In C++, functions can be categorized into several types, including built-in functions, user-defined functions, inline functions, recursive functions, and lambda functions, each serving different purposes in programming.
Here's a simple example of a user-defined function in C++:
#include <iostream>
using namespace std;
// User-defined function
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl; // Output: Sum: 8
return 0;
}
Understanding Functions in C++
A function is a block of code designed to perform a specific task. Functions are essential in programming, including C++, as they facilitate modularization, code reusability, and clarity. By breaking a program into manageable pieces, functions promote better organization and maintenance.
The basic structure of a function in C++ includes a return type, function name, parameters (if any), and the function body. Here’s an example of a simple function declaration and definition:
returnType functionName(parameterType parameterName) {
// Function body
}
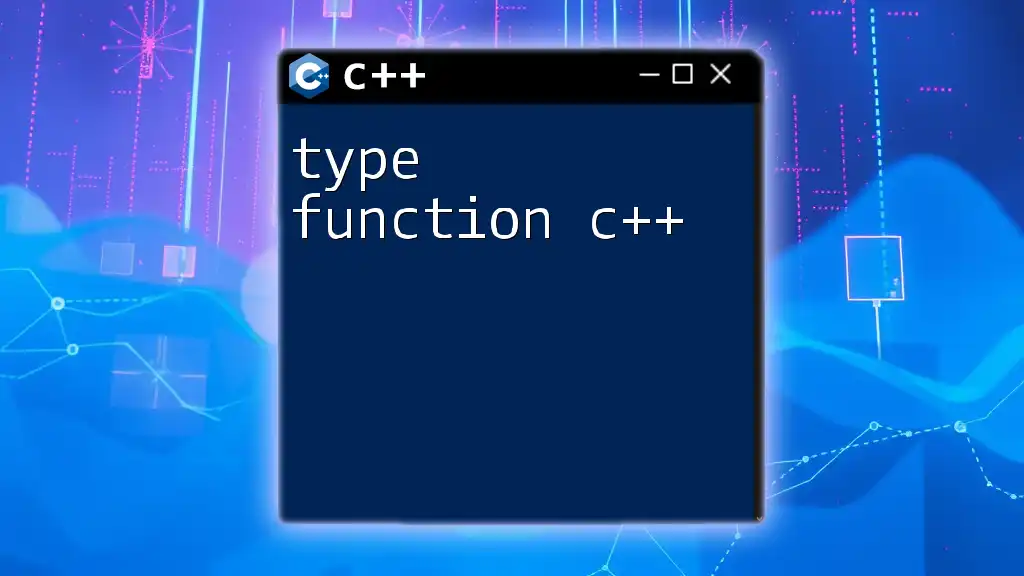
Types of Functions in C++
Built-in Functions
Built-in functions in C++ are those provided by the language itself, allowing developers to perform a variety of tasks without the need to define their implementations. These functions are part of the Standard Library.
Characteristics of Built-in Functions
- Predefined: They come fully developed and ready to use, saving time for the programmer.
- Standardized: Their usage is consistent across different C++ environments, contributing to portability.
Examples
A common example includes input and output functions, such as `std::cout` and `std::cin`:
#include <iostream>
using namespace std;
int main() {
cout << "Enter your name: ";
string name;
cin >> name;
cout << "Hello, " << name << "!" << endl;
return 0;
}
User-defined Functions
User-defined functions are created by programmers to perform specific tasks tailored to their needs. These functions allow for greater flexibility and customization in programming.
Characteristics of User-defined Functions
- Reusability: Once defined, a function can be called multiple times throughout the program, reducing redundancy.
- Modularity: Functions can be developed independently, making it easier to debug and maintain code.
Types of User-defined Functions
Simple Functions
A simple function performs an operation without requiring parameters or returning a value. Here’s a basic example:
void greet() {
cout << "Hello, World!" << endl;
}
Functions with Parameters
Functions can accept parameters, allowing them to operate on provided data. For instance, this function takes one parameter:
void greet(string name) {
cout << "Hello, " << name << "!" << endl;
}
Functions Returning Values
Functions can return values to the calling program. This is useful for calculations or data processing. For example, here’s a function that adds two integers:
int add(int a, int b) {
return a + b;
}
Function Overloading
Function overloading enables multiple functions to share the same name but with different parameter types or counts. This enhances code readability and functionality while maintaining a single function name.
Importance and Benefits
- Ease of Use: Developers can use the same function name for similar operations, leading to cleaner code.
- Flexibility: Different data types can be processed without the need for distinct function names.
Examples
Here’s an example of function overloading in action:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Inline Functions
Inline functions are a way to suggest that the compiler replaces a function call with the function's code itself. This can improve performance by reducing function call overhead. However, it is up to the compiler to adhere to this suggestion.
Advantages of Inline Functions
- Performance Gain: Especially beneficial for small functions that are called frequently.
- Reduced Function Call Overhead: Fast execution due to inlining.
Here's how you can define an inline function:
inline int square(int x) {
return x * x;
}
Recursive Functions
A recursive function is one that calls itself in order to solve a problem. Recursion simplifies code, especially for problems with a clear recursive structure, like calculating factorials.
Benefits and Concerns
- Simplicity: Solves complex problems with elegant code.
- Performance: Can lead to stack overflow if not properly controlled, particularly with deep recursion.
Here’s an example of a recursive factorial function:
int factorial(int n) {
if(n <= 1) return 1;
return n * factorial(n - 1);
}
Lambda Functions
Lambda functions are a feature introduced in C++11 that allows the creation of anonymous function objects. They are syntactically compact and can capture variables from the surrounding scope.
Advantages of Lambda Functions
- Conciseness: Useful for short, single-use functions without the need for formal declarations.
- Versatility: Particularly effective with algorithms, such as `std::sort`.
Here’s an example demonstrating the use of a lambda function to sort numbers:
#include <algorithm>
#include <vector>
int main() {
vector<int> nums = {5, 3, 2, 4, 1};
sort(nums.begin(), nums.end(), [](int a, int b){ return a < b; });
}
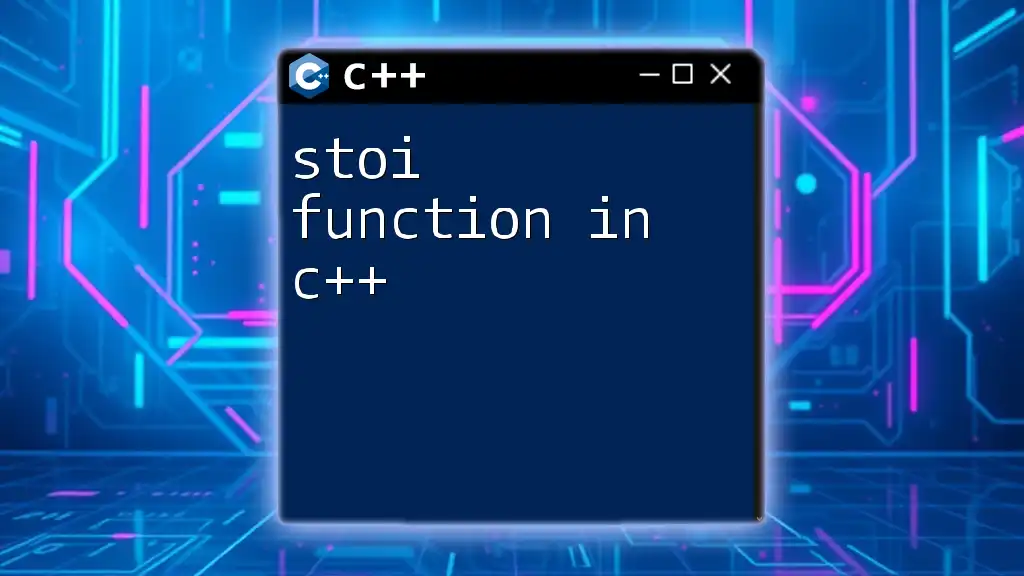
Advanced Types of Functions in C++
Function Pointers
A function pointer is a variable that stores the address of a function. This allows for dynamic function calls, which can be particularly useful in certain programming patterns.
How to Declare and Use Function Pointers
You declare a function pointer by specifying the return type, the pointer’s name, and the function signature. Here’s an example:
void greet() {
cout << "Hello!" << endl;
}
void (*funcPtr)() = &greet;
funcPtr();
Callbacks
Callbacks are a powerful programming concept, where a function is passed as an argument to another function. This allows for custom behavior depending on the needs of the program.
Real-world Applications of Callbacks
Callbacks are often used in event handling, asynchronous programming, and in APIs for defining behavior in response to specific actions (such as mouse clicks).
Here’s a basic example:
void performOperation(void (*callbackFunc)()) {
// Some operation...
callbackFunc(); // callback
}
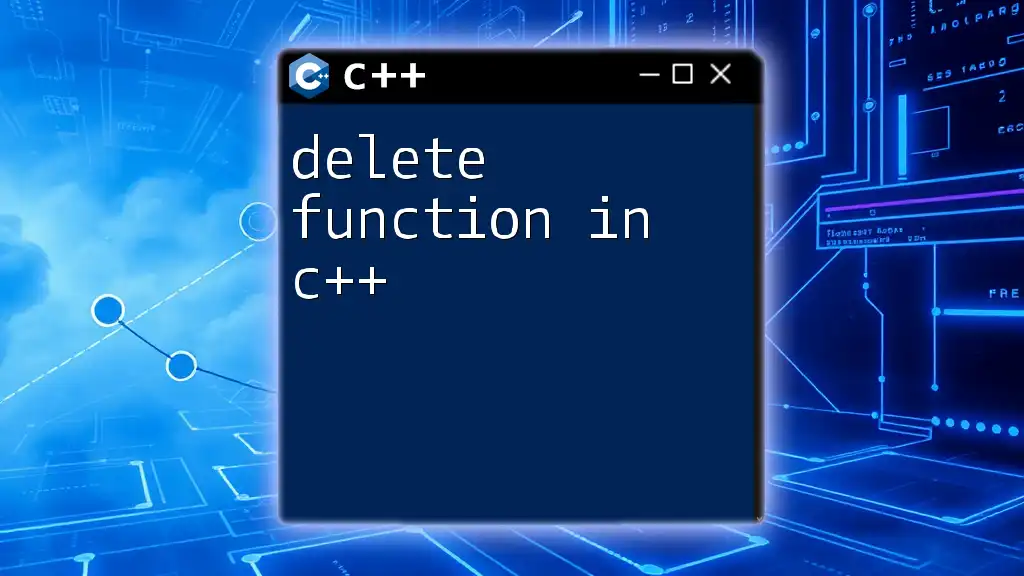
Conclusion
Understanding the various types of functions in C++ is crucial for effective programming. From built-in functions that expedite development to user-defined and advanced constructs such as function pointers and lambda functions, each type adds unique capabilities and flexibility to your code. By mastering these concepts, programmers can enhance code clarity, maintainability, and reusability. Practice the types of function in C++ discussed in this article to solidify your knowledge and improve your programming skills.