In C++, a factorial function calculates the product of all positive integers up to a given number, which can be implemented recursively or iteratively. Here's a simple example of a recursive factorial function:
#include <iostream>
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int num = 5;
std::cout << "Factorial of " << num << " is " << factorial(num) << std::endl;
return 0;
}
Understanding the Factorial Function
What is a Factorial?
A factorial, represented as `n!`, is a mathematical operation that multiplies a given integer `n` by every integer less than it down to 1. For instance, the factorial of 5 is calculated as follows:
5! = 5 × 4 × 3 × 2 × 1 = 120.
Important properties include:
- 0! is defined as 1. This is a special case that establishes a base case for many algorithms.
- The factorial function grows extremely fast. Even small input values lead to significantly large results. For example, 10! = 3,628,800.
Factorials have numerous applications in programming and mathematics, including permutations, combinations, and in algorithms that require calculating probabilities.
Factorial in C++
In C++, the factorial function is commonly used in computational tasks, particularly in combinatorial algorithms or mathematical computations where factorial values are necessary. Implementing a C++ factorial function allows programmers to perform complex calculations more efficiently and demonstrates the functionality of both recursion and iterative methodologies.
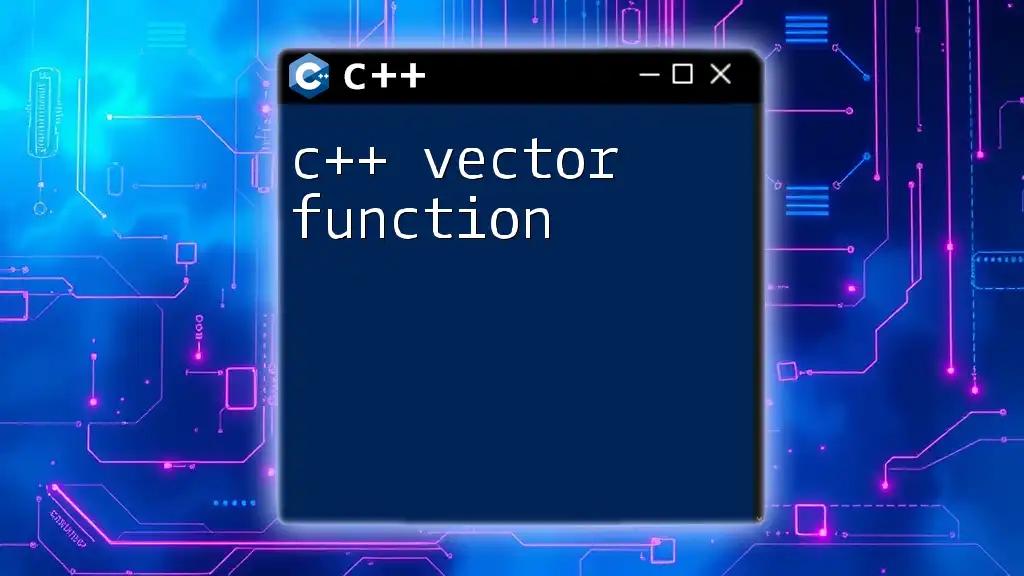
Implementing Factorial Function in C++
Recursive Method
Recursion involves a function calling itself to solve sub-problems, which can simplify coding for certain tasks like calculating factorials.
Here is a simple implementation of the C++ factorial function using recursion:
int factorial(int n) {
if (n == 0) return 1;
return n * factorial(n - 1);
}
In this code:
- The base case is `if (n == 0) return 1`. This effectively ends the recursive calls, as factorial of 0 is defined as 1.
- The recursive case calculates the factorial by multiplying `n` by the factorial of `n - 1`.
While recursion leads to elegant code, it has its disadvantages.
- Stack overflow can occur with large values of `n`, due to the limited depth of the stack in programming languages.
Iterative Method
An iterative approach uses loops instead of recursive calls, making it generally more space-efficient since it does not consume stack space.
Here’s a simple iterative implementation of the C++ factorial function:
int factorial(int n) {
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
In this code:
- We initialize a variable `result` to 1.
- A loop iterates from 2 to `n` and multiplies `result` by each integer `i`.
This method tends to be more efficient in terms of memory and is easier to understand for many programmers. However, it might be considered less elegant compared to the recursive version. Programmers often choose the iterative approach for performance-critical applications.
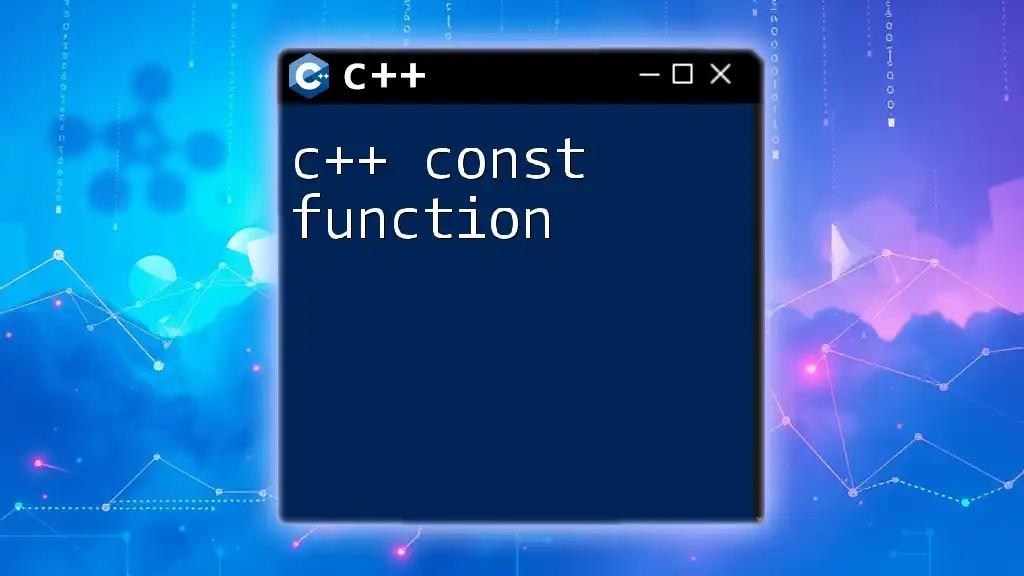
Performance Considerations
Time Complexity Analysis
Both the recursive and iterative approaches to the C++ factorial function have a time complexity of O(n). However, the recursive method involves additional overhead due to function call management, which can degrade performance when calculating larger factorials.
Space Complexity
The space complexity for the recursive method is O(n) because each recursive call uses stack space. In contrast, the iterative version has a space complexity of O(1) since it only uses a fixed amount of space regardless of the input size. This makes the iterative method more suitable for environments with limited memory.
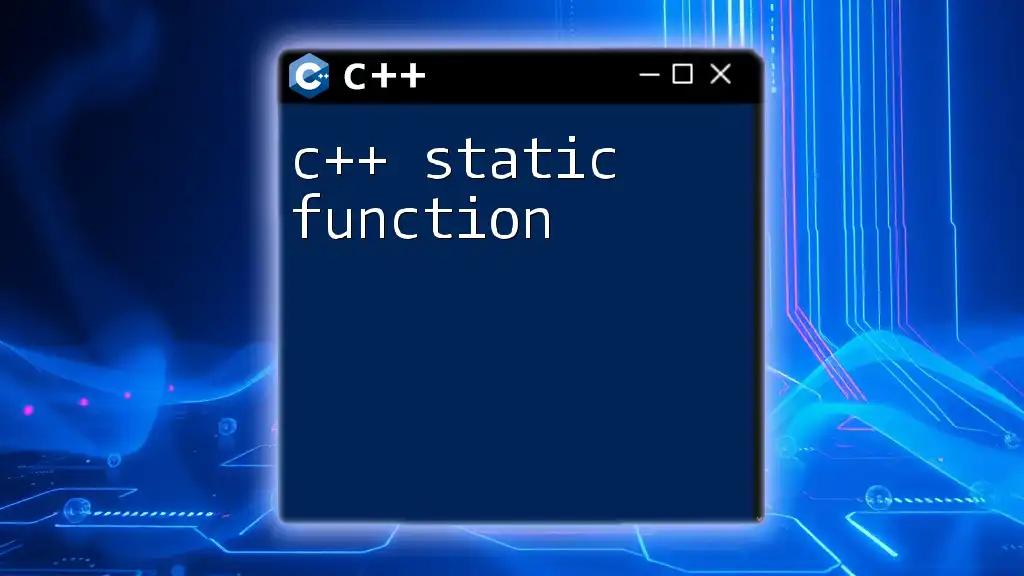
Handling Edge Cases
Validating Input
When implementing the C++ factorial function, it is crucial to ensure that input values are valid. Negative numbers do not have a factorial in traditional mathematics. Here’s how you might implement input validation:
int factorial(int n) {
if (n < 0) {
throw std::invalid_argument("Negative input not allowed.");
}
// Implementation
}
In this code, if a negative integer is passed, an exception is thrown, indicating an invalid argument. Proper exception handling helps maintain robustness within your code.
Calculating Factorial of Large Numbers
Calculating the factorial of large integers can lead to integer overflow with standard data types. C++ provides alternatives such as using `long long` or libraries like GMP (GNU Multiple Precision Arithmetic Library) for handling larger numbers. For example:
long long factorial(int n) {
long long result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
This example uses `long long` to allow for greater numerical capacity, although even this approach has limitations for very large `n` values.

Conclusion
The C++ factorial function serves as an excellent example of utilizing recursion and iteration in programming. Understanding the properties, implementation, and performance implications of factorials can provide a strong foundation in algorithm design.
As you dive deeper into C++ programming, consider experimenting with various implementations of factorials and using them in broader applications, such as combinatorial algorithms or probability calculations. This hands-on approach will solidify your understanding and skill in using C++ effectively.

Additional Resources
For further reading on factorial computation and advanced C++ programming, consider exploring resources that delve into recursion, efficient algorithm design, and mathematical libraries available in C++. Joining online courses and forums dedicated to C++ can also prove invaluable as you continue learning.
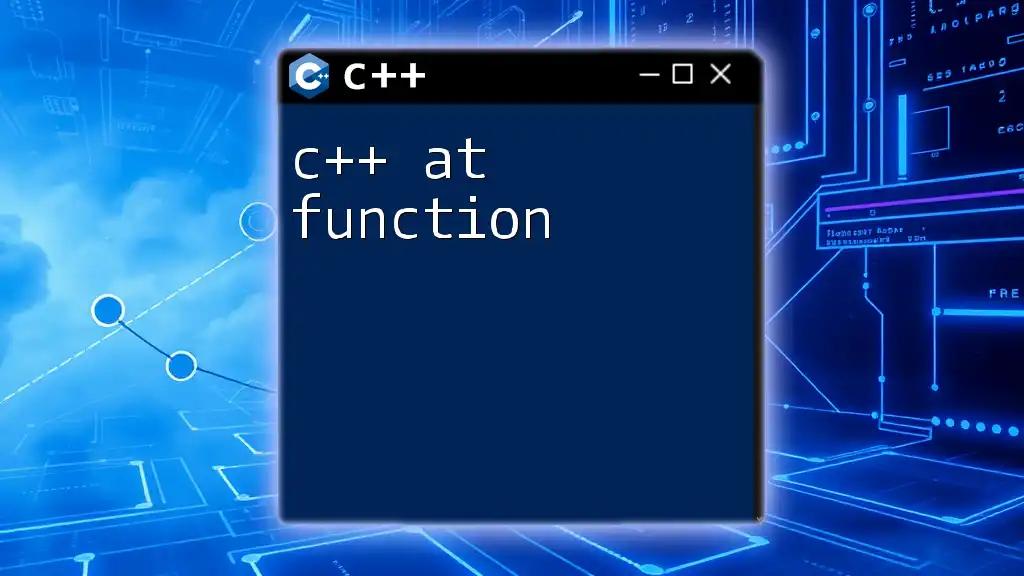
Call to Action
Have you implemented the C++ factorial function in your projects? Share your experiences and any challenges you faced. Subscribe to our insights and receive more tips on optimizing your C++ programming skills!