In C++, the "and" and "or" operators are logical operators that serve as alternative representations for "&&" (logical AND) and "||" (logical OR), respectively, allowing for more readable code.
Here's a code snippet demonstrating their use:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a and b) {
std::cout << "Both are true" << std::endl;
} else if (a or b) {
std::cout << "At least one is true" << std::endl;
} else {
std::cout << "Both are false" << std::endl;
}
return 0;
}
Understanding Logical Operators in C++
What Are Logical Operators?
Logical operators are crucial in programming, as they allow developers to make decisions based on multiple conditions. These operators evaluate boolean expressions, returning either `true` or `false`. The logical operators commonly used in C++ include the “and” operator (`&&`) and the “or” operator (`||`). Understanding how to effectively use these operators is essential for controlling the flow of your programs.
Overview of the "And" and "Or" Operators
In C++, the logical “or” operator allows you to test multiple expressions, returning `true` if at least one of the expressions evaluates to `true`. Conversely, the “and” operator returns `true` only if all expressions are `true`. These operators can be represented either by their symbolic forms or their keyword variants — `||` for “or” and `&&` for “and”.
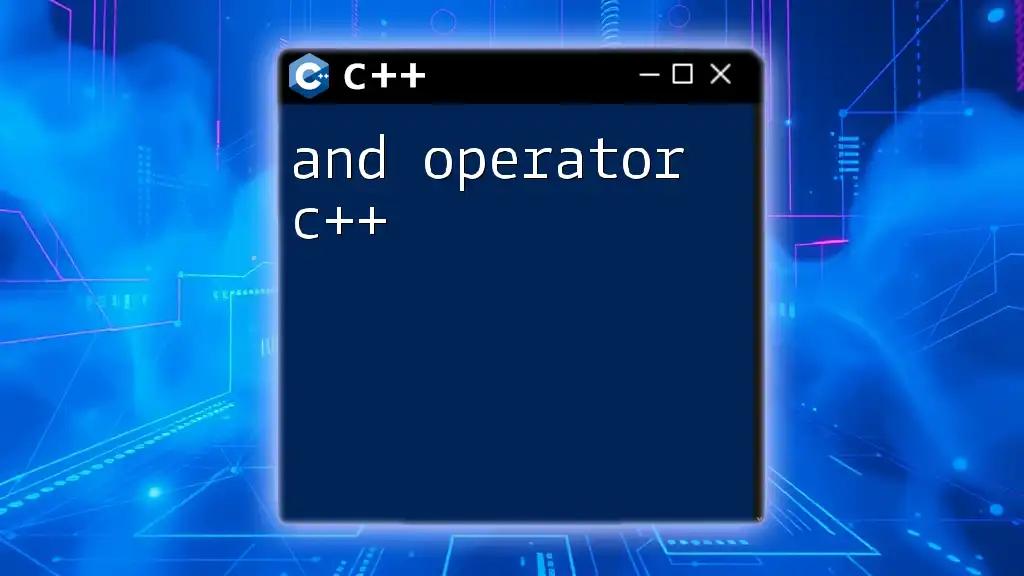
The "Or" Operator in C++
What is the "Or" Operator?
The “or” operator in C++ is used to combine multiple boolean expressions. It allows for flexibility in decision-making by providing an alternative condition that, if met, will result in a `true` outcome. Its symbolic representation is `||`, but you can also use the keyword `or`, making your code clear and readable.
Syntax and Usage
The basic syntax for using the “or” operator in C++ is straightforward. You can use it within an `if` statement or any conditional structure:
if (condition1 || condition2) {
// execute code if either condition is true
}
Example of "Or" Operation
Here’s a simple example to illustrate the usage of the “or” operator:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a || b) {
cout << "At least one is true!" << endl;
}
return 0;
}
In this example, since `a` is `true`, the output will be "At least one is true!". If both `a` and `b` were `false`, the `if` block would not execute.
Combining "Or" with Other Conditions
The “or” operator can be combined with other conditions to create more complex logical statements. You can chain multiple conditions using `||`, enhancing the flexibility of your decision-making processes.
Example of Multiple "Or" Operation
Consider the following code, which checks a person's age:
#include <iostream>
using namespace std;
int main() {
int age = 20;
if (age < 18 || age > 65) {
cout << "Eligible for special program." << endl;
}
return 0;
}
In this case, if `age` is between 18 and 65, the message won't print. However, if `age` is less than 18 or greater than 65, the program will indicate eligibility for a special program.
Short-circuit Evaluation with "Or"
In C++, logical expressions involving the “or” operator utilize short-circuit evaluation. This means that if the first condition evaluates to `true`, the remaining conditions are not evaluated, saving computational resources. This behavior is particularly useful when the conditions involve function calls, as it can prevent unnecessary executions and possible errors.
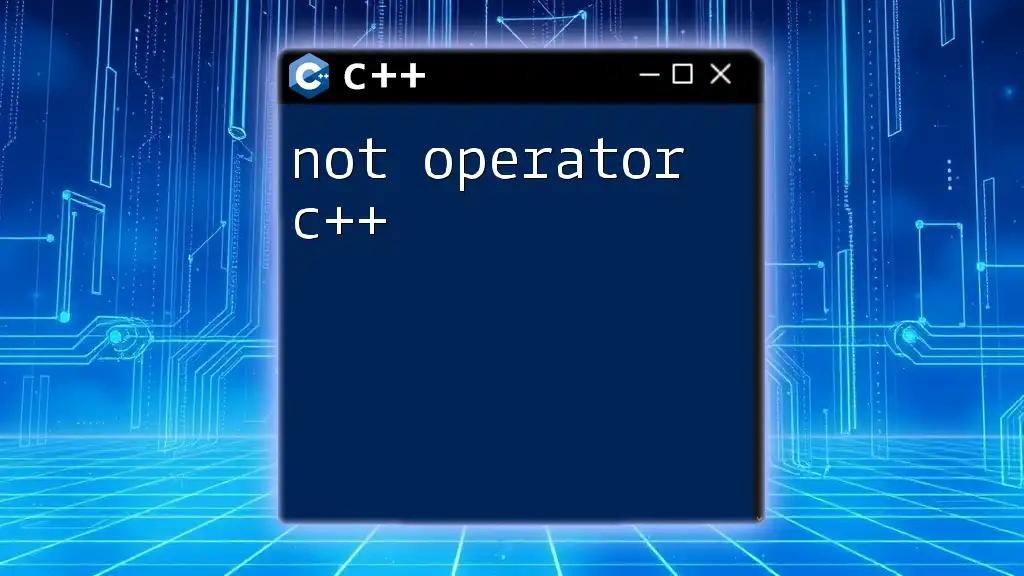
The "And" Operator in C++
What is the "And" Operator?
The “and” operator serves as a way to confirm that multiple conditions are satisfied simultaneously. Its symbolic form is `&&`, while its keyword variant is `and`. Understanding how to utilize the “and” operator can help create precise conditional checks.
Syntax and Usage
Using the “and” operator is similar to the “or” operator:
if (condition1 && condition2) {
// execute code if both conditions are true
}
Example of "And" Operation
Here is an example that demonstrates how the “and” operator works:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = true;
if (a && b) {
cout << "Both are true!" << endl;
}
return 0;
}
In this case, both `a` and `b` are `true`, so the output will be "Both are true!". If either `a` or `b` were `false`, the condition would not hold, and the message would not print.
Combining "And" with Other Conditions
Like the “or” operator, you can chain multiple conditions using the “and” operator. This capability allows for intricate logical checks.
Example of Multiple "And" Operation
Here’s a quick illustration:
#include <iostream>
using namespace std;
int main() {
int age = 30;
if (age >= 18 && age < 65) {
cout << "Eligible for regular program." << endl;
}
return 0;
}
In this example, if `age` lies between 18 and 65, the program confirms eligibility for the regular program. If outside this range, the message won’t display.
Short-circuit Evaluation with "And"
Applying short-circuit evaluation to the “and” operator works similarly to the “or” operator. If the first condition is `false`, C++ doesn’t evaluate the rest, which can improve performance and prevent unnecessary computations.
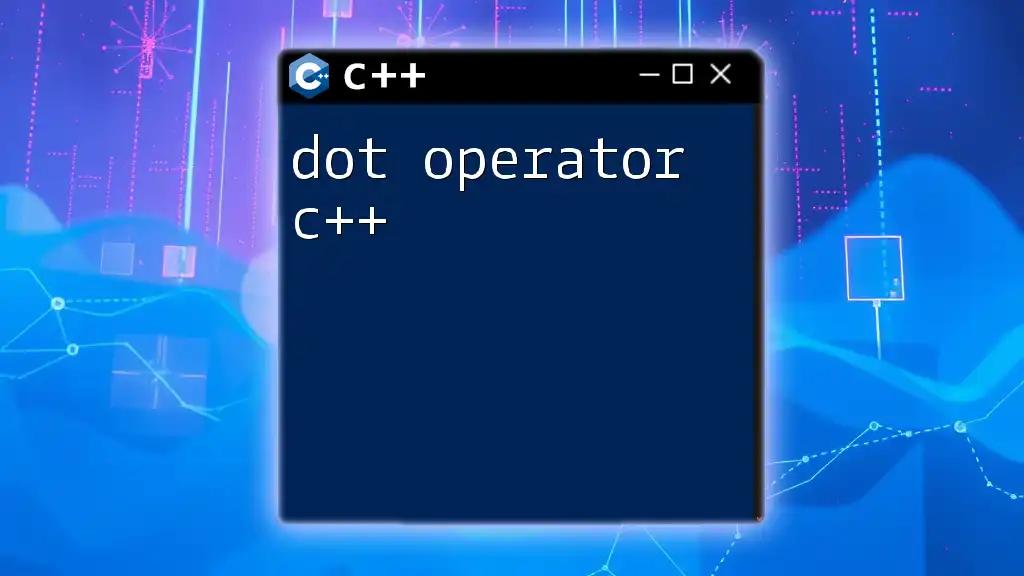
Combining "And" and "Or" Operators
Complex Conditions
Using both the “and” and “or” operators together enables the creation of more complex decision structures. Combining these operators can lead to nuanced logical checks where multiple criteria must be evaluated.
Example of Combined Conditions
Below is an example of how to effectively combine both operators:
#include <iostream>
using namespace std;
int main() {
int age = 20;
bool isStudent = true;
if ((age < 18 || age > 65) && isStudent) {
cout << "Eligible for special student program." << endl;
}
return 0;
}
In this case, the program checks if the person is either younger than 18 or older than 65, while also confirming if they are a student. If both conditions are met, it outputs eligibility for a special program.
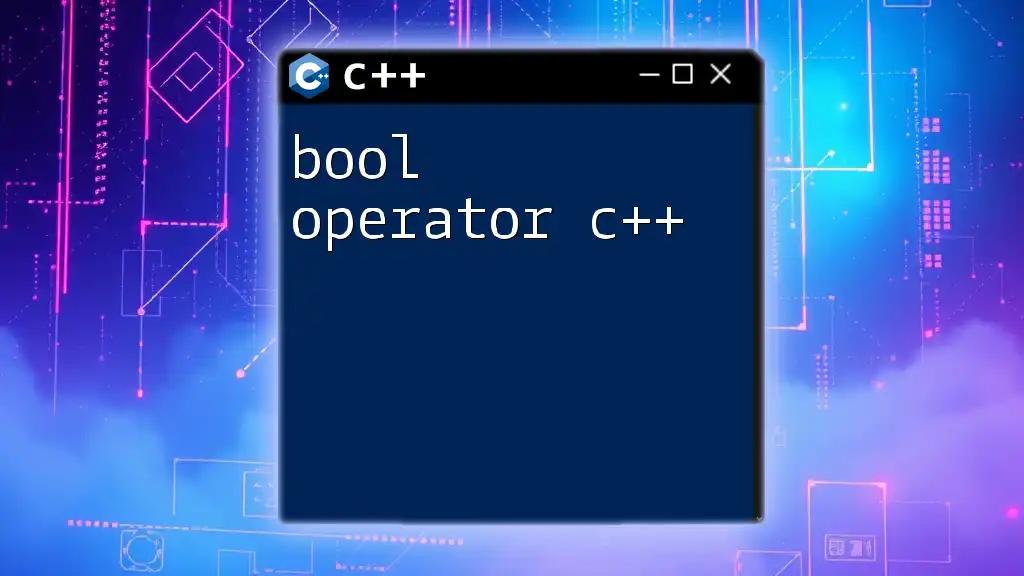
Error Handling with Logical Operators
Common Mistakes to Avoid
When using logical operators, be mindful of common pitfalls. One frequent mistake is treating the operators as if they exhibit arithmetic behavior. Ensure that conditions are properly evaluated to avoid unexpected results.
Best Practices
To enhance code readability and maintainability, strive to use parentheses to clarify the order of operations. This practice aids others (and yourself) in understanding the flow of logic within your code.
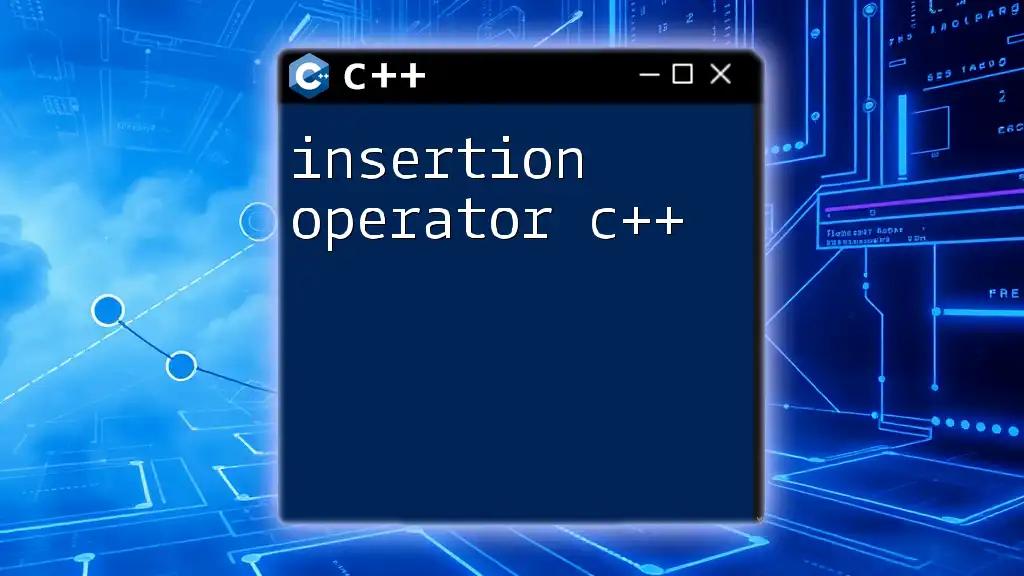
Conclusion
Recap of Key Points
Understanding the “and” and “or” operators is critical for effective programming in C++. They allow you to craft complex logical conditions that can dictate the execution flow of your program. This understanding helps you build clear, efficient, and functional applications.
Call to Action
Take what you’ve learned and start practicing these concepts in your C++ projects. Test various combinations of conditions to see how they influence the execution paths in your programs.
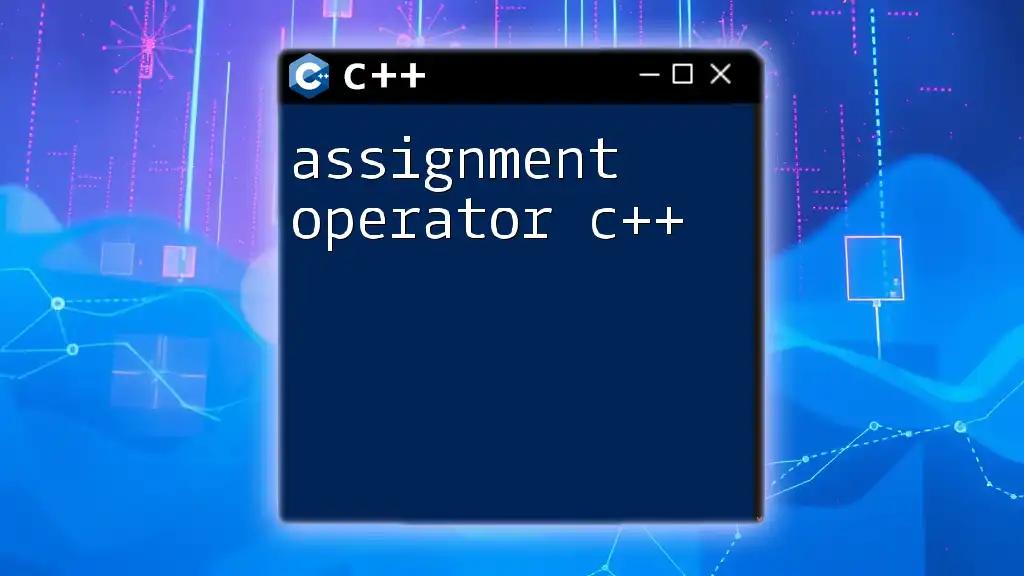
Additional Resources
Recommended Readings
For those keen on deepening their knowledge of logical operators and C++ programming, consider exploring online courses, books, or forums that specialize in coding and technical skills.
Community and Forums
Engage with community forums where you can ask questions, seek guidance, and share your newfound understanding of the and or operator c++. This interaction can significantly enhance your learning experience and help you connect with others in the programming community.