A C++ custom allocator allows you to define your own memory allocation strategy, enabling more control over memory management according to your application's specific needs.
#include <iostream>
#include <memory>
template <typename T>
class CustomAllocator {
public:
using value_type = T;
CustomAllocator() = default;
template <typename U>
CustomAllocator(const CustomAllocator<U>&) {}
T* allocate(std::size_t n) {
if (auto ptr = std::malloc(n * sizeof(T)))
return static_cast<T*>(ptr);
throw std::bad_alloc();
}
void deallocate(T* p, std::size_t) {
std::free(p);
}
};
int main() {
std::allocator_traits<CustomAllocator<int>>::rebind_alloc<int> alloc;
int* arr = alloc.allocate(5);
for (int i = 0; i < 5; ++i) arr[i] = i; // use the allocated memory
alloc.deallocate(arr, 5);
return 0;
}
Understanding Memory Allocation in C++
What is Memory Allocation?
Memory allocation in programming refers to the process of reserving a segment of memory to store data. In C++, memory can be divided into two main types: stack and heap. The stack is managed automatically by the compiler, while the heap is controlled manually by the programmer. Understanding how memory is allocated and managed is crucial for writing efficient and error-free code.
Standard Memory Management in C++
C++ provides several built-in ways to allocate and deallocate memory. The most common methods include using `new` and `delete` operators, along with `malloc`, `calloc`, and `free` functions. The default allocator in C++ allows you to dynamically create objects when their size isn’t known at compile time. While adequate for many applications, it can lead to performance bottlenecks or fragmentation when not optimized.
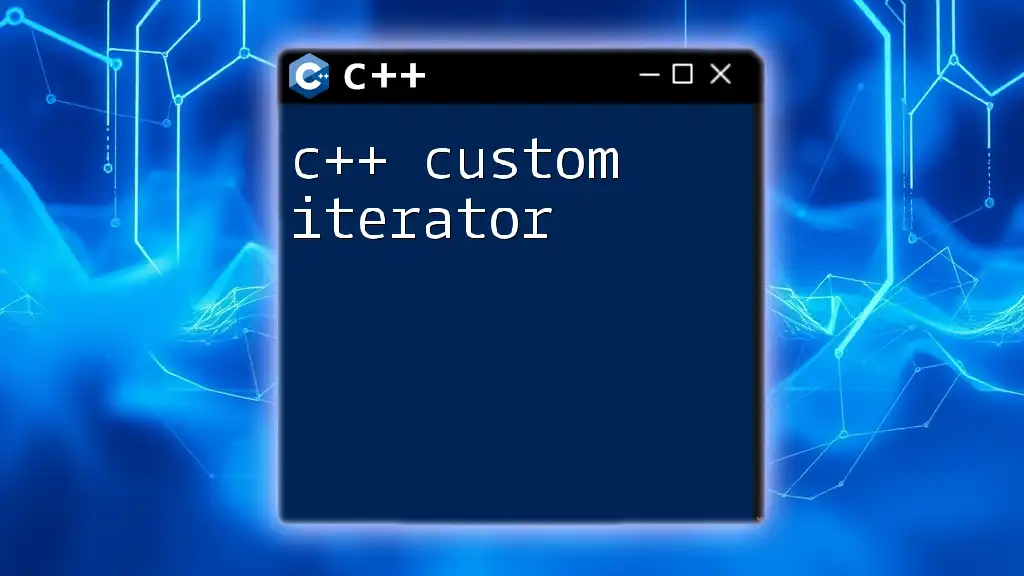
What is a Custom Allocator?
Defining a Custom Allocator
A custom allocator is a user-defined class or function that provides a way to allocate and deallocate memory according to specific criteria or requirements. Custom allocators are beneficial for improving the performance of an application by reducing fragmentation and managing memory more efficiently. By implementing a custom allocator, developers gain increased control over memory usage—tailoring it to specific needs for different data types or allocation patterns.
When to Consider a Custom Allocator
You might want to implement a custom allocator in situations such as:
- When your application requires high-performance memory management.
- For specialized data structures that frequently allocate and deallocate memory of specific sizes.
- In real-time applications where latency is critical, and you want to minimize memory allocation overhead.
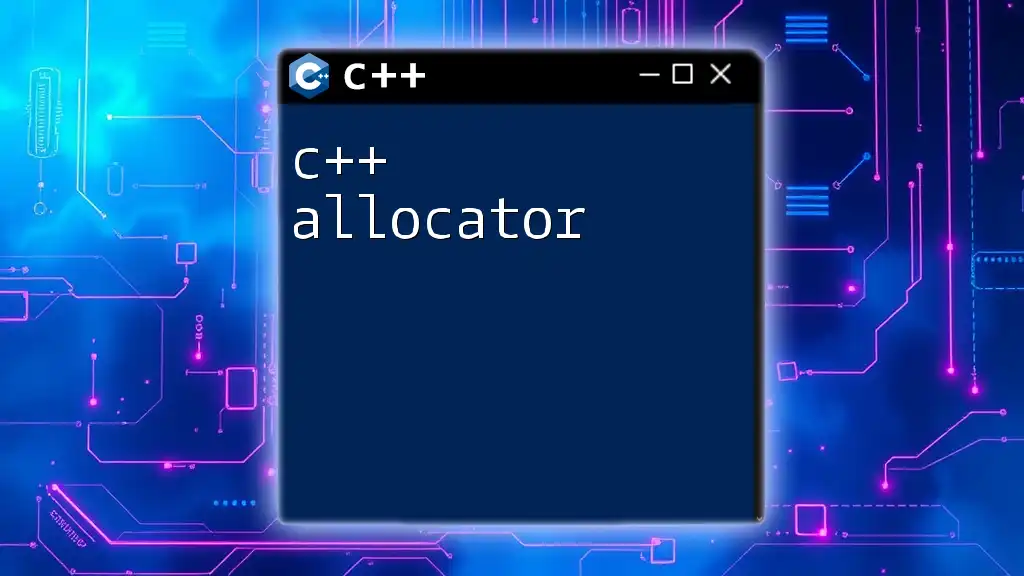
Implementing a Custom Allocator
Basic Structure of a Custom Allocator
To create a custom allocator, you’ll typically define a class that adheres to certain type traits. The key components of a custom allocator include allocate and deallocate methods. Here’s a simple structure of a custom allocator class:
template <typename T>
class CustomAllocator {
public:
using value_type = T;
CustomAllocator() {}
template <typename U> CustomAllocator(const CustomAllocator<U>&) {}
T* allocate(std::size_t n) {
if (n > std::size_t(-1) / sizeof(T))
throw std::bad_alloc();
return static_cast<T*>(::operator new(n * sizeof(T)));
}
void deallocate(T* p, std::size_t) {
::operator delete(p);
}
};
This basic implementation defines how memory is allocated and deallocated, creating a framework that can be tailored for specific needs.
Allocating Memory
The `allocate()` method is responsible for reserving memory. It takes the number of objects to allocate as an argument and returns a pointer to the allocated memory. It’s essential to check if the requested size exceeds the maximum allowable size to prevent overflow and potential crashing of the program.
Deallocating Memory
The `deallocate()` method releases memory previously allocated by the allocator. It is critical to call this function to avoid memory leaks, which can lead to excessive memory consumption and ultimately degrade application performance.
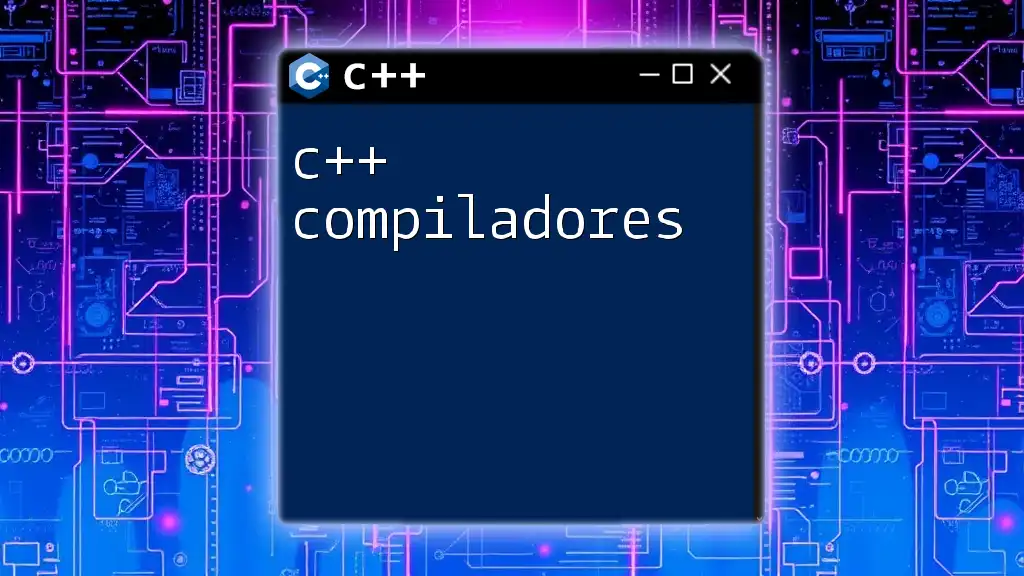
Advanced Custom Allocator Features
Debugging and Tracking Memory Usage
Implementing debugging features can enhance the effectiveness of a custom allocator. By keeping track of allocations, developers can monitor memory usage and identify issues such as leaks. Here’s how you might implement a simple tracking mechanism:
template <typename T>
class DebugAllocator : public CustomAllocator<T> {
private:
std::size_t allocationCount = 0;
public:
T* allocate(std::size_t n) {
allocationCount += n;
return CustomAllocator<T>::allocate(n);
}
void deallocate(T* p, std::size_t n) {
allocationCount -= n;
CustomAllocator<T>::deallocate(p);
}
std::size_t getAllocationCount() const { return allocationCount; }
};
This implementation extends the base allocator to track how many objects have been allocated, which can be invaluable during troubleshooting.
Pool Allocators
A pool allocator is a variation that manages a pool of memory blocks, allocating and deallocating fixed-size objects from that pool. This approach dramatically reduces fragmentation and speeds up allocation time, making it a suitable choice for applications that frequently allocate and deallocate numerous small objects.
Here’s a simple example of how a pool allocator might be implemented.
Example Pool Allocator
template <typename T>
class PoolAllocator {
private:
struct Node {
Node* next;
};
Node* freeList;
public:
PoolAllocator(std::size_t poolSize) {
// Allocate memory for the pool and initialize the free list
freeList = reinterpret_cast<Node*>(::operator new(poolSize * sizeof(T)));
Node* current = freeList;
for (std::size_t i = 0; i < poolSize - 1; ++i) {
current->next = reinterpret_cast<Node*>(reinterpret_cast<char*>(freeList) + (i + 1) * sizeof(T));
current = current->next;
}
current->next = nullptr; // Last node points to null
}
T* allocate() {
if (!freeList) throw std::bad_alloc();
Node* node = freeList;
freeList = freeList->next;
return reinterpret_cast<T*>(node);
}
void deallocate(T* p) {
Node* node = reinterpret_cast<Node*>(p);
node->next = freeList;
freeList = node;
}
~PoolAllocator() {
::operator delete(freeList);
}
};
Customizing the Allocator for Different Object Types
To use a custom allocator efficiently, you may need to customize it for various object types. Strategies include creating typed allocations based on size or characteristics, allowing the allocator to handle different types intelligently.
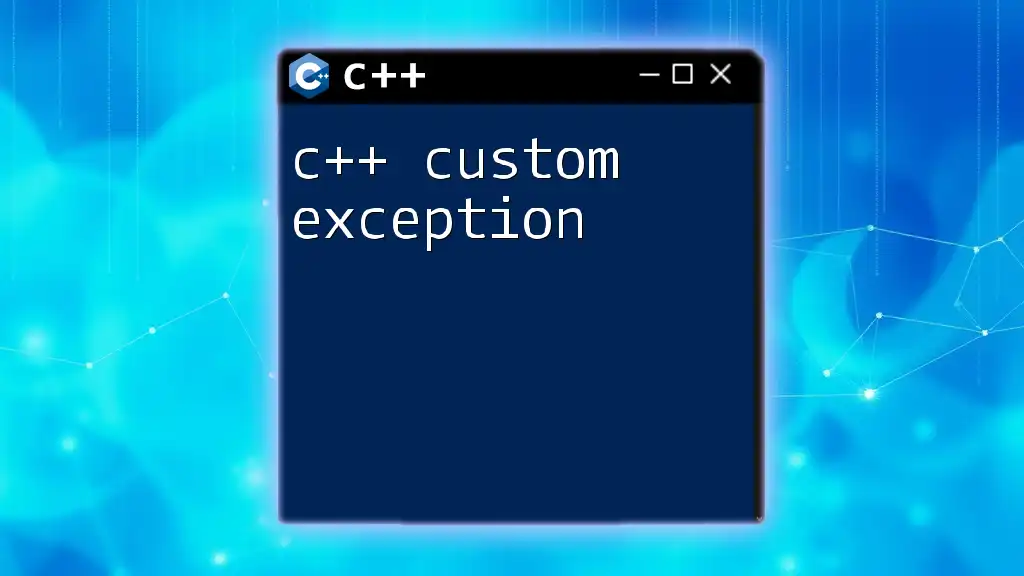
Using Custom Allocators with STL Containers
Integrating Custom Allocators with Standard Library Containers
The power of custom allocators is often fully realized when integrated with Standard Template Library (STL) containers like `std::vector`, `std::list`, or `std::map`. For example:
std::vector<int, CustomAllocator<int>> vec;
vec.push_back(1);
vec.push_back(2);
This code creates a `std::vector` that utilizes the `CustomAllocator`, enabling you to benefit from personalized memory management while leveraging the capabilities of STL containers.
Practical Tips for Using Custom Allocators
When implementing custom allocators, consider the following best practices:
- Keep It Simple: Start with basic features and gradually add complexity.
- Test Thoroughly: Ensure plenty of testing to avoid memory leaks and performance issues.
- Profile Performance: Use profilers to measure the impact of your custom allocator compared to standard methods.
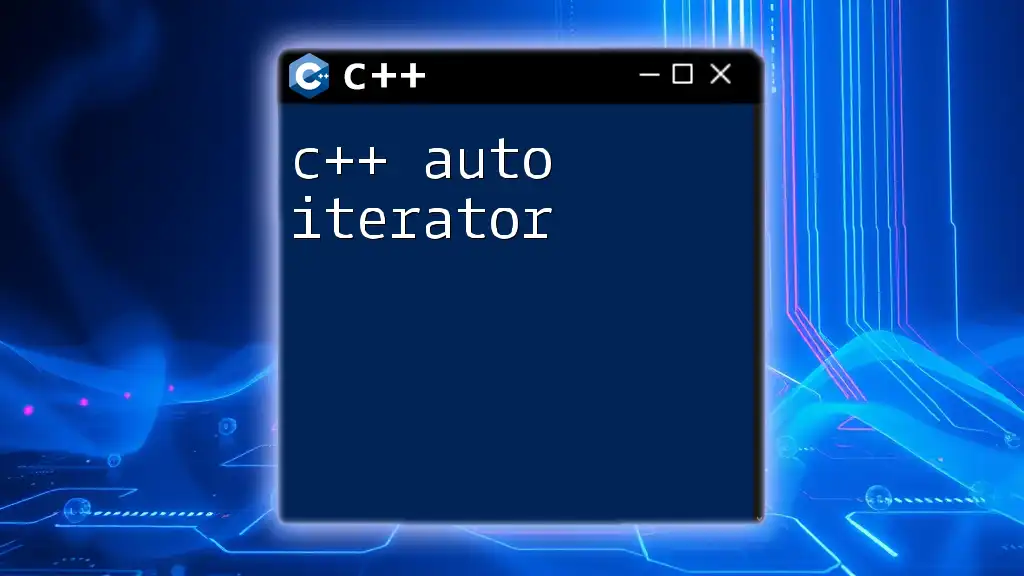
Conclusion
C++ custom allocators offer a powerful way to manage memory effectively, providing enhanced performance and control that can be tailored to your application's needs. Whether you are debugging memory issues, optimizing performance for specific scenarios, or simply experimenting with different allocation strategies, understanding how to implement and use custom allocators is an invaluable skill for any C++ developer.
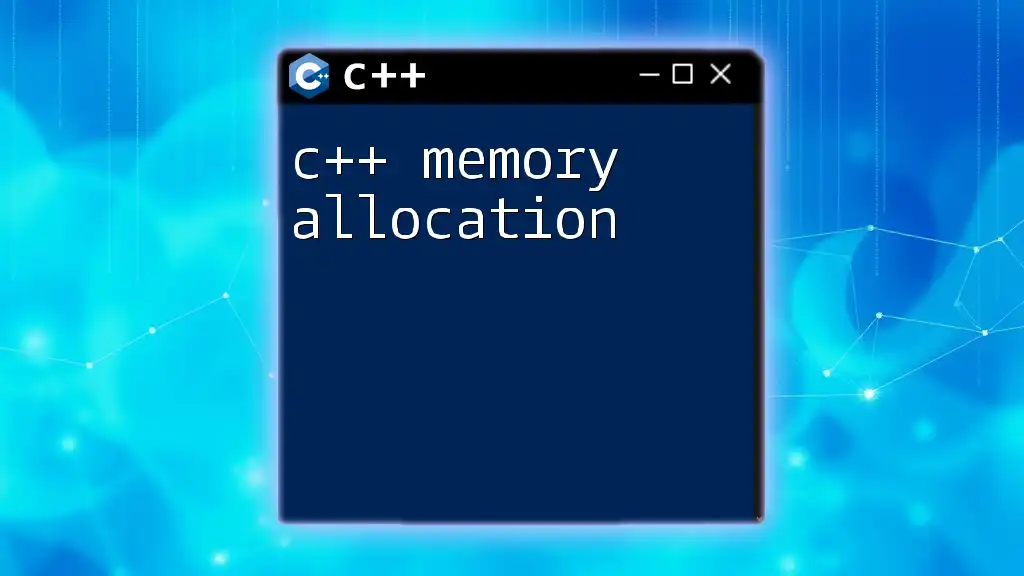
References and Additional Resources
For those eager to delve deeper into the world of C++ custom allocators, consider referring to:
- C++ Programming Language by Bjarne Stroustrup.
- Effective STL by Scott Meyers.
- Various online resources and GitHub repositories showcasing custom allocator implementations.
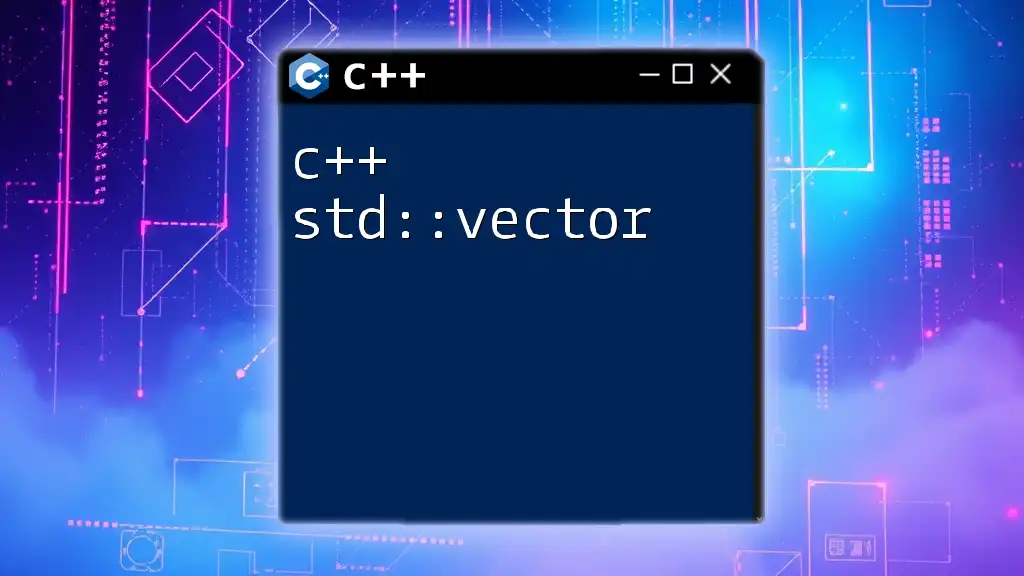
FAQ Section
Common Questions About Custom Allocators
-
What are the main advantages of using a custom allocator?
Custom allocators allow for performance optimizations, memory management flexibility, and tailored memory allocation strategies.
-
Are there any downsides?
Yes, using custom allocators can introduce complexity and require thorough testing to ensure they don't produce unexpected behavior compared to standard allocators.
-
Where can I find more examples?
GitHub repositories and online C++ forums often contain custom allocator implementations and discussions.