In C++, a function is a reusable block of code that performs a specific task and can take inputs and return outputs, allowing for modular programming.
Here's a simple example of a function that adds two integers:
#include <iostream>
using namespace std;
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 3);
cout << "The sum is: " << result << endl;
return 0;
}
What are Functions?
Functions are essentially blocks of code designed to perform a specific task. They are fundamental building blocks of any C++ program. Functions help in compartmentalizing the code, allowing programmers to structure their work better and facilitate easier debugging and testing processes. By encapsulating logic within functions, developers can reuse pieces of code without rewriting them.

Why Use Functions?
Using functions in code presents several advantages:
- Code Reusability: Functions allow you to write code once and use it multiple times throughout your program, reducing redundancy.
- Improved Readability: Well-named functions can give contextual meaning to what the code is doing, making it easier to understand.
- Reduced Complexity: Breaking code into smaller, manageable pieces makes the overall logic easier to follow.
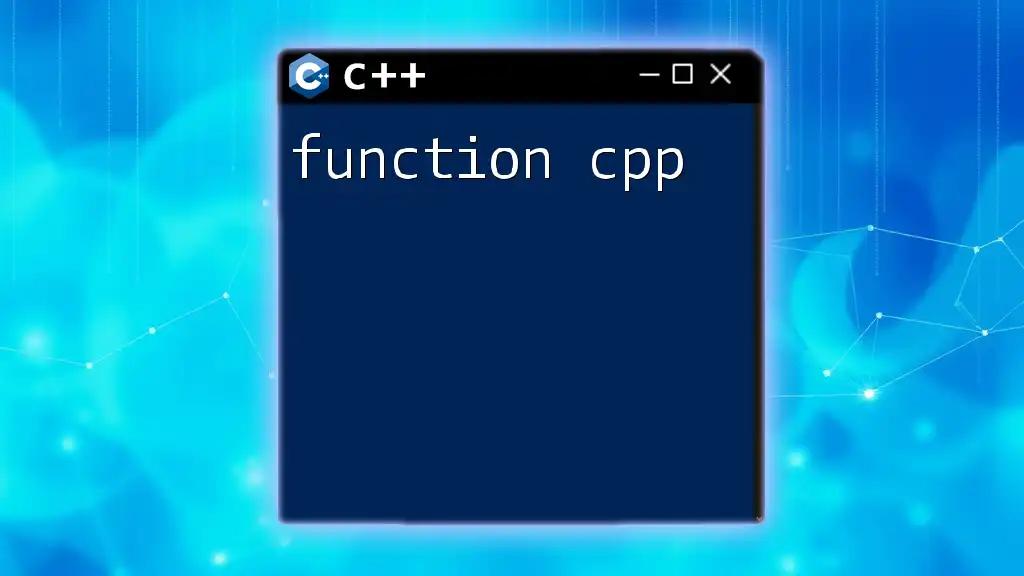
Types of Functions in C++
C++ supports various types of functions, which can be broadly categorized into two groups: built-in functions and user-defined functions.
Built-in Functions
C++ provides a set of functions out-of-the-box that you can use for common operations. These are called built-in functions. Examples include mathematical functions like `sqrt()`, `pow()`, or string manipulation functions from the `cstring` library. These functions simplify coding by providing ready-made solutions for common tasks.
User-defined Functions
User-defined functions are those functions that programmers create themselves to tailor their code to specific needs. They can be divided into two categories:
Void Functions
Void functions do not return a value. They perform actions but do not produce any output that can be assigned to a variable. For example, consider a simple void function that prints a message:
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
This function, when called, will display "Hello, World!" on the console.
Return Functions
Return functions, on the other hand, perform tasks and return a value. This can be particularly useful for calculations. For example:
int add(int a, int b) {
return a + b;
}
This function takes two integers, adds them together, and returns the result.

Function Declaration and Definition
Function Declaration
The function declaration is a prototype that tells the compiler what the function will look like. It includes the function's name, return type, and parameters. For instance:
int multiply(int, int);
This declares a function named `multiply`, which takes two integers and returns an integer.
Function Definition
The function definition provides the actual implementation of the function. It includes the code that will execute when the function is called. The syntax resembles the declaration but includes the function body:
int multiply(int a, int b) {
return a * b;
}
This piece of code defines `multiply` in such a way that it multiplies its two integer parameters and returns the product.

Function Parameters and Arguments
Function parameters are the variables listed in the function's definition that accept values when the function is called.
Parameter Types
There are two main types of parameters:
- Pass by Value: When a function is called, the actual values are assigned to the function's parameters. Changes made inside the function do not affect the original values.
void passByValue(int x) {
x = x + 5;
}
- Pass by Reference: Here, the function parameters refer to the actual variable rather than a copy of it. Any changes made will affect the original variable.
void passByReference(int &y) {
y = y + 5;
}
Understanding the difference between these two types is crucial for effective function design.
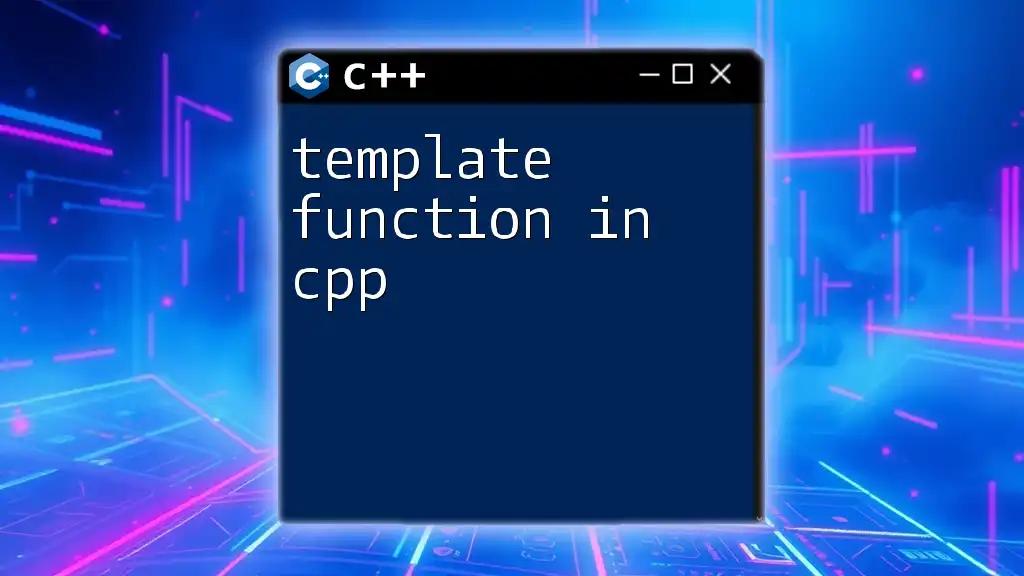
Function Overloading
Function overloading is a feature that allows multiple functions to have the same name with different parameters. This enhances code readability and usability. For instance:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Here, both functions named `add` perform the addition, but one works with integers while the other works with doubles. The compiler distinguishes between them based on parameter types.
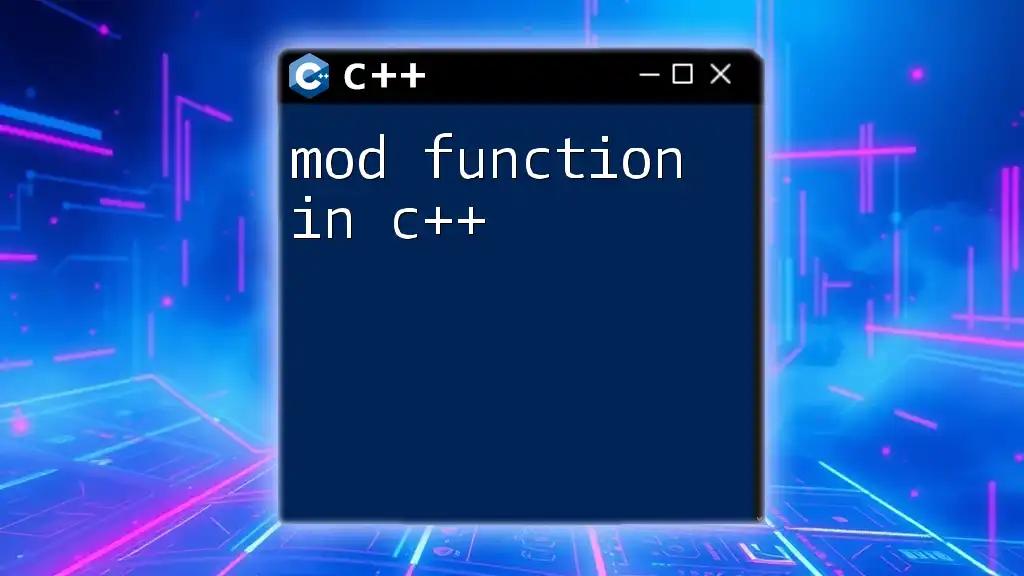
Default Arguments in Functions
Default arguments allow you to define a value that will be used if no value is provided when the function is called. This can lead to more flexible functions. For example:
void display(int a, int b = 10) {
std::cout << "a: " << a << ", b: " << b << std::endl;
}
In this snippet, if you call `display(5)`, `b` will automatically be set to `10`.

Inline Functions
Inline functions are a powerful feature that can enhance performance. They are defined using the keyword `inline`, suggesting to the compiler to insert the function's body where the function call is made, potentially reducing the overhead associated with function calls.
inline int square(int x) {
return x * x;
}
Using inline functions is ideal for small functions that are called frequently.
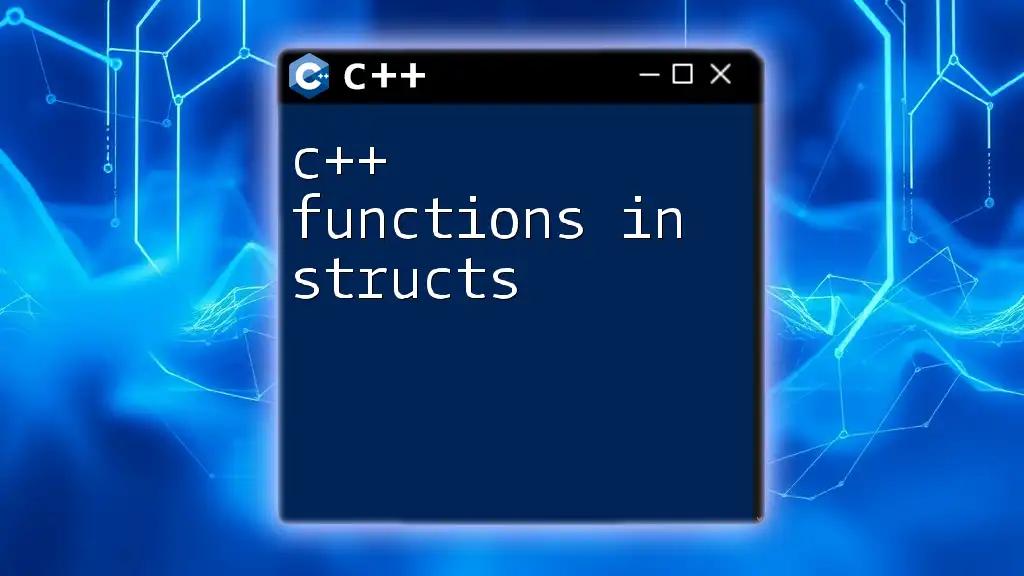
Recursive Functions
A recursive function is one that calls itself in order to solve a problem. Each recursive function has a base case to stop recursion and a recursive case that breaks the problem into smaller sub-problems.
Here is an example of a recursive function that computes the factorial of a number:
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
In this example, as long as `n` is greater than `1`, the function will keep calling itself with a decremented value of `n` until it hits the base case of `1`.
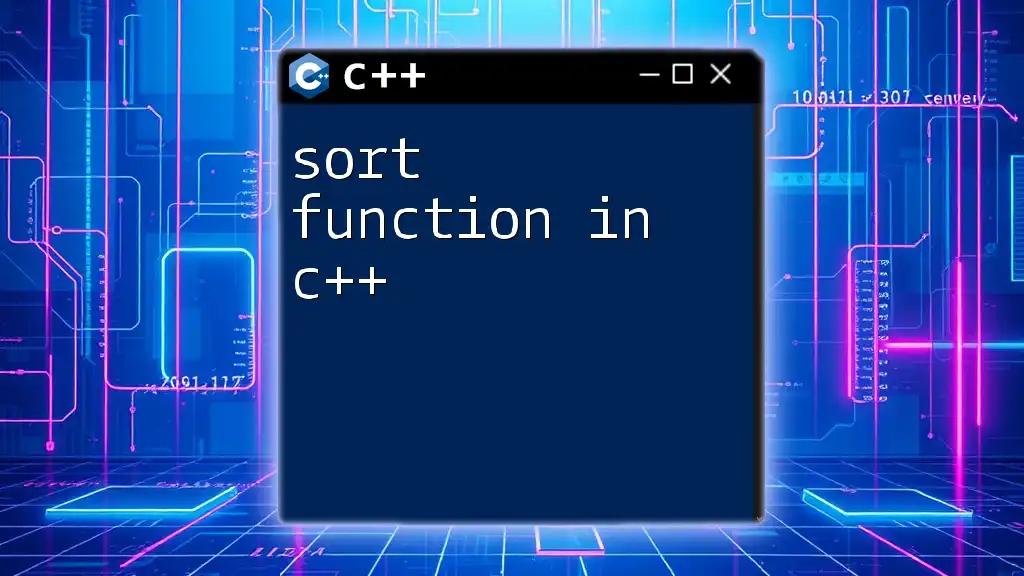
Summary of Key Takeaways
In summary, understanding functions in C++ enhances your ability to write effective, efficient code. Functions offer tools for code reuse, modularity, and clarity, making it essential for any programmer to grasp their various types and applications.
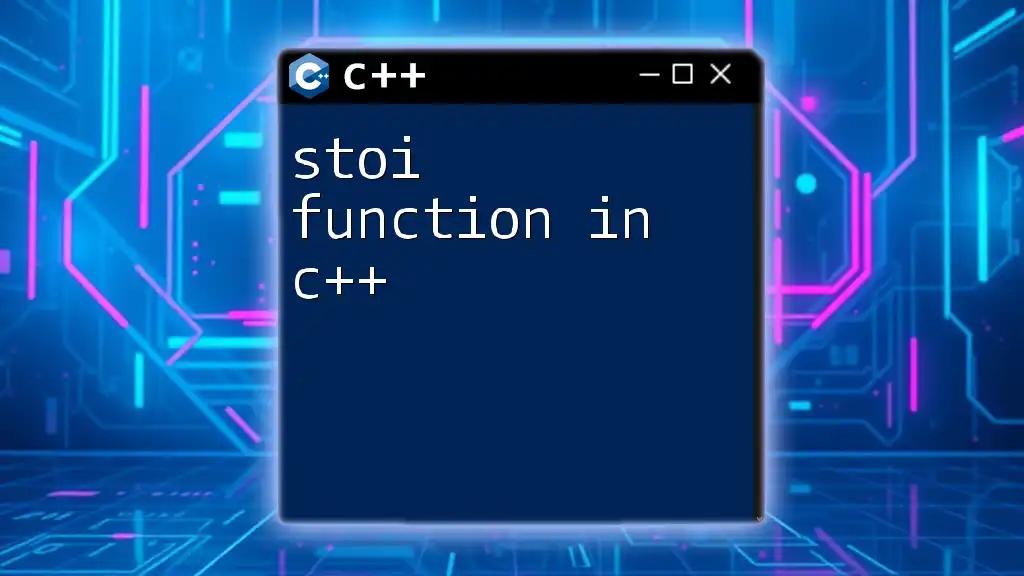
Call to Action
Now that you’ve learned about functions, it's time to apply this knowledge. Start by creating your own functions to solve specific problems in C++. Consider enrolling in practical courses or workshops designed for hands-on experience with functions to deepen your understanding.
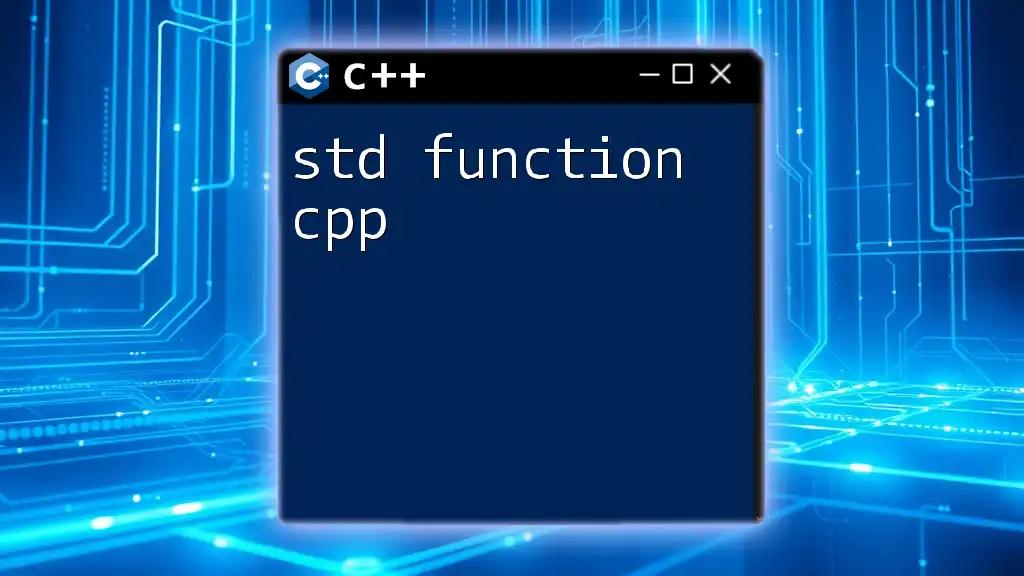
Additional Resources
To further your learning journey, explore additional books, tutorials, and online resources focused on mastering functions in C++. Consider coding platforms that offer challenges and projects to help reinforce these concepts practically.