A custom comparator in C++ allows developers to define their own comparison logic for sorting or ordering elements, typically implemented using a function or a functor.
Here's a simple example using a custom comparator with `std::sort`:
#include <iostream>
#include <vector>
#include <algorithm>
bool customComparator(int a, int b) {
return a > b; // Sorts in descending order
}
int main() {
std::vector<int> vec = {5, 3, 8, 1, 2};
std::sort(vec.begin(), vec.end(), customComparator); // Using custom comparator
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
What is a Comparator?
A comparator is a fundamental concept in programming that allows for the comparison of two elements. In C++, comparators are pivotal in determining how elements are ordered within data structures. Comparators are especially used in sorting operations, where they dictate the sequence in which elements appear after the sort.
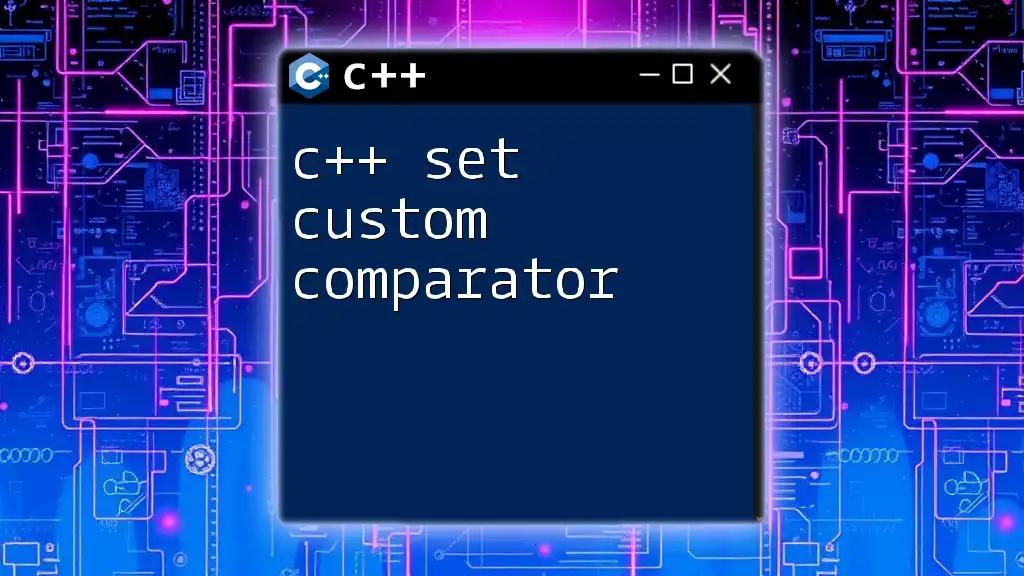
Importance of Custom Comparators
While C++ provides built-in comparison operators (like `<`, `>`, `<=`, `>=`), there are numerous situations where the default comparison mechanism may not suit specific requirements. For instance, when working with custom objects or when needing specialized sorting criteria, a custom comparator can be defined.

Understanding C++ Comparators
Default Comparators in C++
C++ facilitates the use of default comparators, which work seamlessly with standard data structures such as arrays, vectors, and sets. These comparators are typically defined using the built-in operators. For example, sorting a list of integers in ascending order can be achieved easily without any additional specifications.
Syntax of Comparators
In C++, comparators can be expressed in multiple ways. The most common methods include:
- Function pointers: These are standard functions passed as arguments to sorting functions.
- Functors: These are classes that implement the `operator()` to compare objects.
- Lambda functions: These are anonymous functions that can define custom comparators inline, offering a succinct and clear approach.

Creating Custom Comparators
Using Function Pointers
A function pointer comparator allows us to define a standalone function that specifies sorting logic.
Here's how you can define a basic function pointer comparator:
#include <iostream>
#include <algorithm>
bool compareDescending(int a, int b) {
return a > b;
}
int main() {
int arr[] = {5, 3, 6, 2};
std::sort(arr, arr + 4, compareDescending);
std::cout << "Sorted array in descending order: ";
for (int num : arr) {
std::cout << num << " ";
}
std::cout << std::endl;
}
In the example above, the `compareDescending` function specifies the logic for sorting integers in descending order.
Utilizing Functors
A functor is an object that can be called as if it were a function. Functors are particularly useful for defining complex sorting logic.
In this example, we will sort a vector of custom objects:
#include <iostream>
#include <vector>
#include <algorithm>
struct Person {
std::string name;
int age;
};
struct CompareByAge {
bool operator()(const Person &a, const Person &b) {
return a.age < b.age;
}
};
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}};
std::sort(people.begin(), people.end(), CompareByAge());
std::cout << "Sorted persons by age:\n";
for (const auto &person : people) {
std::cout << person.name << " - " << person.age << std::endl;
}
}
In this example, we define a `CompareByAge` functor that compares `Person` objects based on age, enabling sorting of the vector according to this criterion.
Leveraging Lambda Functions
With the advent of C++11, lambda functions provide a concise way to create custom comparators without extra overhead.
Here's how to use a lambda function for sorting:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {4, 1, 3, 9};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) {
return a < b; // Ascending order
});
std::cout << "Sorted numbers in ascending order:\n";
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
}
The lambda function in the `std::sort()` call allows for inline definition of the sorting criteria, making the code both efficient and easily readable.
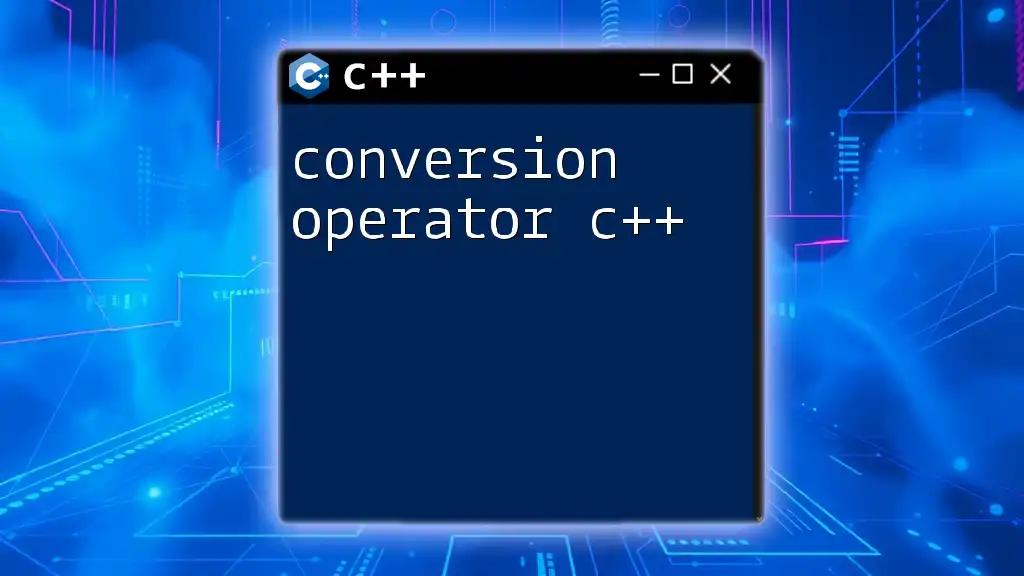
Advanced Custom Comparators
Multi-Criteria Comparators
Sometimes, sorting requires multiple keys. Utilizing multi-criteria comparators involves establishing priorities for each criterion.
Here's an example of a multi-comparator:
struct Employee {
std::string name;
int age;
double salary;
};
struct MultiComparator {
bool operator()(const Employee &a, const Employee &b) {
if (a.salary != b.salary)
return a.salary < b.salary; // Primary: by salary
return a.age < b.age; // Secondary: by age
}
};
In this case, employees are sorted primarily by their salary and secondarily by their age if salaries match, demonstrating the flexibility provided by custom comparators.
Standard Library Support for Custom Comparators
C++’s Standard Template Library (STL) is equipped with several sorting algorithms that accept custom comparators. Functions like `std::sort()` and containers such as `std::set` can be tailored to employ your custom comparison logic, enabling you to harness the power of your defined criteria throughout your data structures.
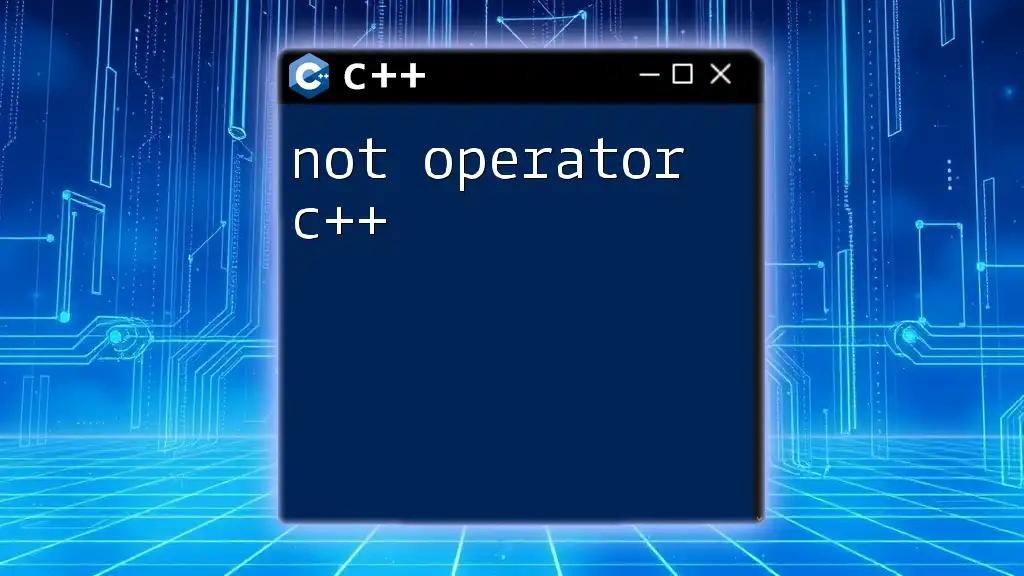
Best Practices for Writing Comparators
When crafting custom comparators, consider the following best practices:
-
Simplicity and Clarity: Strive to maintain simplicity. A comparator should be easy to read and follow. Avoid overly complex logic that can confuse users or future maintainers of your code.
-
Efficiency Considerations: Assess the performance implications of your comparator, especially for larger datasets. Using efficient comparison logic can significantly enhance the sorting process's overall speed, while poorly designed comparators can hinder performance.
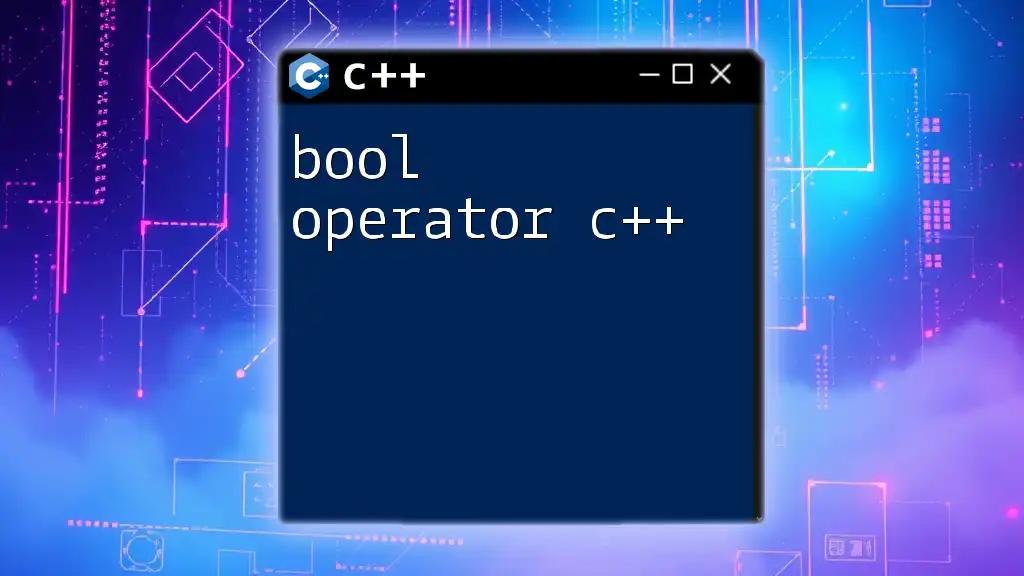
Common Errors and Pitfalls
When implementing custom comparators, it is critical to avoid certain common mistakes that can lead to undefined behavior, such as:
-
Improper Logic: Ensure that your comparator adheres to the strict weak ordering principle, which implies consistency and transitivity.
-
Incorrect Use with STL Algorithms: Ensure that your comparator functions are compatible with the algorithms you employ. For instance, using a comparator that does not properly handle equal elements can lead to incorrect sorting results.
Debugging Tips
To debug issues effectively:
- Use print statements or logging within your comparators to trace the comparison logic.
- Analyze the results of sorted data to identify unexpected behaviors that can point to logical errors in your comparator implementation.
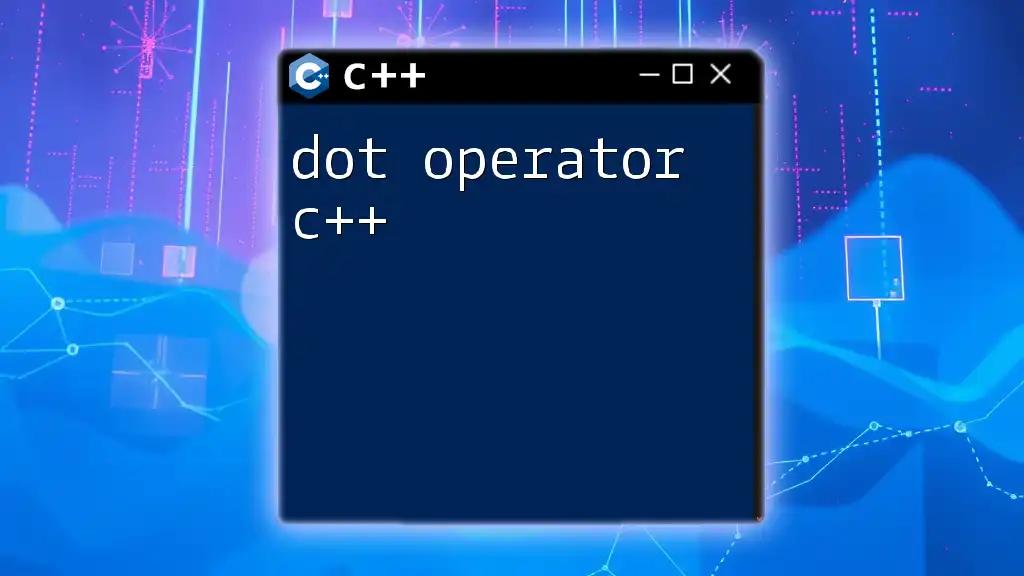
Recap of Key Points
In conclusion, understanding and implementing custom comparators in C++ can greatly enhance your programming capabilities. By defining specific sorting criteria through function pointers, functors, or lambda functions, you can fine-tune how your data is organized.
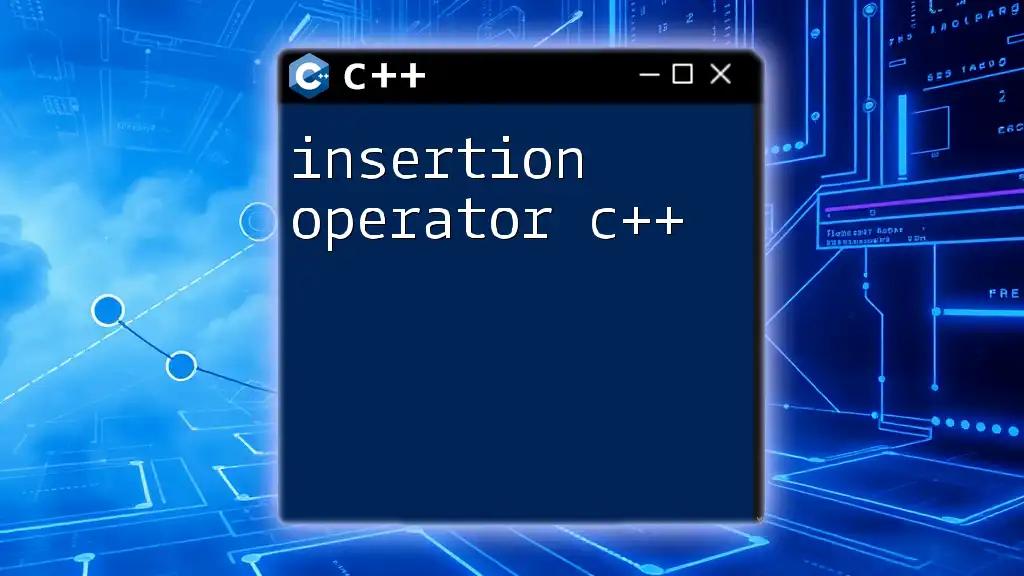
Encouragement to Experiment
I encourage you to experiment with creating your own custom comparators based on different data types and sorting needs. This will deepen your understanding and enhance your C++ programming skill set.
Engage with your community through comments or forums to share experiences and examples of custom comparators in different contexts. Happy coding!