The `main` function is the entry point of a C++ program where execution begins, and it typically returns an integer value to signify the completion status of the program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the Main Function?
The cpp main function serves as the entry point for C++ programs. When you execute a C++ program, the operating system looks for the `main` function to begin executing code. This makes it vital to understand its structure and purpose.
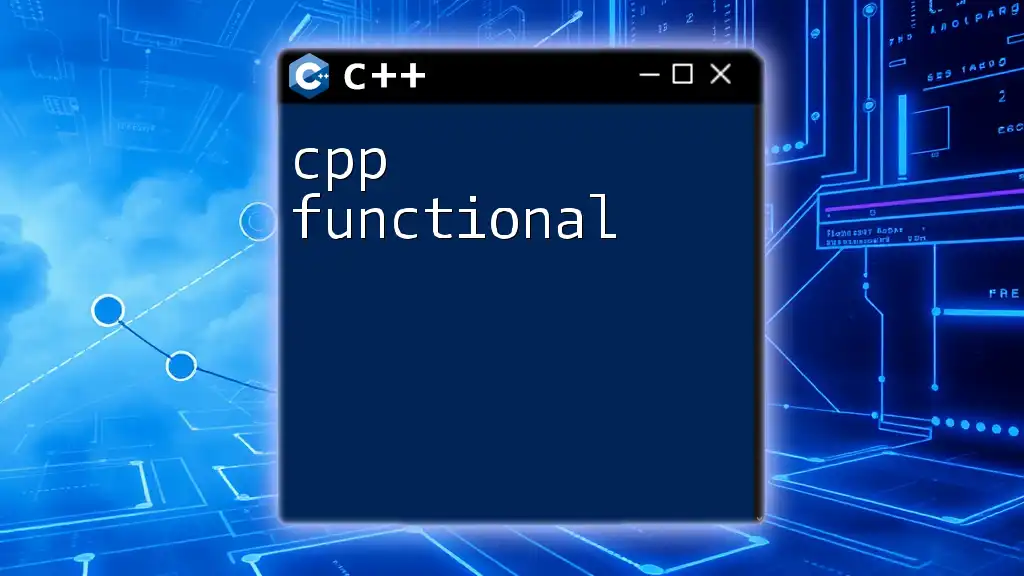
Structure of the C++ Main Function
Basic Syntax of the Main Function in C++
The basic structure of the `main` function is as follows:
int main() {
return 0;
}
In this example, `int` signifies that the function returns an integer. The `return 0;` statement indicates successful execution of the program.
Return Type of the Main Function
The return type of `main` is generally int, which allows the function to send a status code back to the operating system. While typically returning `0` means success, returning a non-zero value indicates an error.
Parameters of the Main Function
The `main` function can accept command-line arguments. This is useful for passing additional information when executing the program. The standard way to write this is:
int main(int argc, char* argv[]) {
// your code here
return 0;
}
Here, `argc` (argument count) is the number of command-line arguments passed, and `argv` (argument vector) is an array of C-style strings. For example, if you run `./program arg1 arg2`, then `argc` would be `3` (including the program name), and `argv` would hold the strings `{"./program", "arg1", "arg2"}`.
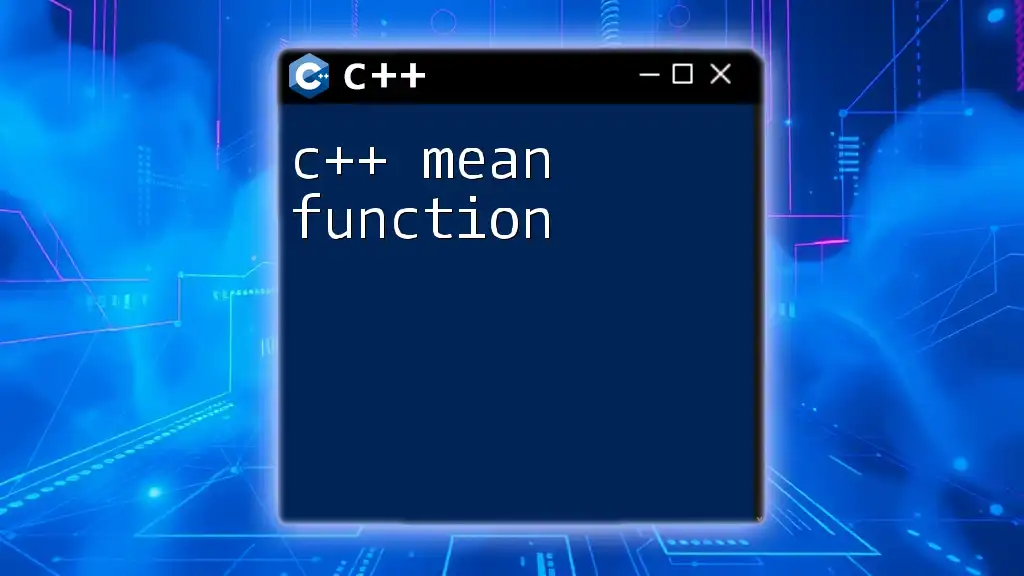
The Role of the Main Function in C++
Entry Point of the Program
The primary role of the `main` function in C++ is to act as the starting point of any executable program. When the runtime environment of C++ starts, it initializes all necessary resources before invoking `main`. This ensures that your code runs smoothly.
What Happens After `main` Completes?
Once `main` completes execution, it sends a return value back to the operating system. If you simply write `return 0;`, your program communicates that it has finished running successfully. A non-zero return value, on the other hand, signals an error state.
int main() {
return 1; // indicates an error
}
This type of exit code can be very useful when running scripts or automating tasks, allowing users to identify what went wrong.
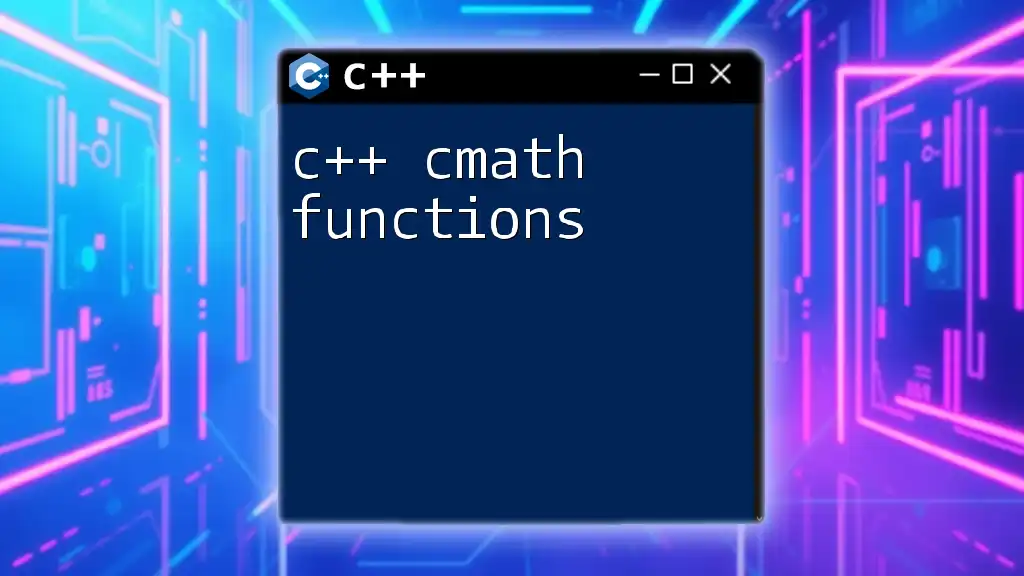
Variations of the Main Function
Using `void main()`
While some may write `void main()`, this usage has historically been frowned upon. The C++ standard specifies that `main` must return an integer, so using `void` can lead to undefined behavior.
void main() {
// Not recommended
}
Main Function with No Parameters
In situations where you don't need to handle command-line arguments, you can simplify `main` even further:
int main() {
// Your code here
return 0;
}
This version is sufficient for many basic applications.
Using the `auto` Keyword in Main Function
With C++17 and beyond, you can utilize the `auto` keyword to infer the return type:
auto main() -> int {
return 0;
}
This syntax allows for a more modern and flexible writing style while still conforming to the C++ standard.
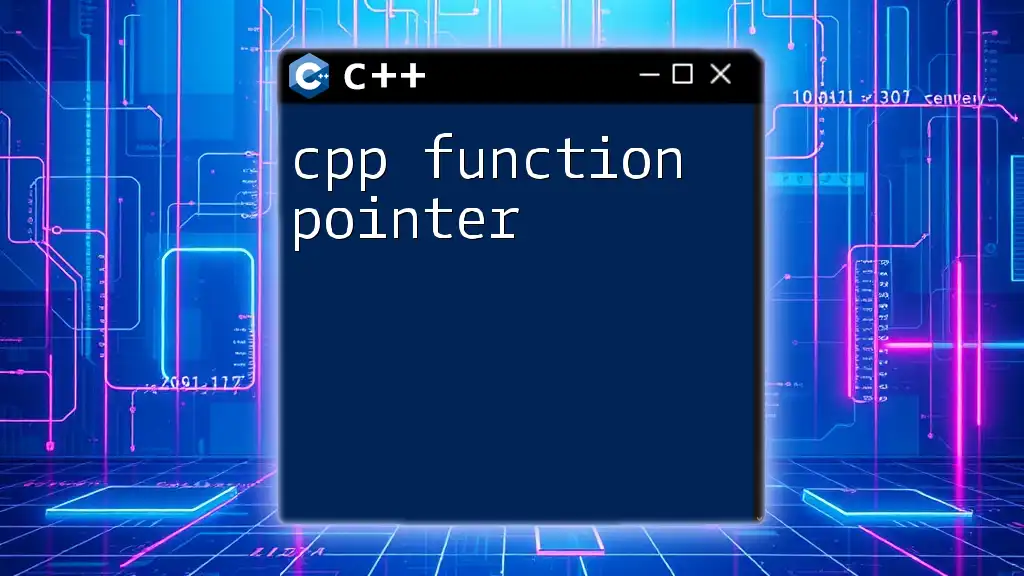
Best Practices for Writing the Main Function in C++
Keep It Simple!
It's beneficial to keep the `main` function concise. Only include essential logic, and defer more extensive operations to separate functions. This approach enhances maintainability and debugging.
int main() {
std::cout << "Program starts here." << std::endl;
return 0;
}
Utilizing Functions and Classes Beyond Main
Good programming practices dictate utilizing functions and classes to organize your code outside the `main` function. This not only improves readability but also makes your code reusable.
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
In this example, the `greet` function is defined outside of `main`, allowing you to call it within `main` for cleaner code.
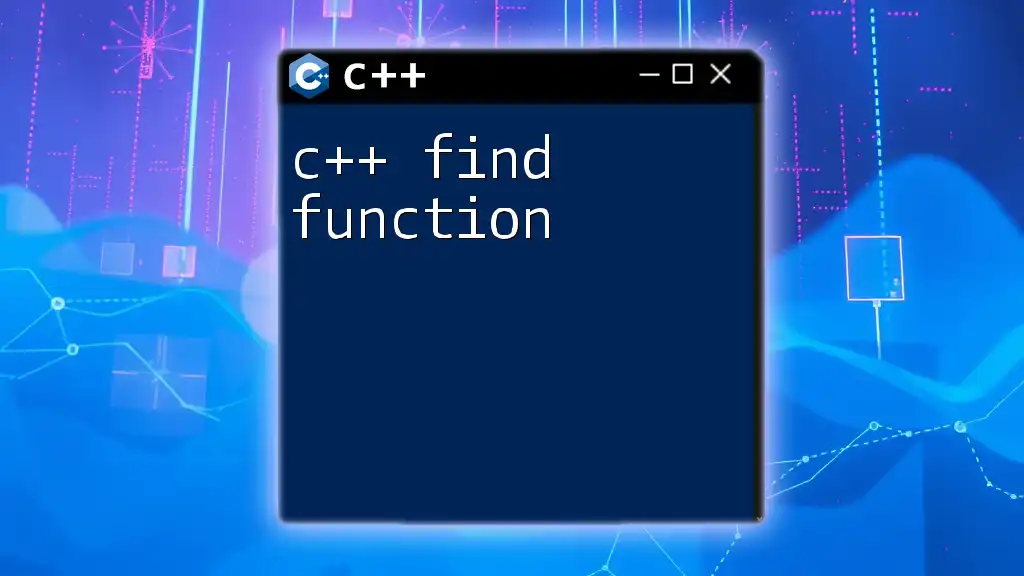
Common Mistakes with the C++ Main Function
Not Returning Values
A common mistake is neglecting to return a value from the `main` function. This can lead to undefined behavior. Always remember to include a return statement.
Incorrect Parameter Usage
When using command-line parameters, ensure you're handling them appropriately. Failing to check for the number of arguments could lead to dereferencing issues.
Ignoring Exit Codes
Setting proper exit codes is crucial for debugging. Return codes help users and other programs understand if a process succeeded or failed, so always set meaningful exit statuses.
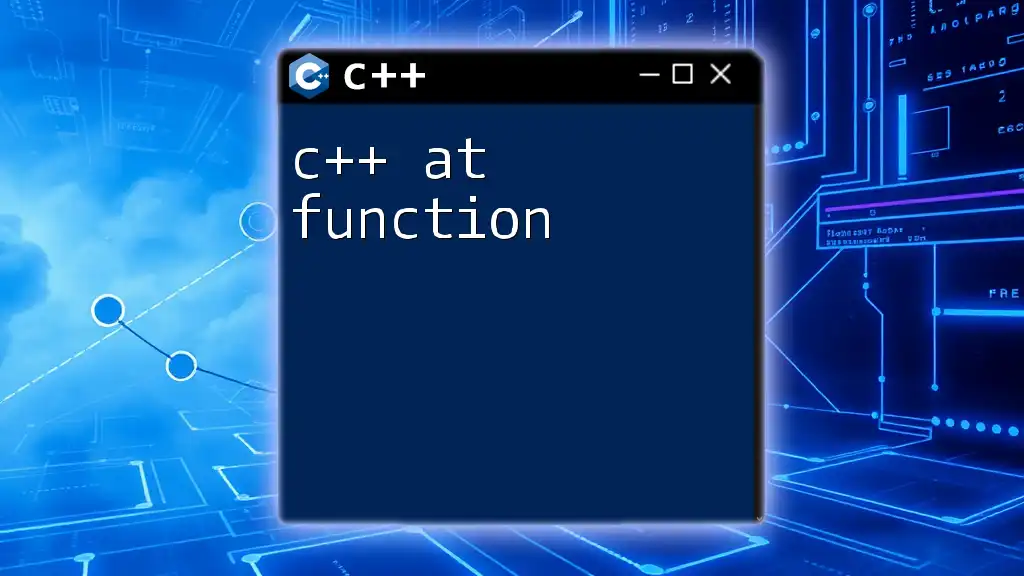
Debugging Tips Related to the Main Function
Using Print Statements Effectively
One of the simplest yet most effective debugging methods is to use print statements. This allows you to track the flow of your program easily.
int main() {
std::cout << "Starting program..." << std::endl;
// Your code here
return 0;
}
This adds an additional layer of clarity during execution.
Using Debuggers
Employing a debugger can offer a granular view of how your program executes. Step through code, inspect variables, and determine where issues may arise.
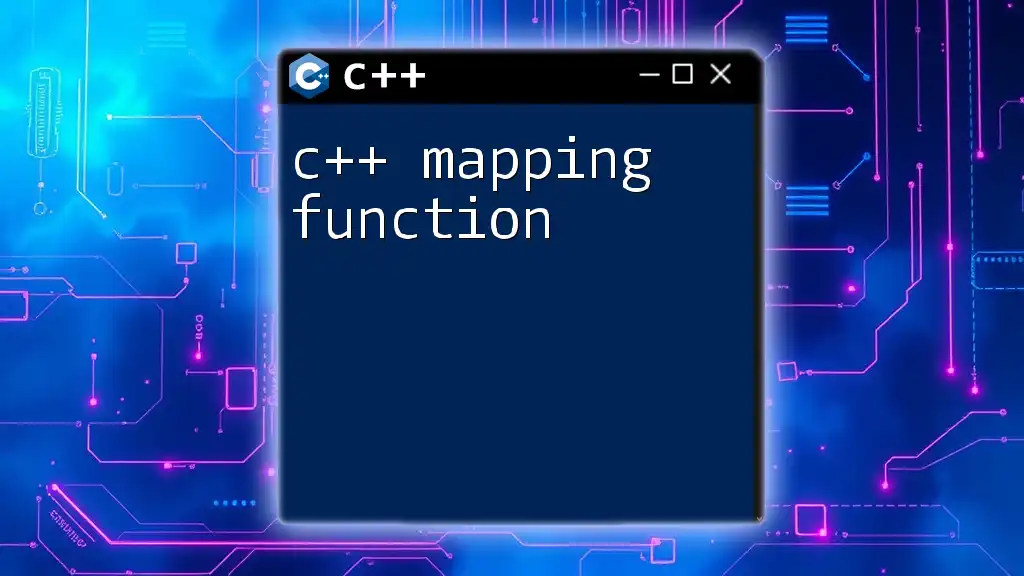
Conclusion
Understanding the cpp main function is essential for anyone delving into C++. By recognizing its structure, various forms, and common pitfalls, you pave the way for more robust programming. Practice writing different versions of the `main` function and implementing them in your projects.
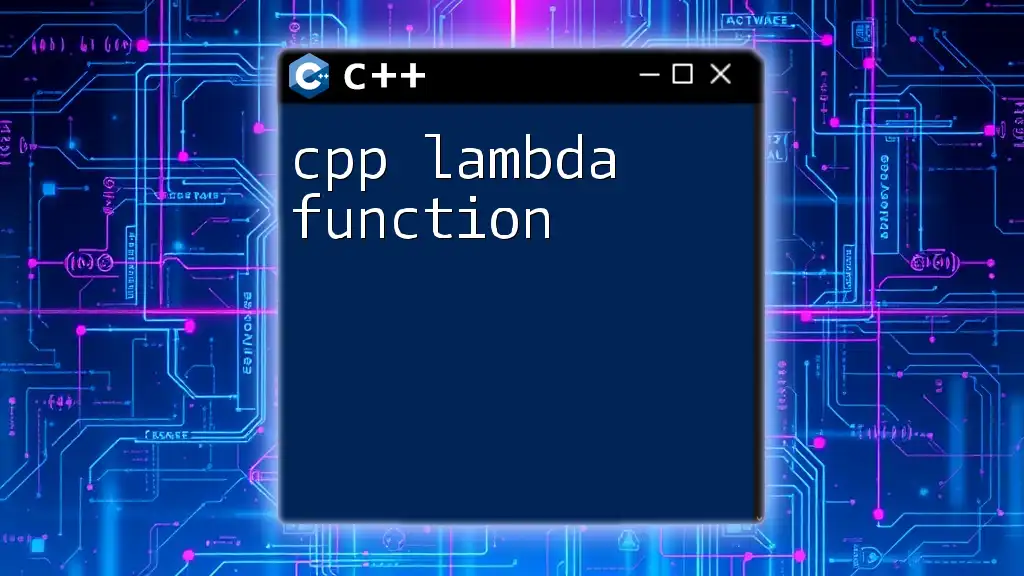
Call to Action
Join our learning community to dive deeper into the realm of C++ programming. Subscribe for more tutorials, commands, and tips to elevate your coding skills!