In C++, custom exceptions can be created by deriving a class from the standard `std::exception` class, allowing you to define specific error types for better error handling.
Here's a simple example:
#include <iostream>
#include <exception>
// Define a custom exception class
class MyCustomException : public std::exception {
public:
const char* what() const noexcept override {
return "This is a custom exception!";
}
};
int main() {
try {
throw MyCustomException();
} catch (const MyCustomException& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
What are Exceptions in C++?
Exceptions in C++ represent unexpected events that can occur during the execution of a program. They indicate that an error has occurred when performing an operation, such as accessing invalid memory or dividing by zero. Handling exceptions correctly is crucial for maintaining the stability and reliability of software applications.
When an exception is thrown, the normal flow of the program is interrupted. The language provides a mechanism to handle these anomalies gracefully using a set of specific keywords: `try`, `catch`, and `throw`.
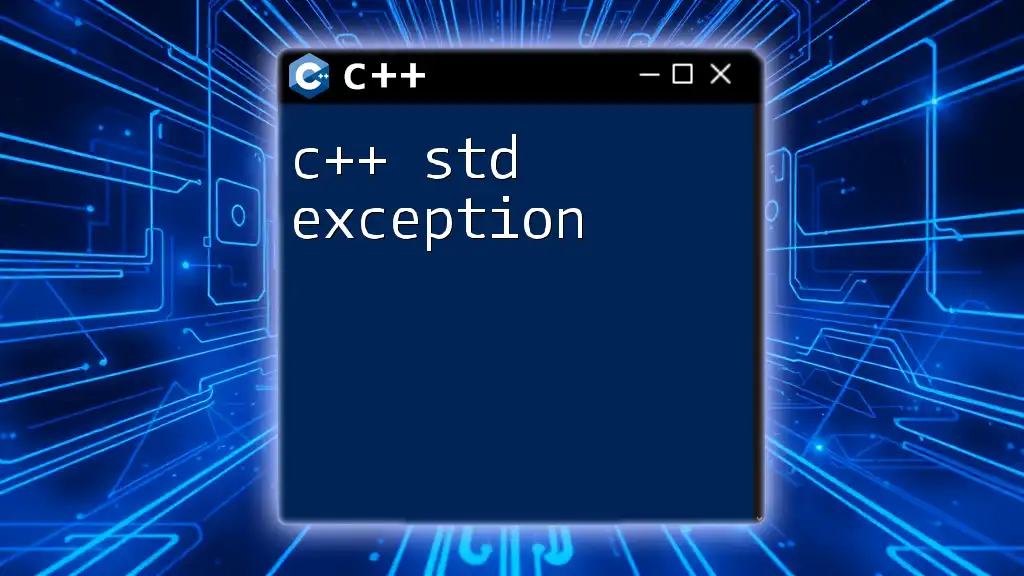
Why Create Custom Exceptions?
While C++ provides a rich set of standard exceptions, there are cases where predefined exceptions may not adequately describe the error conditions specific to your application. Creating custom exceptions is beneficial for the following reasons:
- Clarity: Custom exceptions enable you to convey specific error conditions clearly, making it easier for developers using your code to understand what went wrong.
- Control: You can provide tailored error messages and handling mechanisms that are aligned with your application’s logic.
- Hierarchy: By organizing custom exceptions into a hierarchy, you can catch related exception types in a single `catch` block.
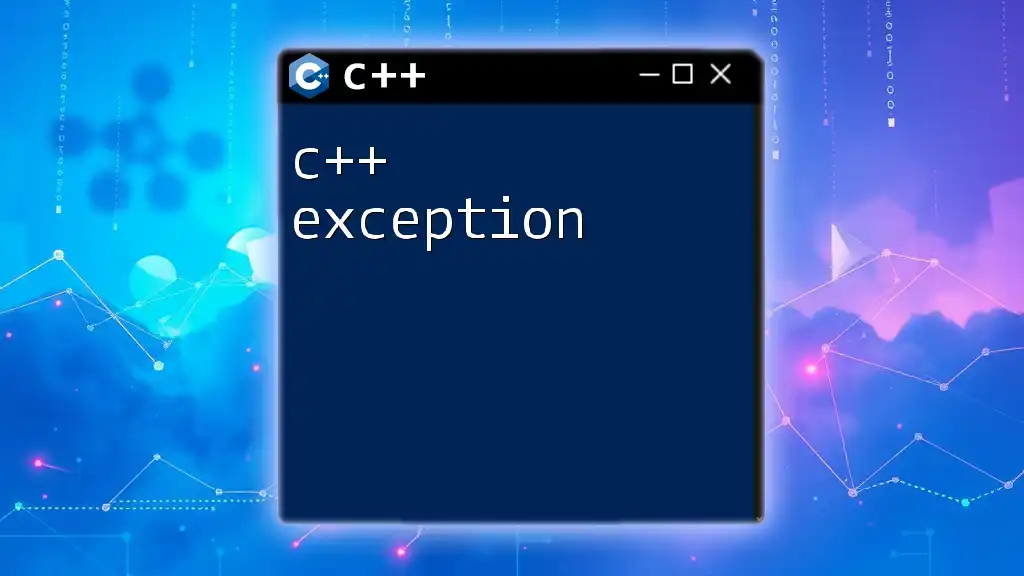
C++ Exception Handling Mechanism
The basic building blocks of exception handling in C++ are `try`, `catch`, and `throw`. Here's how they work:
- `try` block: Where you place code that may throw an exception.
- `throw` statement: Used to signal that an error has occurred.
- `catch` block: Handles the exception thrown in the try block.
For instance, consider the following example which demonstrates how to use standard exceptions in C++:
try {
int x = 10 / 0; // This will throw a division by zero error
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
In this example, dividing by zero leads to an exception, which is then caught and handled appropriately.
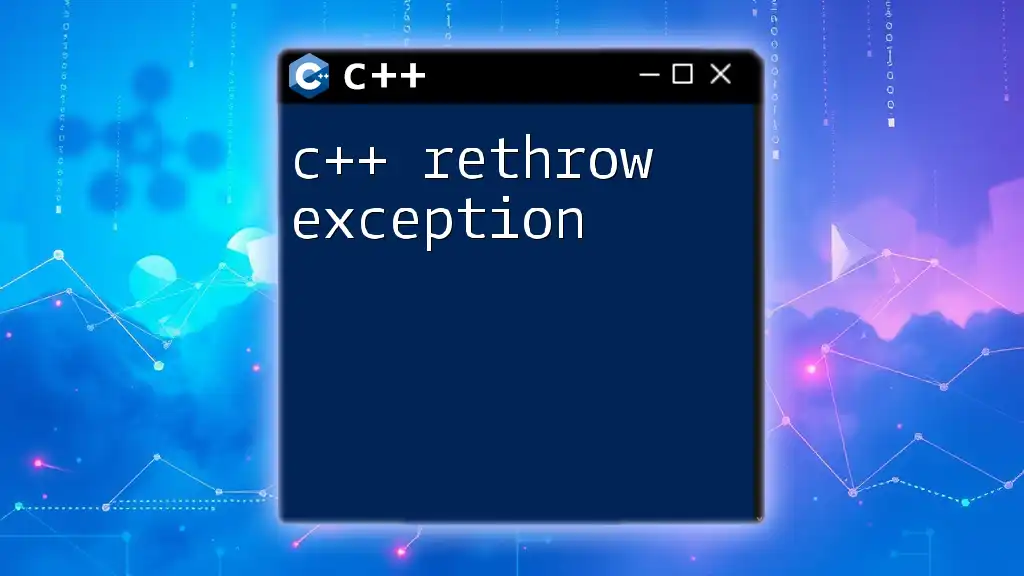
Defining a Custom Exception Class
Creating a custom exception starts with defining a class derived from `std::exception`. This enables you to inherit functionalities such as the `what()` method which returns an explanation of the exception. Here is an example:
class MyCustomException : public std::exception {
public:
const char* what() const noexcept override {
return "This is my custom exception message.";
}
};
In this class, overriding the `what()` function allows you to provide a specific message that can be accessed when the exception is caught.
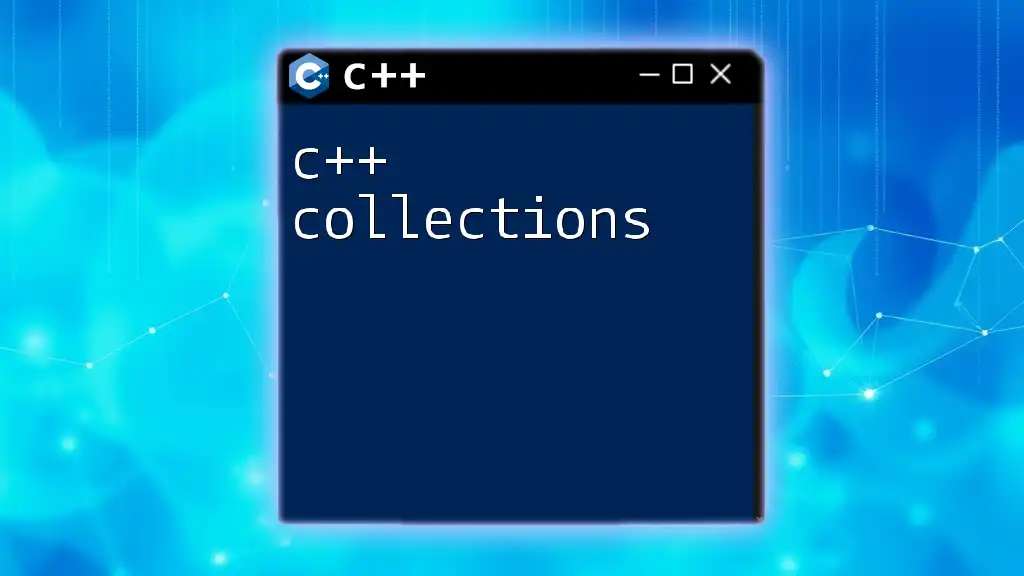
Throwing Custom Exceptions
Throwing a custom exception is straightforward. After defining your exception class, you can throw it in your code like this:
void riskyOperation() {
throw MyCustomException(); // Throws the custom exception
}
try {
riskyOperation();
} catch (const MyCustomException& e) {
std::cerr << "Caught: " << e.what() << std::endl;
}
In this case, `riskyOperation` can lead to a thrown exception that is caught in the catch block, where it prints the error message.
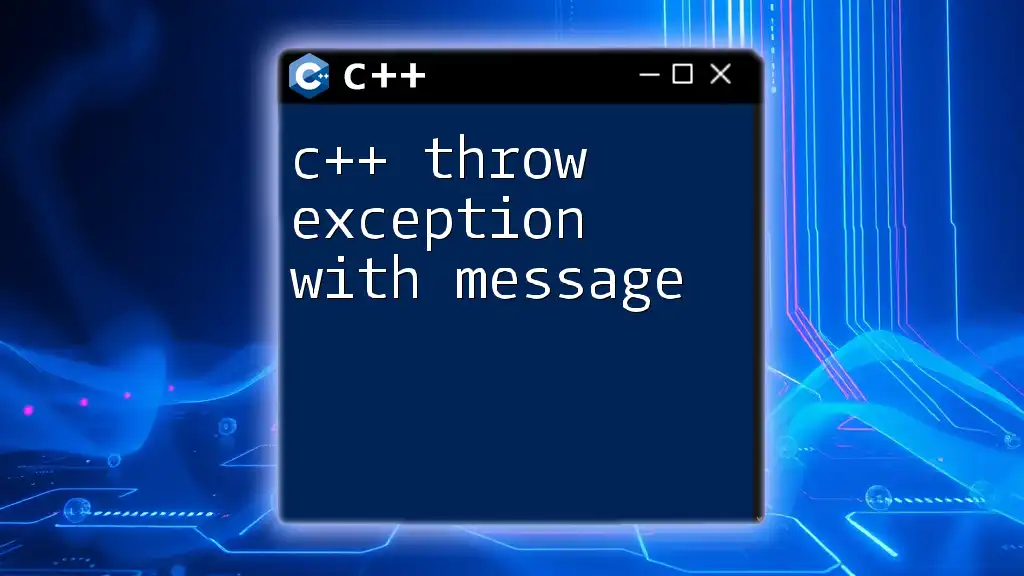
Best Practices for Using Custom Exceptions
Clear and Descriptive Error Messages
When creating custom exceptions, it's vital to provide comprehensive messages that adequately describe the error. This enhances debugging and makes it simpler for users of the code to understand the situation. Consider an exception message like "Invalid user input" rather than a vague message.
Creating Hierarchies of Custom Exceptions
For more complex systems, organizing your exceptions into a hierarchy can provide better clarity and structure. Here’s an example of a base custom exception and its derived classes:
class BaseException : public std::exception {};
class FileNotFoundException : public BaseException {
public:
const char* what() const noexcept override {
return "File not found exception.";
}
};
class PermissionDeniedException : public BaseException {
public:
const char* what() const noexcept override {
return "Permission denied exception.";
}
};
This approach allows you to catch all custom exceptions derived from `BaseException` in a single block, improving code readability in the process.
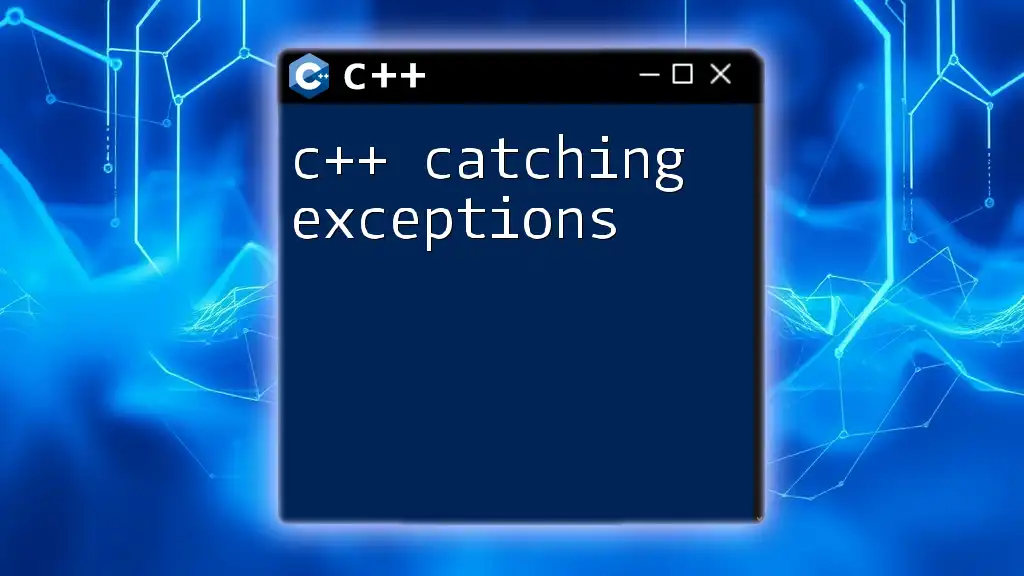
Practical Applications of Custom Exceptions
Use Case: File Operations
Custom exceptions excel in practical applications, such as file operations. Here’s how you can throw a custom exception for a file not found scenario:
void readFile(const std::string& filename) {
if (!fileExists(filename)) {
throw FileNotFoundException(); // Throws custom FileNotFoundException
}
// Read the file logic here
}
When attempting to read a file, if it doesn't exist, the custom exception provides clarity on the specific error.
Use Case: User Input Validation
Validating user input is another area where custom exceptions shine. You can create specific exceptions to indicate various input errors:
void validateInput(int input) {
if (input < 0) {
throw std::invalid_argument("Negative input not allowed."); // Using standard exception
}
}
By using clear exception types, users of the API can easily understand the rules for valid input.
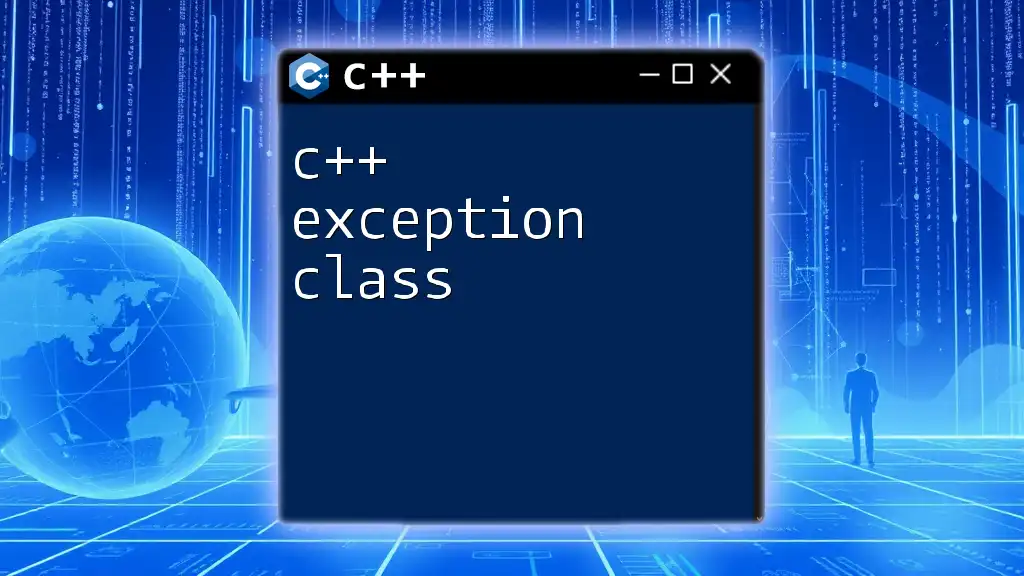
Catching Custom Exceptions
Catching custom exceptions follows the same principles as standard exceptions. It’s often useful to catch specific types of exceptions for tailored error handling. Here’s an example that demonstrates multiple catch blocks:
try {
readFile("non_existent_file.txt");
} catch (const FileNotFoundException& e) {
std::cerr << "Caught: " << e.what() << std::endl; // Handles FileNotFoundException
} catch (const BaseException& e) {
std::cerr << "Caught a base exception: " << e.what() << std::endl; // Catches any BaseException
}
This structure allows you to treat different exceptions differently, providing more targeted responses based on the error.
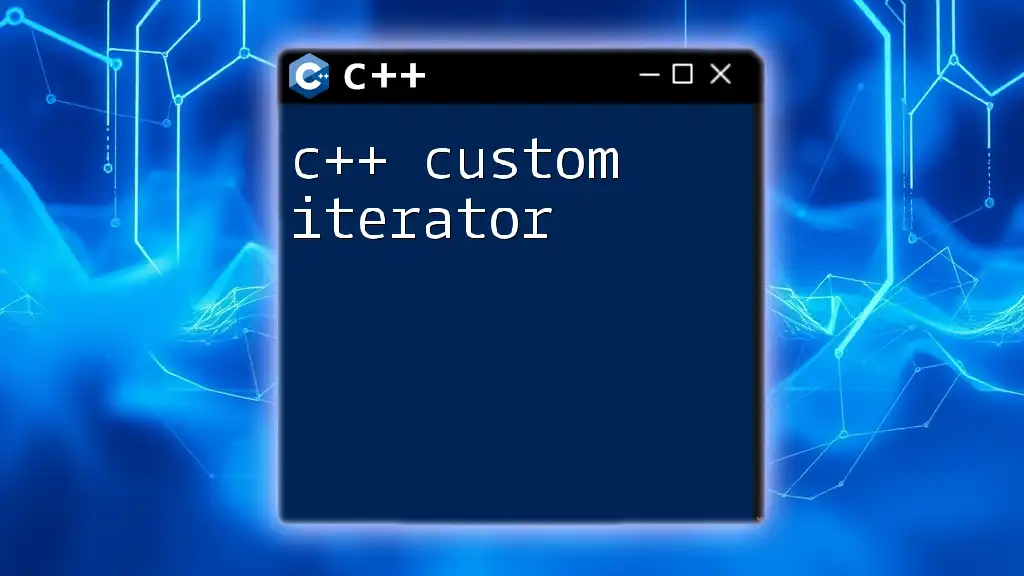
Recap of Key Points
To summarize, custom exceptions in C++ empower developers to create a clear, structured way to handle specific error conditions that may arise during program execution. They enhance clarity through descriptive messages, allow for organized error hierarchies, and improve the robustness of code through better error management.
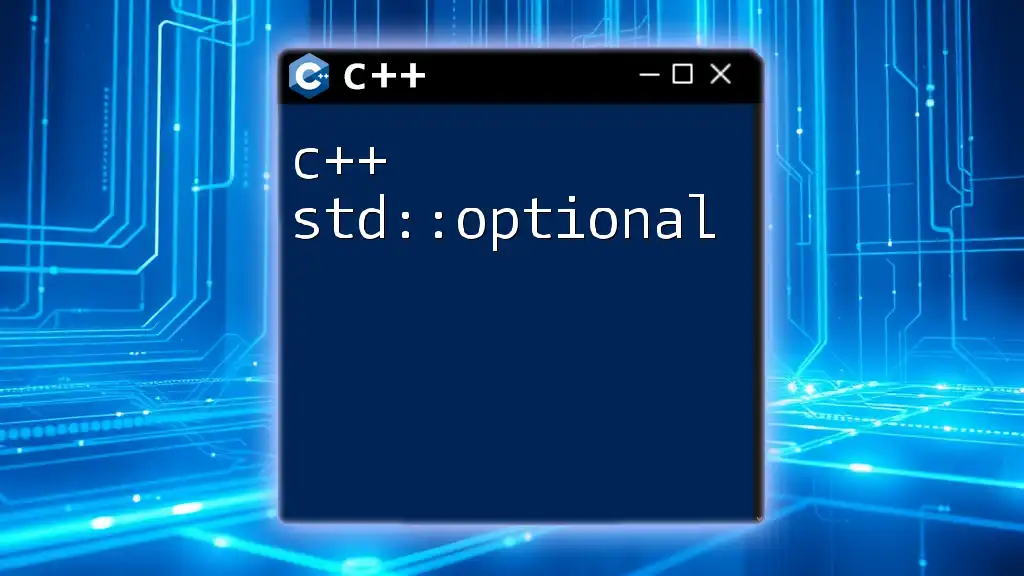
Encouraging Further Learning
As you dive deeper into C++ and explore the nuances of exception handling, consider checking out various online resources and code repositories. These will provide practical examples and projects, helping solidify your understanding of custom exceptions and their best practices.
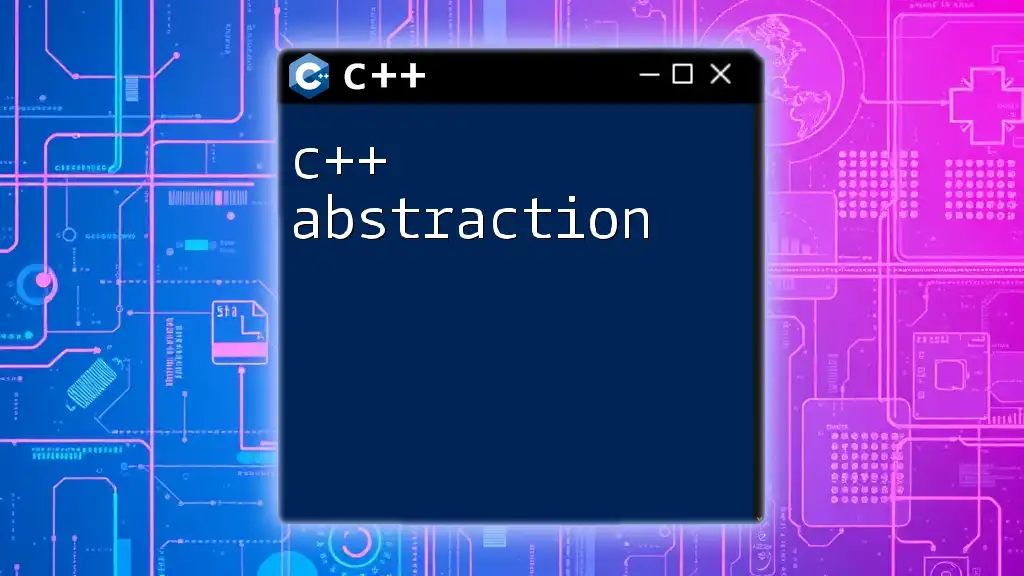
FAQs about C++ Custom Exceptions
What is the difference between standard and custom exceptions? Standard exceptions are predefined in C++ and cover common error scenarios. Custom exceptions are user-defined and tailored to specific application logic.
When should I create a custom exception? Custom exceptions are most useful when existing exception types do not adequately represent the error conditions in your application.
Can custom exceptions have additional member variables? Yes, custom exceptions can include additional member variables to store related error information, allowing for more detailed context when exceptions are caught.