In C++, a function is a block of code that performs a specific task, can take inputs, and may return an output, enabling code reusability and organization. Here's a simple example of a function that adds two integers:
int add(int a, int b) {
return a + b;
}
Understanding the Basics of CPP Functions
What is a Function?
A function in C++ is a block of code designed to perform a specific task. It is one of the fundamental building blocks of any program, providing a way to encapsulate and reuse code. Functions help maintain a clean and organized structure in a program, allowing for modularity and easier debugging. Each function can take inputs, perform computations, and return outputs.
Syntax of a CPP Function
The syntax for a function in C++ follows a specific structure that consists of several key components:
return_type function_name(parameters) {
// function body
}
- Return Type: Defines what type of value the function will return (e.g., `int`, `float`, `void`).
- Function Name: A unique identifier for the function that is used to call it.
- Parameters: A list of inputs that the function can accept, defined within parentheses.
- Function Body: The block of code that executes when the function is called, enclosed in curly braces.
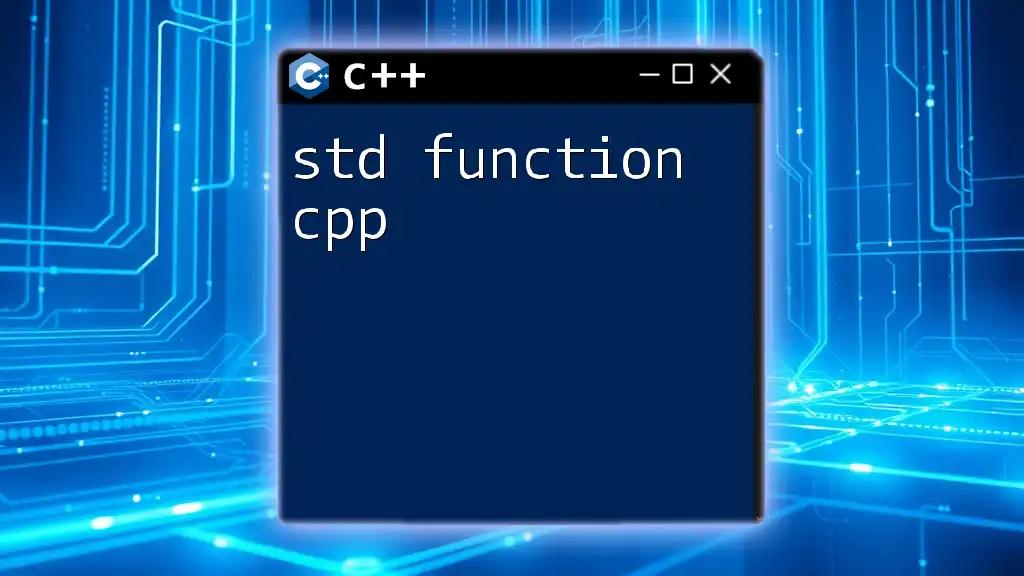
Types of CPP Functions
Built-in Functions
C++ offers numerous built-in functions for various tasks, such as mathematical operations, character manipulation, and data handling. Understanding these functions can help simplify coding and reduce lines of code.
For instance, the `sqrt` function is used to calculate the square root of a number:
#include <iostream>
#include <cmath> // Required for sqrt function
using namespace std;
int main() {
cout << sqrt(16); // Outputs 4
return 0;
}
In this example, the code imports the `<cmath>` library, which includes mathematical functions, allowing access to `sqrt`.
User-defined Functions
User-defined functions allow programmers to create custom functions tailored to specific needs.
Function Declaration and Definition
To use a function, you must first declare it. The function declaration specifies the return type, name, and parameters, while the function definition provides the code that executes when the function is called.
Function Overloading
Function overloading is a powerful feature in C++ that allows creating multiple functions with the same name but different parameters. This enhances code readability and usability.
#include <iostream>
using namespace std;
void display(int i) {
cout << "Integer: " << i << endl;
}
void display(double d) {
cout << "Double: " << d << endl;
}
In this example, both `display` functions can be called with different types of arguments, demonstrating how function overloading can streamline functionality.
Inline Functions
Inline functions suggest to the compiler to insert the function's body directly into the code when called. This can improve performance, especially for small, frequently called functions.
inline int square(int x) {
return x * x;
}
Here, the `square` function computes the square of an integer. The `inline` keyword hints at performance optimization, reducing the overhead of function calls.
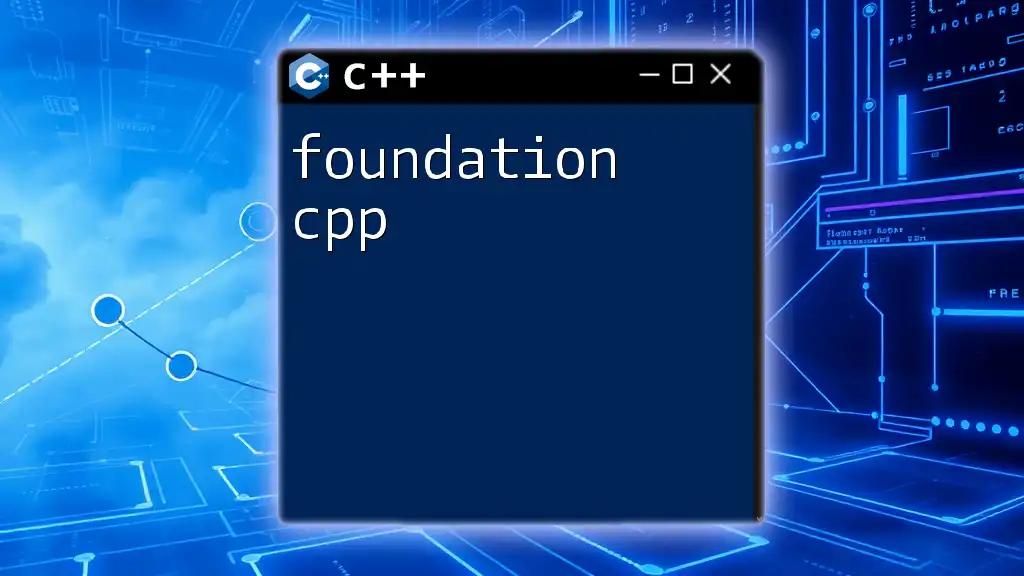
Parameters and Return Types
Passing Parameters to Functions
Pass by Value
When parameters are passed by value, a copy of the variable is provided to the function. Any modifications to the parameter inside the function do not affect the original variable.
void passByValue(int x) {
x = 100; // Changing x does not affect the original variable
}
Pass by Reference
In contrast, passing parameters by reference allows the function to modify the original variable within the caller's scope. This is done using the `&` operator.
void passByReference(int &x) {
x = 100; // Changing x will affect the original variable
}
This approach is useful when working with large objects, as it avoids copying and enhances performance.
Return Types in Functions
Functions can return values, which enable them to produce outputs that can be used elsewhere in your program. The return type must match the data type of the returned value.
int add(int a, int b) {
return a + b; // function returns the sum
}
Here, the `add` function takes two integers as parameters and returns their sum.
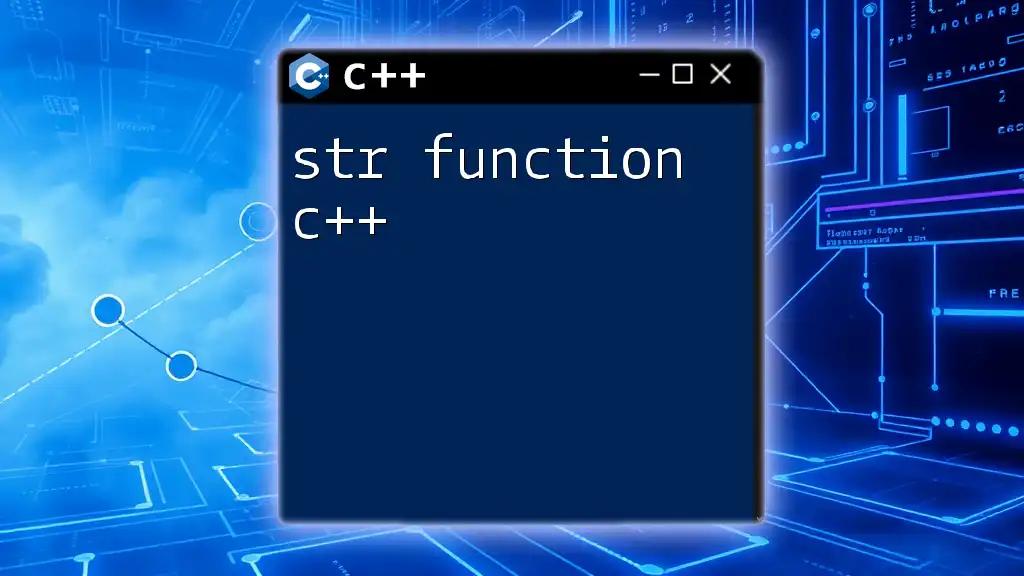
Scope and Lifetime of Functions
Local vs Global Functions
The scope of a function determines where it can be accessed in the program. Local functions are defined within another function and are not visible outside of it, while global functions can be accessed from any part of the program.
int globalVar = 10; // Global variable
void testFunction() {
int localVar = 5; // Local variable
cout << "Global variable: " << globalVar << endl;
cout << "Local variable: " << localVar << endl;
}
In this example, `globalVar` is accessible from anywhere in the program, while `localVar` can only be accessed within `testFunction`.
Static and Dynamic Functions
Static functions have a lifetime limited to the duration of the program execution, while dynamic functions can be created and destroyed during runtime. Understanding their behaviors is crucial for memory management and program efficiency.
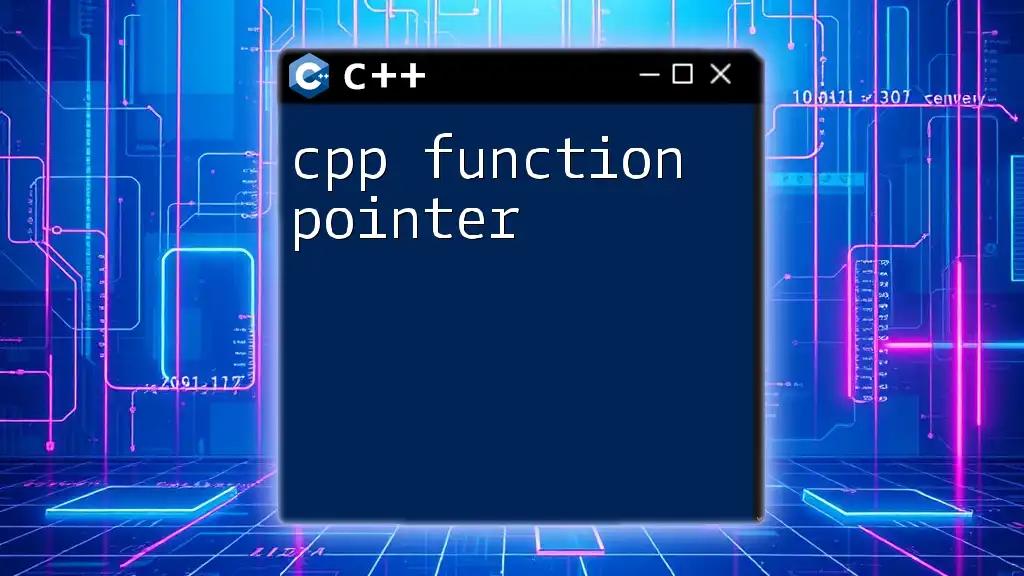
Best Practices for Writing Functions in CPP
Keep Functions Short and Concise
It’s important to design functions that perform a single task or related tasks. This enhances maintainability, making it easier for others (or yourself in the future) to understand and use the function.
Commenting Code Effectively
Effective comments are essential for clear code. Good comments explain the purpose of the function, parameters, and any intricate logic that may not be immediately clear.
// Function to calculate the factorial of a number
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
Testing Functions
Rigorous testing is vital to ensure that functions behave as expected. Utilizing assertions can help validate outcomes during development.
#include <cassert>
int multiply(int a, int b) {
return a * b; // function returns the product
}
int main() {
assert(multiply(2, 3) == 6); // Test case to check correctness
}
In this example, the `assert` function verifies that `multiply(2, 3)` produces the expected result, providing a straightforward method to confirm function correctness.

Conclusion
Functions are critical in C++ programming, promoting reusability, clarity, and modularity. By mastering the art of writing and using functions effectively, you can improve your coding skills significantly. Embrace practice through writing various functions and don’t shy away from exploring more complex topics. The journey to becoming proficient in C++ starts with a solid understanding of functions. Keep learning, and enjoy the programming journey!
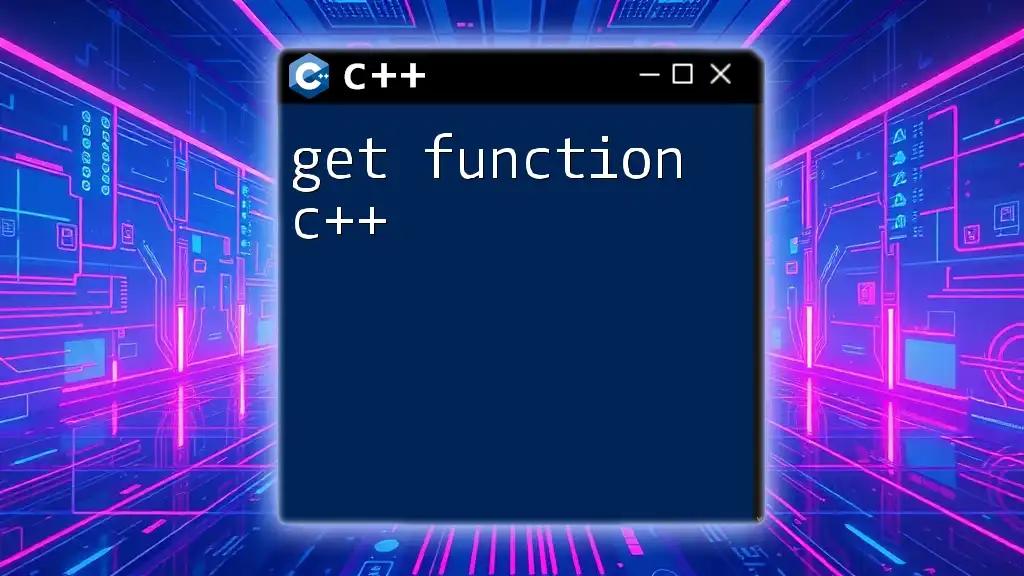
Further Reading and Resources
To deepen your understanding of C++ functions, explore recommended books such as “C++ Primer” or “Effective C++.” Online platforms like Codecademy and LeetCode offer interactive coding exercises that reinforce your skills. Pursue these resources to continue your education in the exciting world of C++ programming!