The `not` operator in C++ is a logical negation operator that reverses the boolean value of its operand.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
int main() {
bool value = true;
std::cout << "The negation of value is: " << not value << std::endl; // Output: 0 (false)
return 0;
}
Understanding the Not Operator
What is the Not Operator?
The not operator in C++ is a logical operator that is used to reverse the truth value of a given boolean expression. Its syntax is:
not expression
This means if the expression evaluates to true, using the not operator will return false, and vice versa.
How the Not Operator Works
To understand the functionality of the not operator, it's useful to examine a basic truth table:
Expression | Result of `not expression` |
---|---|
true | false |
false | true |
From this, one can see that the not operator negates the logical value of an expression, making it a powerful tool in conditional programming.
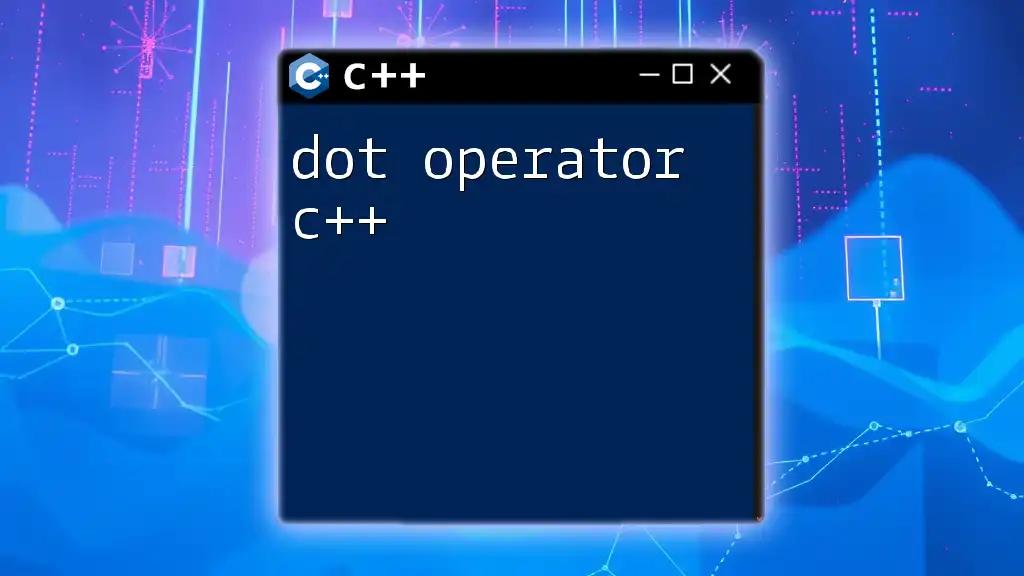
Using the Not Operator in C++
Basic Syntax
The simplest usage of the not operator can be found in conditional statements. For instance:
bool condition = false;
if (not condition) {
// Code to execute if condition is false
}
In this example, since condition is false, the statement within the if block will be executed. This showcases how you can easily express logical conditions using the not operator.
Comparison with Alternative Syntax
Many developers are accustomed to using `!` as the negation operator. The following example illustrates this:
bool condition = false;
if (!condition) {
// Code to execute when condition is false
}
While both `not` and `!` achieve the same result, using the keyword not may enhance readability, especially for those less familiar with programming syntax. This choice ultimately depends on the coding standards of your project or your personal preference.
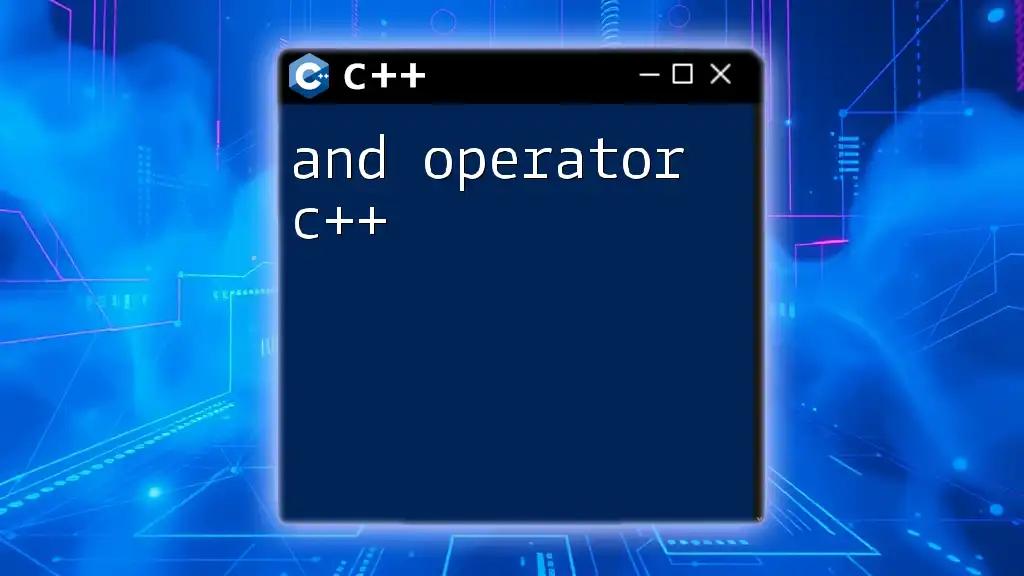
Practical Applications of the Not Operator
Conditional Statements
The not operator is particularly useful in constructing conditional statements. Consider the following:
int num = 5;
if (not (num > 10)) {
// Code to execute if num is NOT greater than 10
}
In this instance, the condition checks whether num is not greater than 10. If we apply the not operator effectively, we ensure that the logic flows clearly, making our intent easier to grasp.
Loops and the Not Operator
You can also use the not operator within looping constructs. Here’s an example using a while loop:
bool active = true;
while (not active) {
// Code that runs while active is NOT true
}
In this situation, the loop will not execute because active starts as true. However, using the not operator in loop conditions can help simplify complex logic by negating defining terms.
Combine with Other Logical Operators
The not operator can also be effectively combined with and/or operators to create robust logical statements:
bool conditionA = true;
bool conditionB = false;
if (not (conditionA && conditionB)) {
// Code executes if NOT both conditions are true
}
Here, the condition checks if not both conditionA and conditionB are true. This combination enhances logical flow and reduces potential confusion in your code.
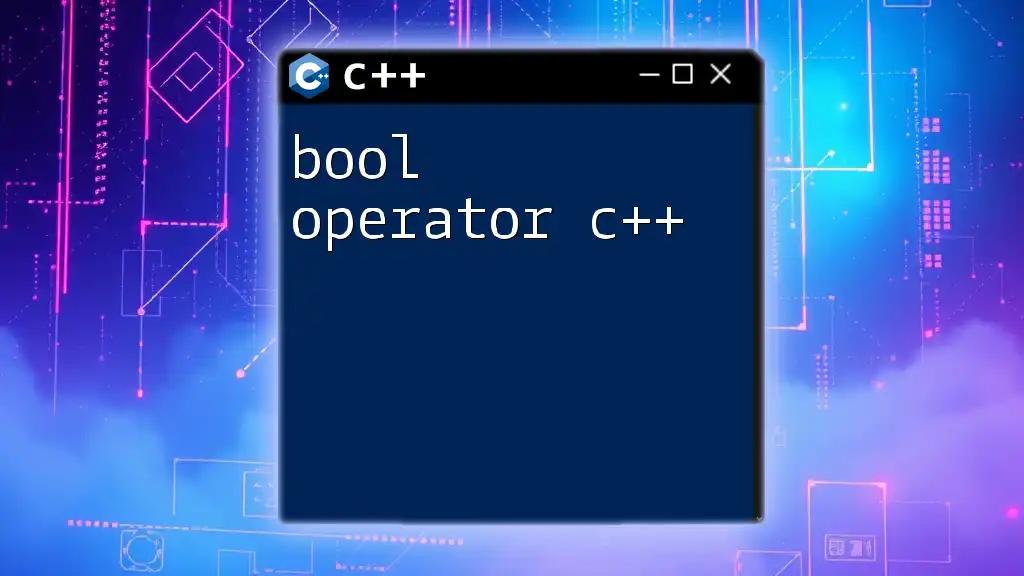
Common Mistakes with the Not Operator
Misunderstanding Boolean Logic
Developers frequently misunderstand boolean logic, leading to improper use of the not operator. It's essential to clearly understand the underlying logic and test conditions before applying the not operator to ensure the desired effect is achieved.
Negating Expressions Incorrectly
An example of misuse might involve misapplying the not operator to expressions that involve multiple conditions. Clarity matters, and being careful with the placement of brackets can prevent errors.
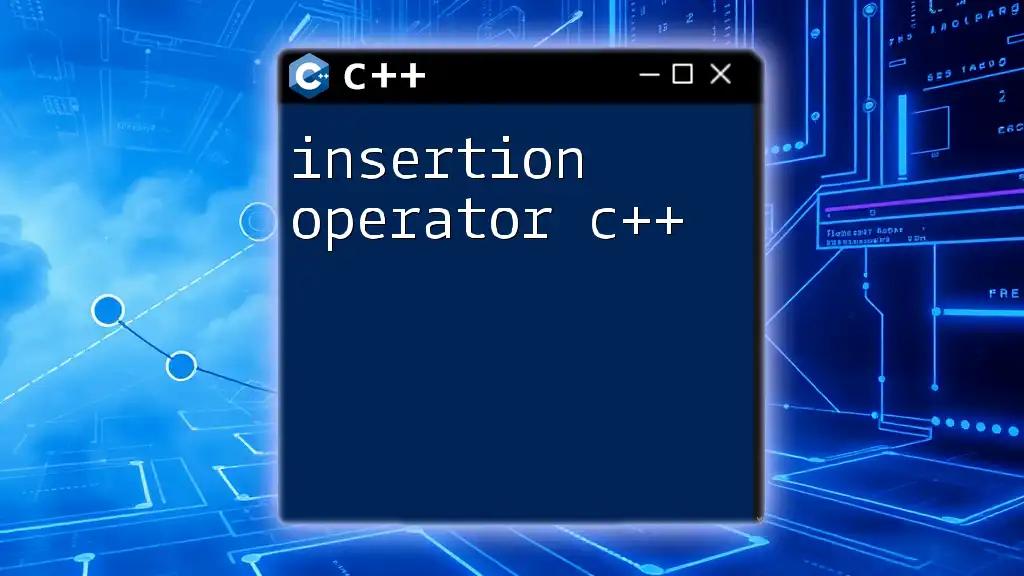
Alternatives and Best Practices
Using Readable Code with Not
Choosing keywords like not can sometimes lead to more readable code compared to traditional symbols:
if (not (x == 0)) {
// Actions when x is not zero
}
In cases of complex conditions, trading off brevity for clarity by using not can significantly enhance the maintainability of your code.
README: When to Avoid Not Operator
Despite its usefulness, there are instances where the not operator complicates logic more than it clarifies it. In cases where expressions are already straightforward or using nested negations, it's wiser to revert to simpler structures or avoid using not altogether.
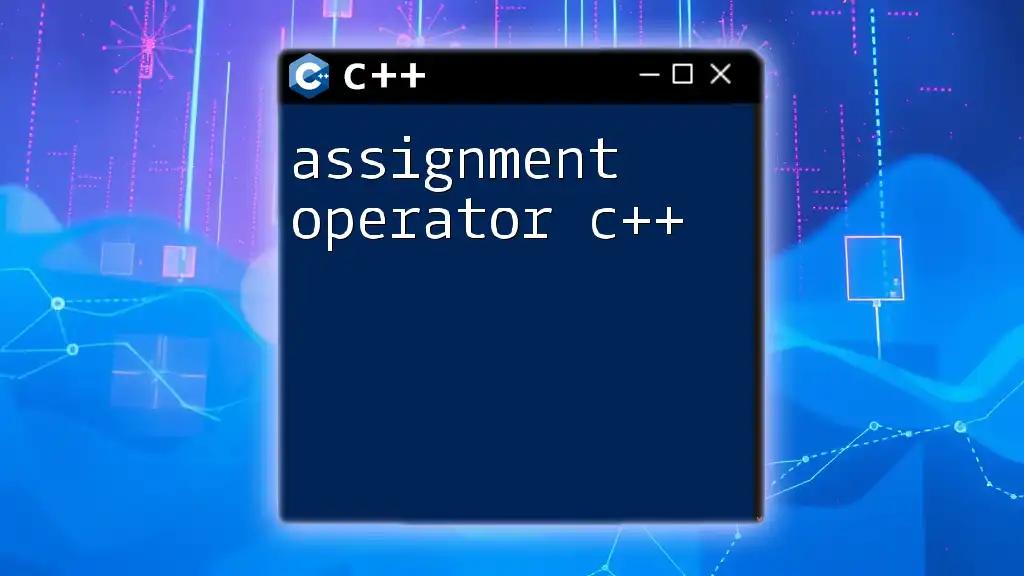
Conclusion
The not operator in C++ serves a critical role in making boolean logic accessible and flexible. By understanding its syntax, application, and common pitfalls, developers can leverage this operator effectively in their coding.
In summary, mastering the not operator will enhance your logical expression capabilities and enable clearer, more concise coding practices. It is an essential tool in a C++ programmer's toolkit, deserving attention and practice.
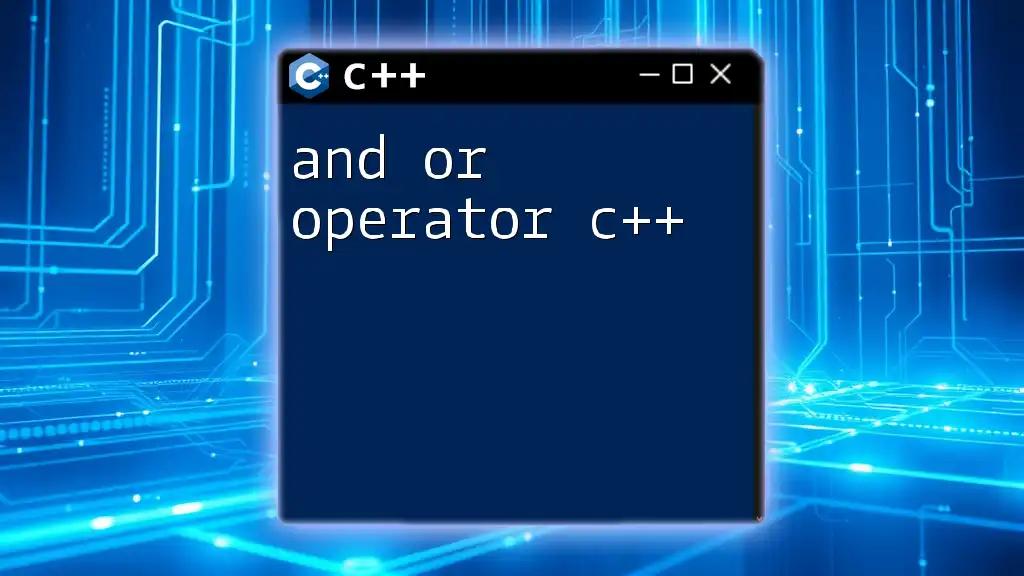
Additional Resources
For further exploration, consider delving into C++ programming tutorials online or examining comprehensive reference guides. Practice makes perfect; try coding exercises that specifically focus on the logical operators.

Call to Action
We invite you to share your own experiences or queries regarding the not operator in C++. Join our community to further refine your understanding of C++ commands!