The insertion operator (`<<`) in C++ is used to output data to the standard output stream, such as `cout`, enabling you to display values and text in a concise manner.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Syntax of the Insertion Operator
The insertion operator in C++ is represented by the symbol `<<`. This operator is primarily associated with output operations in the C++ standard library, allowing you to display data on the console or write it to output streams.
Basic Syntax
The most common usage of the insertion operator is with `std::cout`, which is the standard output stream. The basic syntax is as follows:
std::cout << expression;
Here, `expression` is the data you want to output. This can include literals, variables, strings, and even more complex types.
How It Works
The insertion operator works by invoking a member function called `operator<<` from the `iostream` library. When you use `std::cout << expression`, the operator takes the provided expression, converts it into a format suitable for output, and sends the result to the standard output stream.
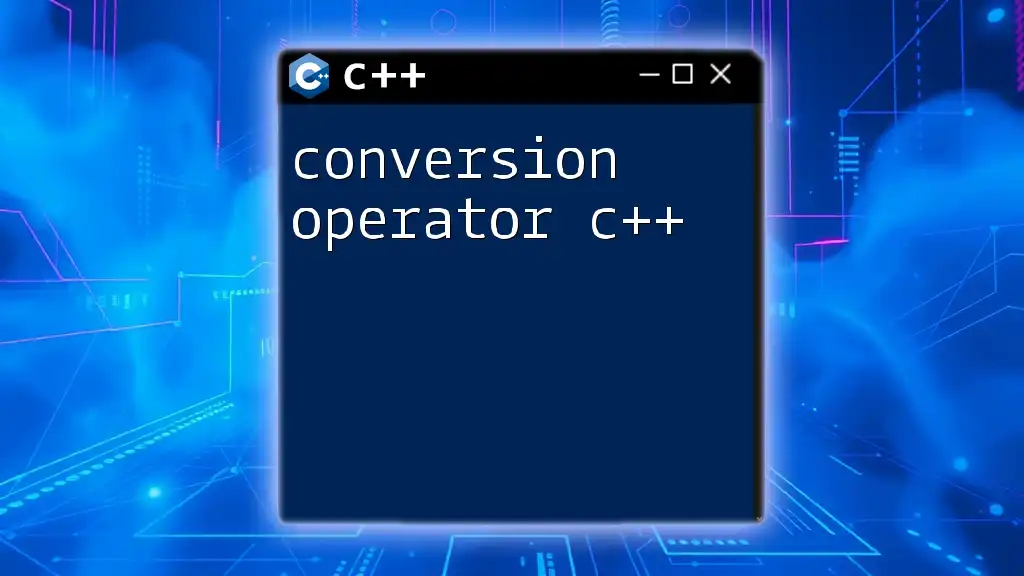
Using the Insertion Operator with Basic Data Types
The insertion operator can be used with various fundamental data types. Below are examples demonstrating how to output different types of data using this operator.
Integer Data Types
When dealing with integers, you can easily insert them into the output stream. The following code snippet illustrates how to use the insertion operator with an integer variable:
#include <iostream>
int main() {
int number = 42;
std::cout << "The answer is: " << number << std::endl; // Outputs: The answer is: 42
return 0;
}
Character Data Types
You can also use the insertion operator for characters. Here’s how that works:
#include <iostream>
int main() {
char letter = 'A';
std::cout << "The letter is: " << letter << std::endl; // Outputs: The letter is: A
return 0;
}
Floating-Point Data Types
The insertion operator is equally effective with floating-point numbers. Below is an example showcasing how to output float and double types:
#include <iostream>
int main() {
float pi = 3.14;
std::cout << "Value of Pi: " << pi << std::endl; // Outputs: Value of Pi: 3.14
return 0;
}
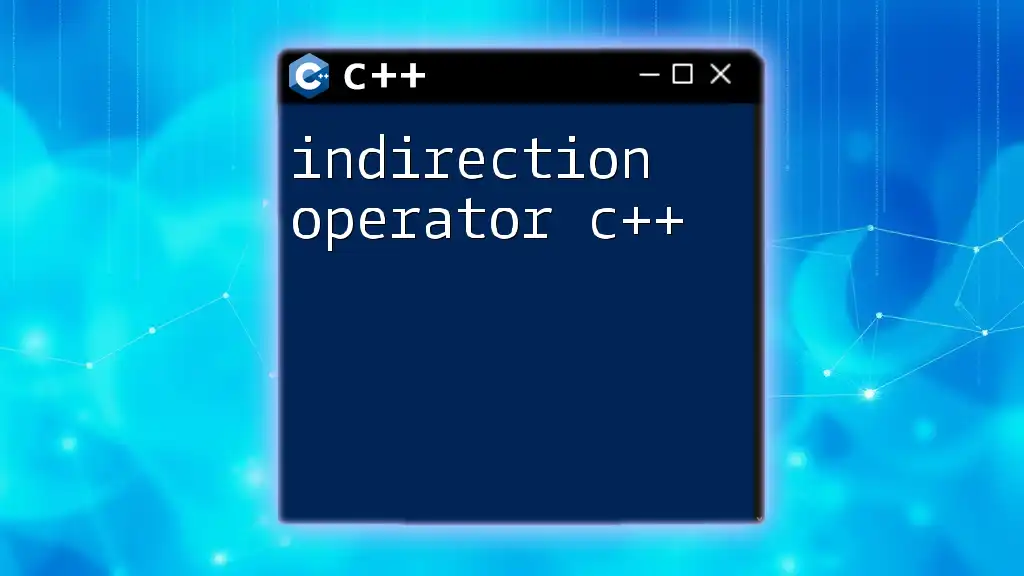
Using the Insertion Operator with Strings
String Handling
In C++, strings can be handled using `std::string` or C-style strings. The insertion operator seamlessly works with both types, allowing for intuitive output.
Code Snippet: Inserting Strings
Here’s a demonstration of how to use the insertion operator with strings:
#include <iostream>
#include <string>
int main() {
std::string name = "Alice";
std::cout << "Hello, " << name << "!" << std::endl; // Outputs: Hello, Alice!
return 0;
}
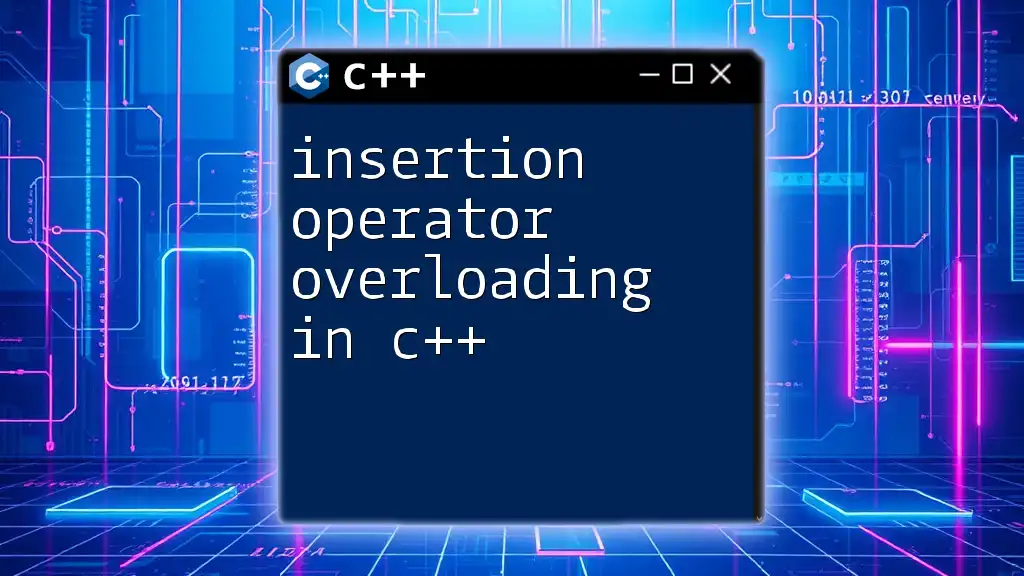
Overloading the Insertion Operator
What is Operator Overloading?
C++ allows you to define how operators behave for your custom classes through operator overloading. This enhances the expressiveness of your code, allowing you to use the same syntax for both built-in and user-defined types.
How to Overload the Insertion Operator
To overload the insertion operator for a custom class, you need to define a friend function. Here’s an example where we overload the insertion operator for a simple `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int xVal, int yVal) : x(xVal), y(yVal) {}
friend std::ostream& operator<<(std::ostream& os, const Point& p);
};
std::ostream& operator<<(std::ostream& os, const Point& p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
int main() {
Point p(1, 2);
std::cout << "Point coordinates: " << p << std::endl; // Outputs: Point coordinates: (1, 2)
return 0;
}
In this example, we define a friend function that allows the insertion operator to access the private members of the `Point` class. This way, we can print objects of the `Point` class just like built-in types.
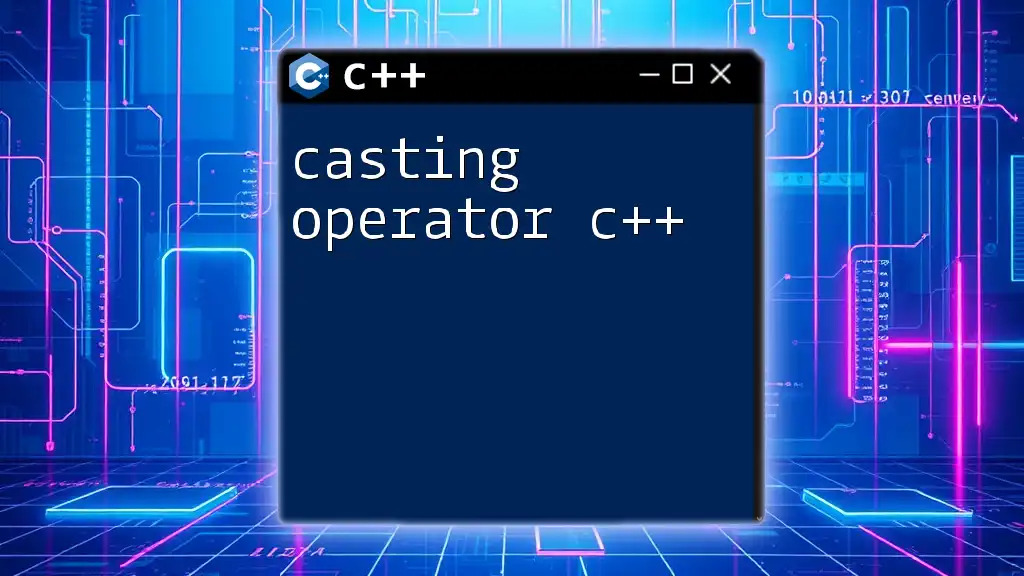
Comparison with Other Output Mechanisms
While the insertion operator is quite powerful, it is worthwhile to compare it with other output mechanisms in C++. The `printf` function, commonly used in C, is one such example.
Using `printf` vs. Insertion Operator
C++ developers often prefer the insertion operator because of its type safety and ease of use.
Here’s a comparison:
#include <cstdio>
#include <iostream>
int main() {
int x = 10;
std::cout << "Value using insertion operator: " << x << std::endl; // Safer due to type checking
printf("Value using printf: %d\n", x); // Needs format specifier
return 0;
}
The insertion operator is more flexible and easier to read, particularly when dealing with multiple types.
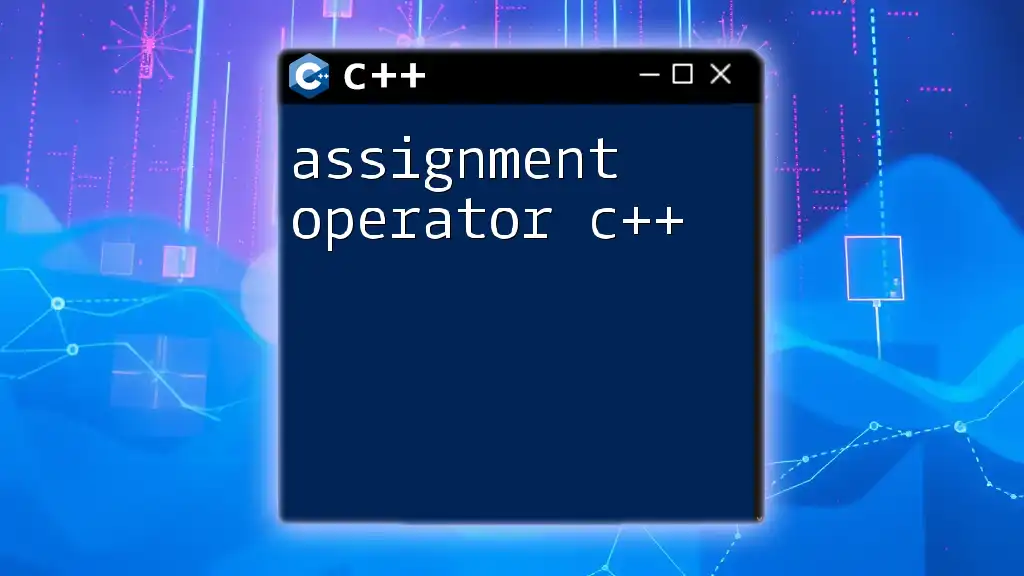
Common Pitfalls with the Insertion Operator
While using the insertion operator can be straightforward, there are common pitfalls to watch out for:
Not Including the Required Libraries
Make sure you always include the necessary header for using `std::cout`:
#include <iostream>
Not doing so will lead to compilation errors.
Confusion with the Stream State
If you encounter unexpected output, consider checking the state of the output stream. The stream can enter a fail state if there’s a problem during output operations, which will affect subsequent calls.
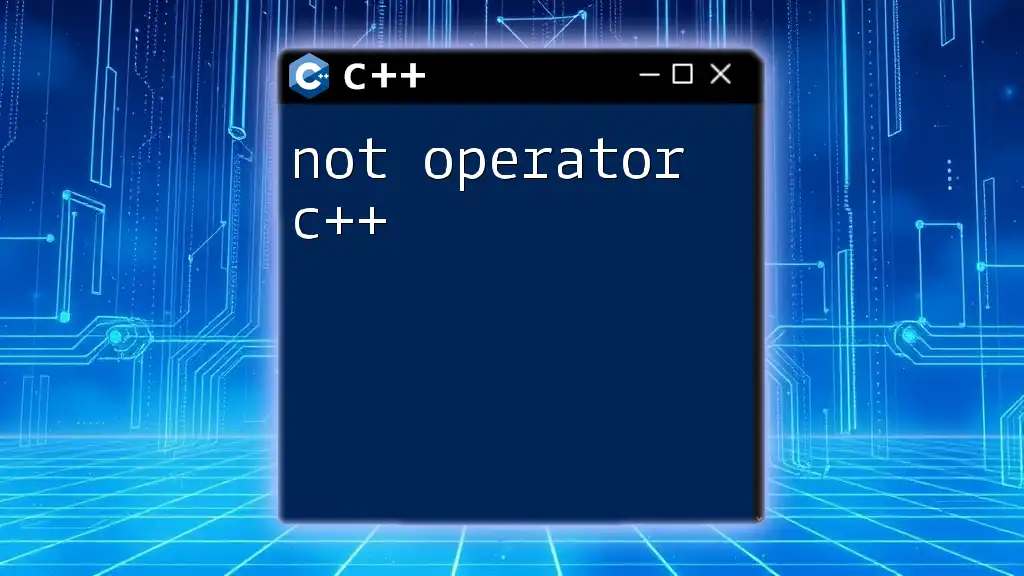
Best Practices for Using the Insertion Operator
Use of Chaining
One of the elegant features of the insertion operator is its ability to chain multiple statements together for a concise output format:
#include <iostream>
int main() {
int a = 1, b = 2, c = 3;
std::cout << "Values: " << a << ", " << b << ", " << c << std::endl; // Outputs: Values: 1, 2, 3
return 0;
}
Handling Different Data Types
When dealing with mixed types in a single output statement, ensure you approach output formatting with care to maintain clarity and avoid type mismatches.
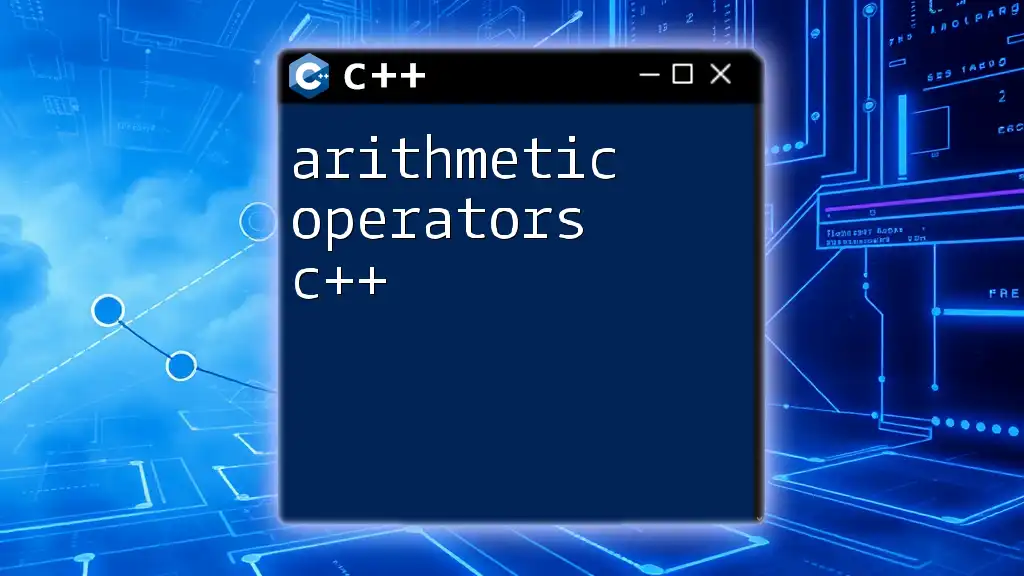
Conclusion
The insertion operator in C++ is a powerful tool for outputting data to the console. Through its simplicity and versatility, it enhances the coding experience in C++, making it easy to display various data types seamlessly. By mastering the insertion operator, you can improve your coding efficiency and readability in C++. Practice using it across different scenarios to truly appreciate its capabilities, and don’t hesitate to experiment with overloading it for your classes.