Function overriding in C++ allows a derived class to provide a specific implementation of a function that is already defined in its base class, enabling polymorphic behavior.
Here's a code snippet to illustrate function overriding:
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() {
cout << "Base class show function called." << endl;
}
};
class Derived : public Base {
public:
void show() override { // Function overriding
cout << "Derived class show function called." << endl;
}
};
int main() {
Base* obj = new Derived();
obj->show(); // Calls the show function in Derived class
delete obj;
return 0;
}
Understanding Function Override in C++
What is Function Override?
Function override in C++ refers to the ability of a derived class to provide a specific implementation of a function that is already defined in its base class. This concept is fundamental to object-oriented programming (OOP) and allows for more dynamic behavior in software design.
Function overriding is crucial because it enables polymorphism, which allows methods to do different things based on the object that it is acting upon, even though they share the same name. This promotes flexibility in programming and leads to cleaner, more maintainable code.
Key Terminology
- Polymorphism: The ability for different classes to be treated as instances of the same class through a common interface, particularly through a base class reference.
- Base Class: The class that is extended or inherited from. It contains the original implementation of methods that can be overridden.
- Derived Class: The class that inherits from the base class. It can override methods from the base class to provide specific behavior.

The Mechanics of Function Overriding in C++
How Function Override Works in C++
Function overriding differs from overloading in that overloading allows multiple functions with the same name but different parameters, while overriding involves redefining an existing function in a base class within a derived class.
The syntax for function overriding is straightforward. It usually involves using the `virtual` keyword in the base class and optionally the `override` keyword in the derived class to indicate that the function is being overridden.
Basic Example of Function Override
This simple code snippet demonstrates how to override a function.
class Base {
public:
virtual void show() {
std::cout << "Base Class Show" << std::endl;
}
};
class Derived : public Base {
public:
void show() override { // Function override
std::cout << "Derived Class Show" << std::endl;
}
};
In this example, the `Base` class defines a method called `show`. The `Derived` class extends `Base` and provides its own implementation of `show`. The keyword `virtual` allows this method to be overridden in the derived class, and using `override` ensures that we are intentionally overriding a base class method, which adds a layer of safety.
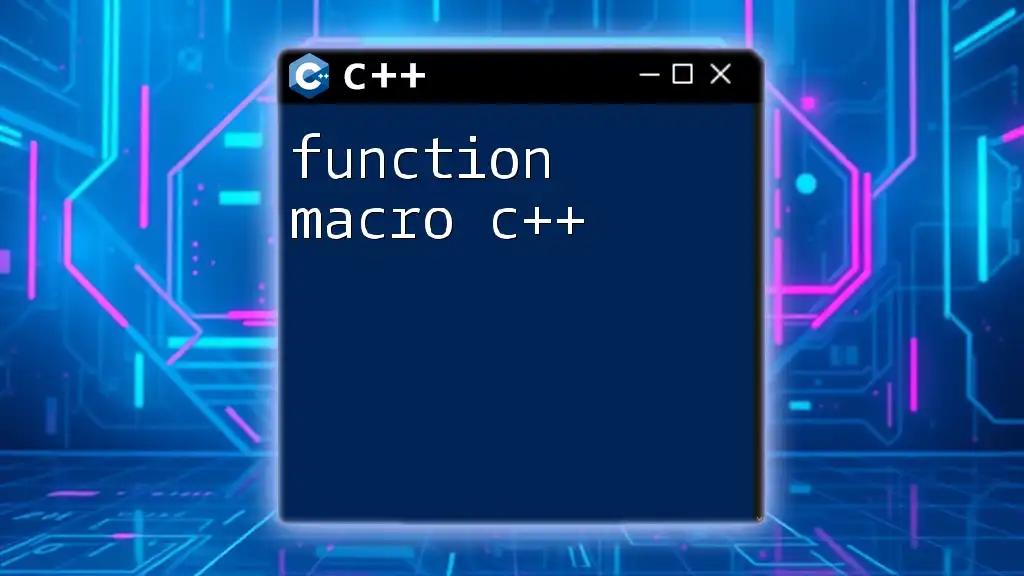
Overriding Functions in C++ - Best Practices
When to Use Function Override
Function overriding is beneficial in scenarios where you want the base class to define a general behavior that can be specialized in derived classes. Common use cases include:
- User interfaces where different elements implement the same action (e.g., buttons, sliders).
- Game development where various entities (enemies, players) may have similar functionalities but distinct behaviors.
Common Pitfalls in Function Overriding
While function overriding is powerful, there are pitfalls to avoid. One key issue is const correctness. When overriding a method, the const-ness of the function must match between the base and derived classes.
For example, consider the following snippet that illustrates a common error:
class Base {
public:
virtual void show() const { // Const function
std::cout << "Base Class Show" << std::endl;
}
};
class Derived : public Base {
public:
void show() override { // Error: void show() should also be const
std::cout << "Derived Class Show" << std::endl;
}
};
In this case, the derived class's `show()` method is not `const`, which leads to a compile-time error if you attempt to override. Paying close attention to the method signatures is essential for correct behavior.
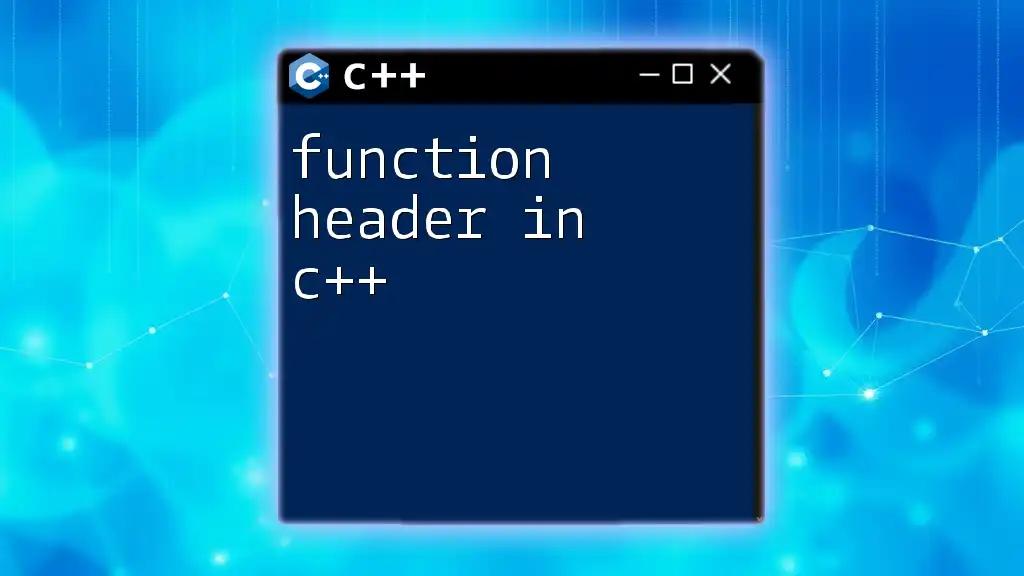
Function Overriding Vs Function Hiding
Understanding Function Hiding
Function hiding occurs when a derived class defines a new function with the same name as a function in its base class, but does not utilize the `virtual` keyword. Instead of overriding, the derived class simply hides the base class's implementation.
class Base {
public:
void show() {
std::cout << "Base Class Show" << std::endl;
}
};
class Derived : public Base {
public:
void show(int x) { // This hides the Base class show()
std::cout << "Derived Class Show with Value: " << x << std::endl;
}
};
In this example, the `show()` method from the `Base` class is hidden in the `Derived` class by defining a new function `show(int x)`. As a result, it is possible to call different implementations depending on the parameters.
Why Prefer Function Overriding?
Benefits of function overriding over hiding include:
- Improved code readability: It’s easier to understand the intention behind a class design.
- Better design: Overriding facilitates polymorphism, allowing you to work interchangeably with various derived class types.
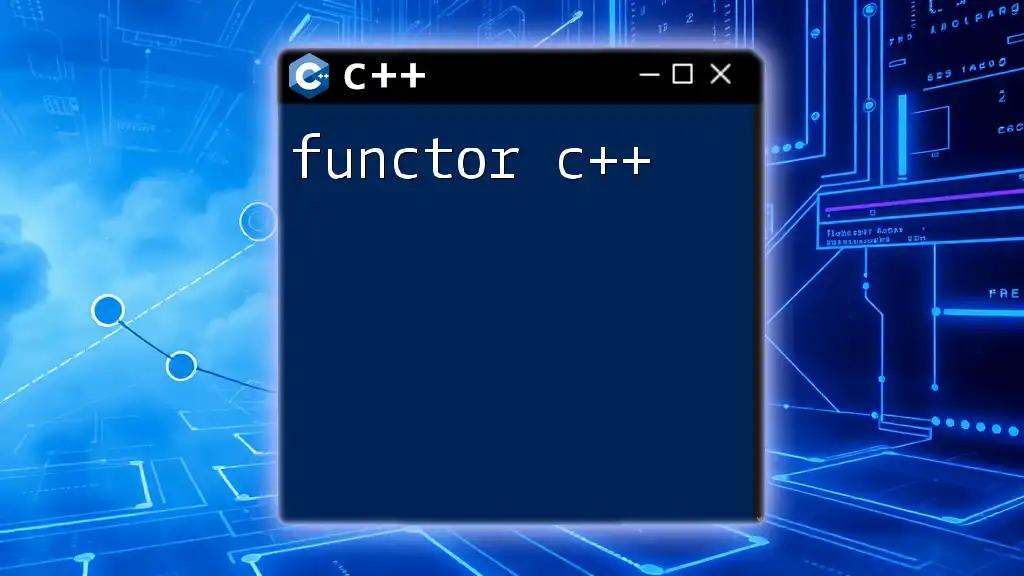
Advanced Concepts in Function Overriding
Pure Virtual Functions
A pure virtual function is a function declared in a base class that has no implementation. It acts as a placeholder and forces derived classes to provide their specific implementations. Pure virtual functions make the base class abstract.
class AbstractBase {
public:
virtual void display() = 0; // Pure virtual function
};
class ConcreteDerived : public AbstractBase {
public:
void display() override {
std::cout << "Concrete Implementation of Display" << std::endl;
}
};
Here, the `AbstractBase` class declares a pure virtual function `display()`, requiring any derived class, such as `ConcreteDerived`, to implement this method. If a derived class does not implement it, it too becomes an abstract class.
Virtual Destructors
When working with class inheritance, it is essential to use virtual destructors in base classes. This ensures that when a base class pointer is deleted, the derived class's destructor is also invoked, avoiding potential memory leaks.
class Base {
public:
virtual ~Base() {
std::cout << "Base Destructor" << std::endl;
}
};
class Derived : public Base {
public:
~Derived() {
std::cout << "Derived Destructor" << std::endl;
}
};
In this example, defining a virtual destructor in the `Base` class ensures that when an object of `Derived` is deleted through a base class pointer, both destructors are called in the correct order.
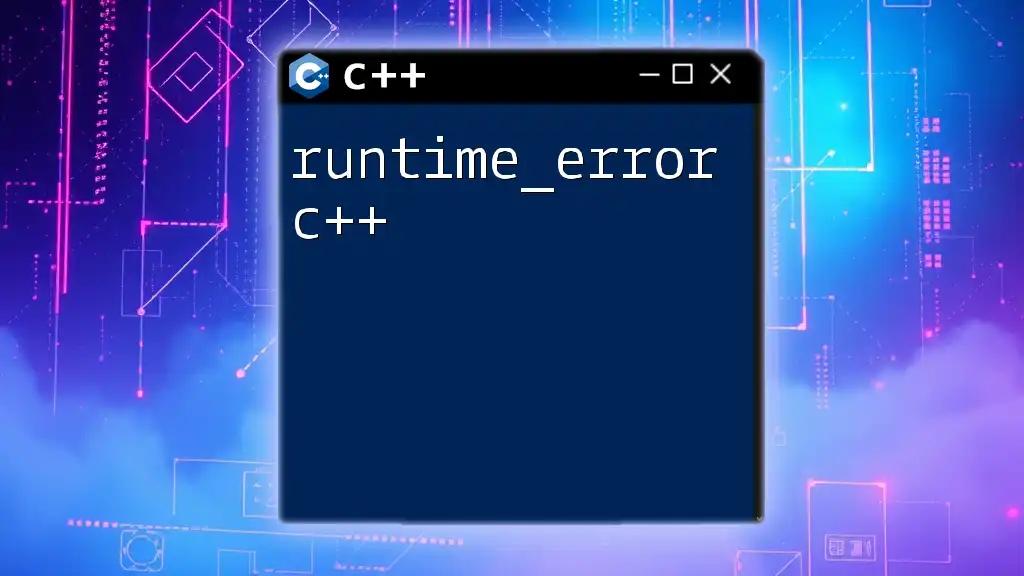
Conclusion
Recap of Function Overriding in C++
In summary, function overriding in C++ is a powerful mechanism that allows derived classes to provide specific implementations for methods defined in a base class. Utilizing this feature properly leads to more flexible, reusable, and maintainable code, establishing the foundation for effective polymorphism.
Resources for Further Learning
For those looking to dive deeper into this topic, several excellent resources—books, online courses, and tutorials—are available on advanced C++ topics. Exploring these can significantly enhance your understanding of function overriding and object-oriented principles.
Call to Action
Now that you have a comprehensive understanding of function override in C++, consider incorporating these concepts into your projects. Experiment with creating your classes and functions and observe how overriding can streamline your code and enhance its functionality!