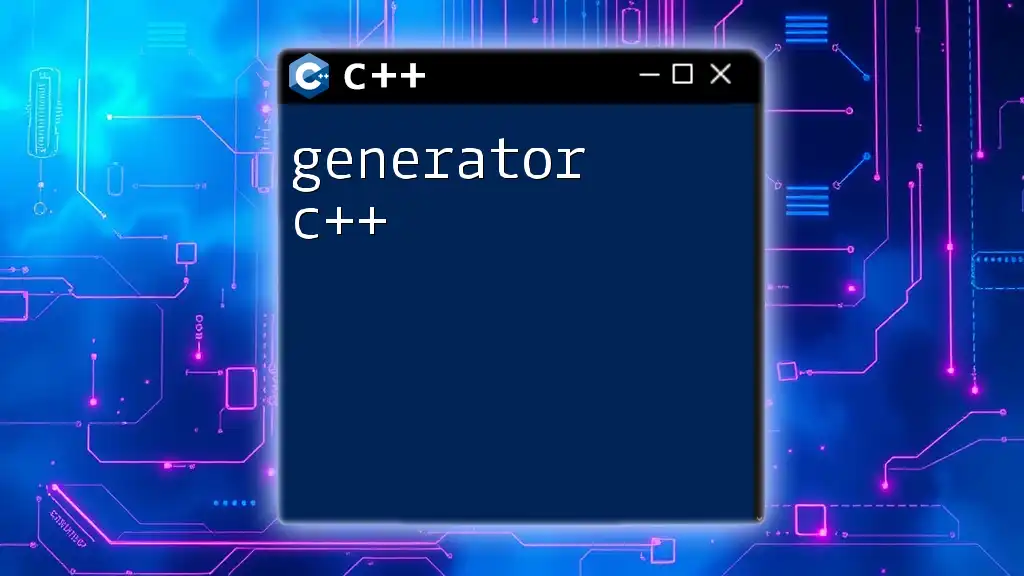
How to Use Iterators in C++
Simple Traversal
Using iterators for basic traversal of collections is straightforward. The following example illustrates how to traverse an array using iterators:
Code Snippet:
#include <iostream>
#include <array>
int main() {
std::array<int, 5> arr = {1, 2, 3, 4, 5};
for (auto it = arr.begin(); it != arr.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
The above example initializes an iterator `it` pointing to the beginning of the array and uses it to print each element.
Advanced Manipulation
Iterators also provide the ability to manipulate collections, such as inserting elements into them.
Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
auto it = vec.begin();
vec.insert(it + 1, 10); // Insert before the second element
for (int n : vec) {
std::cout << n << " "; // Output: 1 10 2 3
}
return 0;
}
This code illustrates how to use an iterator to insert a new element within the vector at a specified position.
Combining Iterators with STL Algorithms
One of the powerful features of iterators is their ability to work seamlessly with the Standard Template Library (STL) algorithms.
Code Snippet:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 3, 4, 1, 2};
std::sort(vec.begin(), vec.end());
for (int n : vec) {
std::cout << n << " "; // Sorted output
}
return 0;
}
This example shows how to sort a vector with the `std::sort` algorithm using iterators to define the range of elements to be sorted.
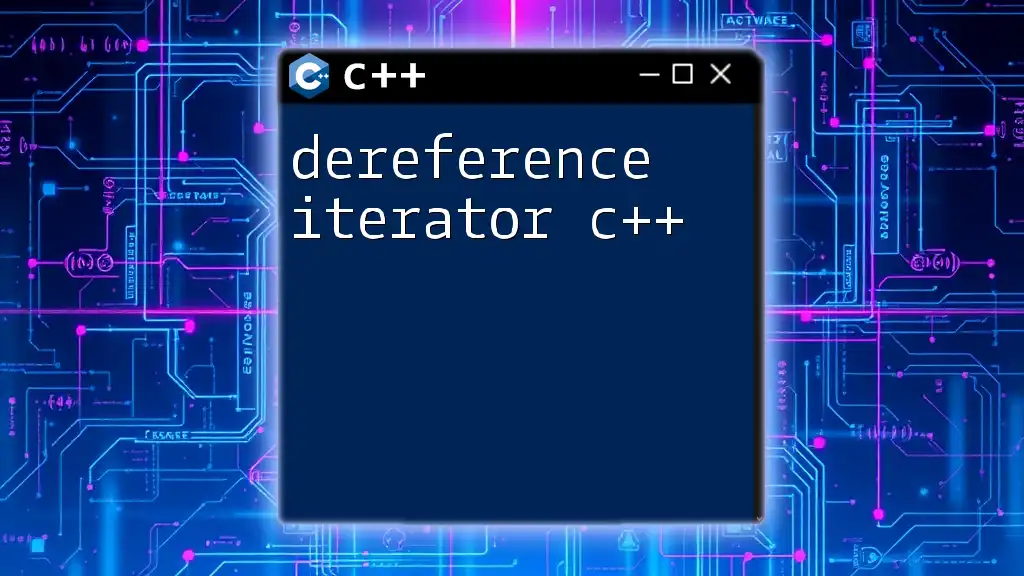
Conclusion
Iterators in C++ are an integral part of modern programming, providing a powerful tool for manipulating and traversing collections. Understanding the different types of iterators—input, output, forward, bidirectional, and random access—and knowing how to effectively use them can greatly enhance your coding efficiency and flexibility.
Engaging with iterators allows for cleaner and more maintainable code, especially when combined with STL algorithms. By practicing the use of iterators in various contexts, you develop a better understanding of their capabilities and can leverage their power in real-world applications.