In C++, a `void` function is a type of function that does not return any value, and it's commonly used to perform a specific task or operation without sending back data. Here’s a simple code snippet demonstrating a `void` function:
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet(); // Calls the void function
return 0;
}
What is a Void Function in C++?
A void function in C++ is a specific type of function that does not return any value. Instead, it performs a particular task and then completes its execution. Understanding the concept of void functions is crucial for effective programming, as they enable better organization of code, allowing developers to break down complex tasks into manageable segments without needing to return values.
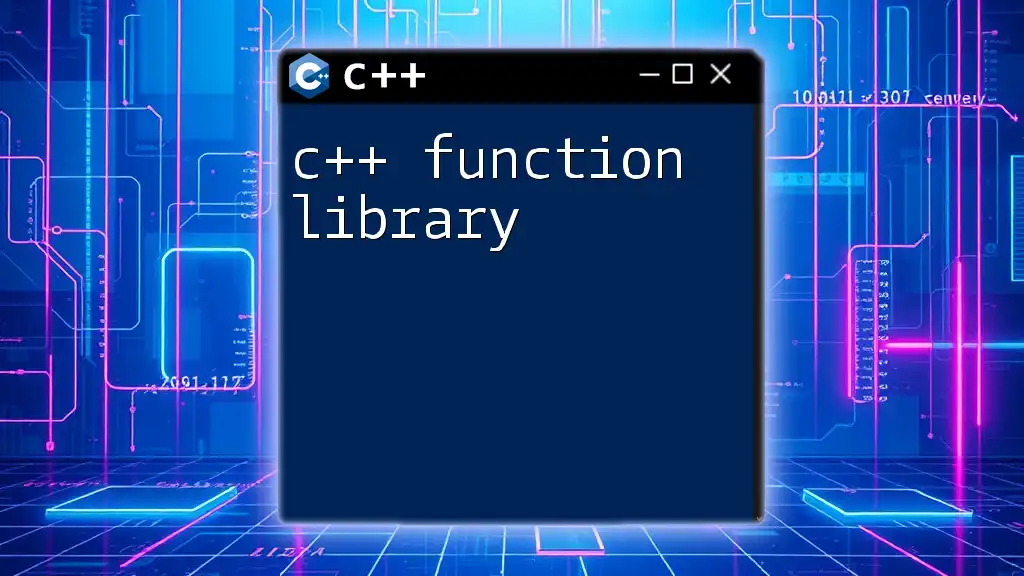
Understanding the Purpose of Void Functions
Void functions serve important purposes in programming:
- Modular Code: By segmenting tasks into distinct functions, code becomes more organized and easier to read.
- Side Effects: Even though void functions do not return a value, they can still produce side effects, such as modifying global variables or displaying output to the console.
- Clarity: Using void functions when a return value is unnecessary helps make the intent of the code clearer.
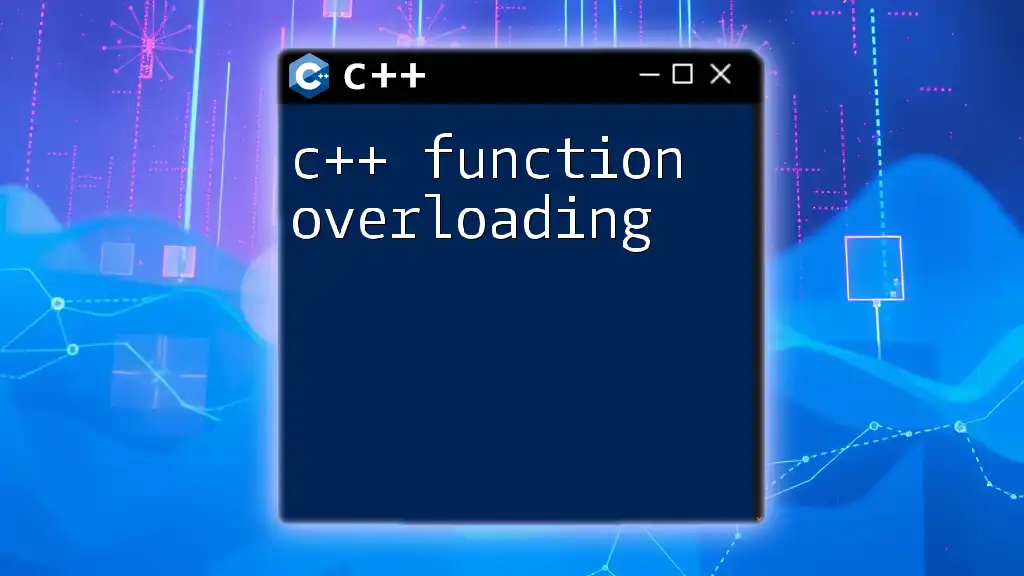
Defining a Void Function in C++
Syntax of a C++ Void Function
The syntax for declaring a void function is straightforward. Here is the basic structure:
void functionName() {
// Code to execute
}
Example of a Void Function
Let’s create a simple void function that prints a message to the console. This will help illustrate how void functions work in practice.
void printMessage() {
std::cout << "Hello, World!" << std::endl;
}
In this example, when `printMessage()` is called, the program executes the code within the function, printing "Hello, World!" to the console.
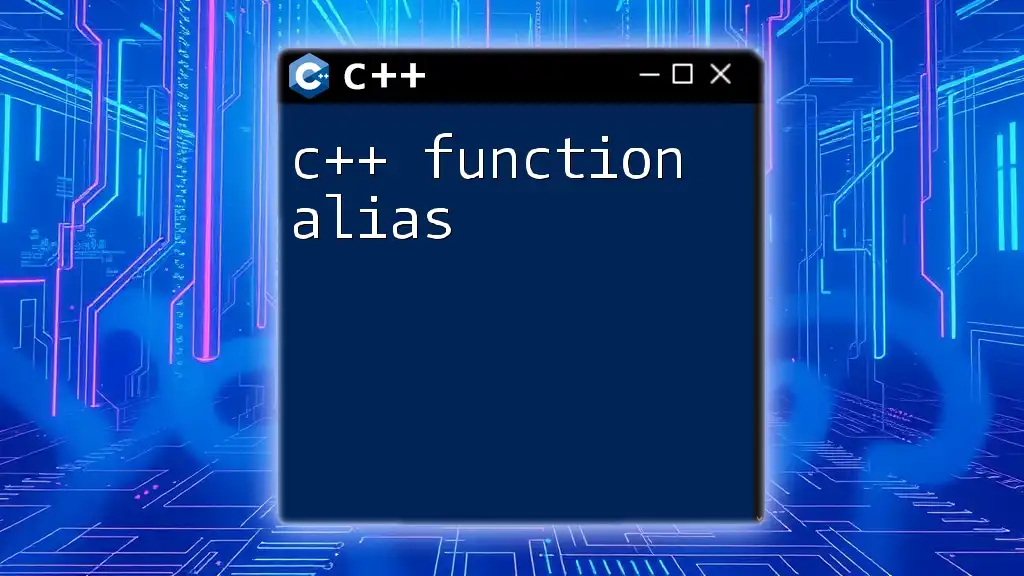
Calling a Void Function
How to Call a Void Function
To invoke a void function, simply call its name followed by parentheses. This is typically done within the `main()` function or any other function.
Here’s an example demonstrating how to call the `printMessage()` function:
int main() {
printMessage(); // Calling the void function
return 0;
}
Best Practices for Calling Void Functions
Clarity is essential in programming. It is important to follow good naming conventions for void functions to convey their purpose effectively. For example, a function that calculates a sum should be named `calculateSum()` rather than something vague like `doTask()`.
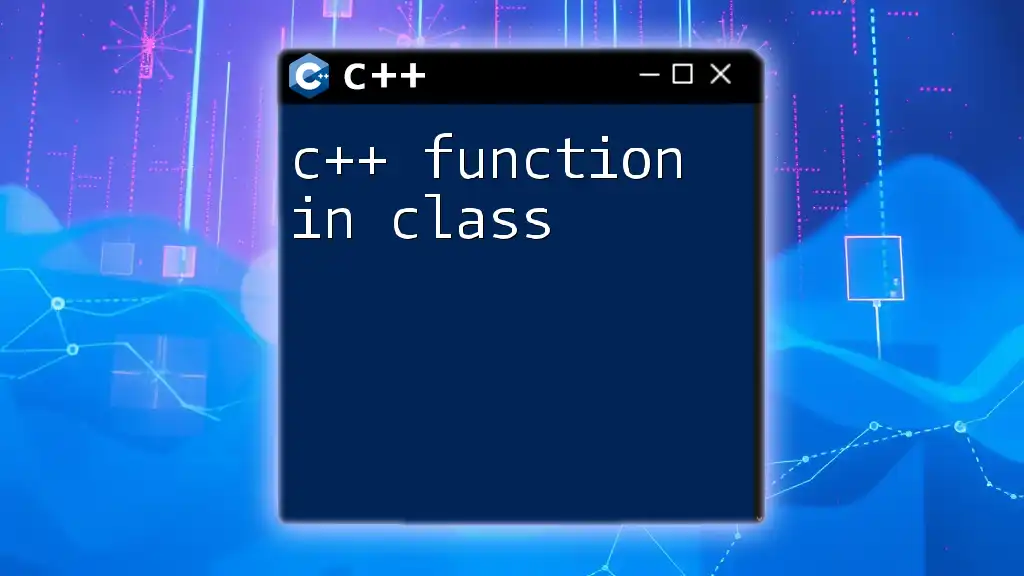
Parameters in C++ Void Functions
Passing Parameters to Void Functions
Void functions can accept parameters, enabling them to perform actions based on input values. Here’s how you can define a void function that takes parameters:
void displaySum(int a, int b) {
std::cout << "Sum: " << (a + b) << std::endl;
}
Calling a Void Function with Parameters
When you call a void function that accepts parameters, you need to pass the appropriate arguments. Here’s how it looks:
int main() {
displaySum(5, 10); // Passing arguments to the void function
return 0;
}
In this example, `displaySum(5, 10);` will compute the sum of 5 and 10, resulting in "Sum: 15" being printed to the console.
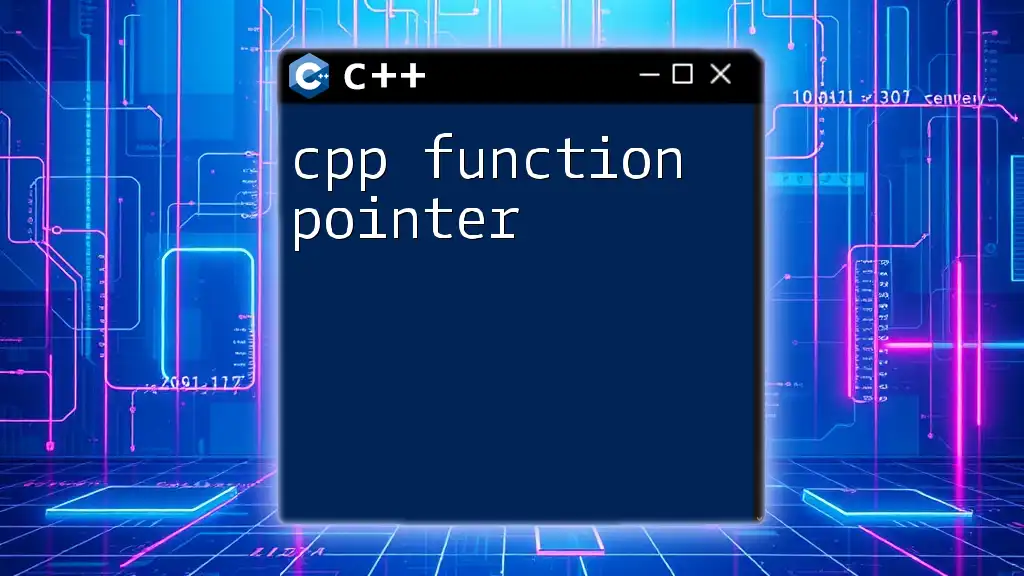
Return Statements in Void Functions
Understanding Return Behavior
One of the defining characteristics of a void function is that it does not return a value. When you need to terminate a function early or exit without performing further actions, you can use a `return;` statement.
Example of Early Return in a Void Function
Consider the following example where we check if a number is negative:
void checkNumber(int num) {
if(num < 0) {
std::cout << "Negative number" << std::endl;
return; // Exit the function early
}
std::cout << "Positive number" << std::endl;
}
In this case, if `num` is negative, the function outputs "Negative number" and terminates. If it's positive, the function continues to the next print statement.
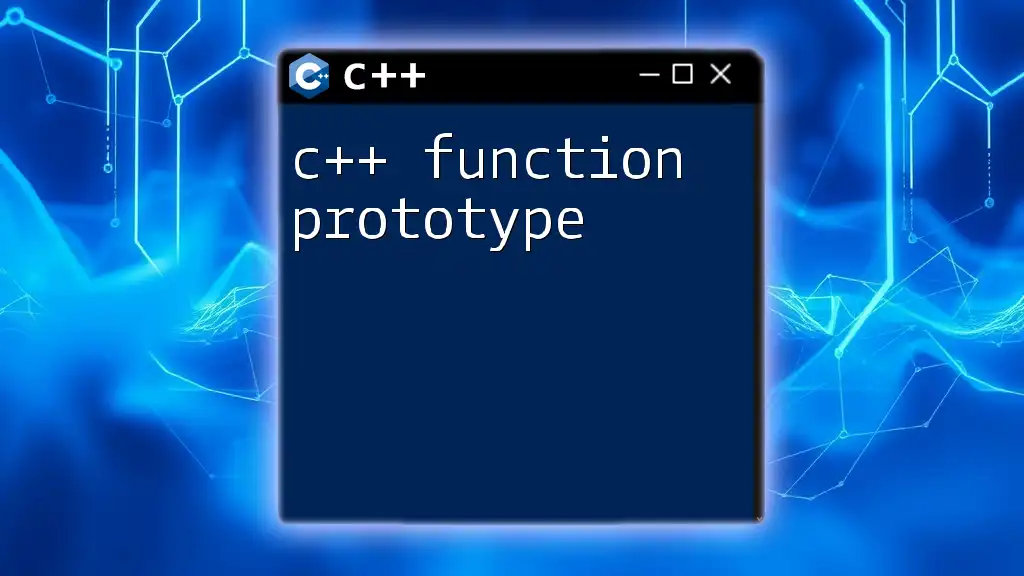
Advantages of Using C++ Void Functions
Enhanced Code Clarity and Maintainability
Using void functions can significantly improve code clarity, making it easier for you and others to understand how the code operates. By using intuitive naming and keeping functions focused on specific tasks, your codebase will be more maintainable.
No Overhead of Return Values
Void functions do not carry the overhead of returning values. This can enhance performance, especially in scenarios involving frequently called functions, minimizing the amount of unnecessary data processing.
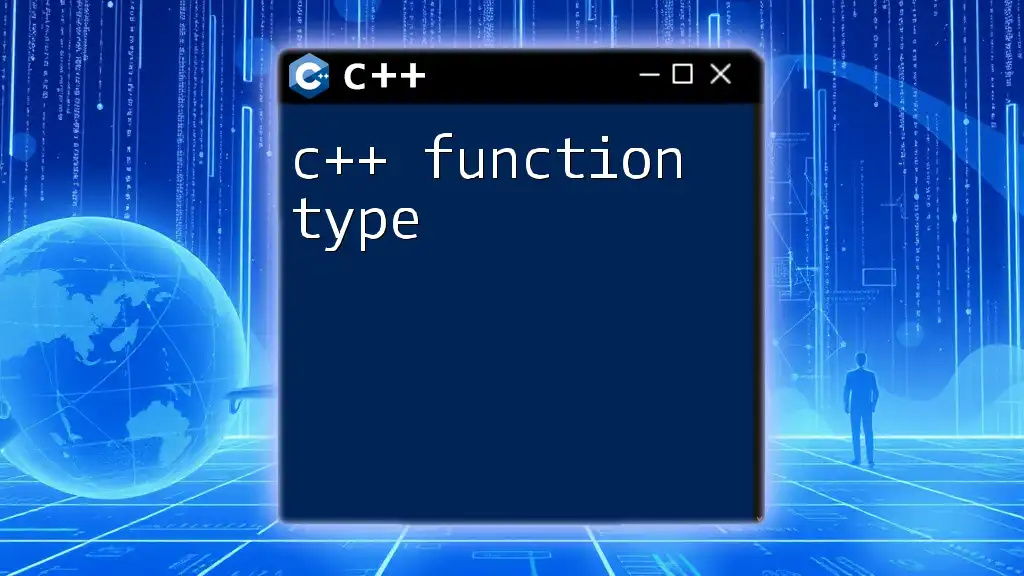
Common Mistakes with Void Functions
Forgetting to Call a Void Function
One common mistake programmers make is forgetting to call a void function. To ensure a function executes, always confirm that it is invoked correctly in your code. Debugging is often required when functions are defined but not called, as this may lead to confusion believing the functionality is present when it is not.
Misusing Parameters
When using parameters in void functions, it's vital to ensure they are passed correctly. Common mistakes include mismatched types or not providing all required arguments, which can lead to compilation errors or unexpected behavior. Always validate input and utilize default values where applicable to enhance function flexibility.
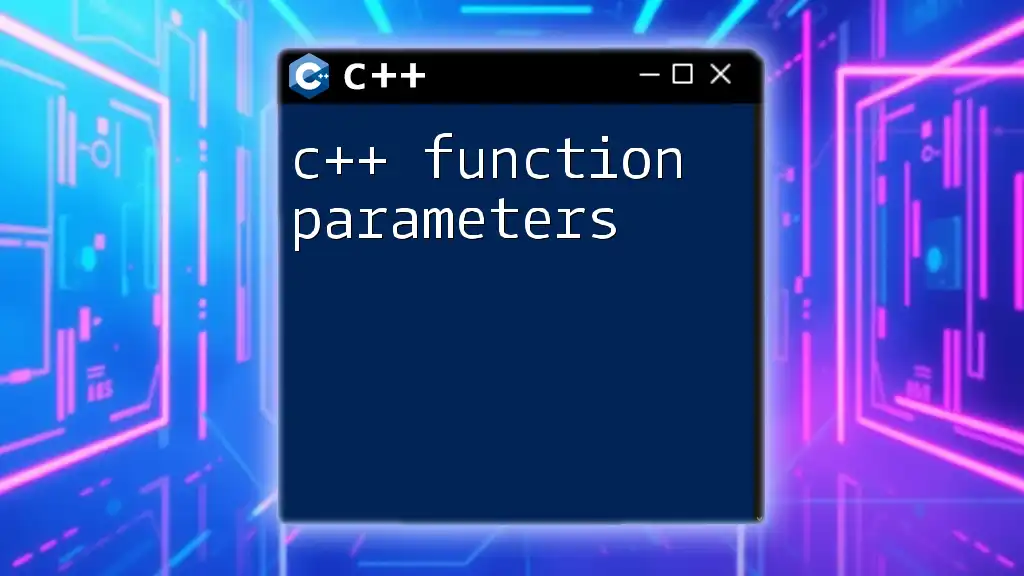
Conclusion
Void functions in C++ are vital components that allow programmers to structure their code more effectively, encouraging clarity and modularity. By breaking tasks into separate functions, you can enhance maintainability while eliminating the need for verbose return handling. Understanding and utilizing void functions will aid in your journey to becoming a proficient C++ developer. Embrace the practice of creating and calling void functions, and explore their various applications in your programming endeavors.