A function header in C++ defines the function's name, return type, and parameters, providing a blueprint for how the function can be called.
int add(int a, int b);
Understanding the Syntax of a Function Header
Components of a Function Header
A function header in C++ consists of several essential components that define how a function behaves and how it can be used.
-
Return Type: This indicates the data type that will be returned by the function. Depending on the function's purpose, return types can be:
- `int`: For integer values.
- `void`: When the function does not return any value.
- `double`: For decimal values, etc.
Example:
int sum() { return 10; }
-
Function Name: This is the identifier for the function and should convey the function's purpose. There are some best practices to follow:
- Function names should be descriptive and typically use CamelCase or underscore_case for readability.
Example:
void calculateArea() { // Function code }
-
Parameter List: This is a set of parameters that the function accepts, which are enclosed in parentheses. Parameters can define what input is necessary for the function's operation.
- Each parameter must specify a type and a name.
Example:
int multiply(int a, int b) { return a * b; }
Basic Structure of a Function Header
The general structure of a C++ function header can be summarized as follows:
returnType functionName(parameterList);
For example:
void displayMessage();
Annotated Example:
Here’s an annotated example showcasing the components:
void displayHello(); // 'void' is the return type, 'displayHello' is the function name, '()' indicates there are no parameters.
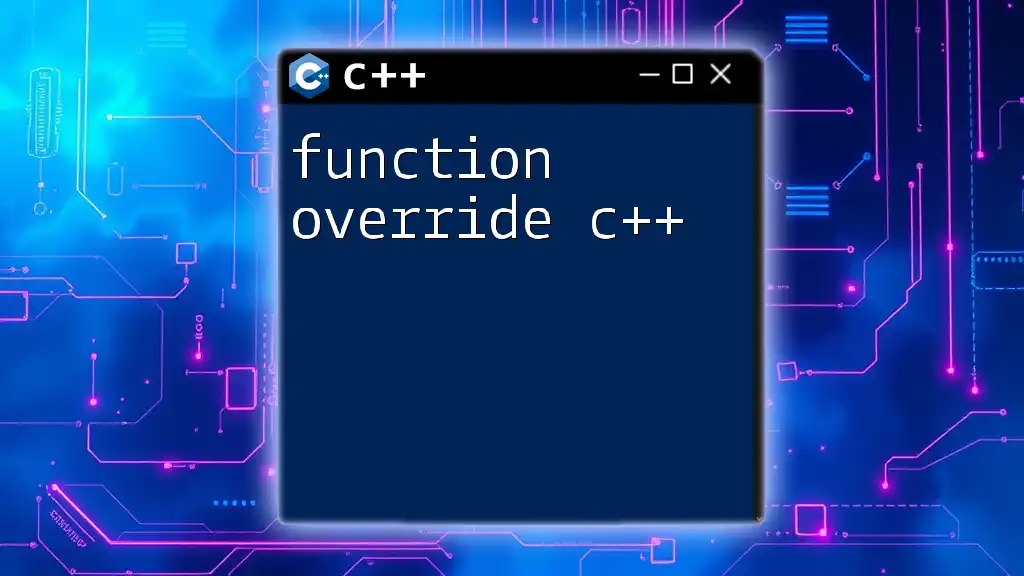
Types of Function Headers
No-parameter Function Headers
These function headers do not require any input parameters. Such functions can perform specific tasks without external data.
Example Code:
void greet() {
std::cout << "Hello, World!";
}
This function, when called, will simply output "Hello, World!" to the console.
Parameterized Function Headers
These function headers accept parameters, allowing for more dynamic operations that depend on the input values.
Example Code:
int add(int a, int b) {
return a + b;
}
The `add` function takes two integer parameters and returns their sum.
Function Headers with Default Parameters
C++ allows functions to be defined with default values for parameters. If the calling function does not provide an argument, the default value will be used.
Example Code:
void printValue(int value = 10) {
std::cout << "Value: " << value;
}
If `printValue()` is called without an argument, it will display "Value: 10".
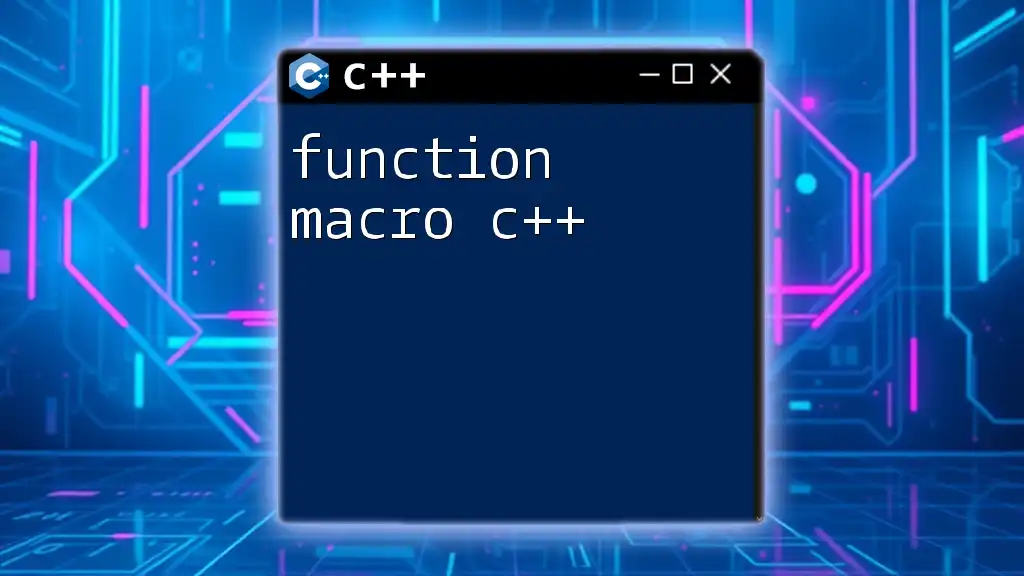
Best Practices for Writing Function Headers in C++
Clarity and Readability
One of the critical aspects of programming is ensuring that your code is clear and easily readable. Use descriptive names for functions that indicate their purpose. This helps others (and yourself) understand your code better in the future.
Consistency
Maintaining consistency in your naming conventions and the structure of your function headers fosters a more understandable codebase. Consistent formatting allows developers to predict function signatures easily.
Documentation
Adding documentation to your function headers can drastically improve the maintainability of your code. Use inline comments to briefly describe the function's action or purpose.
By using a documentation tool like Doxygen, you can automate the generation of documentation from your comments, making your code even more user-friendly.
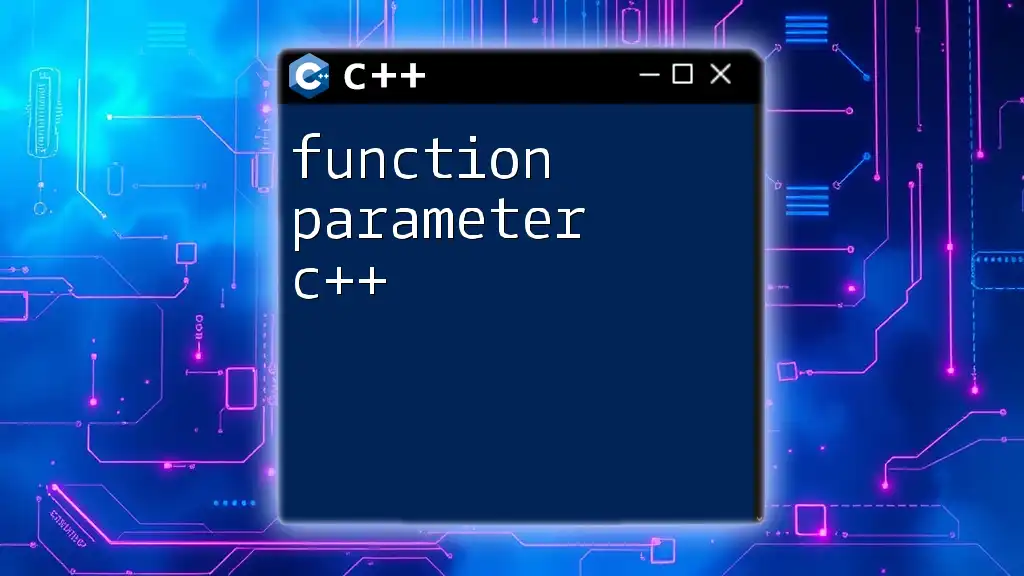
Common Mistakes in Function Headers
Overcomplicating Parameter Lists
A common mistake is to create overly complex parameter lists that can be confusing or unwieldy. This makes your functions harder to read and use.
Example of Poor Parameter Design:
void process(int a, int b, double c, std::string str);
Instead of this, focus on simplicity and clarity, potentially breaking the functionality into smaller, simpler functions.
Ignoring Return Types
Never omit return types from your function headers, as this can lead to confusion and bugs. Each function should explicitly declare what type it will return.
Example Code:
void functionWithoutReturn() {
// This is a function without an explicit return type
}
Naming Conflicts
When naming functions, be aware of potential conflicts with other identifiers in your codebase. Functions sharing the same name in different scopes can lead to ambiguity. Consider using namespaces or unique prefixes to avoid these conflicts.
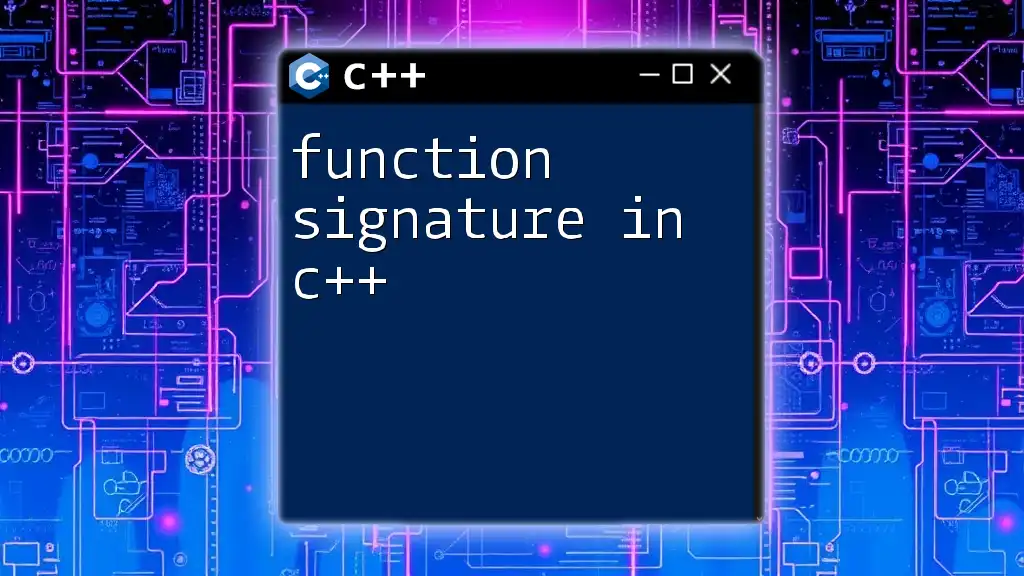
Conclusion
Understanding and correctly implementing function headers in C++ is essential for writing clean, effective code. By following best practices in naming, documentation, and structuring your function headers, you can improve the clarity and usability of your code while minimizing common errors. Embrace these principles as you work, and leverage them to enhance your C++ programming skills!
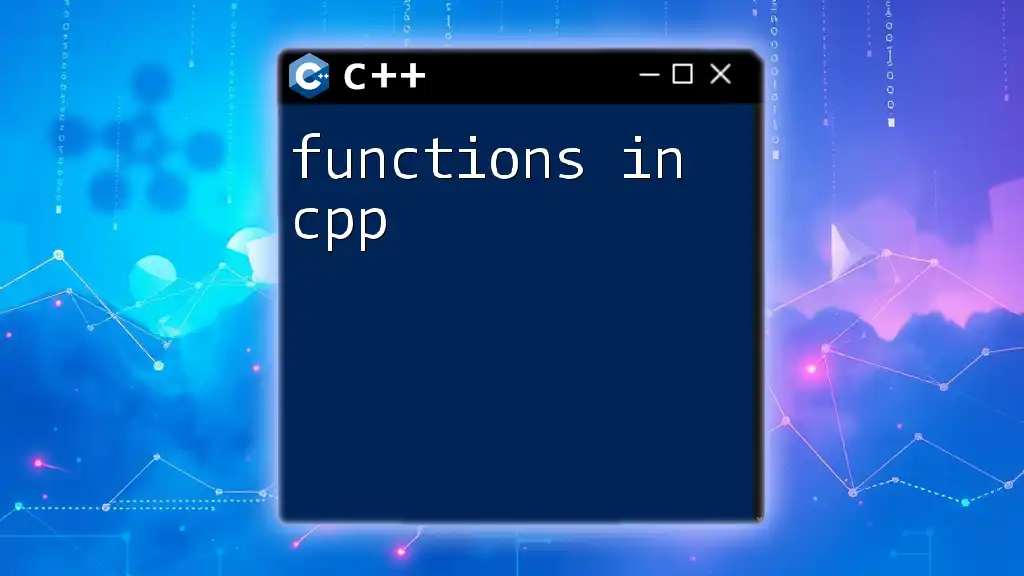
Additional Resources
To further explore the topic, consider diving into recommended books, online courses, and community forums dedicated to C++ programming. Engaging with these resources can offer deeper insights and practical knowledge.