The dot operator (.) in C++ is used to access members (attributes and methods) of a class or structure from an object.
#include <iostream>
class Point {
public:
int x, y;
void display() {
std::cout << "Point(" << x << ", " << y << ")" << std::endl;
}
};
int main() {
Point p;
p.x = 10; // Using the dot operator to set the x attribute
p.y = 20; // Using the dot operator to set the y attribute
p.display(); // Using the dot operator to call the display method
return 0;
}
Understanding the Basics of the Dot Operator
What is the Dot Operator?
The dot operator in C++ is a fundamental concept used primarily to access members of a class—such as data members (attributes) and member functions (methods). It allows you to interact with objects created from classes, seamlessly connecting you to the underlying properties and behaviors defined within those classes. The syntax for the dot operator is quite straightforward: you simply write `objectName.memberName`.
For example, if you have an object named `car` of type `Car`, you would access a member named `brand` with the syntax `car.brand`.
When to Use the Dot Operator
The dot operator is typically used in object-oriented programming when you want to:
- Access instance members of a class from an object.
- Call member functions on class instances.
- Navigate through nested objects, leveraging member access to illustrate complex relationships.
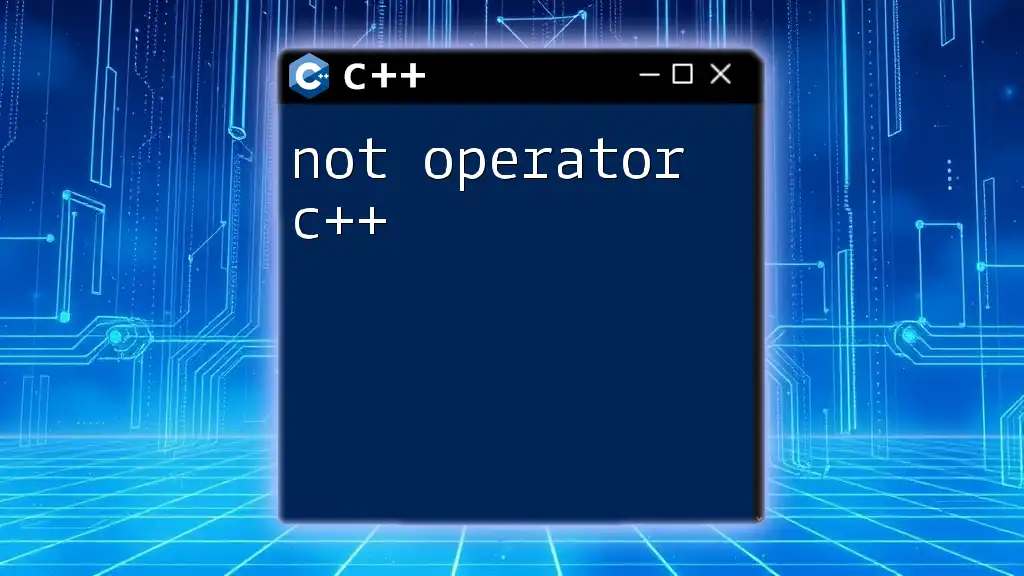
How to Use the Dot Operator
Accessing Members of a Class
To demonstrate the usage of the dot operator, let’s define a simple class, `Car`, which has both an attribute and a member function:
class Car {
public:
string brand; // Attribute
void honk() { // Member function
cout << "Honk! Honk!" << endl;
}
};
int main() {
Car myCar; // Creating an object of Car
myCar.brand = "Toyota"; // Using the dot operator to access attribute
myCar.honk(); // Using the dot operator to call member function
return 0;
}
In this code snippet, we have a class named `Car` with a public attribute called `brand` and a public method named `honk()`. In the `main()` function, we create an object `myCar` of type `Car` and use the dot operator to set the brand and call the honk function, clearly demonstrating how it provides access to an object's members.
Invalid Access Attempts
Just as using the dot operator grants you access to members, trying to access a non-existent member will lead to compilation errors. Consider the following example:
class House {
public:
int rooms; // An attribute representing the number of rooms
};
int main() {
House myHouse;
// myHouse.garage = 1; // This line would cause a compilation error
return 0;
}
In this case, attempting to access `myHouse.garage`, which is not a defined attribute of the `House` class, results in a compilation error. This highlights the importance of knowing the structure of your classes when using the dot operator.
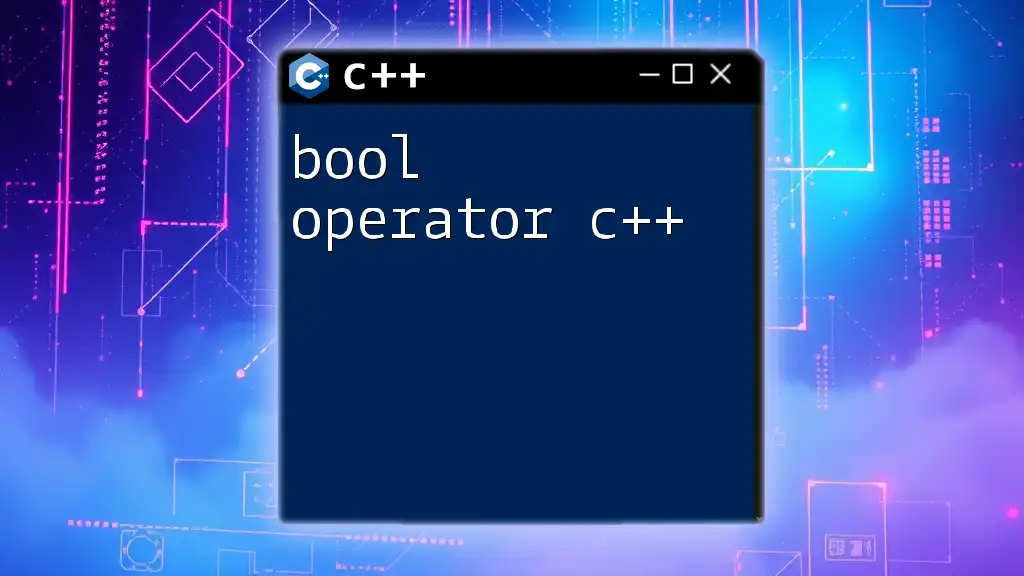
The Dot Operator with Pointers
Using the Dot Operator with Object Pointers
In C++, pointers serve as a powerful way to reference objects. However, when accessing members of an object through a pointer, you must differentiate between using the dot operator and the arrow operator (`->`). The arrow operator is specifically designed for accessing members via pointers.
Here’s an example showcasing the correct use of the arrow operator:
class Person {
public:
string name; // Attribute
};
int main() {
Person* personPtr = new Person(); // Creating a pointer to a Person object
personPtr->name = "John Doe"; // Using the arrow operator
delete personPtr; // Always remember to cleanup dynamically allocated memory
return 0;
}
In this scenario, we dynamically allocate a `Person` object. The use of `personPtr->name` illustrates that when accessing members through pointers, the arrow operator is the correct choice.
Accessing Members with Pointers and the Dot Operator
However, if you have a regular object and you want to utilize a pointer to access its members, you first need to dereference the pointer. Here's how it works:
Person person; // Creating a regular object
person.name = "Alice"; // Setting the name
Person* personPtr = &person; // Create a pointer to the object
cout << personPtr->name; // Outputting the name using the arrow operator
In this example, we declare an object `person` and assign a value to its `name`. We then create a pointer that points to `person` and access the `name` using the appropriate arrow operator, demonstrating how to work seamlessly with both regular objects and pointers.
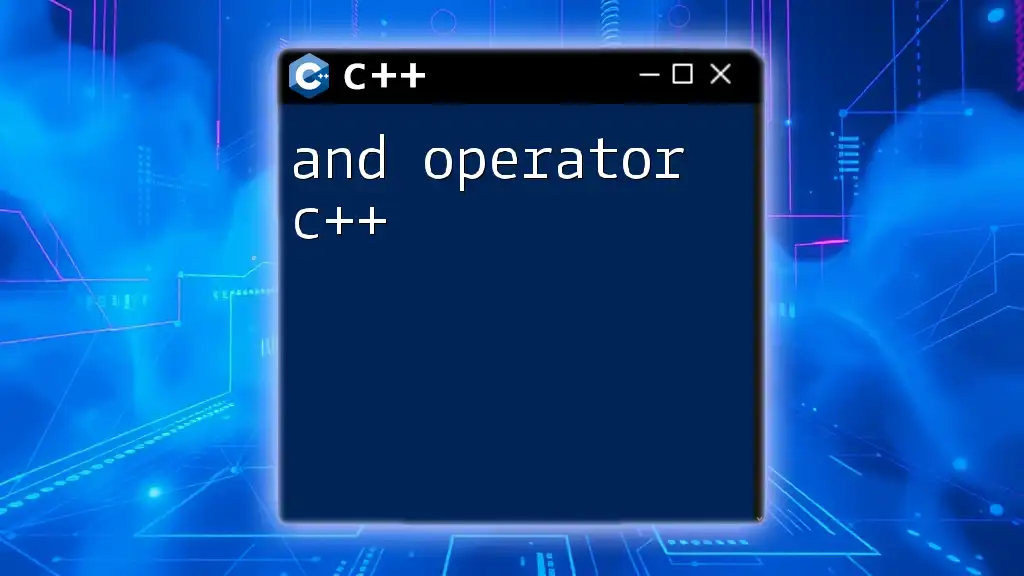
Advanced Usage of the Dot Operator
Chaining Member Access
Another advanced feature of the dot operator is member access chaining. This comes into play when classes contain members that are themselves objects of other classes. For example:
class Engine {
public:
void start() {
cout << "Engine started" << endl; // Simple method to start the engine
}
};
class Car {
public:
Engine engine; // Engine is an object of class Engine
};
int main() {
Car myCar; // Creating a Car object
myCar.engine.start(); // Chaining the dot operator to call start() on the engine
return 0;
}
Here, we see how `myCar.engine.start()` effectively uses the dot operator to access and execute the `start()` method from the embedded `Engine` object. This capability is crucial in working with more intricate structures in C++.
Using the Dot Operator with Inheritance
Inheritance is a core concept in object-oriented programming that allows classes to derive properties and methods from other classes. The dot operator continues to play its vital role here as well. Here's how it works:
class Vehicle {
public:
void drive() {
cout << "Driving the vehicle" << endl; // Base class method
}
};
class Bike : public Vehicle {}; // Inheriting Vehicle class
int main() {
Bike myBike; // Creating a Bike object
myBike.drive(); // Accessing the base class member via the dot operator
return 0;
}
In the example above, the `Bike` class inherits from the `Vehicle` class. We seamlessly access the `drive()` method of the base class using the dot operator with a `Bike` object. This illustrates the dot operator's versatility across inheritance.
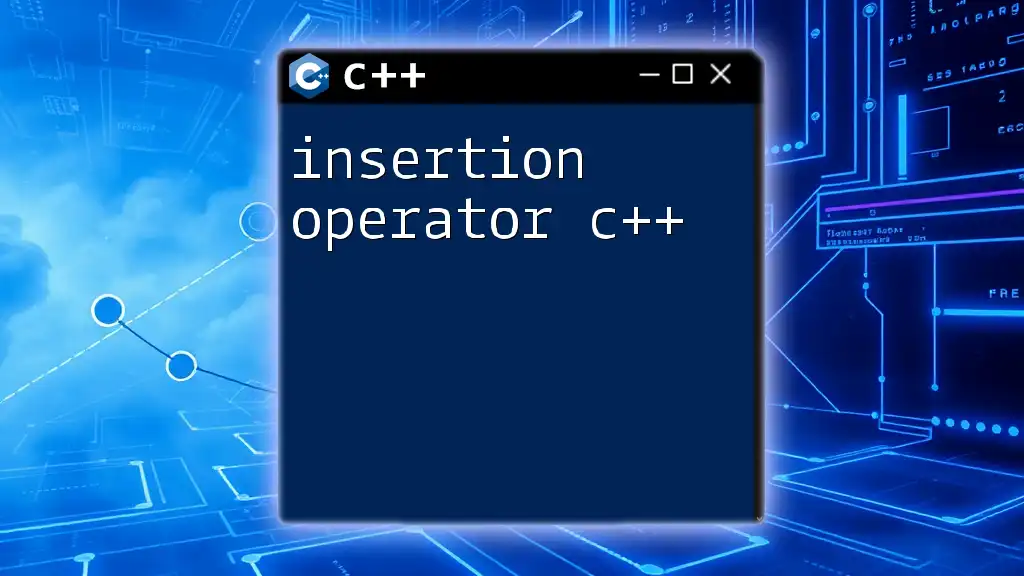
Common Pitfalls with the Dot Operator
Misunderstanding Scope with the Dot Operator
One common mistake programmers make is misunderstanding the scope when accessing members with the dot operator. Improperly using the operator can lead to unintended behavior or compilation errors as seen in the following example:
class BankAccount {
public:
int balance; // Attribute for storing balance
};
int main() {
BankAccount account;
// balance = 100; // Error if not using account.balance
account.balance = 100; // Correct way
}
In this case, the line `balance = 100;` is incorrect because it lacks the scope resolution that associates `balance` with the `account` object. Proper access utilizes the dot operator to avoid confusion.
Accessing Private Members
C++ encourages the use of encapsulation via access specifiers: `public`, `private`, and `protected`. When a member of a class is declared private, it can only be accessed through member functions. Consider this example:
class BankAccount {
private:
int balance;
public:
void setBalance(int b) {
balance = b; // Public function to access private member
}
int getBalance() {
return balance; // Public function to access private member
}
};
int main() {
BankAccount account;
account.setBalance(100); // Accessing private member through a public method
cout << account.getBalance(); // Outputs: 100
return 0;
}
In this scenario, the `balance` attribute is private, which means it cannot be directly accessed using the dot operator outside the class. Instead, we use public methods to modify and retrieve the balance safely.
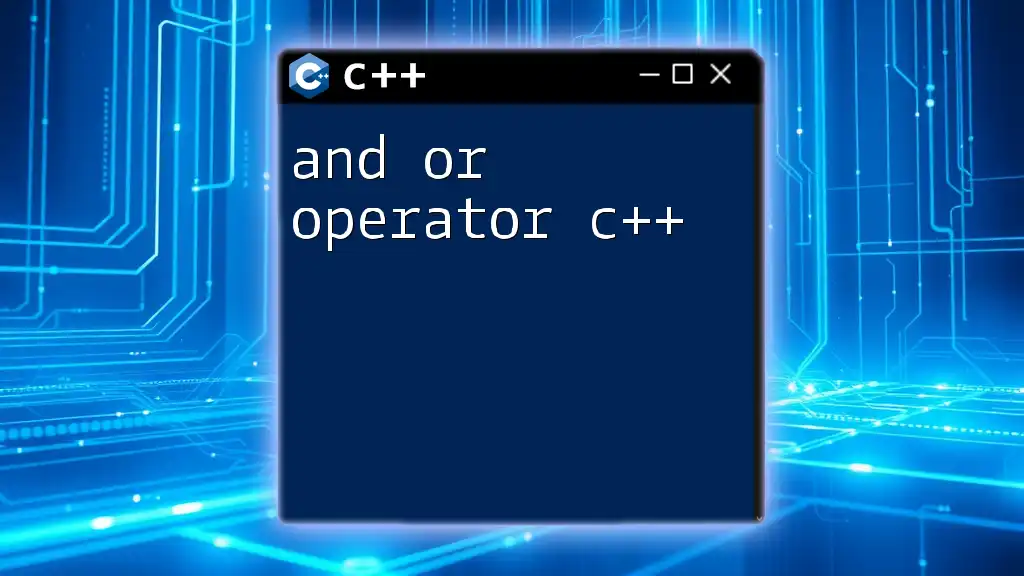
Conclusion
The dot operator in C++ serves as an essential tool for object manipulation, enabling access to class members effectively. By understanding its applications—from accessing simple members to manipulating complex structures with pointers and inheritance—you can leverage the power of C++ object-oriented programming to create robust applications. It is crucial to practice these concepts, challenge yourself with examples, and approach common pitfalls these lessons unveil. Through mastery of the dot operator, you will lay the groundwork for advanced programming techniques within C++.
For those looking to deepen their knowledge further, consider exploring additional resources and practical coding exercises that elaborate on the intricacies of C++ and its member access strategies.