A conversion operator in C++ allows an object of a class to be implicitly converted to a different type, simplifying type casting.
Here's a code snippet that demonstrates a conversion operator:
class Distance {
public:
Distance(int m) : meters(m) {}
// Conversion operator to convert Distance to int
operator int() const {
return meters;
}
private:
int meters;
};
int main() {
Distance d(5);
int m = d; // Implicitly calls the conversion operator
}
Understanding C++ Conversion Operators
What is a Conversion Operator?
A conversion operator is a special member function in C++ that is used to define how an object of a certain class can be converted to another type. This operator enables implicit or explicit conversion between user-defined types and built-in types. Essentially, it provides a way for custom classes to be used interchangeably with fundamental types when needed.
The Need for Conversion Operators
As you work with object-oriented programming in C++, you may encounter situations where it's necessary to convert a class object to a different type. For instance, in mathematics, a `Fraction` class might need to be used in conjunction with `double`s for calculations. The conversion operator enables this by allowing automatic type conversion, enhancing code usability and integration.
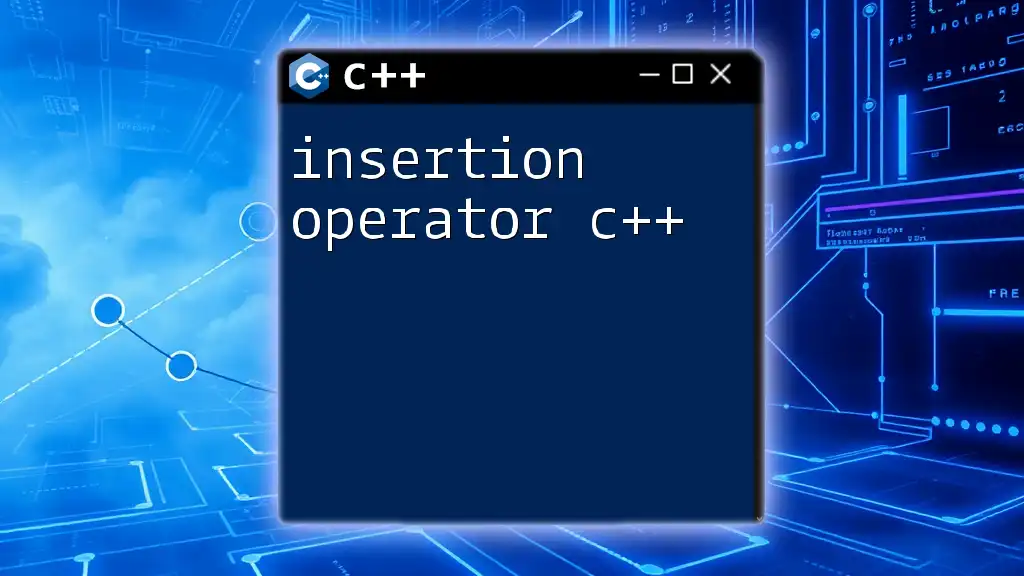
Syntax of Conversion Operators
Basic Syntax
The syntax for declaring a conversion operator is straightforward. It includes the `operator` keyword, followed by the type to which you want to convert:
operator Type() { ... }
This defines a member function that will be called whenever an object of the class is used in a context where it is expected to be of the specified type.
Example of Basic Conversion Operator Syntax
Consider the following `Fraction` class:
class Fraction {
public:
int numerator;
int denominator;
// Conversion operator for double
operator double() const {
return static_cast<double>(numerator) / denominator;
}
};
In this example, the `Fraction` class has a conversion operator that allows it to be treated as a `double`. The `static_cast` ensures that the division yields a double value, preserving numerical precision.
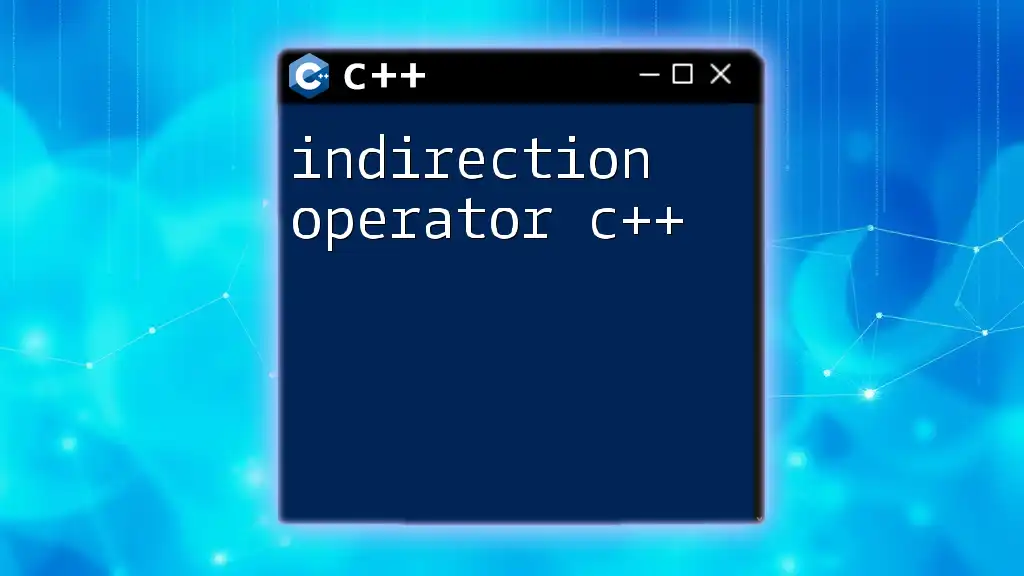
Types of Conversion Operators
Implicit Conversion
Implicit conversion occurs automatically when a class object is used in a context where a different type is expected. This feature is particularly useful but should be implemented carefully to avoid unwanted conversions.
Example of Implicit Conversion Operator
Here's an example with the `Celsius` class:
class Celsius {
public:
double temperature;
Celsius(double temp) : temperature(temp) {}
// Implicit conversion to double
operator double() const {
return temperature;
}
};
In this case, you can instantiate a `Celsius` object and directly assign it to a `double` variable without an explicit cast, as shown below:
Celsius c(100);
double temp = c; // Implicit conversion to double
This demonstrates the convenience of conversion operators in real-world applications.
Explicit Conversion
On the other hand, explicit conversions require a cast from the user, adding a layer of safety against unintended type changes. The `explicit` keyword can be used to define such conversions.
C++11 `explicit` Keyword
Using the `explicit` keyword prevents the compiler from performing implicit conversions, nudging programmers to opt for safer and clearer code.
Example of Explicit Conversion Operator
Take the following `Kelvin` class as an example:
class Kelvin {
public:
double temperature;
Kelvin(double temp) : temperature(temp) {}
// Explicit conversion to double
explicit operator double() const {
return temperature - 273.15; // Convert Kelvin to Celsius
}
};
Using the explicit conversion operator:
Kelvin k(300);
double temp = static_cast<double>(k); // Explicit cast is required
This approach ensures developers clearly understand when conversions are happening, improving code safety and readability.
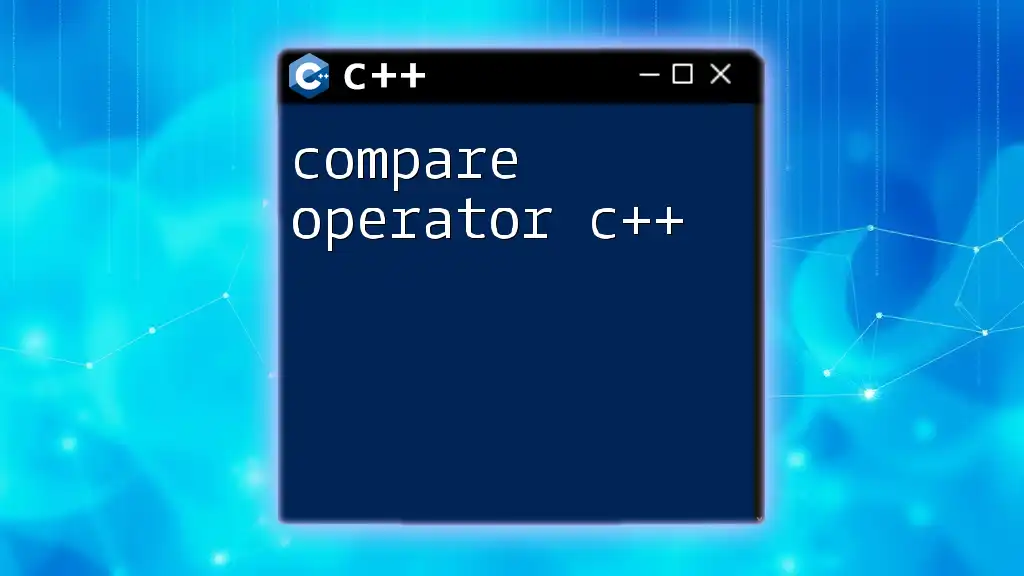
Overloading Conversion Operators
Overloading Conversion Operators for User-Defined Types
C++ allows overloading conversion operators for user-defined types to broaden the usability of objects. By overloading a conversion operator, you can define multiple ways an object can be treated as a different type.
Example Code Demonstration
Consider the `Complex` class, which represents a complex number:
class Complex {
public:
double real;
double imag;
Complex(double r, double i) : real(r), imag(i) {}
// Conversion operator to string
operator std::string() const {
return std::to_string(real) + " + " + std::to_string(imag) + "i";
}
};
Here, the conversion operator allows a `Complex` object to be converted to a `std::string`. This makes it possible to directly use `Complex` objects in string contexts, enhancing flexibility:
Complex c(3.5, 2.0);
std::string complexStr = c; // Conversion to string
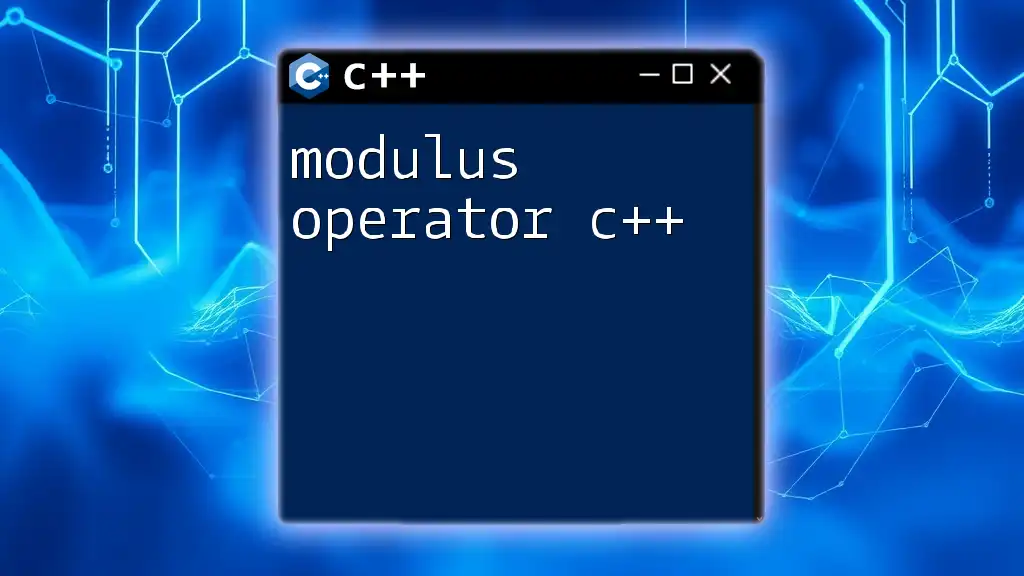
Best Practices for Using Conversion Operators
Avoid Ambiguity
One of the key consideration when using conversion operators is to avoid ambiguity. If multiple conversions can apply to a context, the compiler may fail to determine which conversion to use, leading to errors in your code. Strive to keep the number of conversion operators minimal to ensure clarity.
Use with Caution
While conversion operators can enhance flexibility, it’s essential to use them judiciously. Overusing conversions can make code harder to understand and maintain. Prioritize explicit over implicit conversions wherever possible to maintain clarity for future developers (or your future self).
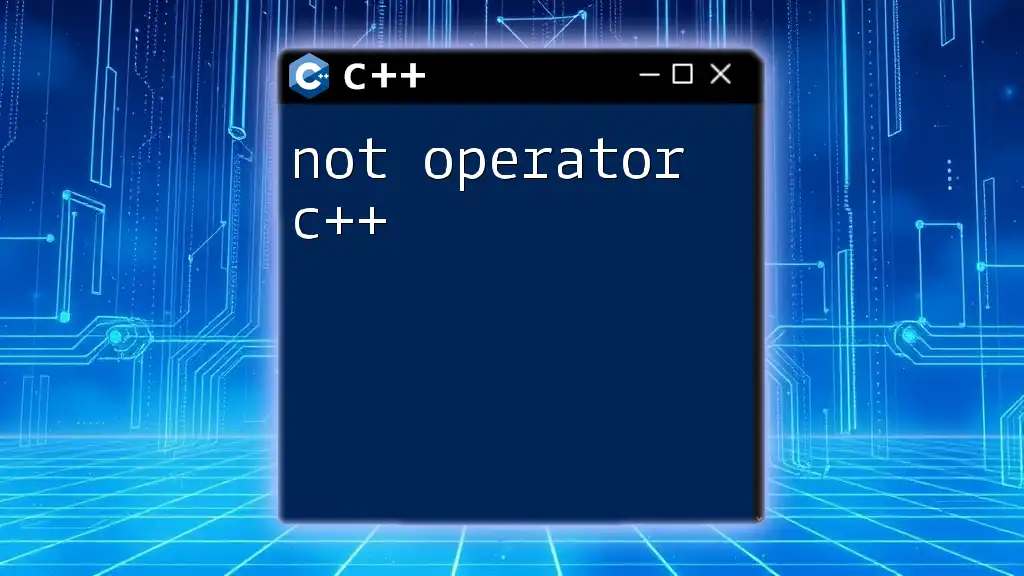
Common Mistakes with Conversion Operators
Misuse of Conversion Operators
A common pitfall is misusing conversion operators, leading to unintended consequences or confusion within the program. Over-reliance on implicit conversions can obscure logic and make debugging difficult.
Real-World Example of Mistakes
Consider the following example:
class String {
public:
const char* str;
// Dangerous conversion to const char*
operator const char*() {
return str;
}
};
Here, converting a `String` object directly to `const char*` can lead to serious issues if the `str` member is not properly managed. Such misuse highlights the importance of assessing the potential risks associated with enabling automatic conversions.
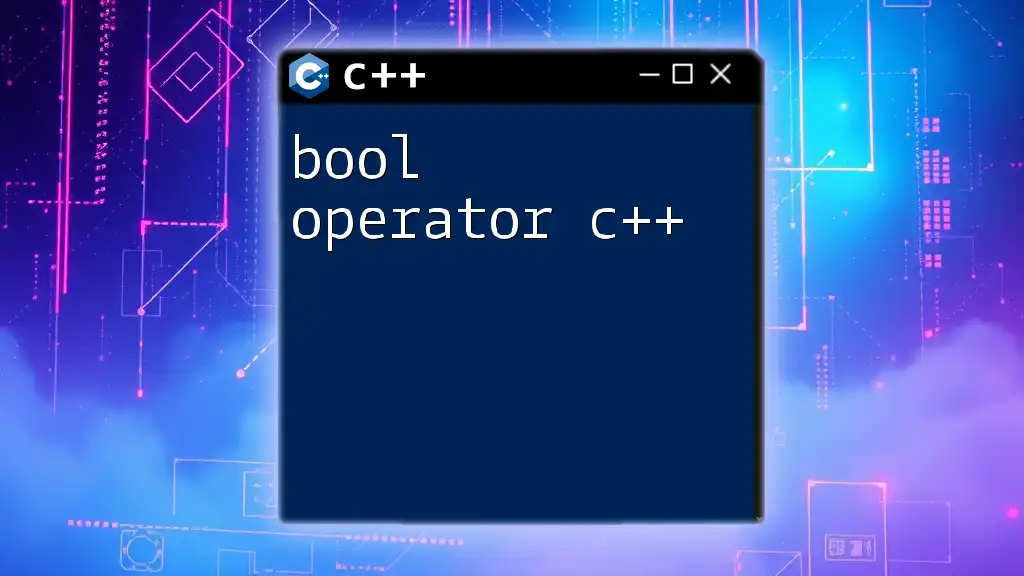
Conclusion
Recap of Key Points
In summary, conversion operators in C++ are powerful tools that allow you to define how your classes interact with other types. By understanding implicit versus explicit conversions and implementing them thoughtfully, you can enhance the usability of your classes while maintaining clarity and safety in your code.
Further Learning Resources
For those interested in delving deeper into conversion operators, consider exploring authoritative books on C++, online courses that focus on object-oriented programming, and official C++ documentation for nuanced guidance on best practices and advanced techniques.
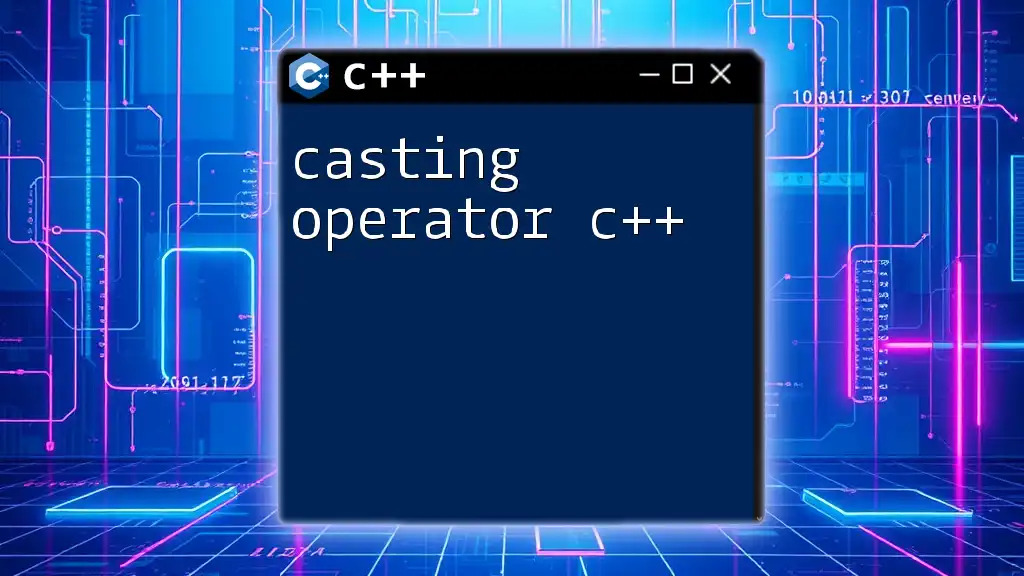
Call to Action
We invite you to stay tuned for more concise guides on mastering C++ programming. Share your experiences with conversion operators or any challenges you've faced in your coding journey; your insights could inspire other developers navigating similar paths.