C++ cmath functions provide a collection of mathematical operations and utilities, such as trigonometric calculations, logarithmic functions, and rounding, that enable precise and efficient computations within your programs.
Here’s a simple example demonstrating the use of some cmath functions:
#include <iostream>
#include <cmath>
int main() {
double x = 3.14;
std::cout << "Sin: " << sin(x) << std::endl;
std::cout << "Cos: " << cos(x) << std::endl;
std::cout << "Exp: " << exp(x) << std::endl;
std::cout << "Log: " << log(x) << std::endl;
return 0;
}
Understanding the C++ cmath Library
The cmath library is an essential component of C++ programming, specifically designed to handle mathematical operations efficiently. It provides a variety of mathematical functions and constants that enhance computational capabilities.
Although cmath is built upon the older `math.h` header, it comes with additional features, such as improved type safety and namespace management. It is imperative to include this library in your program for using any of its functions.
To incorporate the cmath library in your code, simply use the following directive at the beginning of your program:
#include <cmath>
This line makes all functions and constants from the cmath library accessible in your program, allowing for complex mathematical computations to be performed effortlessly.
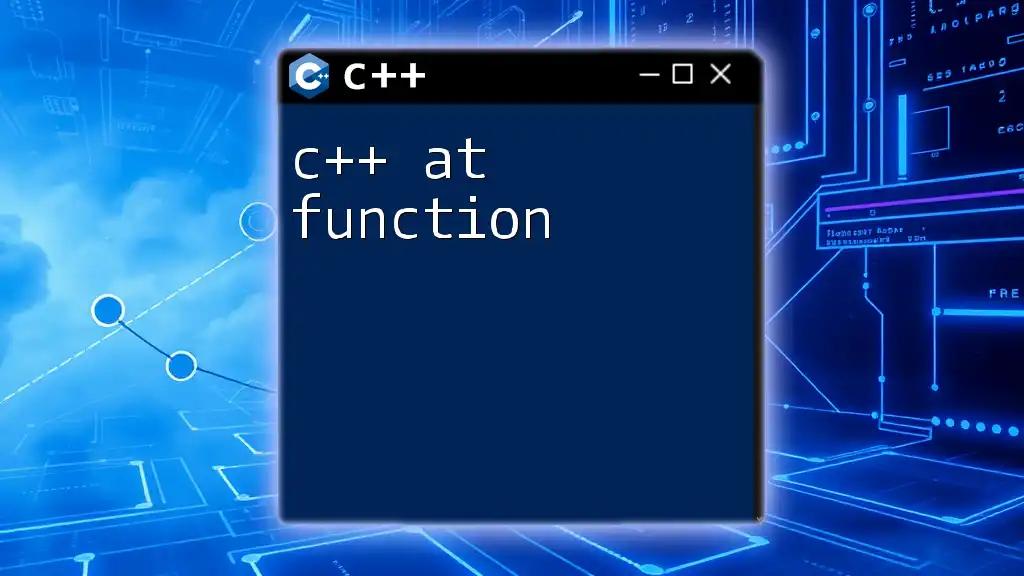
Core C++ cmath Functions
The C++ cmath functions can be broadly categorized based on their functionality, including trigonometric functions, exponential and logarithmic functions, rounding functions, and hyperbolic functions.
Trigonometric Functions
Trigonometric functions are fundamental in various fields, including physics, engineering, and computer graphics. These functions relate angles to the lengths of triangle sides.
Key functions in this category include:
- `sin()`: Computes the sine of an angle.
- `cos()`: Computes the cosine of an angle.
- `tan()`: Computes the tangent of an angle.
- `asin()`: Computes the arcsine (inverse sine).
- `acos()`: Computes the arccosine (inverse cosine).
- `atan()`: Computes the arctangent (inverse tangent).
Example Usage:
#include <iostream>
#include <cmath>
int main() {
double angle = 45.0; // degrees
double radians = angle * (M_PI / 180.0); // convert degrees to radians
std::cout << "Sine of 45 degrees: " << sin(radians) << std::endl;
return 0;
}
In this example, we've converted an angle from degrees to radians, which is necessary since the `sin()` function expects the angle in radians.
Exponential and Logarithmic Functions
Exponential and logarithmic functions are crucial for computational mathematics. They find applications in various fields such as finance, computer science, and natural sciences.
Key functions include:
- `exp()`: Computes the exponential of a number.
- `log()`: Computes the natural logarithm (base e).
- `log10()`: Computes the base 10 logarithm.
- `pow()`: Computes a number raised to a specified power.
Example Usage:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
std::cout << "2 raised to the power of 3 is: " << pow(base, exponent) << std::endl;
return 0;
}
Here, the `pow()` function is utilized to perform exponentiation, demonstrating its clear and concise syntax.
Rounding Functions
Rounding functions are essential for managing precision in numerical calculations. They help truncate or adjust the values to integer forms when necessary.
Key rounding functions include:
- `ceil()`: Rounds up to the nearest integer.
- `floor()`: Rounds down to the nearest integer.
- `round()`: Rounds to the nearest integer using standard rules.
- `trunc()`: Truncates the decimal part, effectively rounding towards zero.
Example Usage:
#include <iostream>
#include <cmath>
int main() {
double value = 4.7;
std::cout << "Ceiling of 4.7: " << ceil(value) << std::endl; // Outputs 5
std::cout << "Floor of 4.7: " << floor(value) << std::endl; // Outputs 4
return 0;
}
In this snippet, we see how the rounding functions work. `ceil()` rounds up, while `floor()` rounds down, yielding precise control over numerical outcomes.
Hyperbolic Functions
Hyperbolic functions are useful in various mathematical contexts, particularly in calculus and complex analysis. They resemble trigonometric functions but are based on hyperbolas rather than circles.
Key functions include:
- `sinh()`: Computes the hyperbolic sine.
- `cosh()`: Computes the hyperbolic cosine.
- `tanh()`: Computes the hyperbolic tangent.
Example Usage:
#include <iostream>
#include <cmath>
int main() {
double x = 1.0;
std::cout << "Hyperbolic sine of 1: " << sinh(x) << std::endl;
return 0;
}
This code illustrates how to compute the hyperbolic sine using the `sinh()` function. Each hyperbolic function has its distinct applications, especially in areas involving growth models and physics.
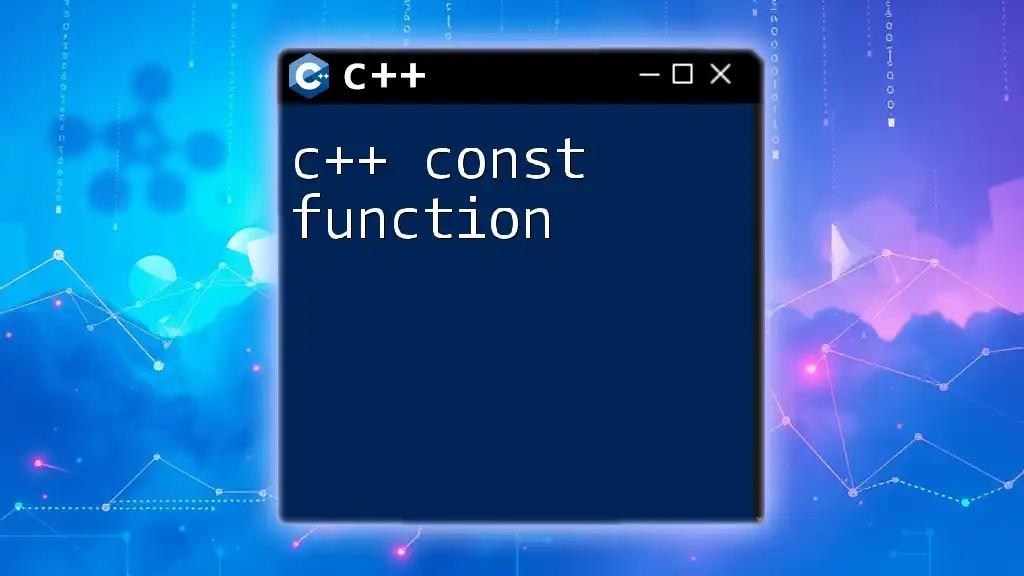
C++ Cmath Constants
Constants play a vital role in mathematical computations, providing essential values that are frequently used. Among the well-known constants in the cmath library, we have:
- `M_PI`: Represents the value of π (Pi).
- `M_E`: Represents Euler's number e.
Example Usage:
#include <iostream>
#include <cmath>
int main() {
std::cout << "Value of Pi: " << M_PI << std::endl; // Outputs 3.14159...
return 0;
}
Using constants such as `M_PI` allows developers to write clearer and more readable code, reducing the risk of numeric errors and improving maintainability.
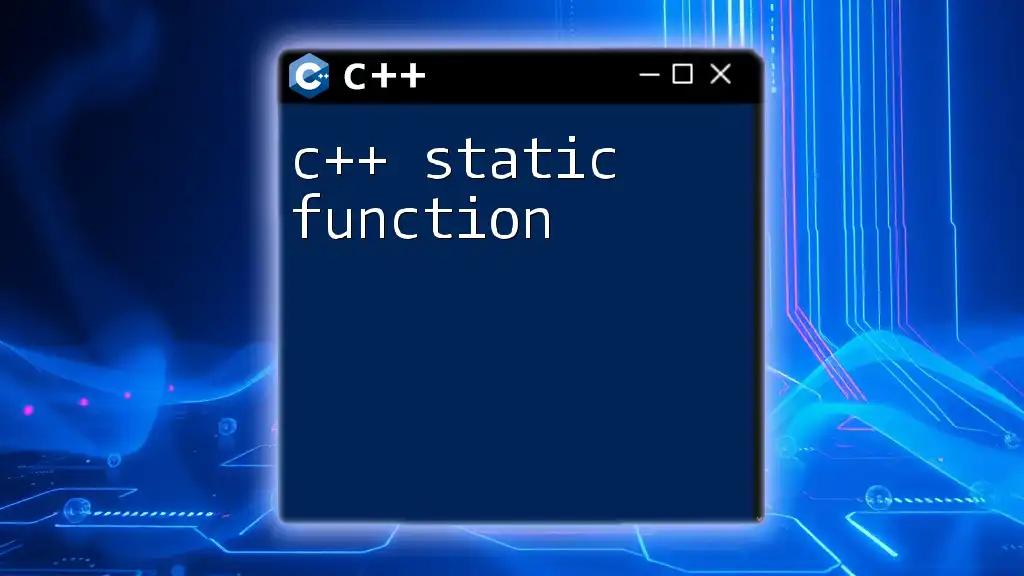
Best Practices for Using C++ Cmath Functions
When utilizing C++ cmath functions, consider the following best practices:
- Optimize Performance: When using cmath functions in performance-critical applications, it's best to analyze which functions are computationally heavy and optimize their usage.
- Be Aware of Precision: Understand that floating-point arithmetic can introduce errors. Hence, rounding should be handled carefully to avoid inaccuracies.
- Double-check Function Parameters: Always verify that the input values are within the expected range to prevent runtime errors.
Additionally, common pitfalls, such as assuming all sine, cosine, and tangent inputs are in degrees instead of radians, should be avoided. Such oversights can lead to erroneous results.
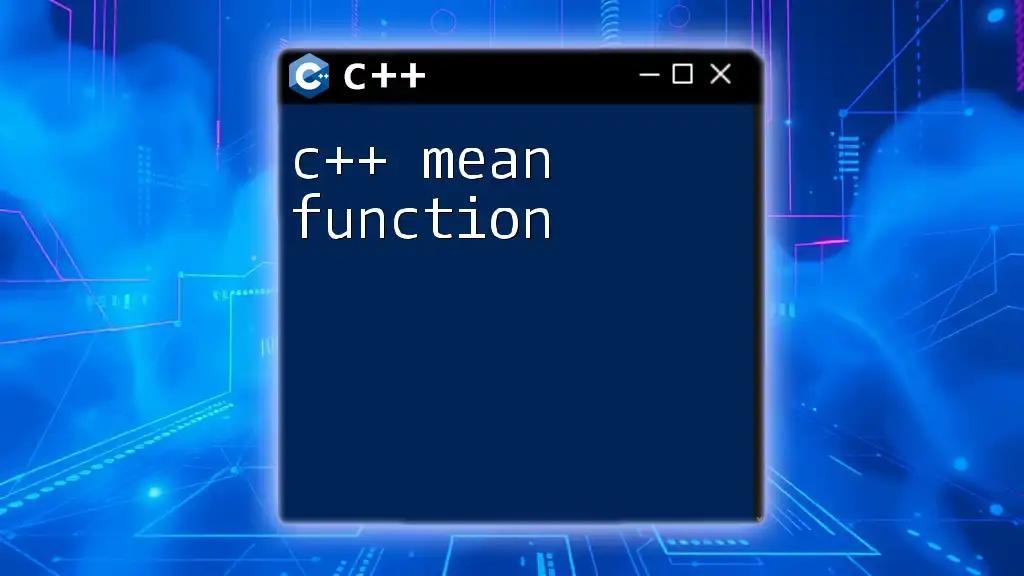
Conclusion
C++ cmath functions serve as a powerful toolkit for developers, enabling them to perform complex mathematical operations efficiently. By mastering the utilization of these functions, programmers can optimize their applications and improve the quality of their calculations. Exploring the cmath library is a worthy investment for those looking to enhance their proficiency in C++ programming.
Additional Resources
For further exploration, consider referencing the official C++ standards on cmath and additional tutorials available online to deepen your understanding and practical skills with C++ cmath functions.