The C++ `max` function returns the larger of two values, which can be useful for comparisons in programming.
#include <iostream>
#include <algorithm> // For std::max
int main() {
int a = 5, b = 10;
int maximum = std::max(a, b);
std::cout << "The maximum value is: " << maximum << std::endl;
return 0;
}
Understanding the Maximum Function in C++
The C++ maximum function is a fundamental utility that allows developers to efficiently determine and return the larger of two given values. This functionality is crucial in various programming tasks, from simple comparisons to complex algorithms. Understanding how to use the maximum function can greatly simplify your code and improve performance.
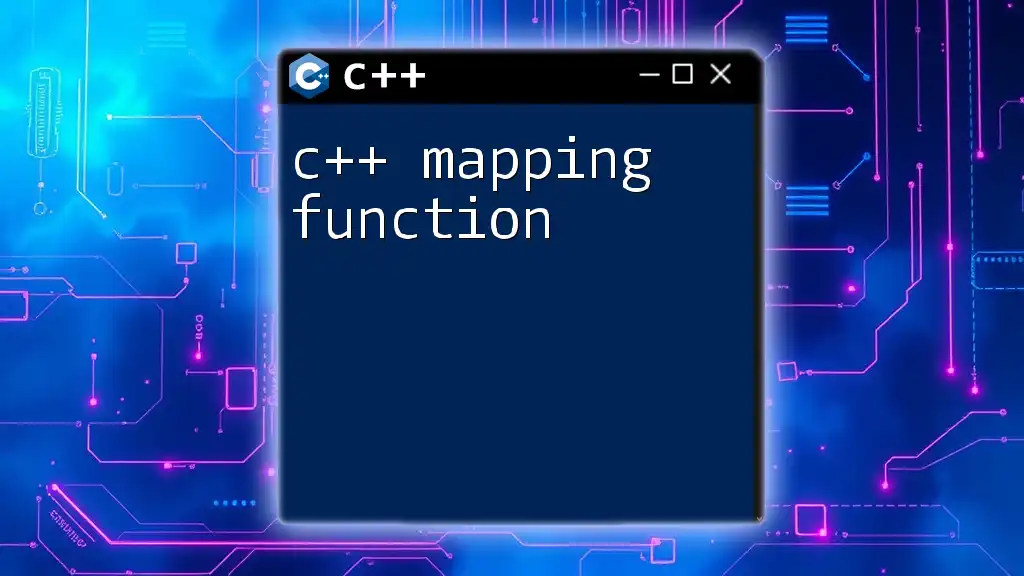
Syntax and Parameters of the max Function
The syntax for the `max` function in C++ is straightforward:
max(type a, type b)
- Parameters: The function takes two parameters, `a` and `b`, which must be of the same type or compatible types.
- Return Type: The function returns the value that is the greater of the two arguments. If both values are equal, it returns the first value.
Built-in vs User-defined max Function
Built-in max Function
C++ provides a built-in `max` function through the `<algorithm>` header, which is optimized for various data types and includes type deducing features. To utilize this, you must include the header in your program:
#include <algorithm>
User-defined max Function
While the built-in `max` function suffices for most scenarios, there may be cases where you need to create a custom `max` function, especially if you're dealing with complex types or specific conditions. Here’s an example of a simple user-defined max function:
template<typename T>
T myMax(T a, T b) {
return (a > b) ? a : b;
}
This template function can now handle various data types, making it versatile for multiple use cases.
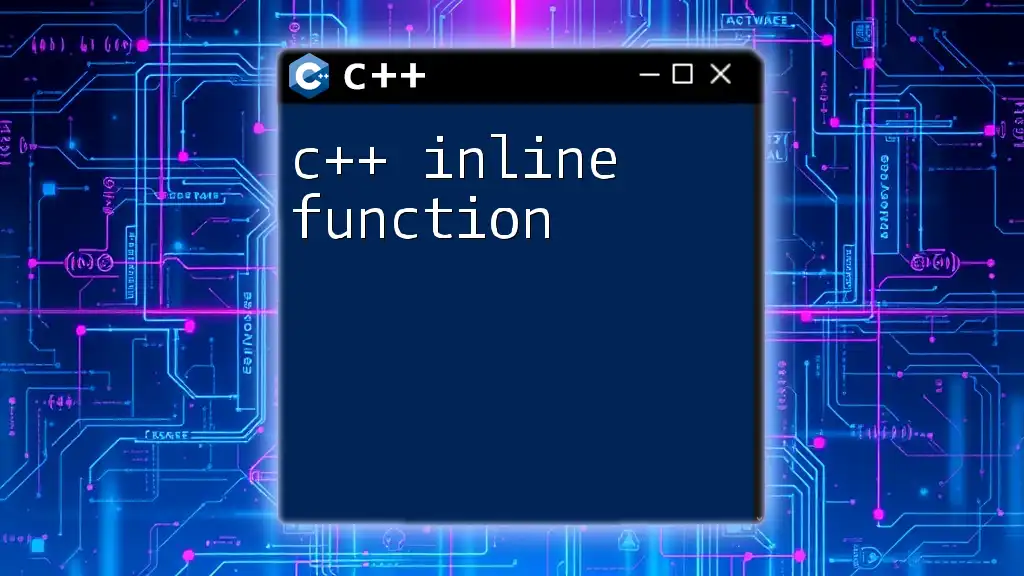
Using max Function in C++
Utilizing the C++ maximum function is both simple and effective. Below are basic examples showcasing how to employ it in various scenarios.
Example 1: Finding Maximum in a Simple Scenario
Here’s a straightforward example of finding the maximum of two integers:
#include <iostream>
#include <algorithm>
int main() {
int a = 10, b = 20;
std::cout << "Maximum: " << std::max(a, b) << std::endl;
return 0;
}
This code snippet declares two integers and outputs their maximum. The result will be `20`.
Example 2: Using max Function with Different Data Types
The `max` function can handle different data types seamlessly. Consider the following example using `double` values:
#include <iostream>
#include <algorithm>
int main() {
double x = 3.5, y = 7.2;
std::cout << "Maximum: " << std::max(x, y) << std::endl;
return 0;
}
In this case, the output will be `7.2`, demonstrating the function's capability to operate on floating-point numbers.
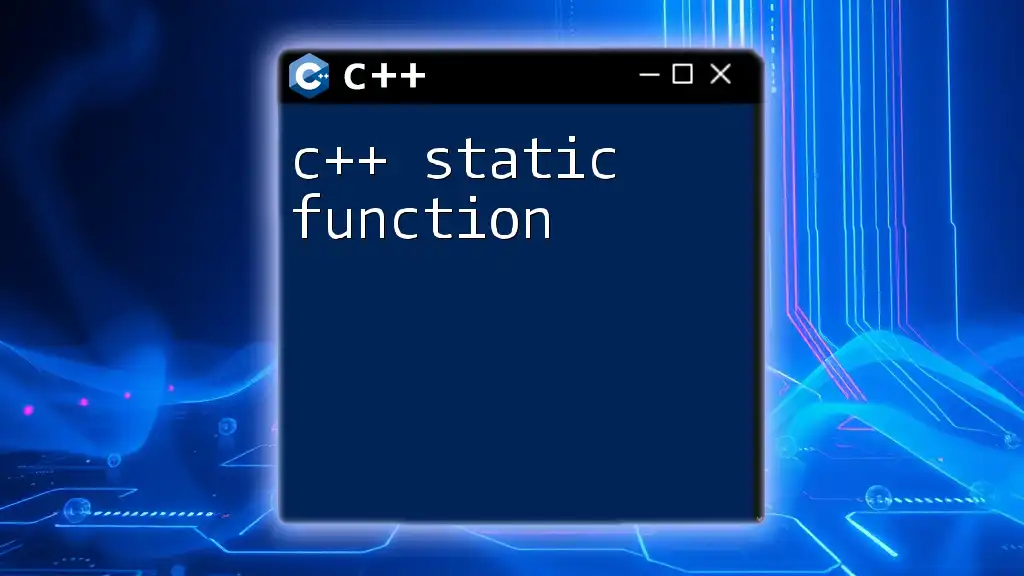
Advanced Usage of the Max Function
The C++ maximum function can also be employed in conjunction with containers and iterables, offering even more flexibility in programming.
Example 3: Finding Maximum in a Vector
Using the `max` function with a vector highlights its utility in real-world applications:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 5, 3, 9, 2};
auto maxElement = *std::max_element(vec.begin(), vec.end());
std::cout << "Maximum in Vector: " << maxElement << std::endl;
return 0;
}
In this example, `std::max_element` is used to find the maximum value within a vector of integers. The output will be `9`, the highest value in the collection.
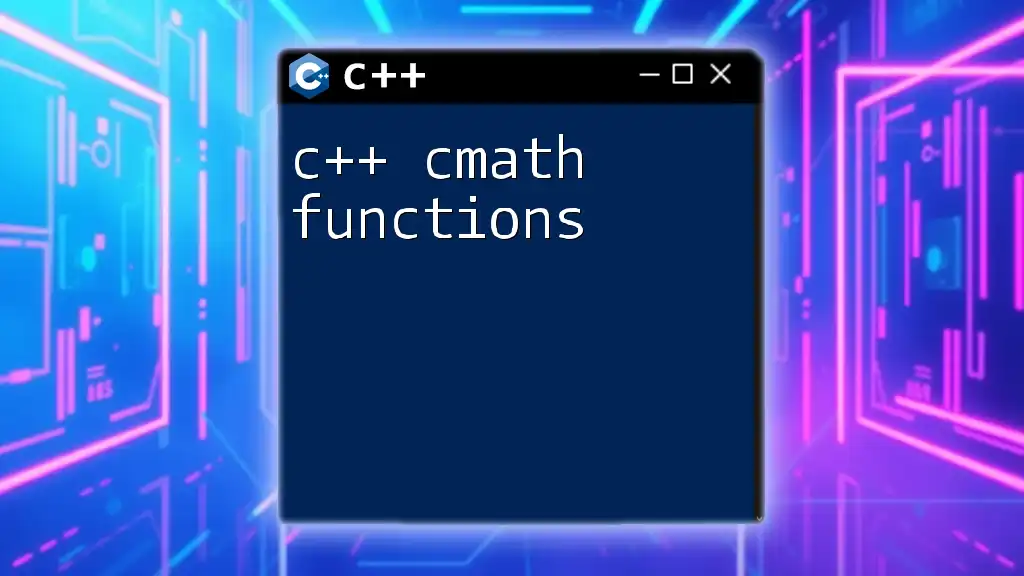
The C++ Math max Function
C++ also provides the `std::fmax` function in the `<cmath>` header for floating-point comparisons, which behaves differently compared to `std::max`:
Example 4: Using std::fmax for Floating Point Comparison
Consider the following example, which demonstrates the handling of special floating point values:
#include <iostream>
#include <cmath>
int main() {
float f1 = 3.5, f2 = std::nan("");
std::cout << "Maximum: " << std::fmax(f1, f2) << std::endl; // Explanation of NaN handling
return 0;
}
Here, if one of the values is NaN (not-a-number), `std::fmax` will return the other number, showcasing its robustness in numerical computations.
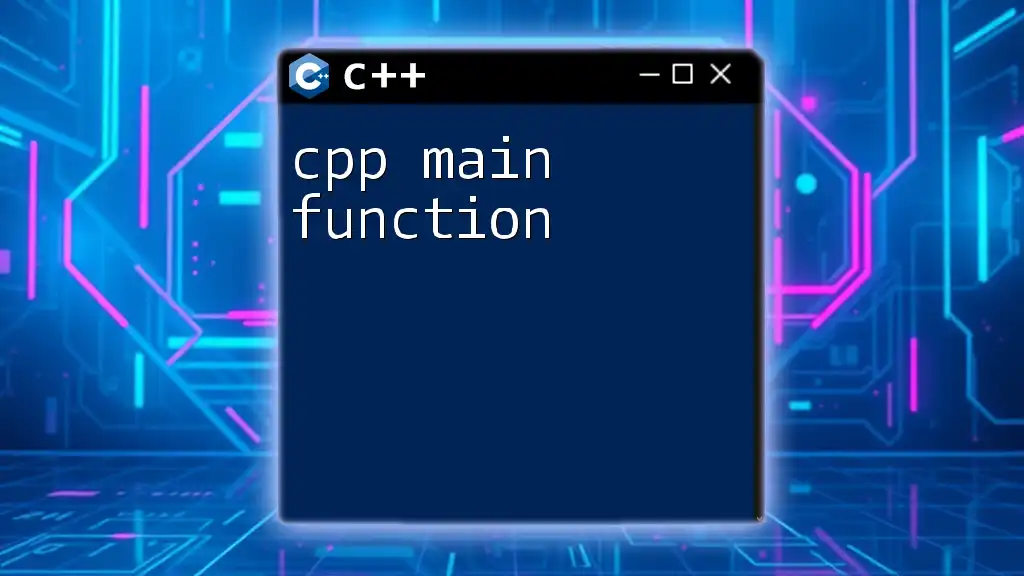
Common Mistakes with the Max Function
While working with the C++ maximum function, developers may encounter several common pitfalls:
- Type Promotion Misunderstanding: If incompatible types are passed to the `max` function, it may lead to unexpected results due to implicit type conversions. Ensure that arguments are of compatible types.
- Using Uninitialized Variables: Passing uninitialized variables to the `max` function can lead to undefined behavior. Always initialize variables before comparison.
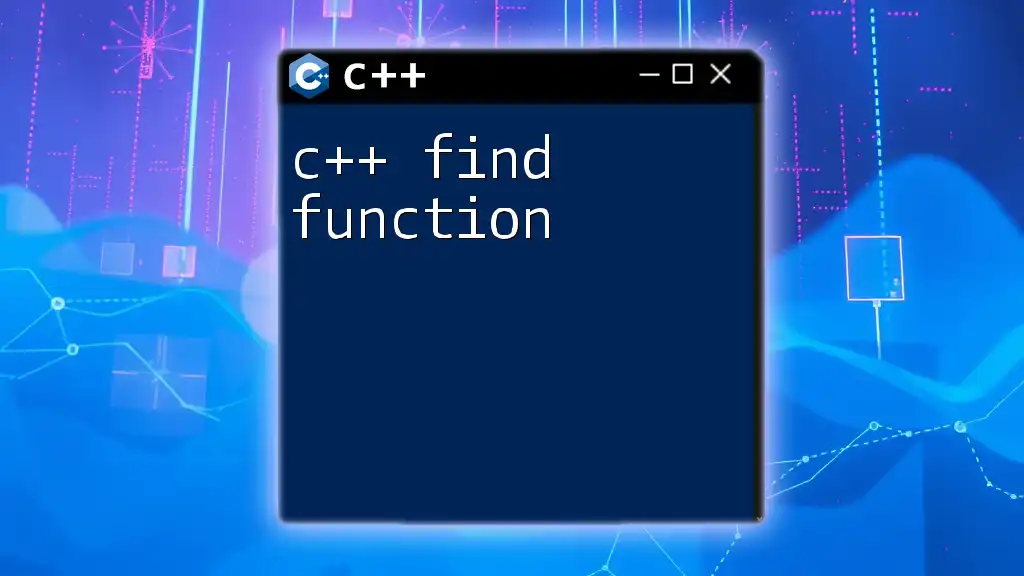
Performance Considerations
When employing the C++ maximum function, performance can significantly vary based on use case:
- Built-in Efficiency: The built-in `max` function is often optimized and should be your first choice unless there is a compelling reason to implement your own.
- Custom Function Scenario: In specific high-performance or memory-limited environments, a custom implementation may outperform the standard function by reducing overhead or making assumptions about data types.
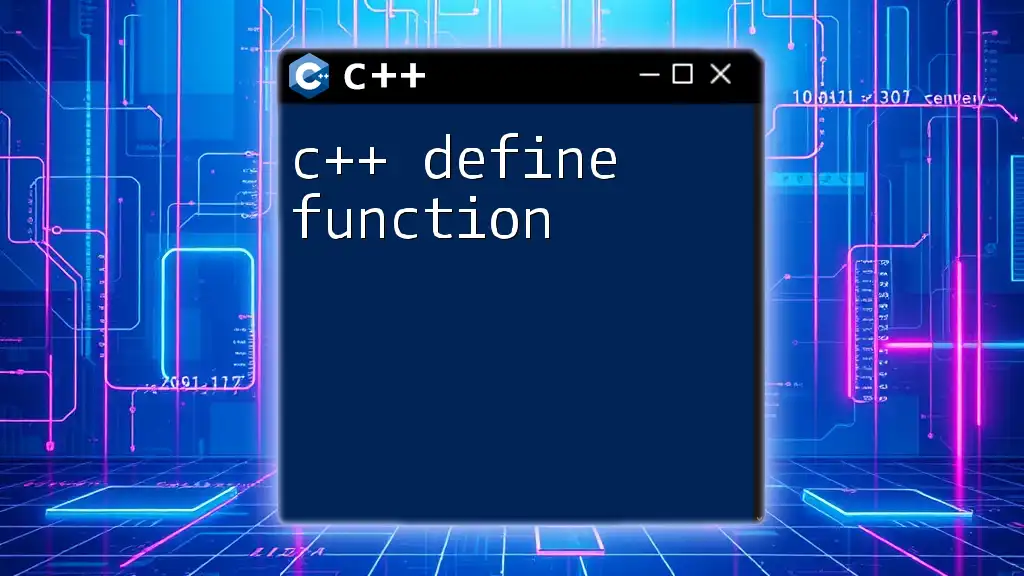
Conclusion
The C++ maximum function is an invaluable tool for any programmer’s toolkit. Understanding its uses, syntax, and potential pitfalls enhances code quality and efficiency. Practice using the `max` function in various scenarios to solidify your understanding and confidence in your C++ skills.
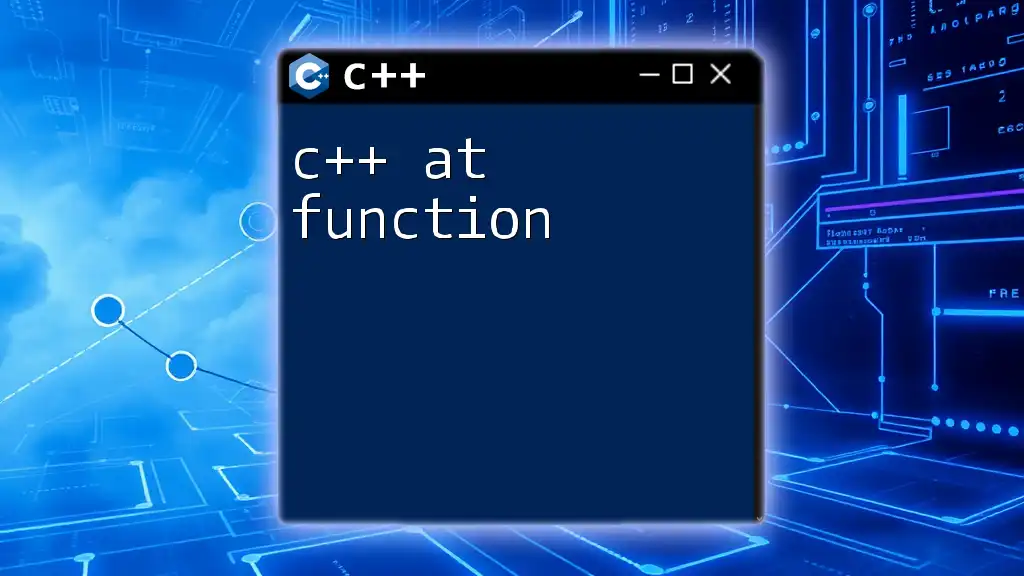
Additional Resources
For further reading on the C++ maximum function, you can explore the following:
- Official C++ documentation on `<algorithm>`
- Tutorials on general function overloading in C++
- Best practices for working with STL containers
Call to Action
We encourage you to share your experiences and challenges with the `max` function. Consider enrolling in our C++ programming course to advance your skills and tackle more complex topics in C++.