The "and" operator in C++ is a logical operator that returns true if both operands are true, and it can be used as an alternative to the "&&" operator.
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a and b) { // uses the "and" operator
std::cout << "Both are true." << std::endl;
} else {
std::cout << "At least one is false." << std::endl;
}
return 0;
}
What is the And Operator in C++?
The and operator in C++ is a logical operator that allows you to evaluate multiple conditions. It serves to determine if two Boolean values are both true, returning true only when both are true.
Logical operators, such as the and operator, play a crucial role in controlling the flow of logic in software applications. They enable developers to create complex conditional statements that facilitate decision-making processes in code.
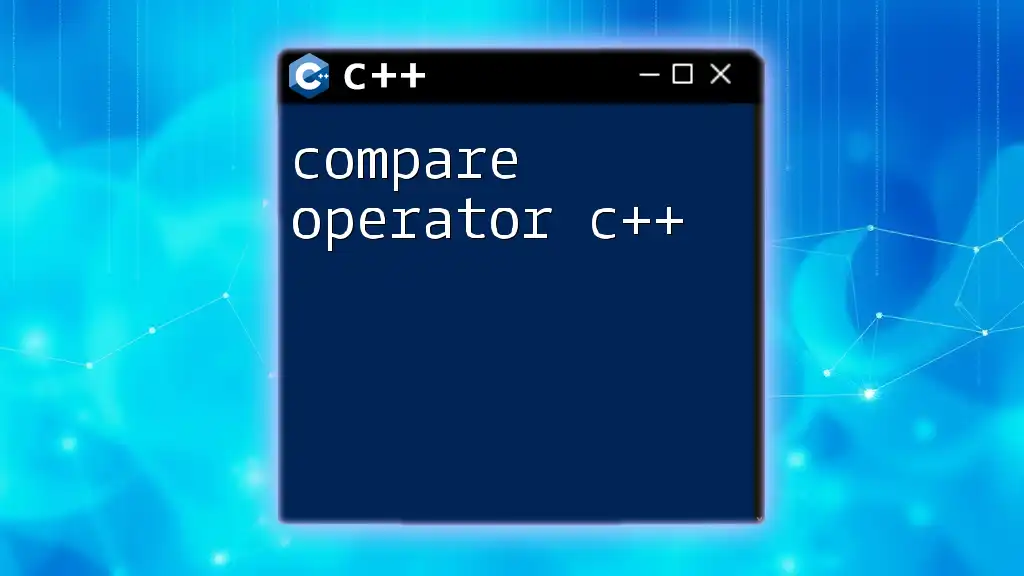
Importance of the And Operator
The and operator is essential in programming because it allows for highly specific condition checks. In practical terms, this means that you can combine multiple criteria in your decision-making processes, leading to more nuanced and robust code.
Example Use Case
Consider a scenario where you need to verify that a user meets certain criteria before allowing access to a system. The and operator can efficiently check these criteria in one concise statement.
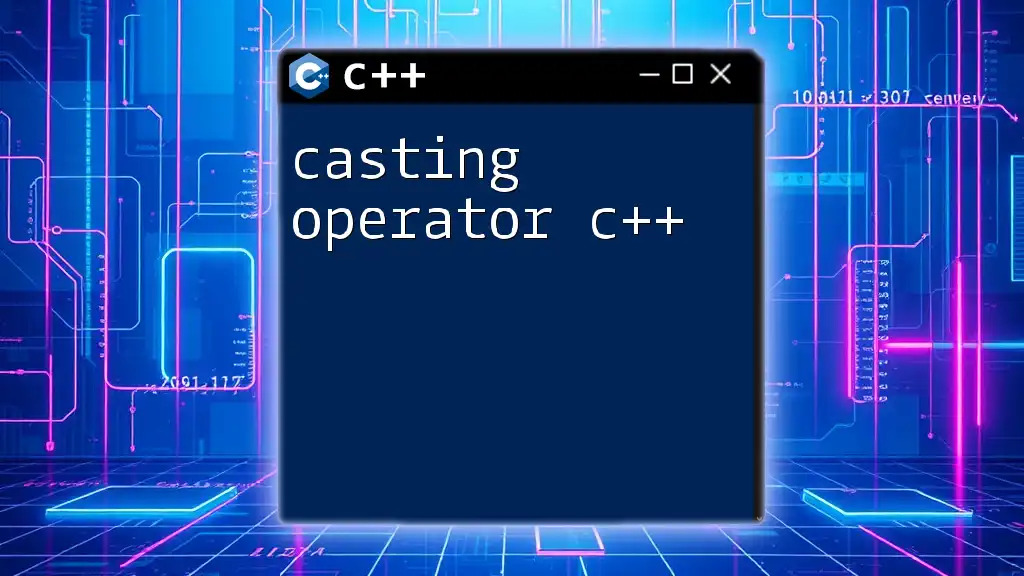
Understanding the And Operator
Definition and Syntax
In C++, the and operator is represented by `&&` but can also be written as the keyword `and` thanks to C++'s support for alternative representations.
Example:
bool result = (true && false); // result will be false
How the And Operator Works
The and operator evaluates expressions based on a simple truth table:
A | B | A && B |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
Through the above table, you can see that both conditions must be true for the expression as a whole to evaluate to true.
Short-Circuit Evaluation
One key feature of the and operator is its short-circuit evaluation. When evaluating conditions, if the first condition is false, the second condition is not even checked because the result is guaranteed to be false.
Example Demonstrating Short-Circuit Evaluation:
bool firstCondition = false;
bool secondCondition = (firstCondition && someFunction()); // someFunction() will not be executed
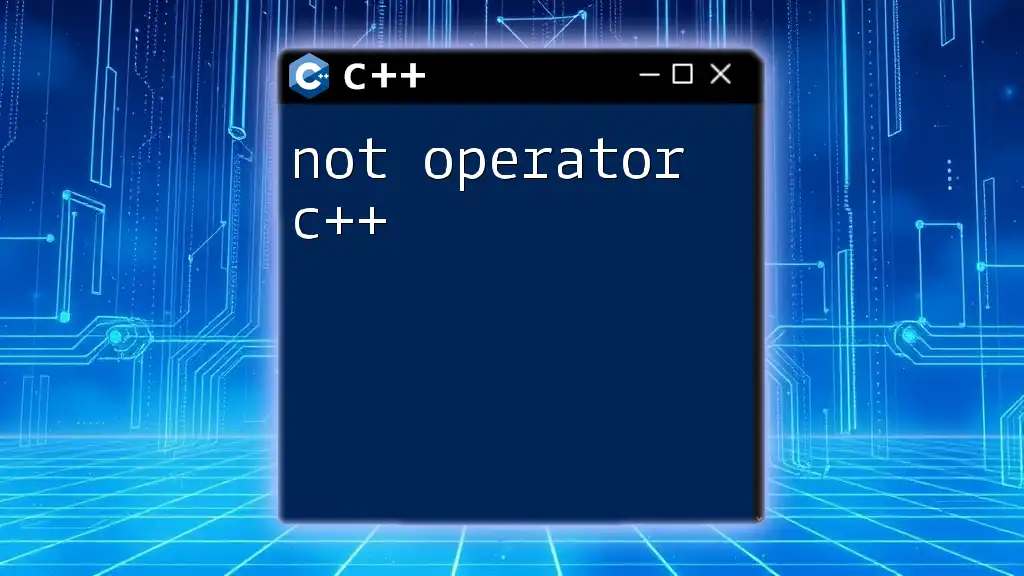
Using the And Operator in C++
Basic Usage of the And Operator
The and operator simplifies multiple condition evaluations. Its primary application is in conditional statements, where you want to check whether many criteria are simultaneously met.
Example:
bool a = true;
bool b = false;
if (a && b) {
// This block will not execute since b is false
}
Combining Multiple Conditions
Often, you want to combine more than two conditions, which is straightforward with the and operator.
Example:
int age = 25;
bool hasLicense = true;
if (age >= 18 && hasLicense) {
// The user is eligible to drive
}
This statement checks if the user is both 18 years or older and possesses a valid license.
The And Operator in Conditional Statements
The and operator finds practical application across various control structures such as `if`, `while`, and `for` statements, allowing seamless integration of multiple conditions.
Example with an `if` statement:
if (age >= 18 && hasLicense) {
// User can drive
}
Example with a `while` loop:
while (condition1 && condition2) {
// Execute code while both conditions are true
}
Example with a `for` loop:
for (int i = 0; i < 10 && i % 2 == 0; i++) {
// The loop only executes while i is less than 10 and i is even
}
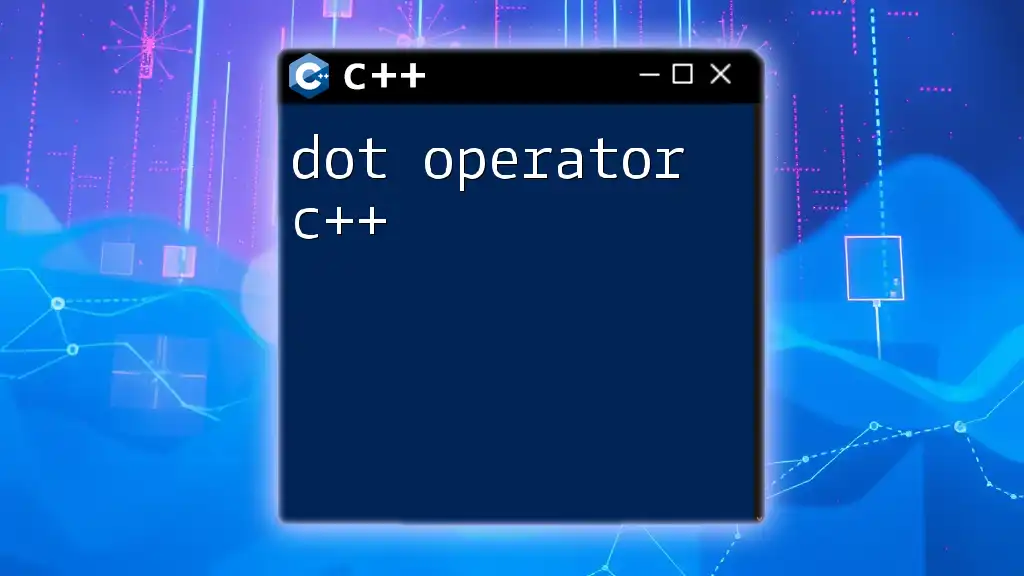
Practical Applications
Using the And Operator with Functions
You can write functions that harness the capabilities of the and operator. This promotes cleaner and more maintainable code.
Example of a Function:
bool isEligible(int age, bool hasLicense) {
return (age >= 18 && hasLicense);
}
This function checks if both conditions—age and possession of a license—are met, returning true if they are.
Error Handling with the And Operator
The and operator is also useful for error handling, particularly when validating user input.
Scenario-based Example:
std::string username;
std::string password;
if (!username.empty() && !password.empty()) {
// Proceed with authentication
}
Here, the code checks whether both username and password fields are filled before proceeding, enhancing user experience and software stability.
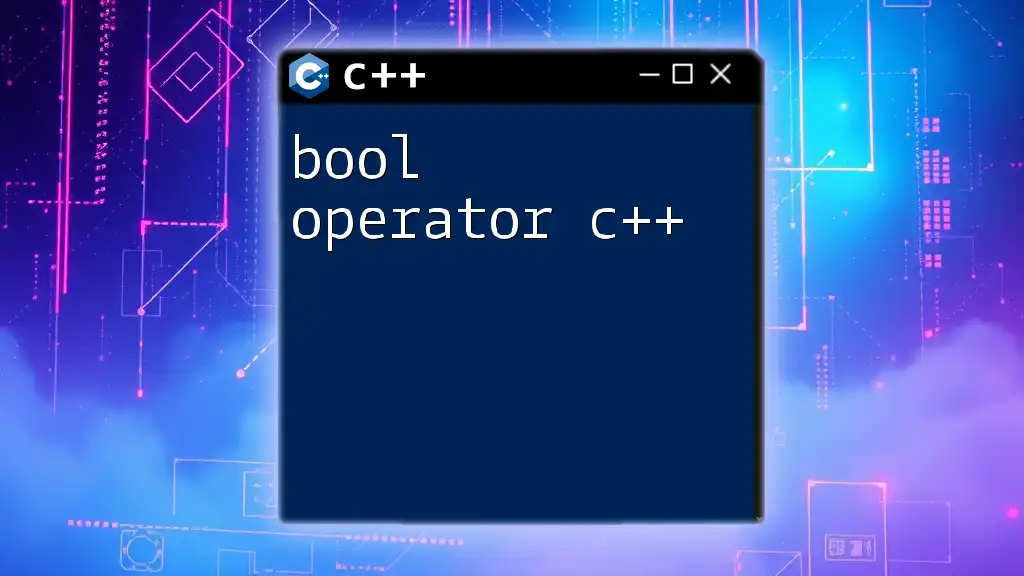
Common Mistakes to Avoid
Misunderstanding Short-Circuit Evaluation
New C++ developers might misunderstand how short-circuit evaluation works, resulting in unexpected behavior. It's crucial to understand that if the first condition in an `&&` expression is false, the second condition will not execute.
Neglecting Parentheses
Even though the precedence of the and operator is clear, it's a good practice to use parentheses. This can clarify your intentions and prevent potential mistakes.
Example Demonstrating Confusion:
if (a && b || c) { /* Confusing without parentheses */ }
// Better written as:
if ((a && b) || c) { /* Clear intention */ }
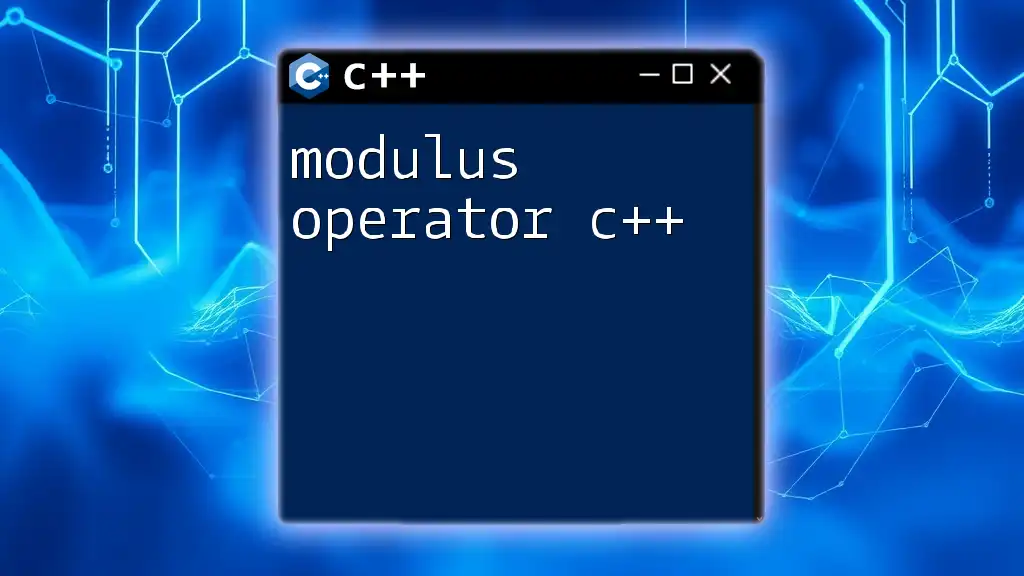
Conclusion
The and operator in C++ is an essential tool for creating logical conditions and enhancing decision-making capabilities in programming. Mastering this operator can significantly improve your coding techniques and lead to more efficient and error-free applications.
Engage with practical projects, explore different use cases, and familiarize yourself with these concepts to solidify your understanding and application of the and operator.
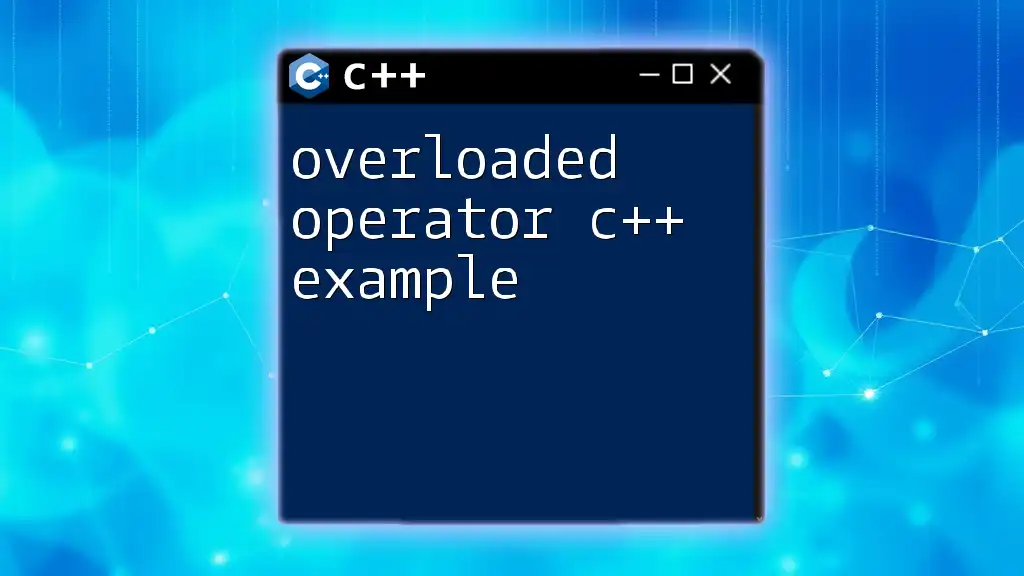
Additional Resources
Recommended Reading
To further deepen your understanding of C++, consider exploring popular textbooks and online courses tailored for both beginners and advanced programmers.
Community and Forums
Joining C++ programming forums can facilitate learning by allowing you to ask questions and engage with a community of experienced developers.
Q&A Section
Feel free to leave comments or questions regarding the and operator, as interaction fosters broader learning opportunities for all readers.