In C++, a generator is a function that uses the `co_yield` keyword to produce a sequence of values lazily, allowing for efficient iteration without storing all values in memory at once.
Here’s a simple example using a generator to produce a sequence of integers:
#include <iostream>
#include <coroutine>
#include <optional>
struct Generator {
struct promise_type {
Generator get_return_object() { return {}; }
std::suspend_always yield_value(int value) {
std::cout << "Yielding: " << value << std::endl;
return {};
}
void return_void() {}
void unhandled_exception() {}
};
// Other necessary members can be added here
};
Generator generate_numbers() {
for (int i = 1; i <= 5; ++i) {
co_yield i;
}
}
int main() {
auto gen = generate_numbers();
// Iterate through the generator (this needs a proper coroutine handling setup)
return 0;
}
Note: This code is a simplified representation, and setting up coroutine handling correctly requires additional implementation details not fully covered here.
What is a Generator in C++?
A generator in C++ is a special type of function that allows for the production of a sequence of values over time. Unlike traditional functions that compute results and return them all at once, generators enable lazy evaluation, yielding intermediate results as they are requested. This characteristic makes generators especially useful in scenarios where the complete dataset may be large or infinite.
Generators can produce sequences of numbers, strings, or any other data type, making them an essential tool for various applications, including simulations, data processing, and real-time data streaming.
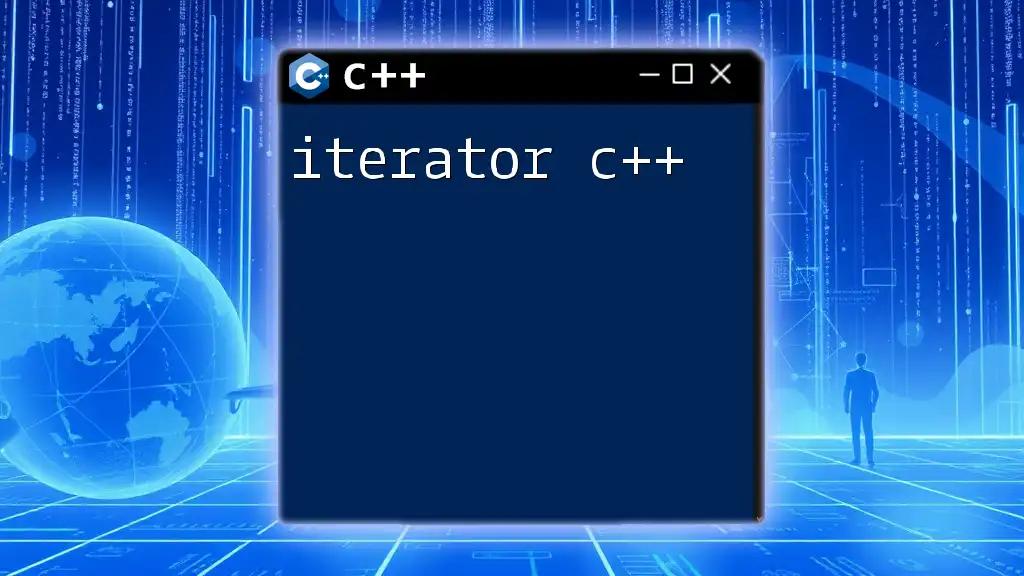
The Concept of Yielding Values
In the context of generators, yielding is the process by which a function returns a value temporarily, pausing execution to allow other tasks to take place. When you call a generator function, it does not execute the entire function immediately; instead, it runs until it hits a `yield` statement.
Benefits of using yielding over returning values include:
- Efficiency: Only necessary values are computed and returned, reducing memory usage.
- Control: More fine-grained control over the flow of the program, making complex algorithms easier to implement.
Example of a Simple Generator
Let’s look at a straightforward example of a generator that produces a series of numbers:
// Simple C++ generator example
#include <iostream>
#include <vector>
std::vector<int> generate_numbers(int count) {
std::vector<int> numbers;
for (int i = 0; i < count; ++i) {
numbers.push_back(i);
}
return numbers;
}
int main() {
auto nums = generate_numbers(5);
for (auto n : nums) {
std::cout << n << " ";
}
return 0;
}
In this example, the `generate_numbers` function generates a vector containing numbers from 0 to `count - 1`. The `main` function then prints these numbers. The output will be `0 1 2 3 4`, demonstrating how the generator function produces a sequence of values dynamically.

Implementing a Generator in C++
Creating a generator in C++ involves understanding a few key components. Primarily, you will define a function that yields values and maintains its state between calls.
Example: Fibonacci Sequence Generator
The following example demonstrates a Fibonacci sequence generator using a static variable to preserve state:
#include <iostream>
#include <utility>
std::pair<int, int> fibonacci() {
static int a = 0, b = 1; // maintains state across function calls
int next = a;
std::tie(a, b) = std::make_pair(b, a + b);
return {next, a};
}
int main() {
for (int i = 0; i < 10; ++i) {
auto [current, next] = fibonacci();
std::cout << current << " ";
}
return 0;
}
Here, the `fibonacci` function uses `static` variables `a` and `b` to keep track of the last two numbers of the Fibonacci series. Each time the function is called, it yields the next value in sequence until the desired number of calls is reached. The output of this code will be the first 10 numbers of the Fibonacci series: `0 1 1 2 3 5 8 13 21 34`.
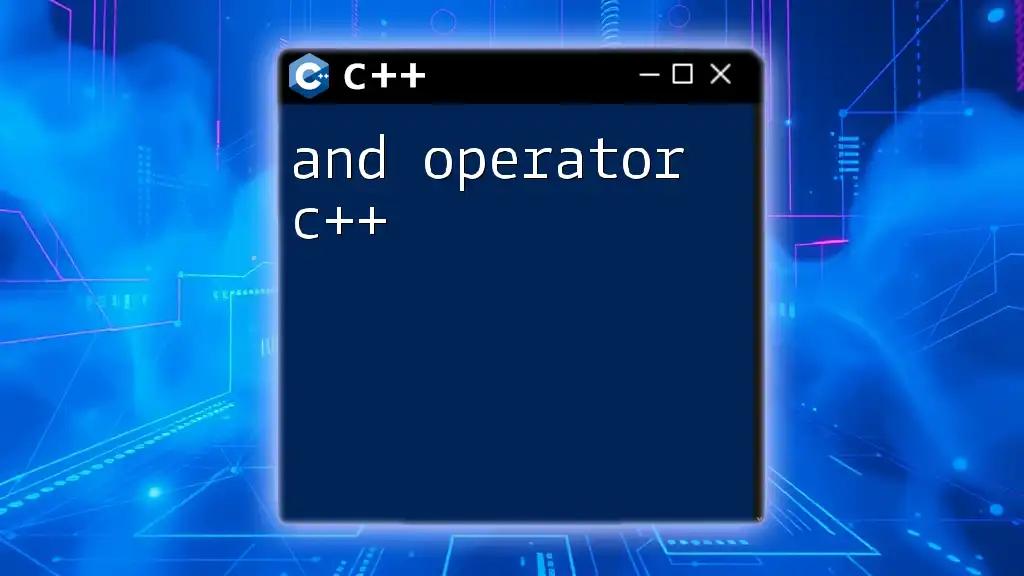
Advantages of Using Generators in C++
There are several advantages to using generators in your C++ programs:
-
Memory Efficiency: Generators compute values on-the-fly, reducing the need for large data structures and minimizing memory overhead.
-
Improved Code Readability: Generators help to simplify the logic of generating sequences, which can make your code cleaner and more understandable.
-
Lazy Evaluation: With generators, values are computed only when requested, ideal for handling large datasets or potentially infinite sequences without overwhelming system resources.
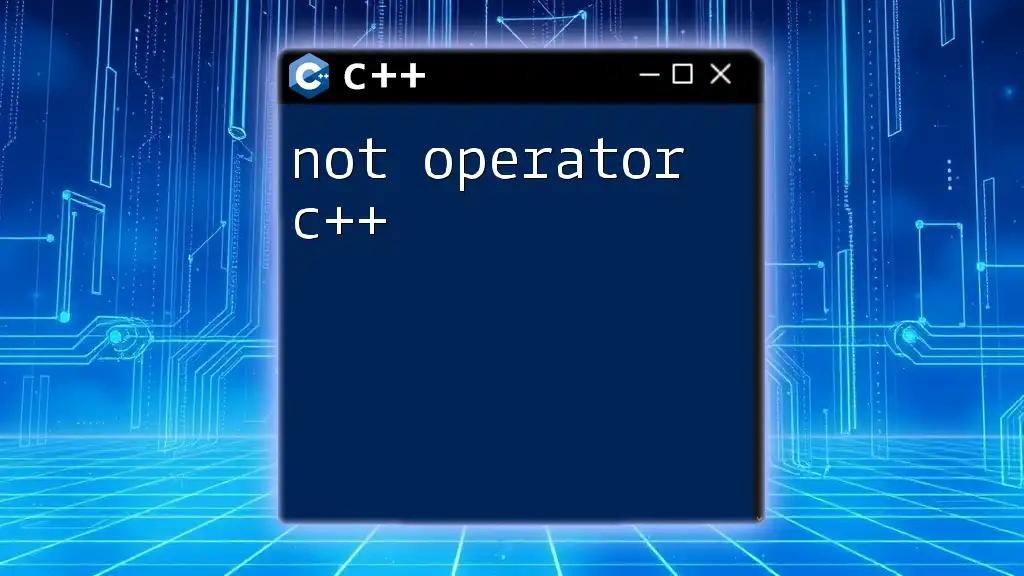
Common Patterns in Generators
C++ generators often employ various patterns to produce their values efficiently:
-
Iterative Patterns: Generators can be designed to produce a series of values in a loop.
-
Recursive Patterns: Sometimes, a recursive approach is fitting, especially for number series or tree structures.
-
Usage with Range-Based Loops: C++11 introduced range-based loops which work seamlessly with generators, enhancing the way we can iterate through generated sequences.
Example: Generator as a Range
Here’s a more advanced example of how a generator can be implemented to represent a range of numbers:
#include <iostream>
#include <vector>
class Range {
public:
class iterator {
int current;
public:
iterator(int start) : current(start) {}
int operator*() const { return current; }
const iterator& operator++() { ++current; return *this; }
bool operator!=(const iterator& other) const { return current != other.current; }
};
Range(int start, int end) : start(start), end(end) {}
iterator begin() { return iterator(start); }
iterator end() { return iterator(end); }
private:
int start, end;
};
int main() {
for (int i : Range(1, 10)) {
std::cout << i << " ";
}
return 0;
}
In the above example, the `Range` class creates an iterable range. The inner `iterator` class defines how to traverse through the numbers. When invoked in the `main` function, it produces the output: `1 2 3 4 5 6 7 8 9`, showing an elegant way to handle ranges using a generator-like pattern.
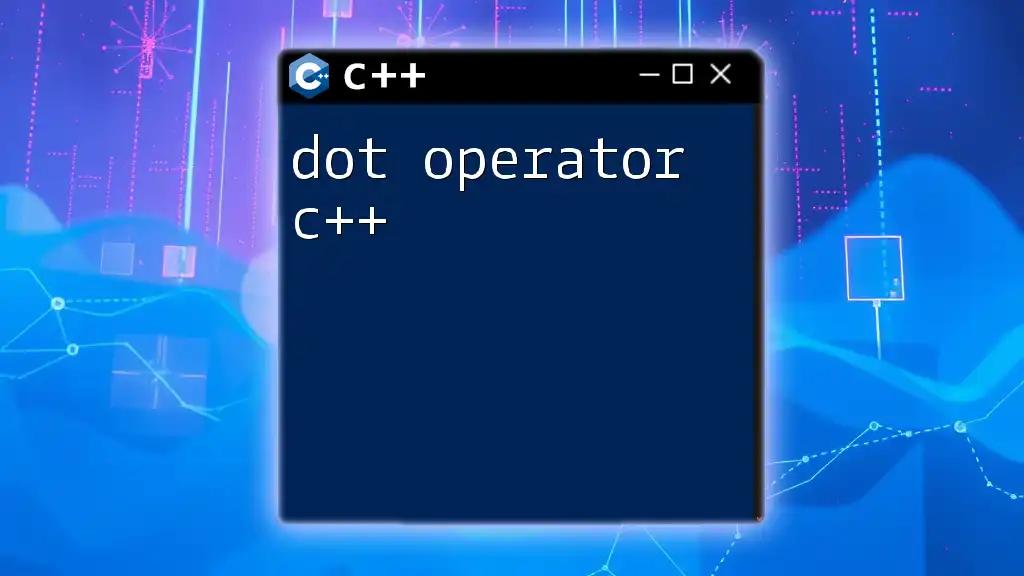
Best Practices for Using Generators
When implementing generators, consider the following best practices to maximize their effectiveness:
-
Keep Functions Pure: Ensure that your generator does not produce side effects to maintain a predictable and manageable codebase.
-
Handle Exceptions Gracefully: Implement exception handling within your generator functions to deal with exceptional cases without crashing the program.
-
Resource Management: Be aware of resource allocation and deallocation when using generators, especially in scenarios involving large data sets or file I/O.
-
Avoid Common Pitfalls: Watch out for shared mutable state across generator invocations, which can lead to unexpected behaviors and bugs.
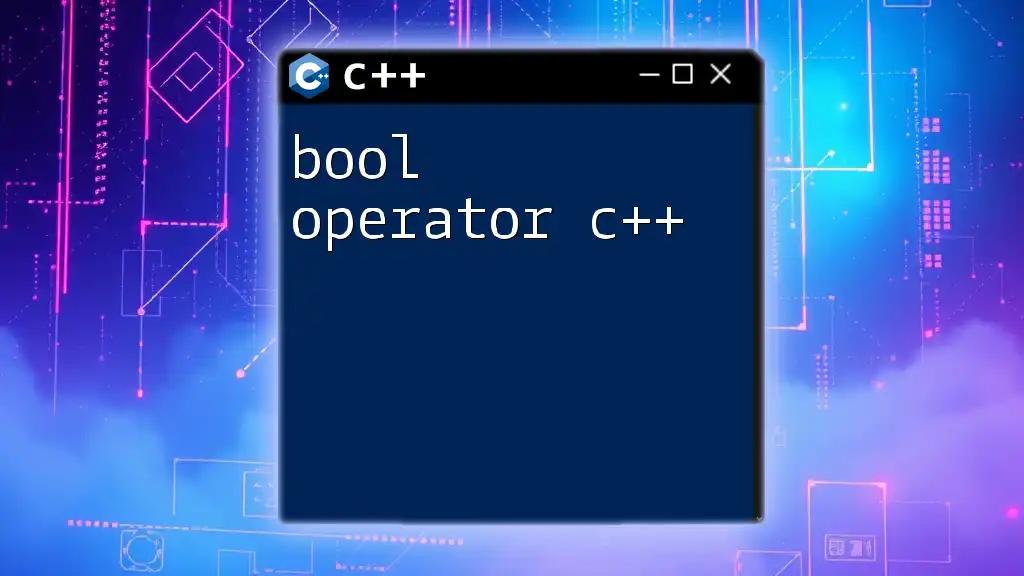
Real-World Applications of Generators
Generators in C++ have numerous applications, including:
-
Data Streaming and Processing: You can utilize generators to read and process data in chunks rather than loading it all into memory at once.
-
Infinite Sequences: Generators allow for the creation of infinite sequences, perfect for simulations or procedural generation.
-
Simulation and Modeling: In applications that require the generation of random numbers or simulated environments, generators can provide the necessary tools to produce sequences in real-time.
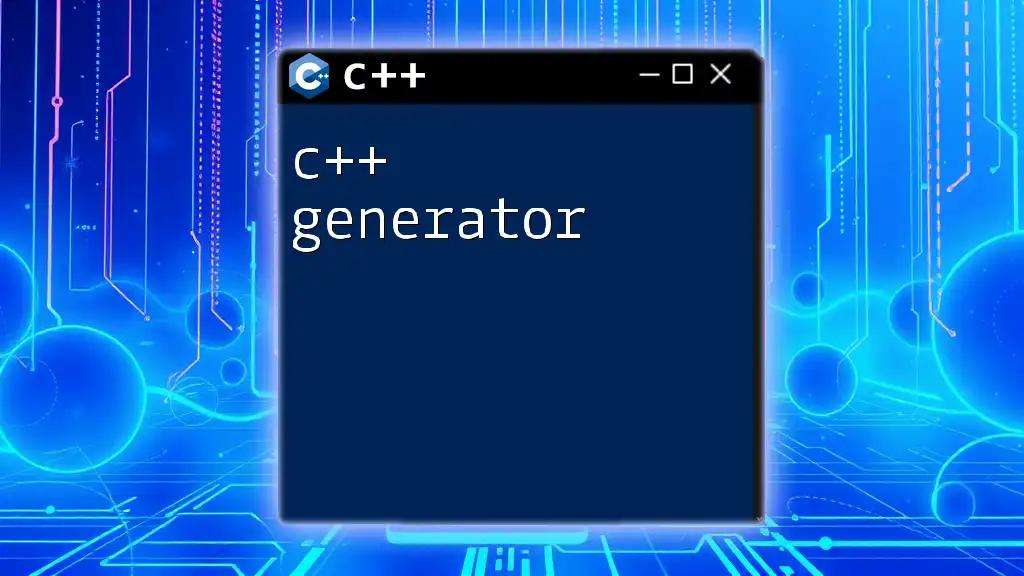
Conclusion
In summary, the generator C++ concept serves as a powerful programming tool, allowing developers to yield values dynamically while maintaining resource efficiency. Through generators, code becomes not only more readable but also capable of handling large and possibly infinite data sequences gracefully. As you explore the potential of generators in your projects, you may discover even more innovative applications.
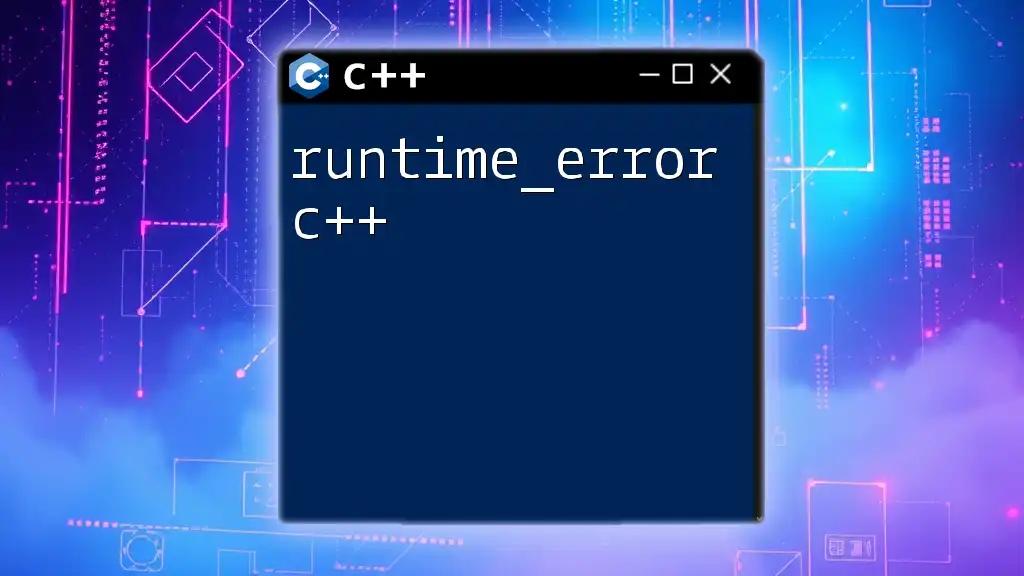
Additional Resources
For those looking to deepen their understanding of C++ generators, consider the following:
- Books on C++ programming and advanced coding techniques
- Online tutorials that focus on functional programming concepts in C++
- Engaging with community forums for discussion, support, and shared learning

Call to Action
Have you experimented with generators in your own C++ projects? Share your experiences, challenges, and successes in the comments below! And be sure to subscribe to our blog for more insights into concise C++ programming techniques.