In C++, a mapping function is often implemented using the `std::transform` algorithm to apply a specified transformation to each element in a range, outputting the results to a new sequence.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int> mappedNumbers;
std::transform(numbers.begin(), numbers.end(), std::back_inserter(mappedNumbers), [](int n) { return n * n; });
for (int num : mappedNumbers) {
std::cout << num << " ";
}
return 0;
}
Understanding the C++ Map Data Structure
What is a Map in C++?
In C++, a map is a powerful associative container that stores elements as key-value pairs. Each key must be unique, and it is used to access the corresponding value efficiently. The underlying data structure for a map is typically a red-black tree, ensuring that the data is always sorted based on the keys and offering logarithmic time complexity for insertions, deletions, and lookups.
Advantages of Using Maps
Maps offer several advantages:
- Fast Retrieval: Thanks to the efficient data structure, retrieving values using keys has a time complexity of O(log n).
- Organized Structure: Maps maintain order based on keys, making it easy to iterate over the data.
- Real-World Applications: Maps are widely used in applications like databases (for indexing), phone books, or any scenario where quick lookups are needed.
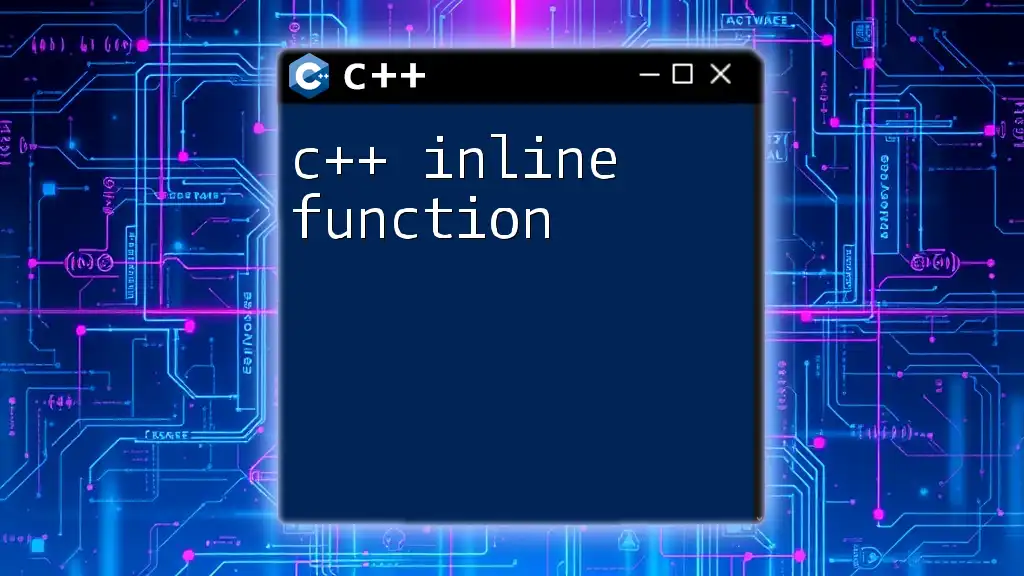
C++ Map Functionality
Overview of Map Functions in C++
C++ provides a variety of map methods that simplify the management of key-value pairs. Understanding these methods helps improve both the efficiency and readability of your code.
Common C++ Map Functions
Insertion Functions
One of the primary operations in working with maps is inserting elements. Here are the main methods to do so:
-
insert(): This method allows you to insert a pair into the map.
- Syntax: `map.insert(make_pair(key, value));`
- Code Example:
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; myMap.insert(std::make_pair(1, "Apple")); myMap.insert(std::make_pair(2, "Banana")); std::cout << "Inserted: " << myMap[1] << ", " << myMap[2] << std::endl; return 0; }
-
emplace(): This method constructs elements in-place, which can improve efficiency by avoiding unnecessary copying.
- Syntax: `map.emplace(key, value);`
- Code Example:
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; myMap.emplace(3, "Cherry"); // In-place construction std::cout << "Inserted: " << myMap[3] << std::endl; return 0; }
Accessing Elements
Accessing values in a map can be done through the following methods:
-
operator[]: This allows you to directly access the value associated with a specific key. If the key doesn’t exist, it will insert a default value.
- Code Example:
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; myMap[4] = "Date"; // Inserts with operator[] std::cout << "Accessed: " << myMap[4] << std::endl; return 0; }
-
at(): This method provides safe access, meaning if the key does not exist, it throws an exception.
- Code Example:
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; myMap[5] = "Elderberry"; try { std::cout << "Accessed: " << myMap.at(5) << std::endl; // Safe access std::cout << "Accessed: " << myMap.at(6) << std::endl; // Throws exception } catch (const std::out_of_range& e) { std::cout << "Exception occurred: " << e.what() << std::endl; } return 0; }
Deletion Functions
Removing elements from a map is straightforward with the following method:
- erase(): You can remove elements from the map using this method by key or by iterator.
- Syntax: `map.erase(key);`
- Code Example:
#include <iostream> #include <map> int main() { std::map<int, std::string> myMap; myMap[1] = "Apple"; myMap[2] = "Banana"; myMap.erase(1); // Removes the element with key 1 std::cout << "Remaining keys: "; for (const auto& pair : myMap) { std::cout << pair.first << " "; } return 0; }
Iterating Over Maps
Using Iterators
Iterators are a versatile way to traverse the elements within a map.
- begin() and end(): Using these methods allows you to access the first and last elements of the map, respectively.
- Code Example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
Using Standard Algorithms
You can also utilize standard algorithms to process maps. For example, `std::for_each` can be applied in conjunction with iterators.
- Code Example:
#include <iostream>
#include <map>
#include <algorithm>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
std::for_each(myMap.begin(), myMap.end(), [](const auto& pair) {
std::cout << pair.first << ": " << pair.second << std::endl;
});
return 0;
}
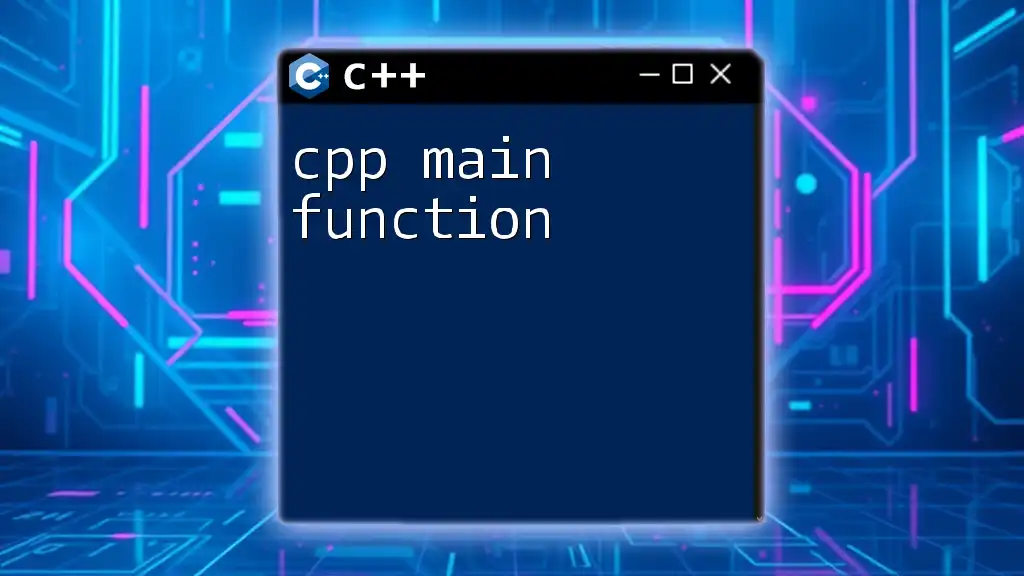
Practical Use of C++ Mapping Functions
Implementing a Simple Map Example
Consider a scenario where you want to create a simple phone book that maps names to phone numbers. Using a map simplifies the implementation significantly.
- Code Example:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, std::string> phoneBook;
// Inserting contacts
phoneBook.emplace("Alice", "123-456-7890");
phoneBook.emplace("Bob", "987-654-3210");
// Accessing a number
std::string name = "Alice";
std::cout << "Phone number for " << name << ": " << phoneBook.at(name) << std::endl;
return 0;
}
In the above code, we utilized the `emplace()` method to add contacts to our phone book and accessed a phone number using the `at()` method, demonstrating the practical advantages of C++ mapping functions.
Performance Considerations
Maps provide efficient data management with an average time complexity of O(log n) for most operations. When handling large datasets, this efficiency becomes crucial.
Keep in mind, as the number of elements increases, the performance of operations can vary depending on factors like key distribution and the overall design of your program. Profiling your application can help in understanding performance bottlenecks.
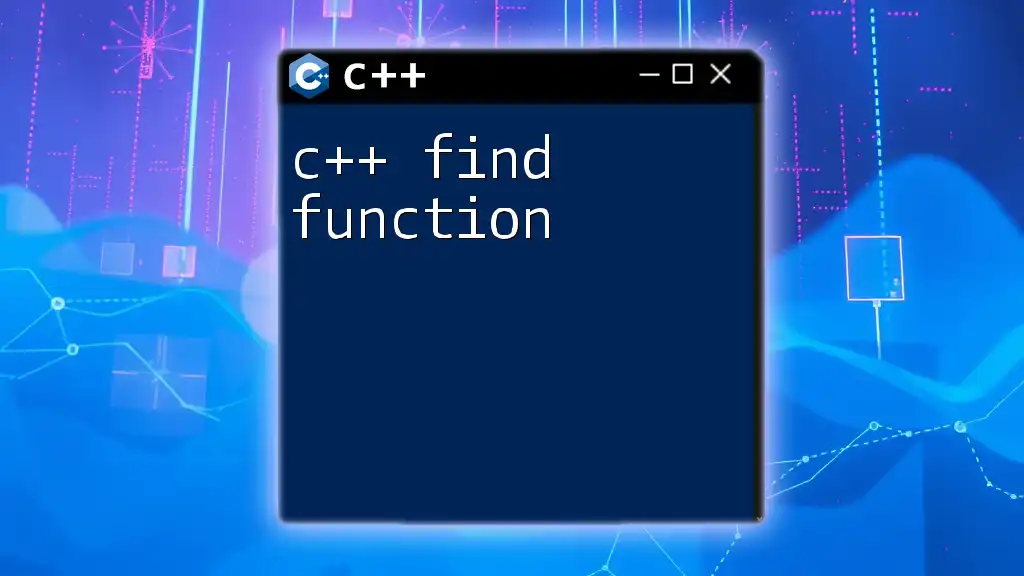
Advanced C++ Mapping Techniques
Custom Key Types
You can define custom data types as keys in a map. However, to use custom types effectively, you must provide an appropriate way to compare them. This is often done by overloading the comparison operators.
- Code Example:
#include <iostream>
#include <map>
#include <string>
struct Person {
std::string name;
int age;
// Overloading the < operator
bool operator<(const Person& other) const {
return name < other.name;
}
};
int main() {
std::map<Person, std::string> peopleMap;
peopleMap.emplace(Person{"Alice", 30}, "Engineer");
peopleMap.emplace(Person{"Bob", 25}, "Designer");
for (auto const& entry : peopleMap) {
std::cout << entry.first.name << " is a " << entry.second << std::endl;
}
return 0;
}
In this example, we've created a `Person` struct and implemented the `<` operator to allow our map to perform comparisons based on the name.
Using Maps with Functions
Maps can be passed to and returned from functions seamlessly. When passing maps, consider using references to avoid unnecessary copies.
- Code Example:
#include <iostream>
#include <map>
#include <string>
void printPhoneBook(const std::map<std::string, std::string>& phoneBook) {
for (const auto& entry : phoneBook) {
std::cout << entry.first << ": " << entry.second << std::endl;
}
}
int main() {
std::map<std::string, std::string> phoneBook = {
{"Alice", "123-456-7890"},
{"Bob", "987-654-3210"}
};
printPhoneBook(phoneBook);
return 0;
}
In this code snippet, we passed the phone book map to the `printPhoneBook` function by constant reference, ensuring that the function cannot modify the map and avoids unnecessary copying.
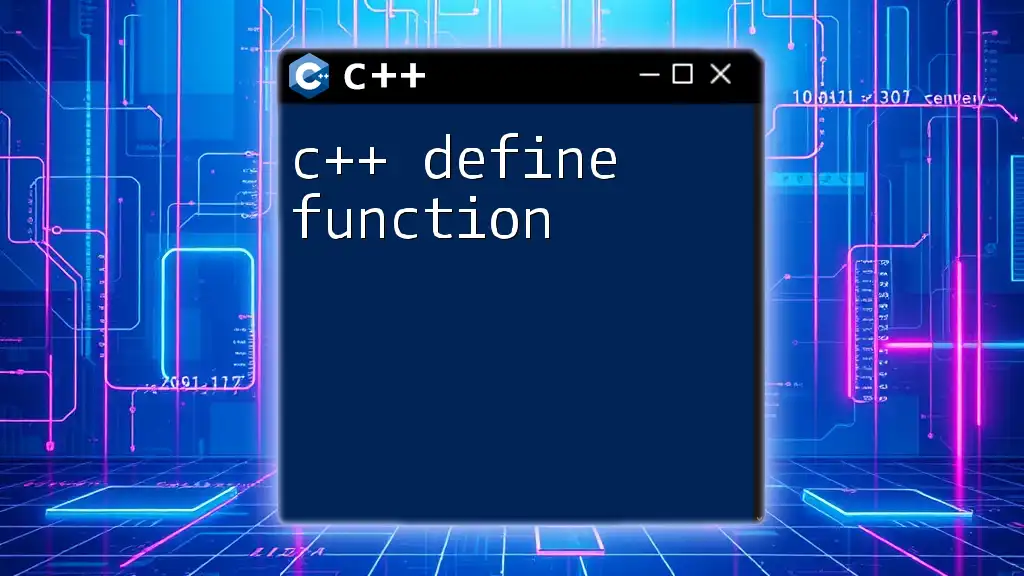
Conclusion
In this article, we explored the C++ mapping function, which plays a pivotal role in creating efficient and organized applications. Maps not only facilitate rapid retrieval of values but also enhance the clarity and maintainability of your code. As you grow your understanding of C++ and its mapping functions, consider the diverse applications you can implement in your projects.
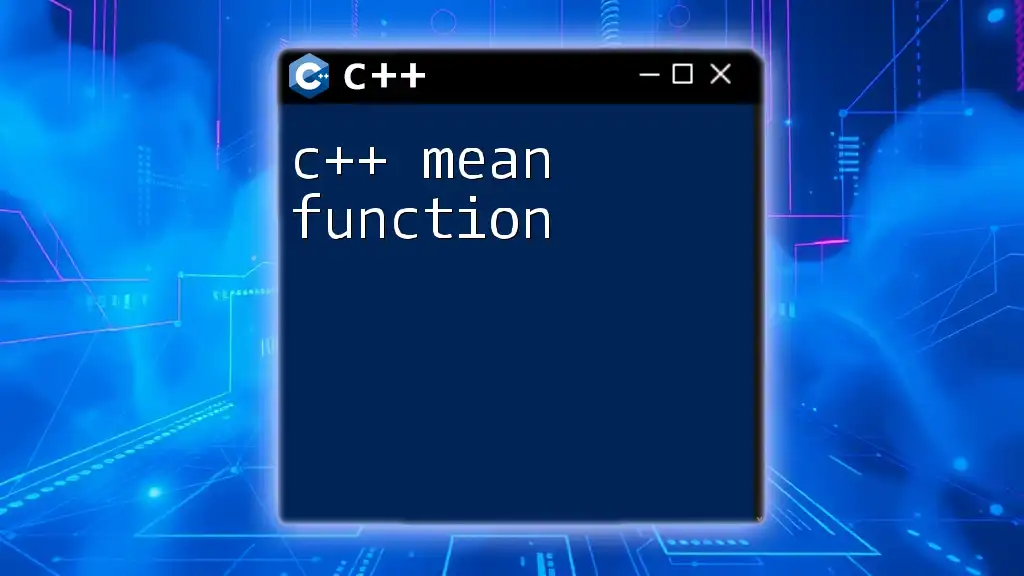
Additional Resources
To further your journey in mastering C++ and its mapping functions, consult the official C++ documentation for maps, explore recommended books, and participate in community forums that discuss C++ development and methodologies. Happy coding!