In C++, an "at function" typically refers to the `at()` member function of the `std::vector` or `std::map` containers, which provides bounds-checked access to elements by index or key.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::cout << "Element at index 2: " << vec.at(2) << std::endl; // Outputs: 30
return 0;
}
What is the `at` function?
The `at` function is a member function found in several containers of the Standard Template Library (STL) in C++. It provides a safe way to access elements at specified positions while ensuring bounds checking. Unlike the traditional index operator (`operator[]`), which does not perform bounds verification, the `at` function throws an exception when the accessed index is out of the valid range, thereby enhancing the robustness of code by preventing out-of-bounds errors.
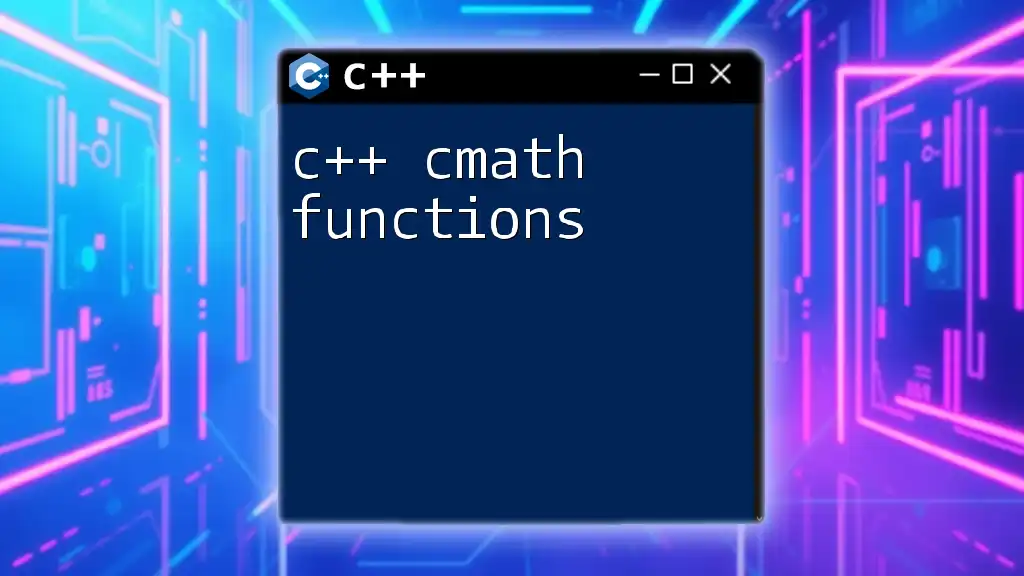
Overview of STL Containers
The Standard Template Library (STL) provides a rich set of template classes for data structures such as vectors, maps, sets, and others. These structures enable developers to manage collections of data easily. The `at` function is commonly used in the following STL containers:
- `std::vector`: A dynamic array that can grow and shrink in size.
- `std::map`: An associative container that stores elements in key-value pairs.
- `std::deque`, `std::array`, and others also support the `at` function.
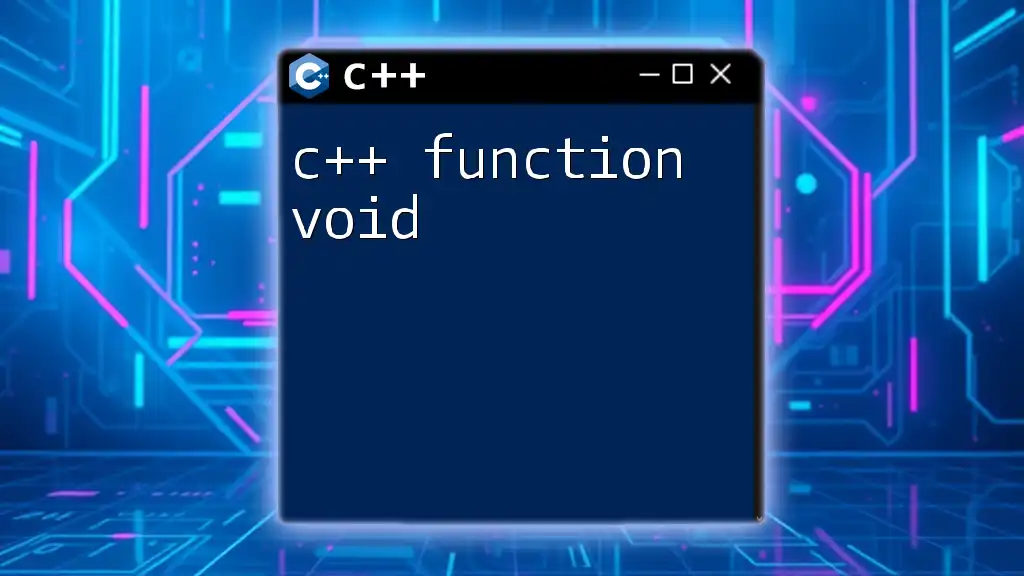
When to use the `at` function?
The `at` function should be preferred in scenarios where safety is a concern, particularly when working with user-generated input or unknown data sizes. Its built-in bounds checking is especially useful to avoid runtime errors and undefined behavior.
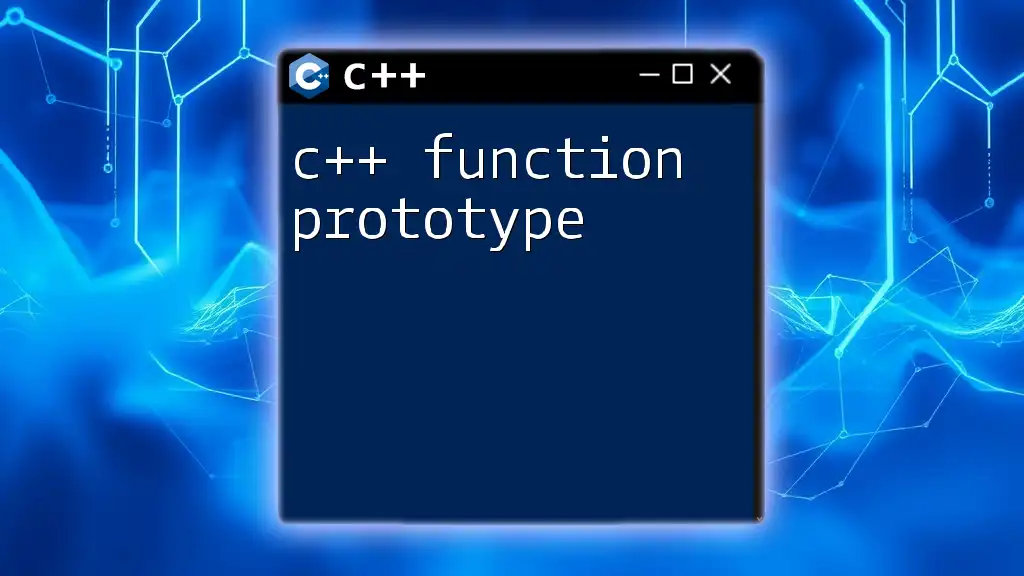
Using the `at` Function with Different Containers
Using `at` with `std::vector`
The `std::vector` is one of the most commonly used containers in C++. To access an element using the `at` function, the syntax is simple:
vector_name.at(index);
Example: Accessing Vector Elements
Here is an example showing how to use `at` with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
std::cout << "Element at index 2: " << numbers.at(2) << std::endl;
return 0;
}
In the code above, we define a vector of integers and use `at(2)` to fetch the element at index 2. The output will be `30`, demonstrating how easily you can access specific elements.
Using `at` with `std::map`
The `at` function is also utilized with associative containers like `std::map`. The syntax for accessing a value by its key is as follows:
map_name.at(key);
Example: Accessing Map Elements
Here's a practical example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ages = {{"Alice", 30}, {"Bob", 25}};
std::cout << "Alice's age: " << ages.at("Alice") << std::endl;
return 0;
}
In this example, we create a map that associates names with their ages. By calling `ages.at("Alice")`, we retrieve Alice's age, which outputs `30`.
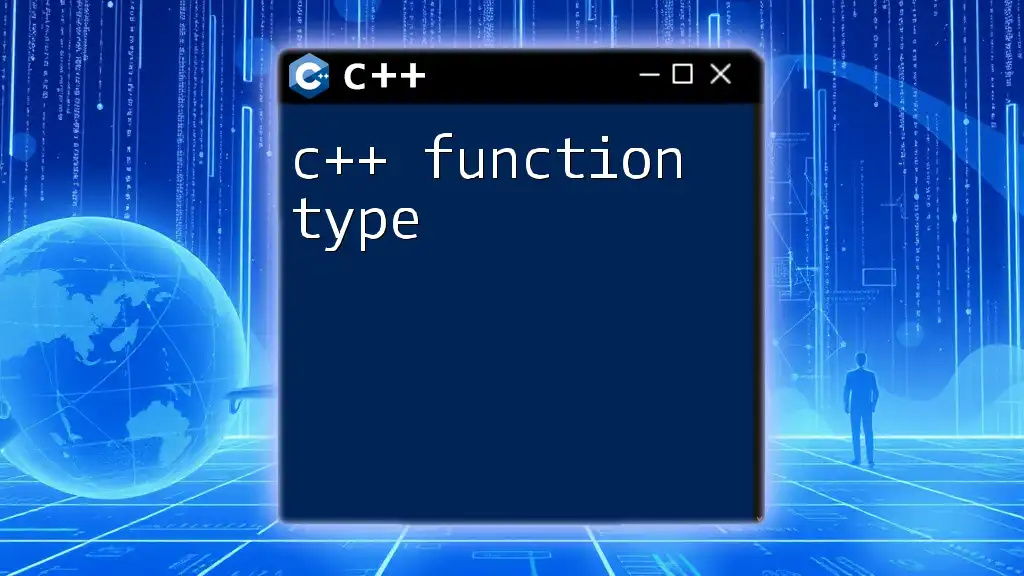
Benefits of Using the `at` Function
Safety and Error Handling
One of the most significant advantages of the `at` function is its bounds checking capability. If an attempt is made to access an element outside the valid index, `at` throws a `std::out_of_range` exception.
This behavior can prevent common pitfalls in data access that can lead to undefined behavior, making your code cleaner and safer.
Comparison with `operator[]`
While `operator[]` allows direct access to elements in containers, it does not perform bounds checking, which can result in runtime errors if an out-of-bounds index is accessed. For instance:
std::vector<int> vec = {1, 2, 3};
std::cout << vec[5]; // This can cause undefined behavior
In contrast, using `at` would generate an exception in the above scenario, allowing developers to catch it and handle it appropriately:
try {
std::cout << vec.at(5);
} catch (const std::out_of_range& e) {
std::cout << "Out of range error: " << e.what();
}
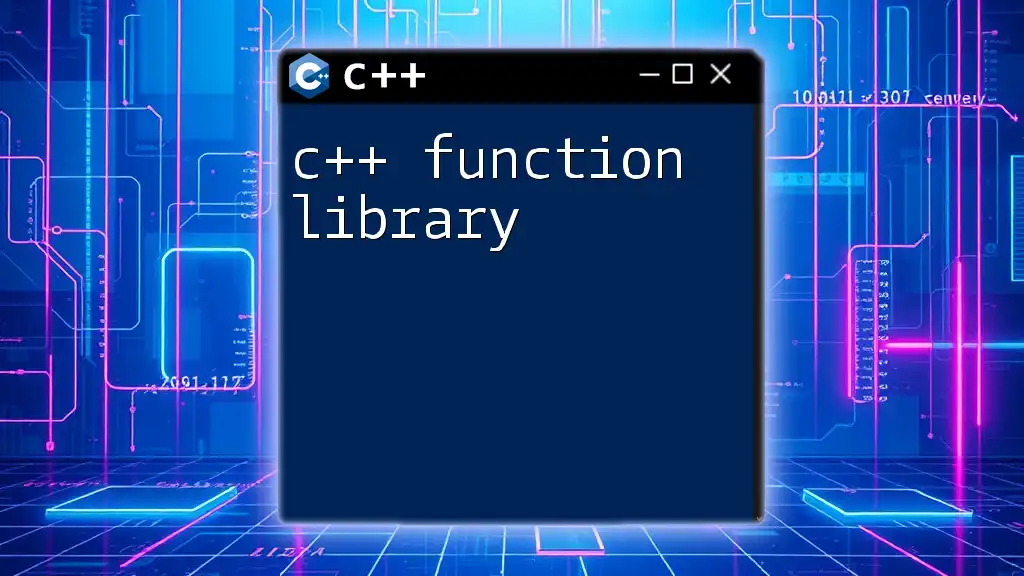
Best Practices for Using the `at` Function
When to Prefer `at`
The `at` function is particularly useful in applications that require robustness and reliability. Consider using it in:
- User input scenarios: When dealing with dynamic or user-generated data where out-of-bounds access is more likely.
- Debugging: During the development phase, to catch errors and exceptions gracefully.
Performance Considerations
Though `at` provides essential safety features, it has a slight performance overhead due to bounds checking. In scenarios where performance is critical and the data integrity is guaranteed, using `operator[]` might yield better results. Always weigh the need for safety against performance requirements.
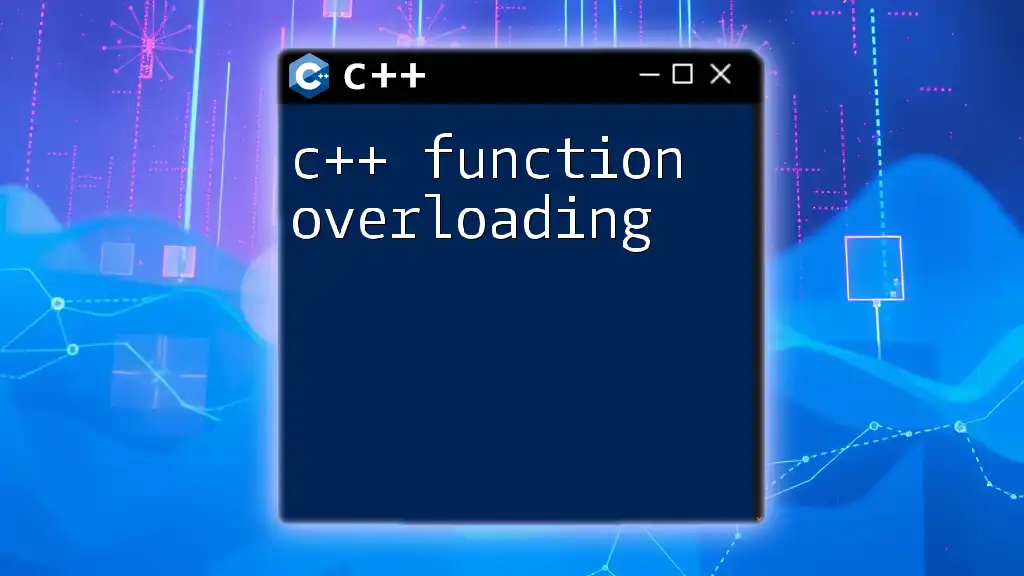
Common Mistakes and Troubleshooting
Misusing the `at` function
A common mistake occurs when developers mistakenly believe that `at()` and `operator[]` behave identically. Always ensure you are aware of the bounds checking capabilities of `at` as this can lead to confusion and errors.
Handling Exceptions Gracefully
Proper handling of exceptions raised by `at` is crucial for maintaining a smooth user experience.
When implementing, use `try-catch` blocks as shown earlier. This not only prevents crashes but also allows you to provide meaningful feedback in case of erroneous input.
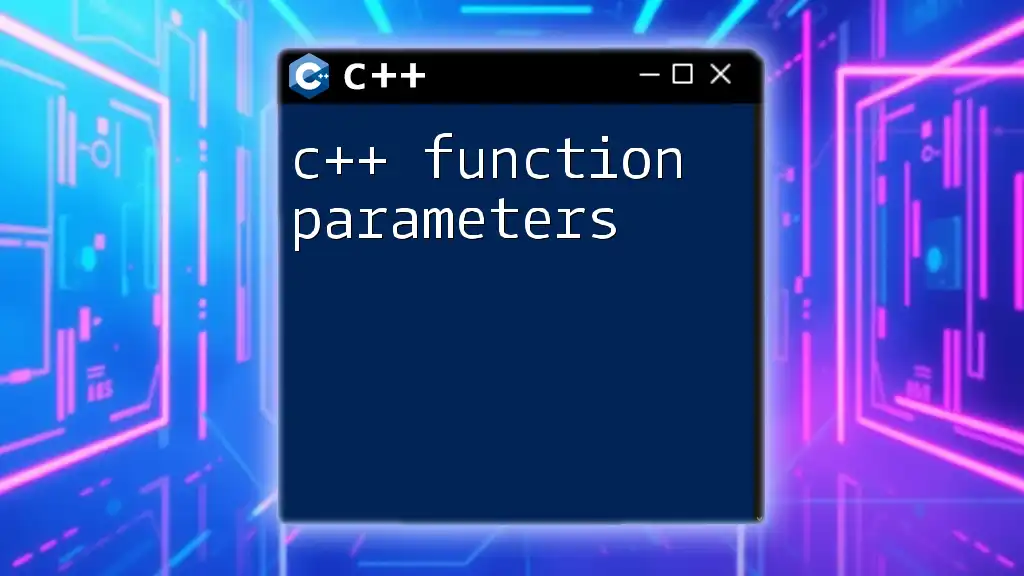
Conclusion
The C++ `at` function is a powerful tool for safely accessing elements in standard library containers. By understanding its benefits, appropriate use cases, and careful exception handling, developers can enhance code stability while minimizing the risk of errors.
Encouragement to practice using the `at` function will help solidify your understanding of STL containers, empowering you to incorporate these safe access methods into your C++ coding repertoire.
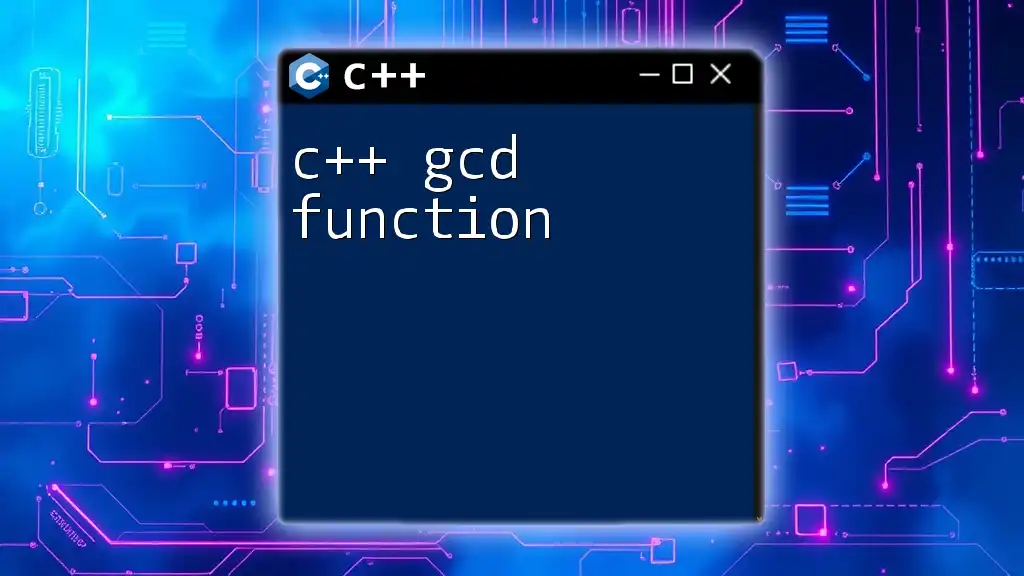
Additional Resources
To further enhance your understanding of the `at` function in C++, explore the following resources:
- Official C++ STL Documentation
- Online tutorials and courses focused on C++ programming
- Community platforms like Stack Overflow or C++ subreddits for additional help and insights