In C++, a custom comparator can be defined to sort items in a specific order using the `std::sort` function by passing a user-defined function or lambda as the comparator parameter.
#include <algorithm>
#include <iostream>
#include <vector>
bool customComparator(int a, int b) {
return a > b; // Sorts in descending order
}
int main() {
std::vector<int> nums = {5, 2, 8, 1, 3};
std::sort(nums.begin(), nums.end(), customComparator);
for (int num : nums) {
std::cout << num << " ";
}
return 0;
}
What is a Custom Comparator?
A custom comparator in C++ is a function that defines a specific way to compare two elements for the purpose of sorting. While the default behavior uses the `operator<` to determine the order of elements, custom comparators allow you to customize how this comparison is carried out, thereby altering the sorting logic according to your needs.
Types of Comparators
-
Binary Comparators: These comparators take two arguments and return a boolean value. They define the logical comparison between two values. For example, a comparator that sorts integers in descending order.
-
Custom Comparators: More complex comparators can be used for specialized sorting needs, such as sorting data structures or using multiple fields for comparison.
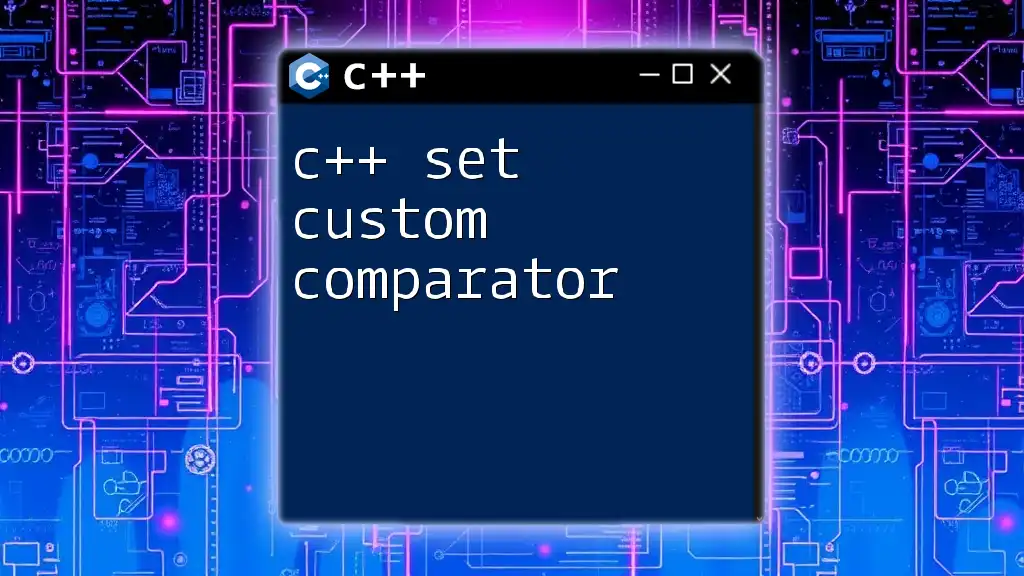
Implementing Custom Comparators in C++
C++ provides the `std::sort` function from the `<algorithm>` header for sorting collections of items. To utilize custom comparators, you simply pass them as an additional argument to this function.
Basic Example of Custom Comparator
Here’s a foundational example showing how to sort integers in descending order using a custom comparator:
#include <iostream>
#include <vector>
#include <algorithm>
bool customComparator(int a, int b) {
return a > b;
}
int main() {
std::vector<int> numbers = {5, 2, 8, 1, 3};
std::sort(numbers.begin(), numbers.end(), customComparator);
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this code snippet, the function `customComparator` defines that `a` should come before `b` if `a` is greater than `b`. Consequently, when `std::sort` is called, it sorts the `numbers` vector in descending order. This highlights how you can customize the sorting behavior beyond the standard ascending order.
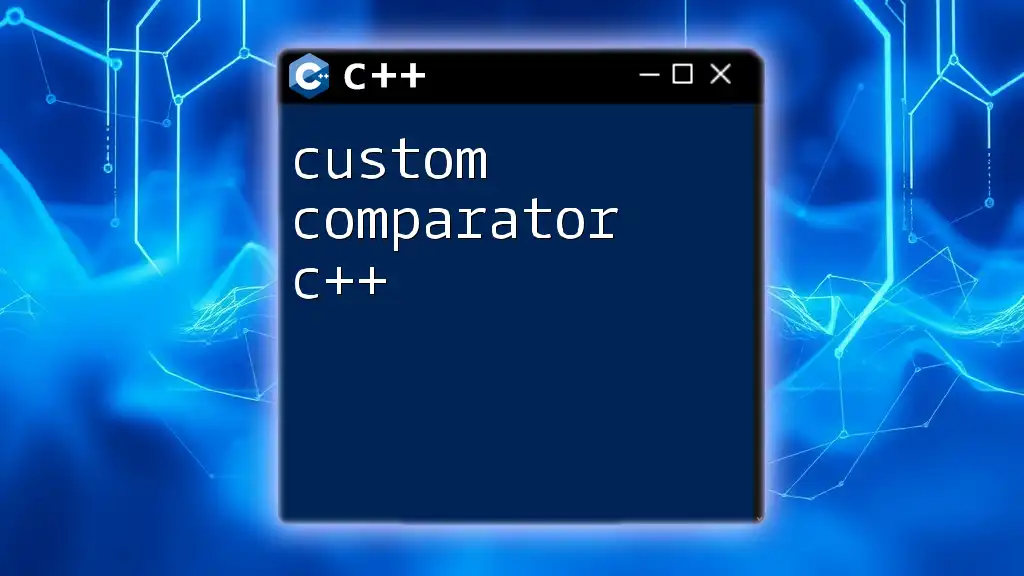
Creating a Custom Comparator with Structures and Classes
When you're dealing with custom data types, such as structs or classes, creating comparators becomes essential.
Example: Sorting a Vector of Structs
Consider this example where we sort a list of students by their grades. We can achieve this by defining a custom comparator function:
#include <iostream>
#include <vector>
#include <algorithm>
struct Student {
std::string name;
double grade;
};
bool compareByGrade(const Student &a, const Student &b) {
return a.grade < b.grade; // Sorts by ascending grade
}
int main() {
std::vector<Student> students = {
{"John", 90.0}, {"Jane", 95.5}, {"Doe", 85.5}
};
std::sort(students.begin(), students.end(), compareByGrade);
for (const auto &student : students) {
std::cout << student.name << ": " << student.grade << "\n";
}
return 0;
}
The `compareByGrade` function compares the `grade` fields of two `Student` objects. Sorting the `students` vector with this comparator organizes the students in ascending order based on their grades. This demonstrates how custom comparators can effectively manage sorting operations involving complex data types.
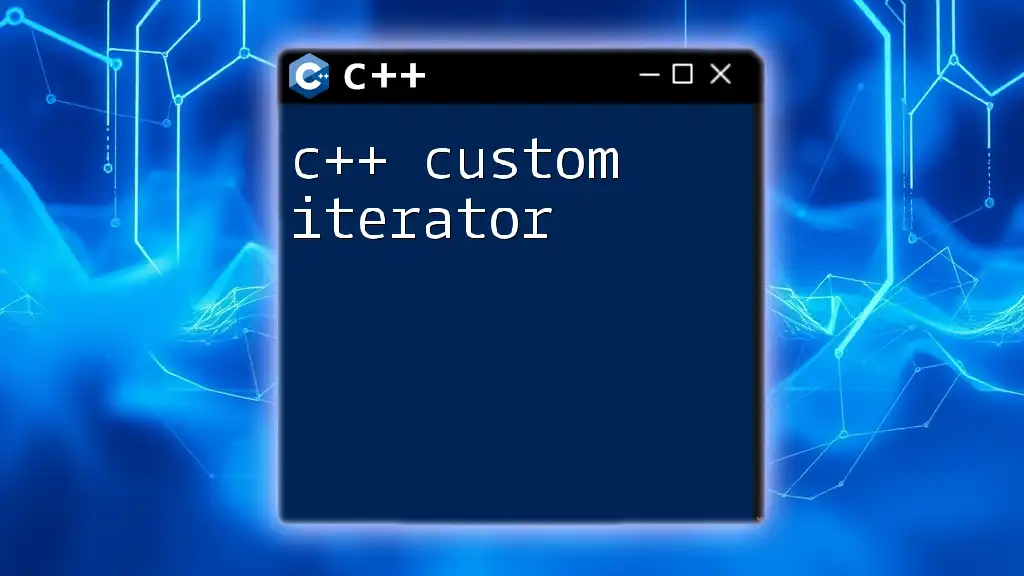
Lambda Expressions as Custom Comparators
In modern C++, you can streamline the process of writing custom comparators using lambda expressions. These allow you to define comparators inline, making your code cleaner and more concise.
Example: Using Lambda Functions for Inline Comparators
Here’s how you can use a lambda expression to sort a list of strings by their length:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<std::string> names = {"Alice", "Bob", "Christina", "Dan"};
std::sort(names.begin(), names.end(), [](const std::string &a, const std::string &b) {
return a.length() < b.length(); // Sort by length
});
for (const auto &name : names) {
std::cout << name << " ";
}
return 0;
}
In the code above, the inline lambda function sorts the `names` vector based on the length of each string. Using a lambda expression allows for quick and readable custom comparators when the logic is simple or used only once.
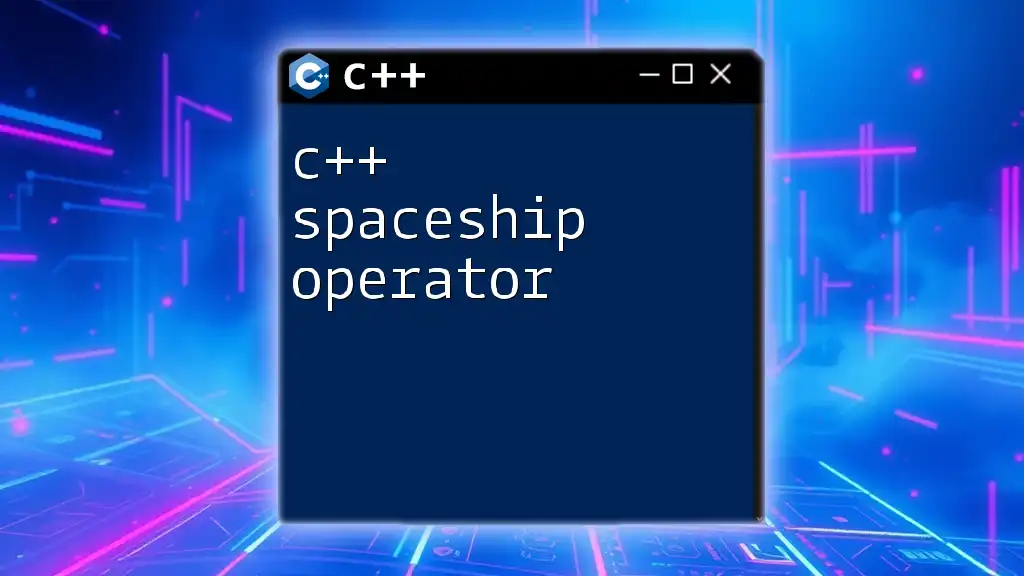
Best Practices for Writing Custom Comparators
When creating custom comparators, adhering to best practices is essential for ensuring clarity, maintainability, and performance.
-
Keep Comparators Simple: Strive for clarity in your comparator logic. A straightforward comparator is easier to read, maintain, and debug.
-
Consistency in Comparisons: Ensure that your comparator adheres to the principles of strict weak ordering. This means that for any two elements `a` and `b`, if `compare(a, b)` returns `true`, it should also hold that `compare(b, a)` returns `false`. It's crucial to maintain consistency across comparisons, as incorrect implementations can lead to undefined behavior in sorting.
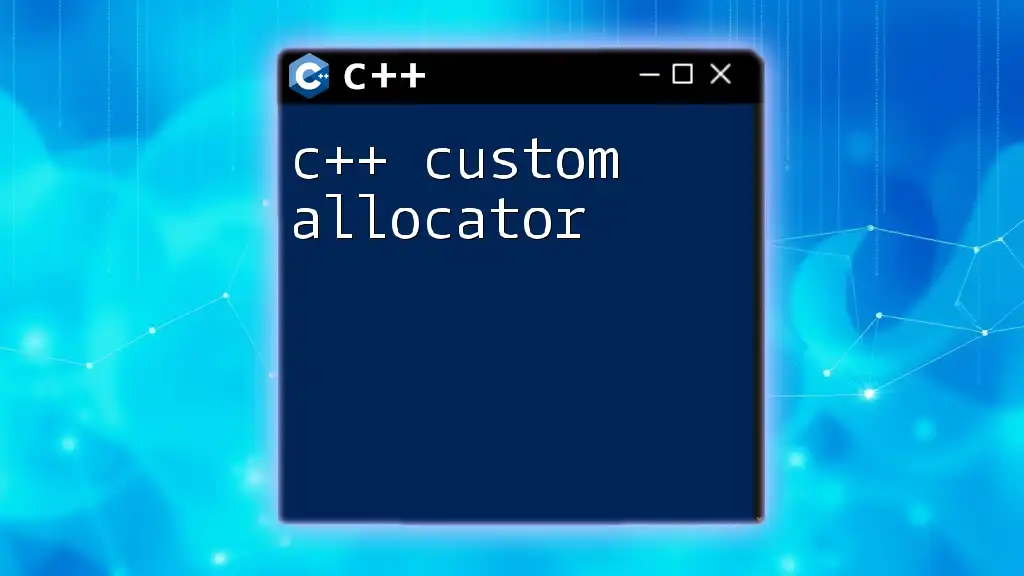
Common Pitfalls When Using Custom Comparators
While using custom comparators can enhance your sorting capabilities, there are some potential pitfalls to avoid:
-
Overcomplicating the Logic: Avoid creating overly complex comparators that can confuse readers or future maintainers of the code. Simplicity is key.
-
Forgetting to Return Bool: Ensure your comparator always returns a boolean value. Failing to do so can result in erratic sorting behavior, as `std::sort` depends on the comparator's output for determining the order of elements.
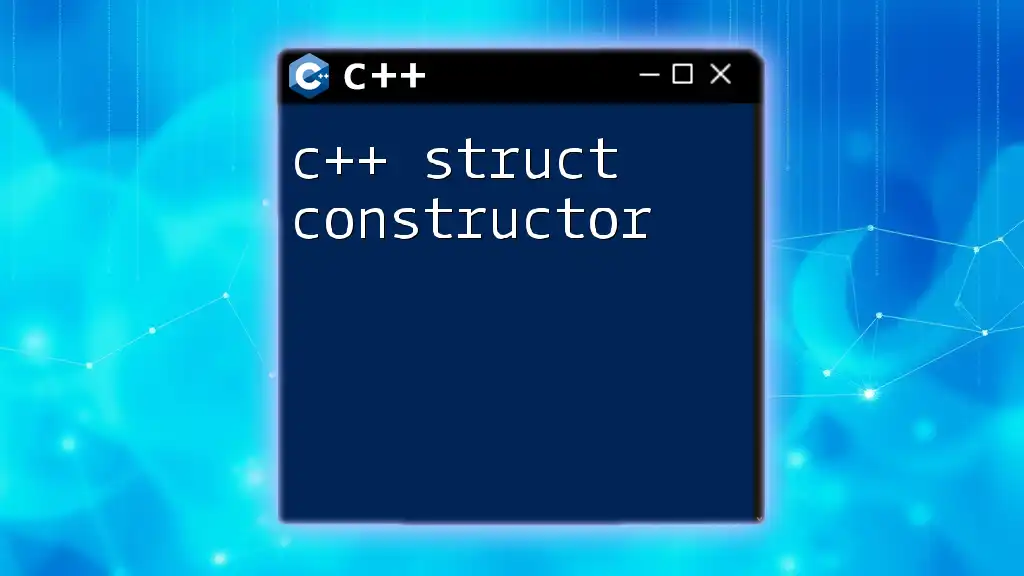
Conclusion
Understanding how to utilize c++ sort custom comparator capabilities can significantly enhance your programming toolkit. By mastering the art of creating custom comparators, you can optimize sorting routines tailored to your data structures and needs, unlocking greater flexibility and efficiency in your C++ applications.
Feel encouraged to experiment with the examples provided and apply custom comparators in your own projects, as practical implementation can solidify your understanding and skills in this powerful feature of C++.
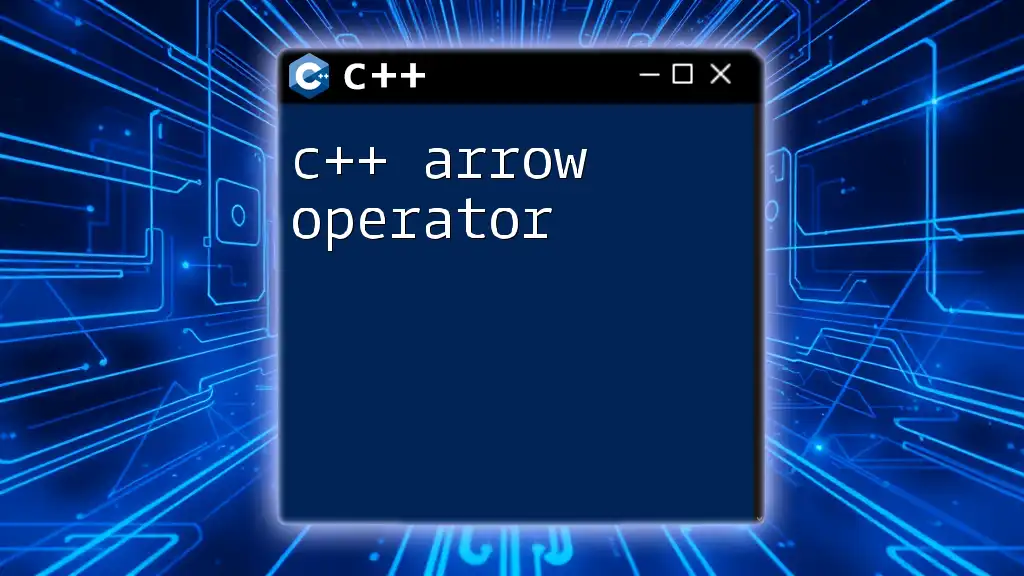
Additional Resources
For further reading, consider exploring the C++ documentation on `<algorithm>`, which provides in-depth insights into sorting algorithms and comparator functions, along with tutorials for various sorting techniques. Engage with the developer community to share your custom comparator examples and learn from others' experiences.