The `delete` function in C++ is used to deallocate dynamically allocated memory previously obtained with `new`, ensuring efficient memory management and preventing memory leaks.
Here’s an example of how to use the `delete` function:
#include <iostream>
int main() {
int* ptr = new int; // dynamically allocate memory
*ptr = 42; // assign value
std::cout << *ptr << std::endl; // output the value
delete ptr; // deallocate memory
return 0;
}
Understanding Memory Management in C++
What is Dynamic Memory Allocation?
Dynamic memory allocation is a crucial concept in C++ that allows programmers to manage memory more flexibly. When you allocate memory dynamically, you request memory from the heap instead of the stack, which enables you to create variables that can vary in size during the program's runtime. This is particularly useful when the size of your data structures is not known at compile time.
The Role of Pointers in C++
Pointers act as variables that store the memory address of another variable. They are central to handling dynamic memory allocation in C++. By using pointers, you can create and manipulate dynamically allocated objects or arrays without needing to know of their size until runtime.
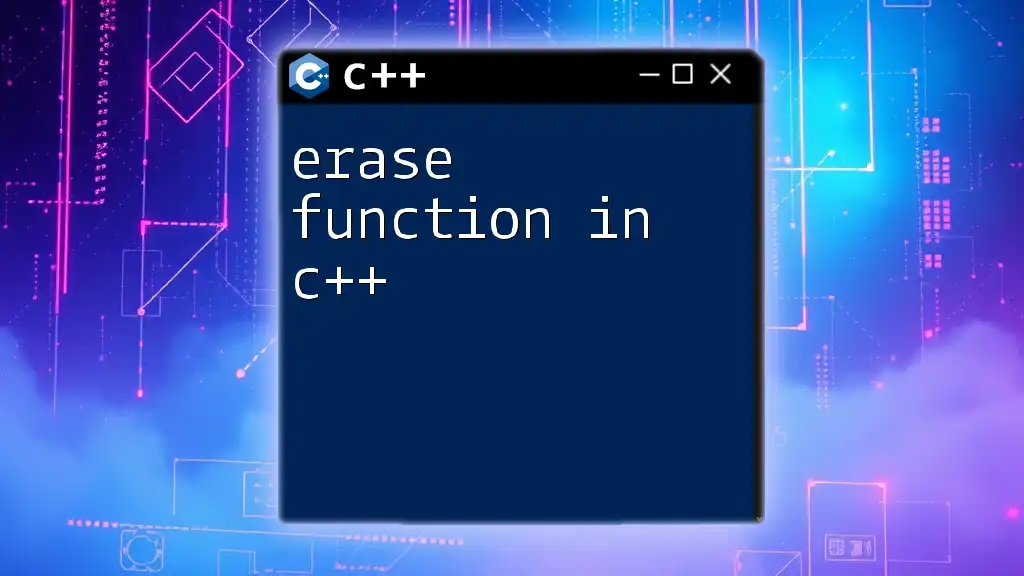
The Delete Function in C++
The Basics of the Delete Function
The delete function in C++ is critical for freeing dynamically allocated memory. When you allocate memory using `new`, it's your responsibility to release it when it's no longer needed. The syntax for using `delete` is straightforward:
delete pointer;
This command deallocates the memory block that the pointer points to, thus preventing memory leaks.
The Types of Delete Functions
Delete for Single Objects
The delete function handles memory management for single objects allocated via `new`. For example, consider the following code:
class MyClass {
public:
MyClass() { /* constructor */ }
~MyClass() { /* destructor */ }
};
MyClass* obj = new MyClass();
// Do something with obj
delete obj; // Free the allocated memory
In this snippet, we allocate an instance of `MyClass` on the heap using `new`. The corresponding `delete obj;` line deallocates the memory once we’re done using the object, ensuring resources are released properly.
Delete for Arrays
When dealing with arrays, it's essential to use the `delete[]` syntax to prevent undefined behavior. For instance:
int* arr = new int[10]; // Allocating an array of 10 integers
// Use the array
delete[] arr; // Freeing the allocated memory for the array
Using `delete[]` correctly ensures that the destructor of each element in the array is called, allowing for proper cleanup.
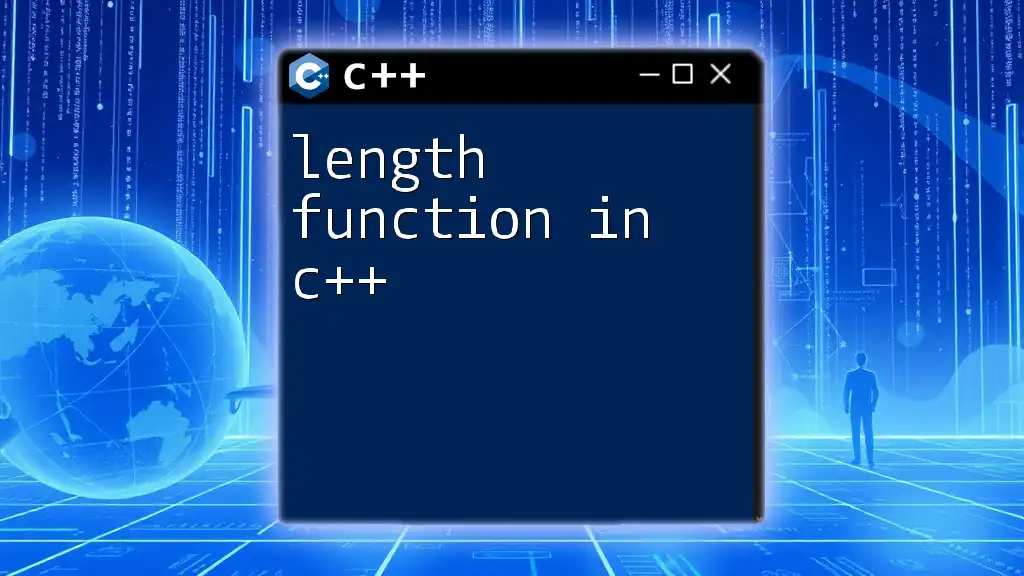
Common Scenarios and Best Practices
Memory Leaks
A memory leak occurs when a program allocates memory but fails to deallocate it. This can lead to reduced performance and eventual program crashes, especially in long-running applications. The delete function prevents memory leaks by explicitly releasing memory blocks:
int* num = new int; // Allocate memory
// forget to delete
// Memory leak occurs
delete num; // Should be called to clean up
Dangling Pointers
A dangling pointer arises when an object is deleted but the pointer still points to that same memory location. Accessing it can lead to unpredictable behavior. After deleting a pointer, always set it to `nullptr`:
int* num = new int(42);
delete num; // Memory is freed
num = nullptr; // Prevents dangling pointer
By setting `num` to `nullptr`, you make it clear that the pointer no longer points to a valid memory location.
Best Practices for Using Delete in C++
- Always match new with delete: For every use of `new`, there should be a corresponding use of `delete`. Similarly, for every `new[]`, use `delete[]`.
- Initialize pointers: Always initialize pointers before you use them. This helps avoid accidental access to garbage values.
- Set pointers to `nullptr` after deletion: This practice helps avoid issues with dangling pointers.
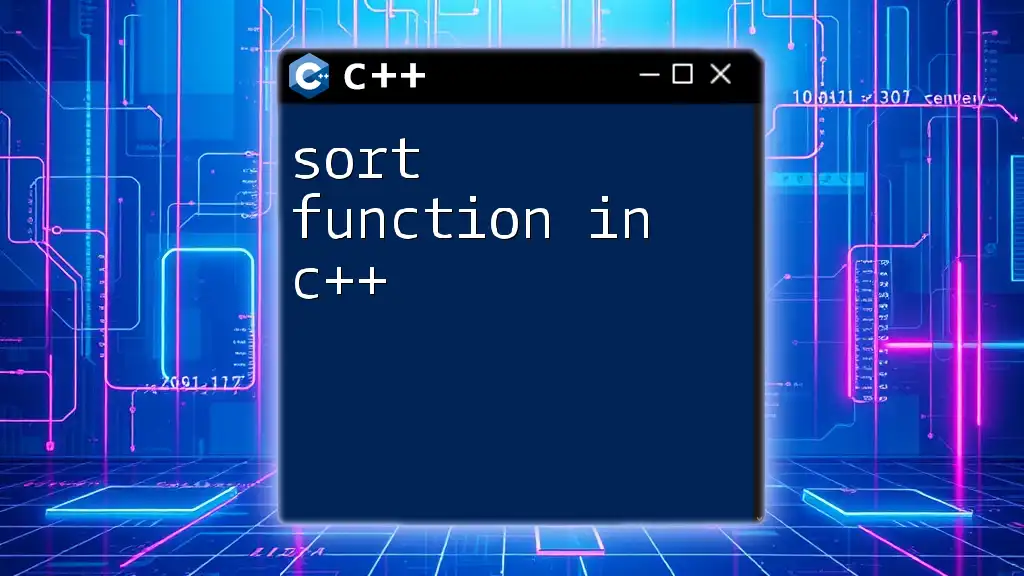
Advanced Topics
Overloading the Delete Operator
C++ allows operator overloading, which means you can define custom behavior for the `delete` operator. Overloading can help manage complex resource management within your class:
class MyClass {
public:
void* operator new(size_t size) {
// Custom allocation logic
}
void operator delete(void* pointer) {
// Custom deallocation logic
}
};
By defining these operators, you can control how instances of your class are allocated and deallocated, giving you flexibility and control.
Smart Pointers as an Alternative to Raw Pointers
In modern C++, it is often better to use smart pointers like `std::unique_ptr` or `std::shared_ptr`. Smart pointers manage memory automatically, significantly reducing the risk of memory leaks and dangling pointers:
#include <memory>
std::unique_ptr<MyClass> obj = std::make_unique<MyClass>();
// No need for delete, memory will be freed automatically when obj goes out of scope
Using smart pointers is a best practice because they handle memory management transparently, allowing you to focus more on your application logic without worrying about explicit memory management.
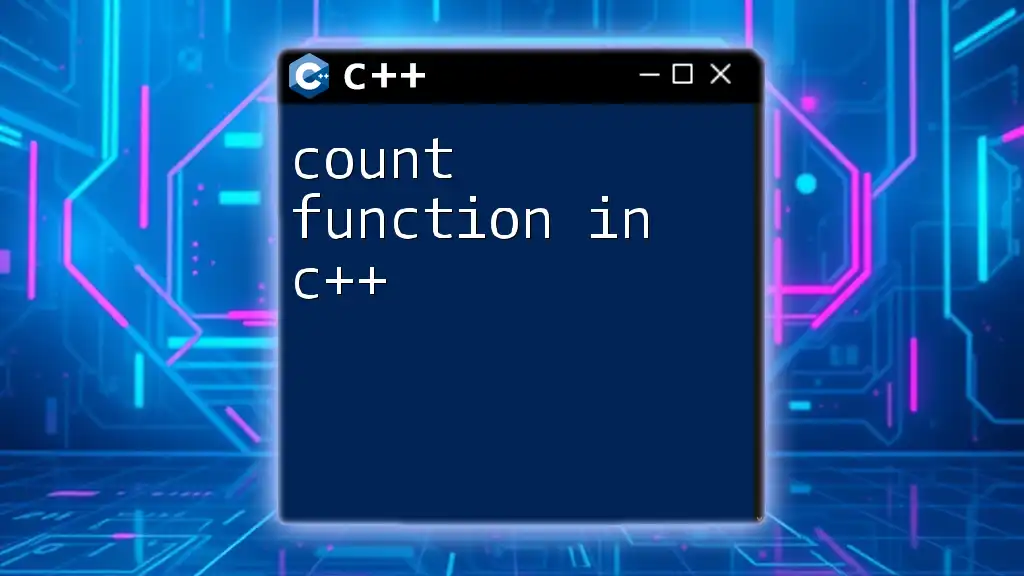
Conclusion
Understanding the delete function in C++ is essential for effective memory management. By correctly using `delete` and `delete[]`, you can prevent memory leaks and dangling pointers. Adopting best practices, including using smart pointers, can greatly simplify memory management in your applications, allowing you to write cleaner and more efficient C++ code.
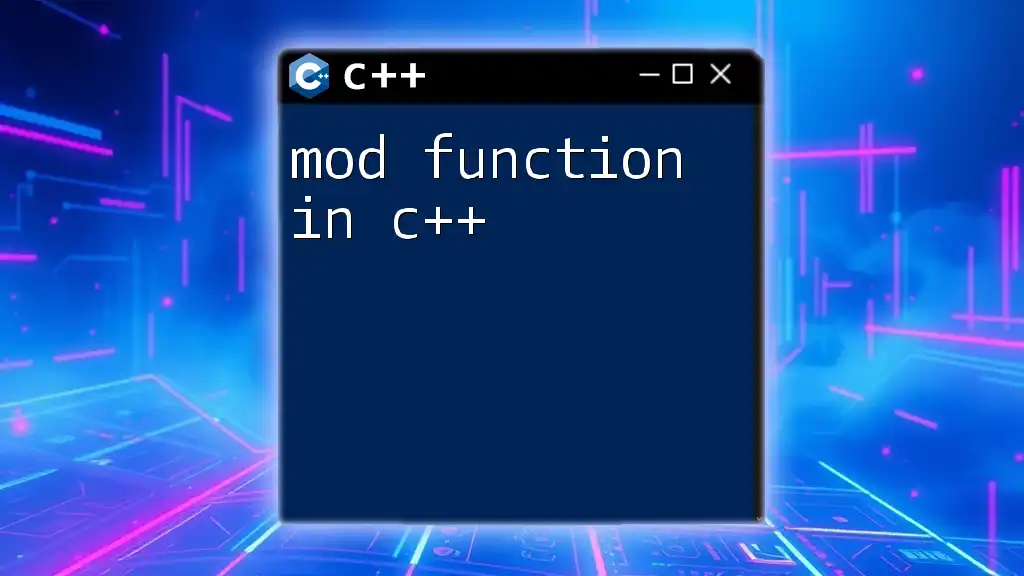
Additional Resources
Consider exploring more on C++ memory management or tools like Valgrind for debugging memory issues. Engaging with practical exercises will solidify your understanding and mastery of using the delete function effectively in your C++ projects.