A function prototype in C++ is a declaration of a function that specifies its name, return type, and parameters, allowing the function to be called before its actual definition.
Here’s an example of a function prototype:
int add(int a, int b); // Function prototype
What is a Function Prototype in C++?
Definition
A function prototype in C++ is essentially a declaration of a function that specifies the function's name, its return type, and its parameters (if any), without providing the actual body of the function. This prototype informs the compiler about the function's type signature, allowing it to ensure that calls to the function are made with the correct arguments and return types.
Importance of Function Prototypes
- Enhancing Code Readability: Function prototypes make it clear what functions are available in a program without having to delve into their definitions.
- Allowing for Function Declaration Before Invocation: Prototypes enable you to call functions before they are defined, which is particularly useful in organizing code logically.
- Facilitating Function Overloading: With prototypes, C++ can distinguish between different versions of functions that share the same name but have different parameter types or numbers.
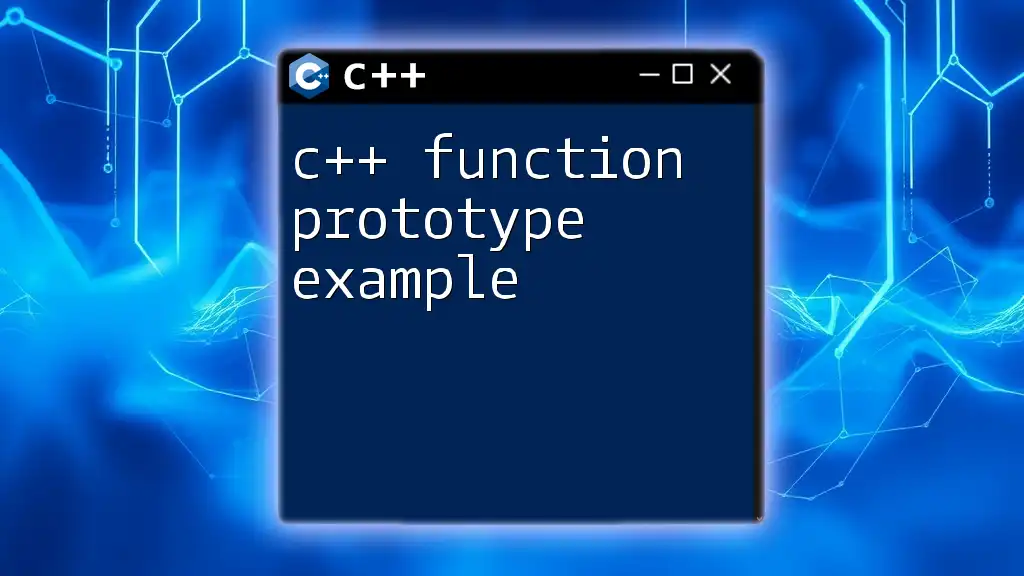
Syntax of a Function Prototype in C++
Basic Syntax Structure
The syntax for a function prototype consists of the following components: the return type, the function name, and the parameter types and names (optional).
Here is an example of a simple function prototype:
int add(int a, int b);
Detailed Syntax Breakdown
-
Return Type: This indicates what type of value the function will return. If the function does not return a value, the return type should be `void`. For instance:
void printMessage();
-
Function Name: The chosen name should be descriptive of the function's purpose, following C++ naming conventions.
-
Parameters: Parameters are the inputs to the function. Each parameter has a type and a name. The name can be omitted in the prototype, but it's often included for clarity. For example, a prototype with multiple parameters could look like this:
void displayInfo(const std::string &name, int age);
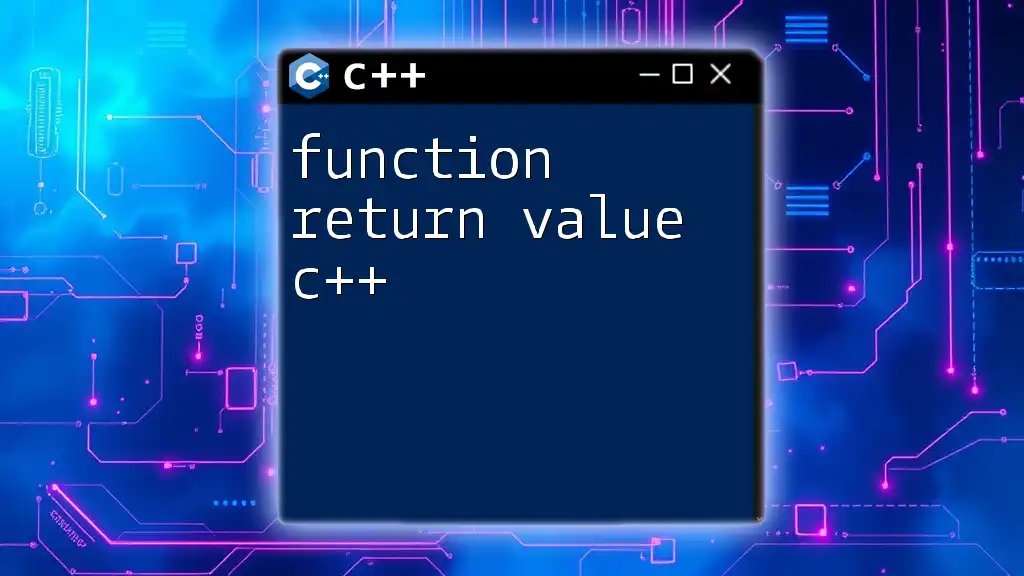
Creating a Function Prototype in C++
Step-by-Step Guide
Creating a function prototype involves identifying the need for a function, defining its return type, choosing an appropriate name, and identifying and specifying its parameters.
-
Identifying the Need: Determine the functionality you wish to include in your program that requires a function.
-
Writing the Prototype: Draft the prototype using the correct syntax.
-
Examples Based on Common Functions: Consider a prototype to calculate the area of a circle, where the formula returns a double value:
double calculateArea(double radius);
Placement of Function Prototypes
Prototypes can be placed in various locations within a code file:
- Before the main function: This is the simplest approach and works well for small programs.
- In headers for larger projects: For larger applications, it’s common to place function prototypes in header files to ensure all necessary function declarations are available throughout the project.
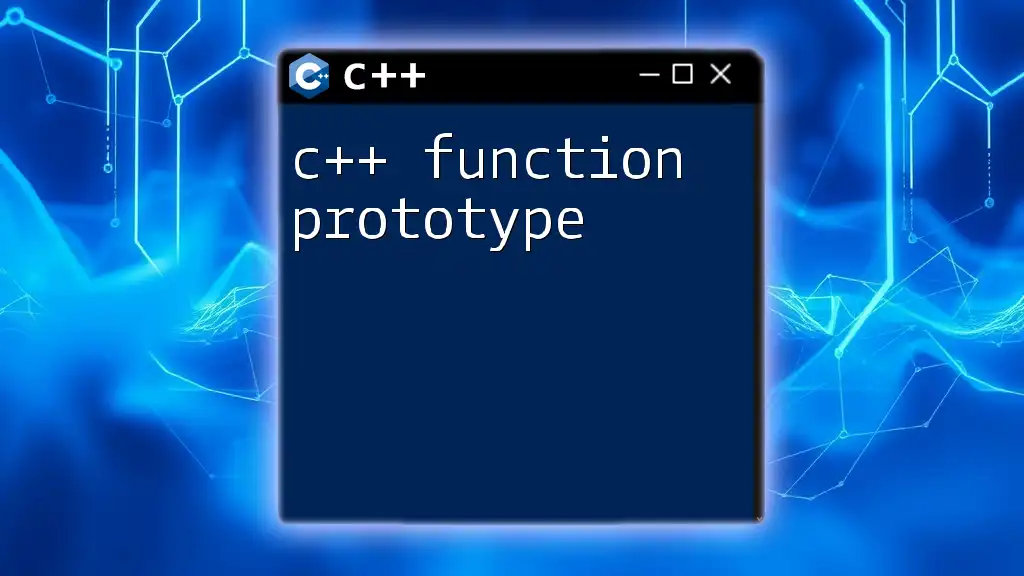
Advantages of Using Function Prototypes in C++
Enhancements to Code Organization
By utilizing prototypes, your code becomes more organized and manageable. They make the structure of your program clear from the outset, allowing programmers to understand available functions without needing to scroll through extensive code. To illustrate, a program without prototypes may appear disorganized, making it difficult to comprehend the function calls.
Flexibility in Function Usage
Prototypes allow you to call functions before their actual definitions appear in the code. This capability opens up flexibility in coding order. For instance, you can call a function like this:
int result = multiply(5, 10); // This can come before the definition
If the function had no prototype, attempting to call it before its definition would lead to a compilation error.
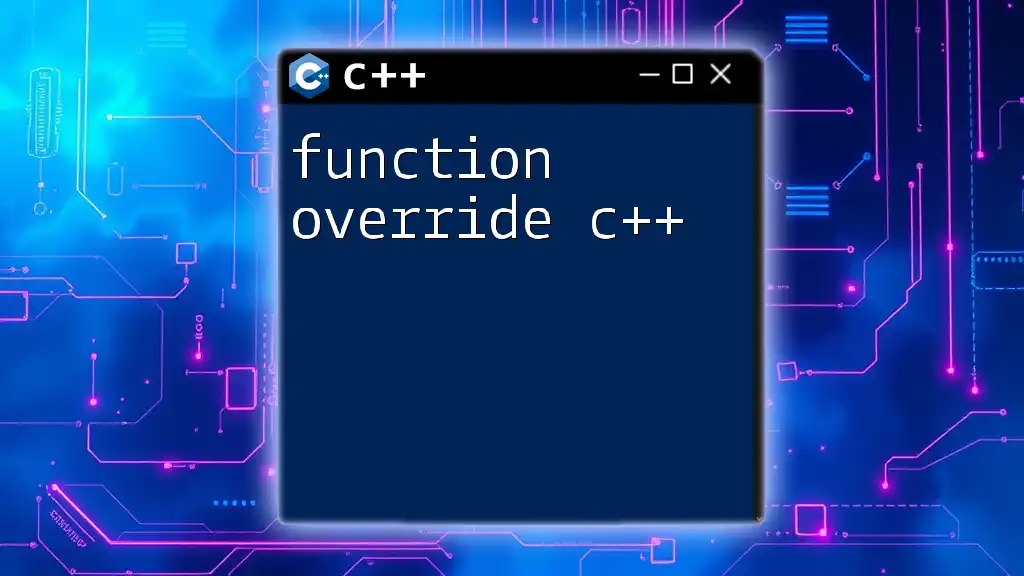
Function Prototype and Function Definition
Understanding the Relationship
It's critical to differentiate between function prototypes and function definitions. The prototype declares the function and its signature, while the definition provides the actual body of the function, detailing what the function does.
Example of Both in a Simple Program
Here’s how you can use both the prototype and the function definition in a simple program:
double multiply(double x, double y); // Prototype
double multiply(double x, double y) { // Definition
return x * y;
}
This structure ensures that whether you define your function before or after you call it, the compiler understands how to process the function.
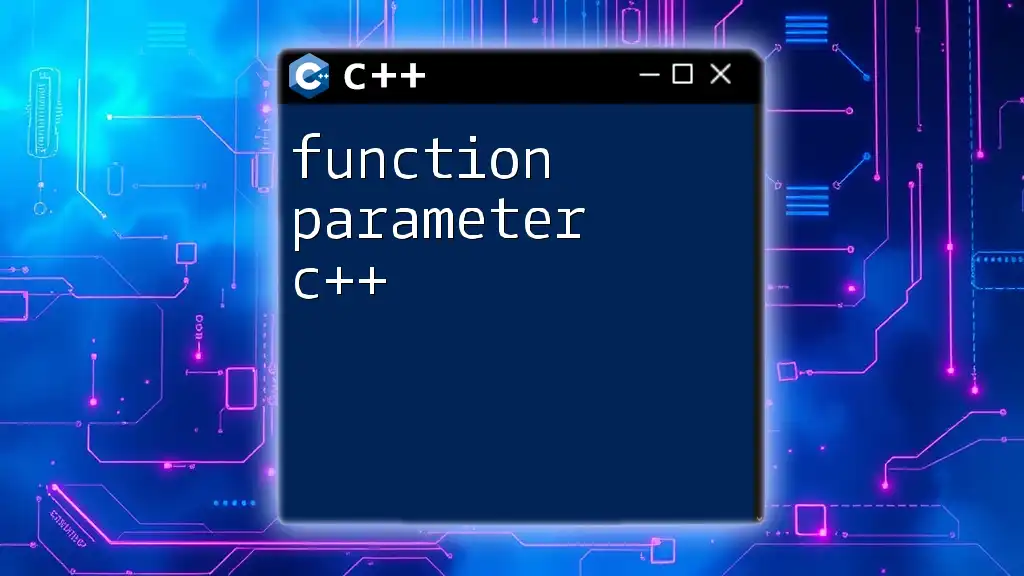
Common Mistakes with Function Prototypes in C++
Not Matching Parameters
One frequent mistake is failing to match the parameters in the prototype with those in the function definition. It’s crucial to maintain consistency in both sections to avoid undefined behavior or compilation errors. For example, consider this incorrect prototype:
void subtract(int a, double b); // Issue with types
If the definition had different types, it would cause confusion and errors during execution.
Forgetting to Include Prototypes
Another common issue is forgetting to include function prototypes entirely. This oversight can lead to compilation errors, as the compiler will not recognize function calls that precede their definitions. For instance, if a main function calls a function without its prototype, like below, the program will fail to compile:
int main() {
int result = computeSum(5, 10); // Error: computeSum not declared
return 0;
}
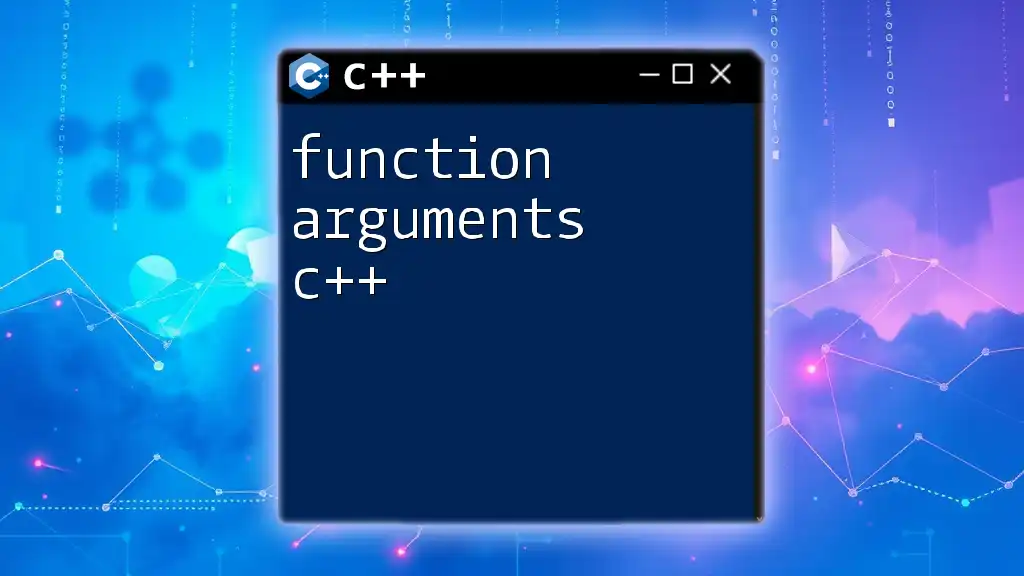
Conclusion
In summary, understanding a function prototype example in C++ is vital for effective programming. Function prototypes enhance readability, maintainability, and overall coding practices, making them a significant part of C++ development. They allow for a more organized approach to writing code, especially in larger projects. As you advance in your C++ programming journey, always consider utilizing function prototypes to improve your coding efficiency and clarity.
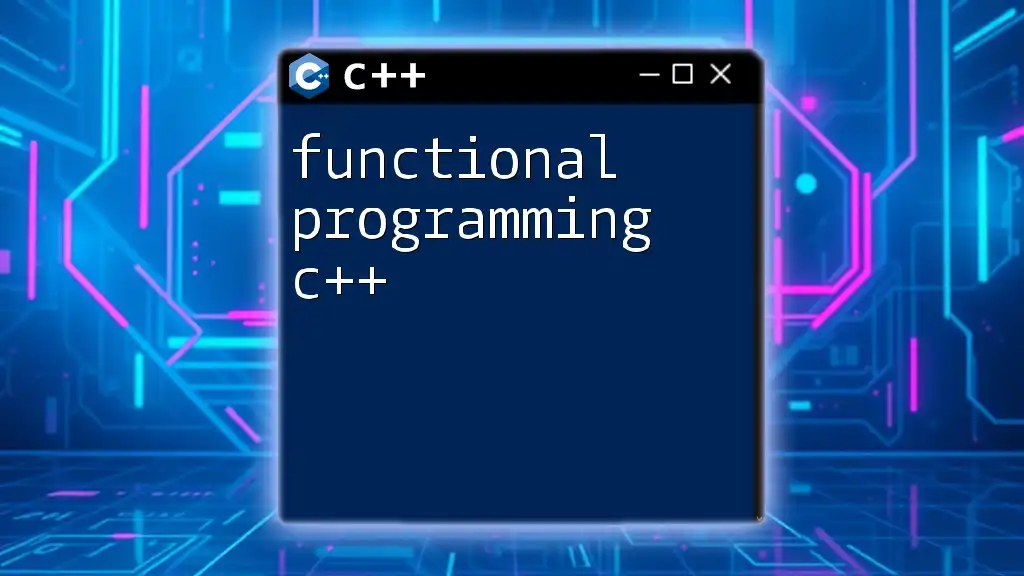
Additional Resources
To continue your learning journey, refer to:
- The official C++ documentation, which offers detailed insights into syntax and best practices.
- Recommended books and online courses dedicated to C++.
- Community forums and programming platforms where you can engage with fellow learners and experienced developers for additional support and resources.