The `stoi` function in C++ converts a string to an integer, making it easy to handle numerical input from strings.
Here is a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << "The integer value is: " << num << std::endl;
return 0;
}
Understanding the stoi Function in C++
The stoi function in C++ is a powerful utility that allows developers to convert a string into an integer. This function is particularly beneficial when working with user inputs, file parsing, or even program configurations that involve numeric values stored as strings. By understanding how to effectively use stoi, programmers can ensure smoother data handling and manipulation in their applications.
How to Use stoi in C++
Basic Usage of stoi
To begin, the basic usage of the stoi function is straightforward. The function takes a string as an argument and converts it to an integer.
Here’s a simple example demonstrating the conversion:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << "Converted number: " << num << std::endl;
return 0;
}
In this snippet, the string `"123"` is converted to the integer `123`. It's a fundamental operation in many programs, showcasing the utility of the stoi function.
Using the Index Parameter
The stoi function allows for an optional parameter, `idx`, which enables you to track the position in the string where the conversion stopped. This can be crucial when you're working with mixed content in strings and only want the numeric part.
Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str = "123abc";
std::size_t idx;
int num = std::stoi(str, &idx);
std::cout << "Converted number: " << num << ", next character index: " << idx << std::endl;
return 0;
}
In this example, the variable `idx` will indicate that the next character following the numeric part starts at index 3. This provides additional control when parsing strings that contain both numbers and letters.
Understanding the Base Parameter
A unique feature of the stoi function is the ability to specify a base for the conversion. The base can be useful when dealing with different numeral systems like binary, octal, or hexadecimal.
Consider the following example where we convert binary and hexadecimal strings:
#include <iostream>
#include <string>
int main() {
std::string binaryStr = "1010"; // Represents 10 in decimal
std::string hexStr = "1A"; // Represents 26 in decimal
int binaryNum = std::stoi(binaryStr, nullptr, 2);
int hexNum = std::stoi(hexStr, nullptr, 16);
std::cout << "Binary to integer: " << binaryNum << std::endl;
std::cout << "Hexadecimal to integer: " << hexNum << std::endl;
return 0;
}
In this code, we specify the base in the stoi function calls. The binary string `"1010"` is converted to `10`, while the hexadecimal string `"1A"` converts to `26`. It highlights the versatility of the stoi function in handling various formats of numeric strings.
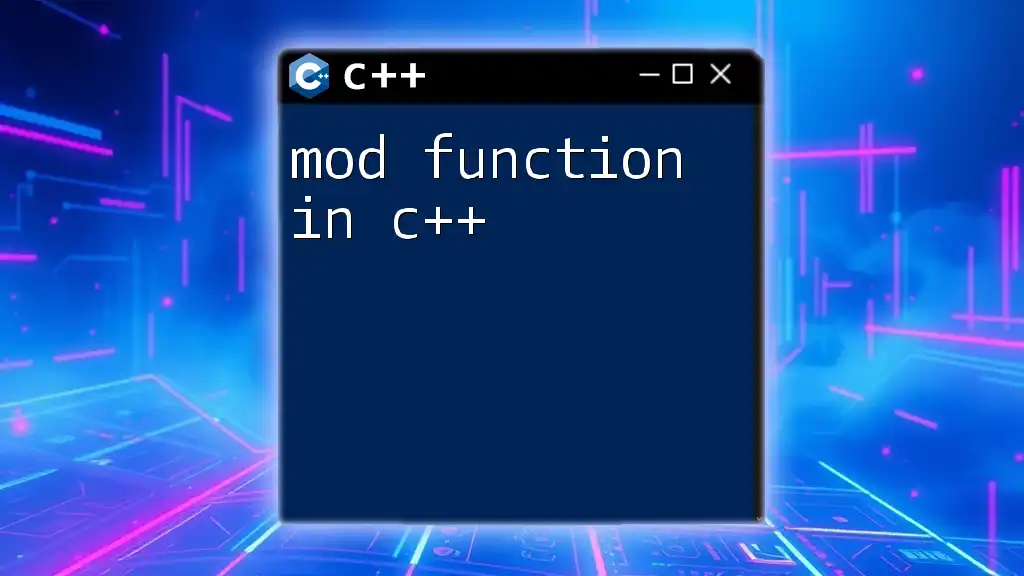
Error Handling with stoi
Using the stoi function can lead to exceptions if the input string is not suitable for conversion. Two common exceptions are:
- std::invalid_argument: Thrown when the input string does not contain a valid representation of an integer.
- std::out_of_range: Thrown when the converted value is outside the range of representable values for an `int`.
Proper error handling is essential to create robust applications. Here’s a code snippet illustrating exception handling:
#include <iostream>
#include <string>
int main() {
std::string str = "xyz"; // Invalid string
try {
int num = std::stoi(str);
} catch (const std::invalid_argument& e) {
std::cout << "Invalid argument: cannot convert to int." << std::endl;
} catch (const std::out_of_range& e) {
std::cout << "Out of range: the number is too large." << std::endl;
}
return 0;
}
In this example, if the string passed to stoi is invalid (like `"xyz"`), the program gracefully handles the exception, informing the user instead of crashing.
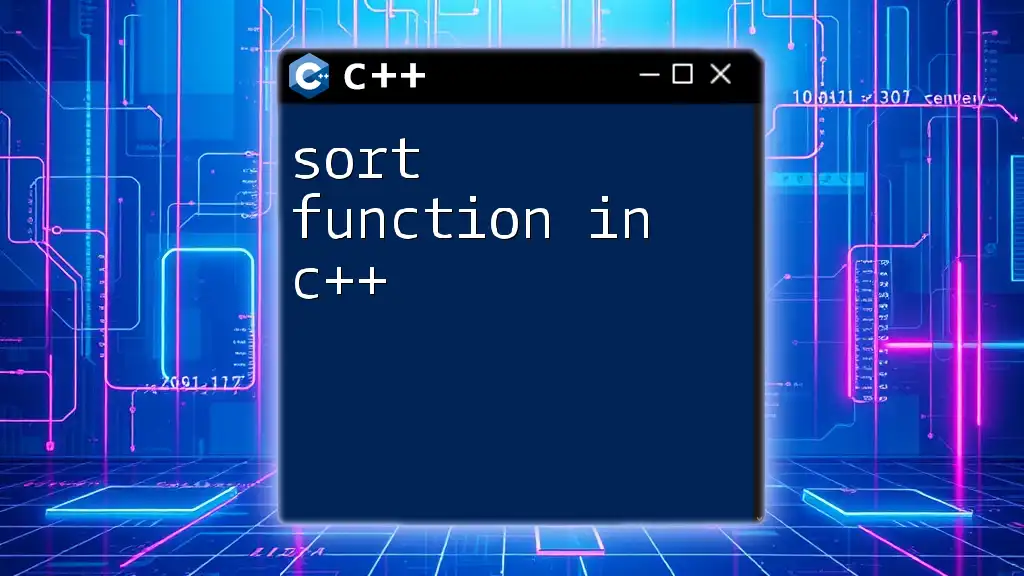
Best Practices for Using stoi
When to Use stoi
The stoi function in C++ is beneficial in many scenarios. Common use cases include:
- User Input Processing: When accepting numeric input from users in a text format.
- File Parsing: Extracting numbers from structured text files.
- Converting Configurations: When reading numeric values from configuration files that might be formatted as strings.
However, it’s vital to ensure that the input string is cleaned and validated before attempting conversion.
Performance Considerations
While the stoi function is efficient for most applications, performance can vary depending on the context. When dealing with large datasets or requiring high performance, consider the following:
- If the use case demands frequent conversions, optimizing the logic around input handling can yield significant performance improvements.
- For converting large volumes of strings, consider std::stringstream as an alternative. This method might offer better performance in specific scenarios due to lower overhead in complex parsing.
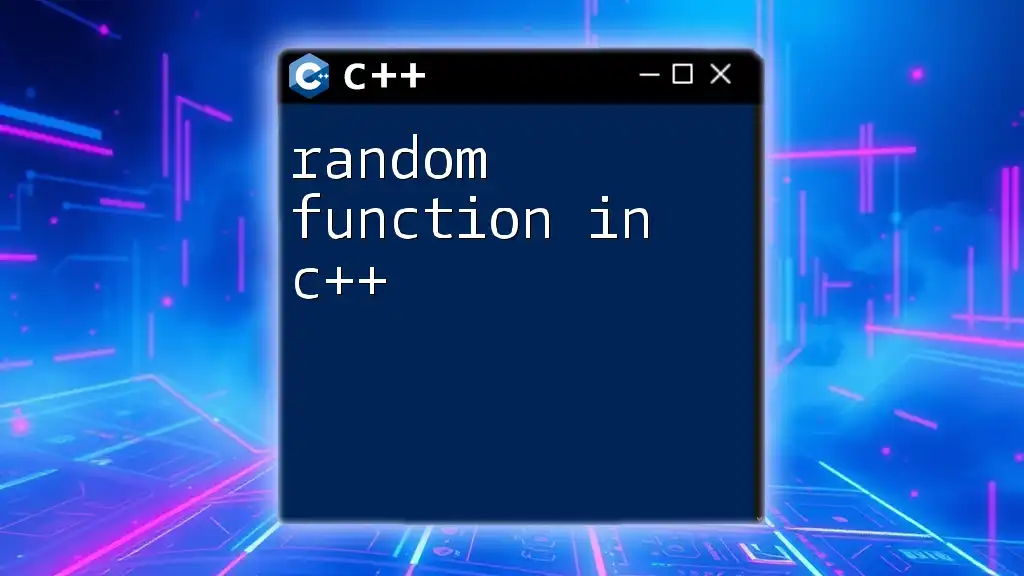
Conclusion
The stoi function in C++ is a powerful tool for converting strings to integers, essential for handling various types of data input. Understanding its parameters, such as the optional index and base, along with effective error handling, enhances your programming capabilities and ensures robust application functionality.
By implementing best practices and tailoring usage to specific needs, you can maximize the effectiveness of the stoi function in your projects. As you continue to explore C++, experimenting with functions like stoi will deepen your understanding and improve your programming finesse.