A user-defined function in C++ allows programmers to encapsulate a specific task into a reusable block of code, improving modularity and readability. Here's a simple example:
#include <iostream>
using namespace std;
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl; // Output: Sum: 8
return 0;
}
Benefits of Using User-Defined Functions
User-defined functions in C++ bring significant advantages to programming. First, they enhance code reusability. When we define a function, we can call it multiple times throughout our program, eliminating the need to rewrite the same code. This adheres to the DRY (Don't Repeat Yourself) principle, making our programs cleaner and easier to manage.
Second, functions promote modularity. By breaking a complex problem down into smaller, manageable parts, each with its dedicated functionality, we make our code easier to understand and maintain. This approach not only simplifies debugging but also allows for easier enhancements in the future.
Third, the use of user-defined functions leads to improved readability and maintainability. Organized code with descriptive function names helps other developers (and ourselves, when we return to it later) understand the program's structure and flow.
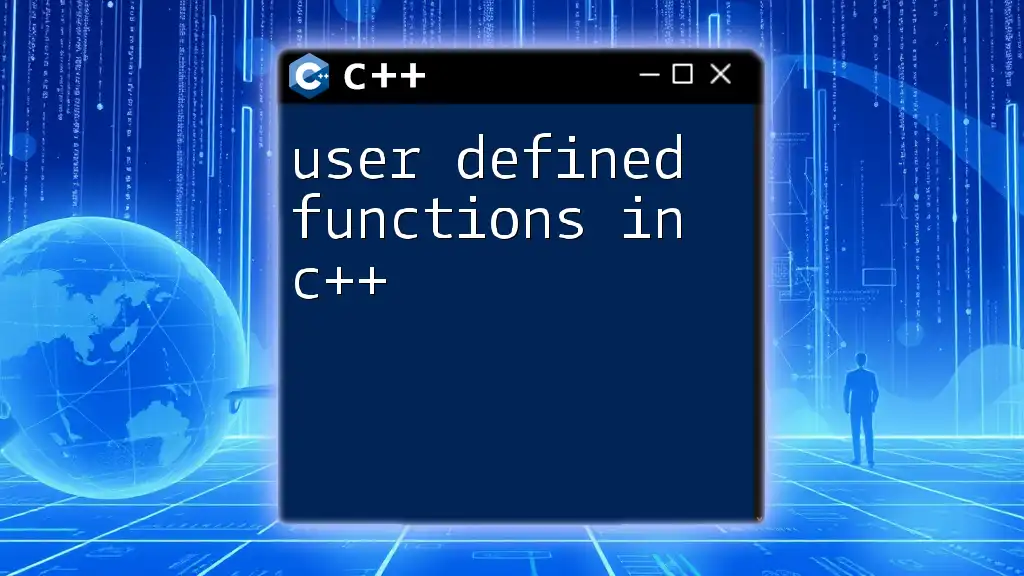
Components of a User-Defined Function
To fully grasp user-defined functions in C++, it's crucial to understand their main components: function declaration, function definition, and function call.
Function Declaration
The function declaration is where we specify the return type, name, and parameters. For instance, the syntax looks like this:
return_type function_name(parameters);
For example, let’s declare a function that adds two integers:
int add(int a, int b); // Declaration of a function named add
Function Definition
Following the declaration, we must define the function, where we implement its behavior. The syntax remains similar, but we include the function body:
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
Function Call
Finally, to utilize the function, we perform a function call. The call executes the function with specified arguments:
int result = add(5, 10); // Calling the add function
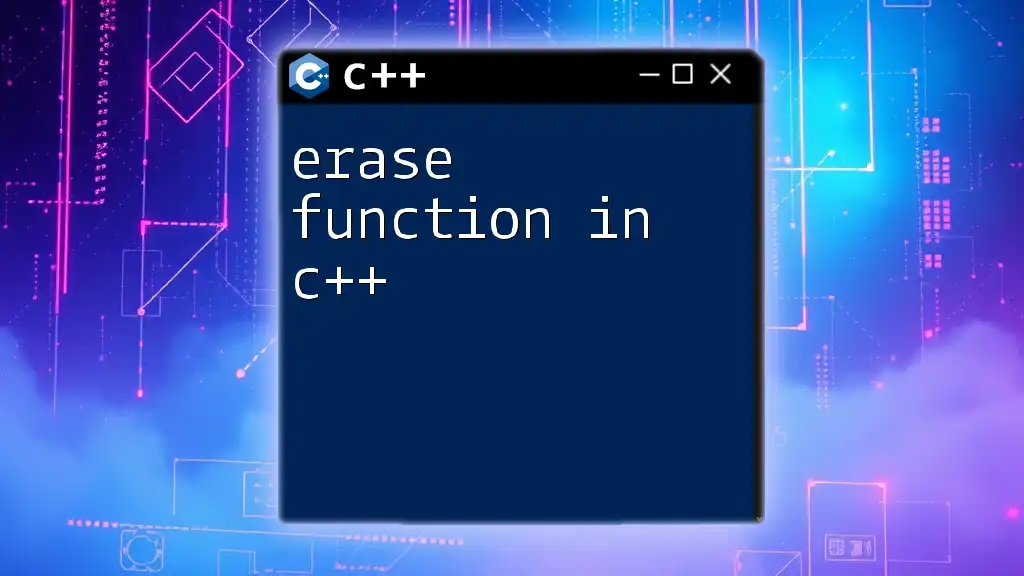
Creating a Simple User-Defined Function
To illustrate user-defined functions more concretely, let’s walk through an example: creating a function to calculate the factorial of a number.
Step 1: Function Declaration
int factorial(int n);
Step 2: Function Definition
Now, let’s define the function to calculate factorial recursively:
int factorial(int n) {
if (n <= 1)
return 1; // Base case
else
return n * factorial(n - 1); // Recursive call
}
Step 3: Function Call
Here’s how we can call this function in our main program:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a positive integer: ";
cin >> num;
cout << "Factorial of " << num << " is " << factorial(num) << endl;
return 0;
}
In this complete example, when a user inputs a positive integer, the program will compute and display its factorial using the user-defined function.
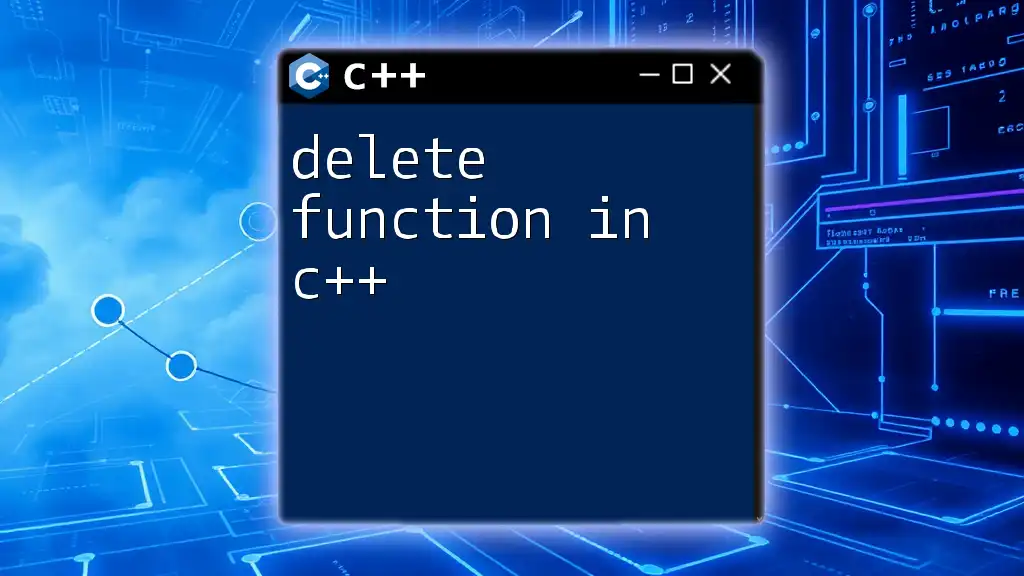
Types of User-Defined Functions
Void Functions
Void functions do not return any value. Such functions perform actions but do not send anything back to the caller. An example is:
void printMessage() {
cout << "Hello, World!" << endl; // Does not return a value
}
Functions with Return Type
On the other hand, functions with a return type provide a value back to the calling code. The earlier `add` function serves as an excellent example, as it returns the sum of two integers.
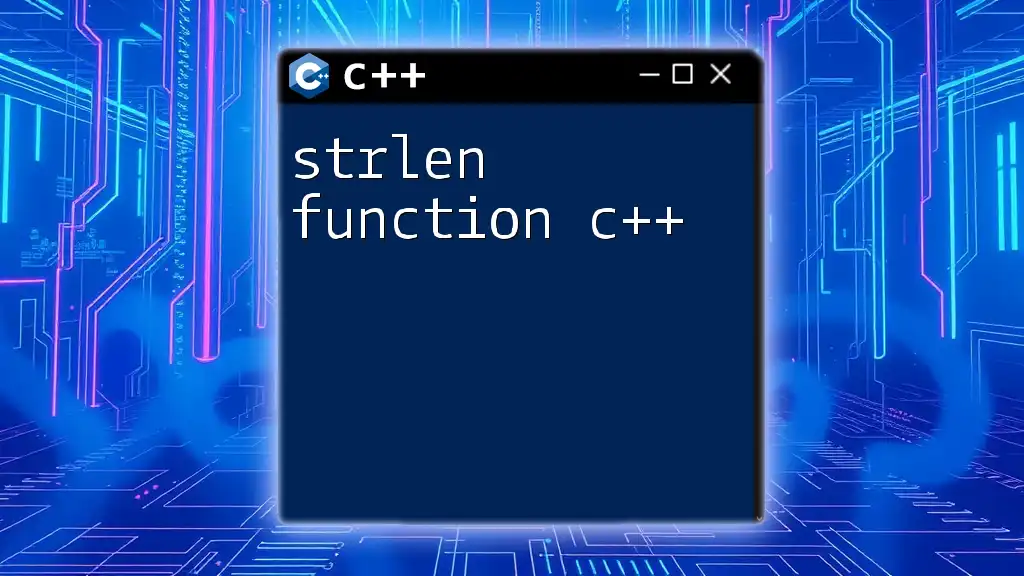
Passing Arguments to Functions
When calling functions, we can pass arguments in two primary ways: pass by value and pass by reference.
Pass by Value
In passing by value, a copy of the argument is made. Thus, any modifications to the parameter in the function do not affect the original variable. Consider the following:
void modifyValue(int x) {
x = 10; // Modifies only the local copy
}
If we pass a variable to this function, changing `x` will not change the original variable outside the function scope.
Pass by Reference
In contrast, passing by reference allows changes to affect the original variable. This is achieved by using a reference parameter:
void modifyValue(int &x) {
x = 10; // Modifies the original variable
}
Here, if we pass a variable to this function, it alters the initial variable even outside the function.
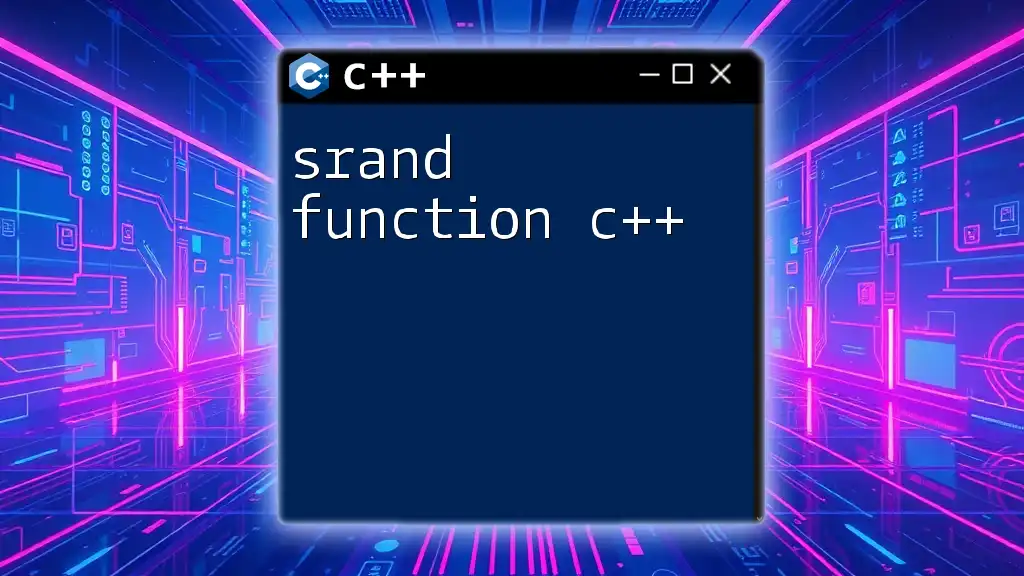
Function Overloading
Function overloading is a powerful feature in C++. It allows us to create multiple functions with the same name, as long as their parameter types or counts differ. This can greatly enhance code readability and flexibility.
For example, we can overload the `add` function to handle both integers and doubles:
int add(int a, int b);
double add(double a, double b);
Here, the compiler determines which function to call based on the argument types.
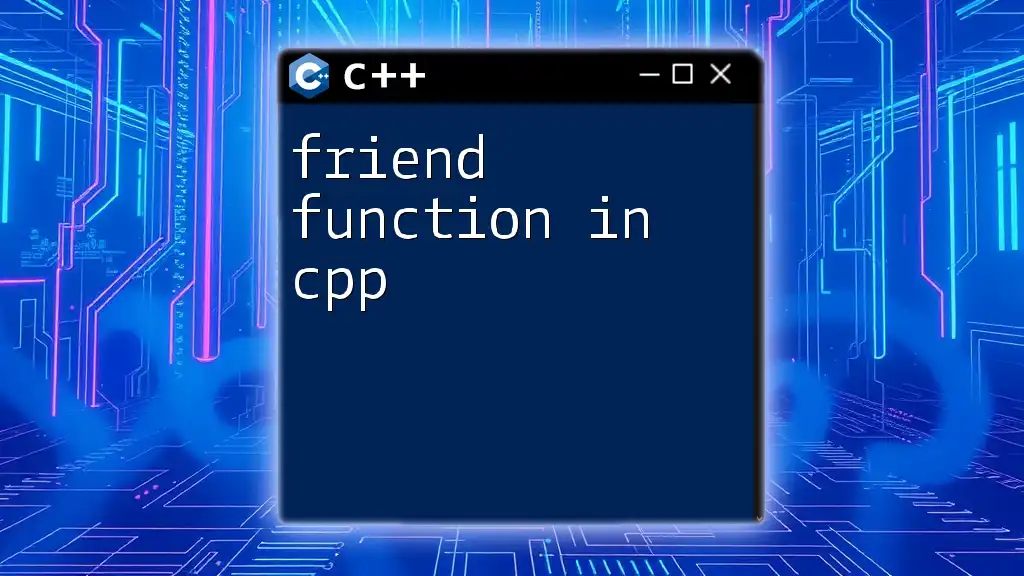
Recursion in Functions
Recursion is a concept where a function calls itself to solve a problem. Understanding recursion is essential for problems that can be defined in terms of smaller subproblems, such as calculating Fibonacci numbers.
Here’s how a recursive Fibonacci function looks:
int fibonacci(int n) {
if (n <= 1) return n; // Base case
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive calls
}
In this example, the function continues to call itself until it reaches the base case, enabling it to calculate Fibonacci numbers effectively.
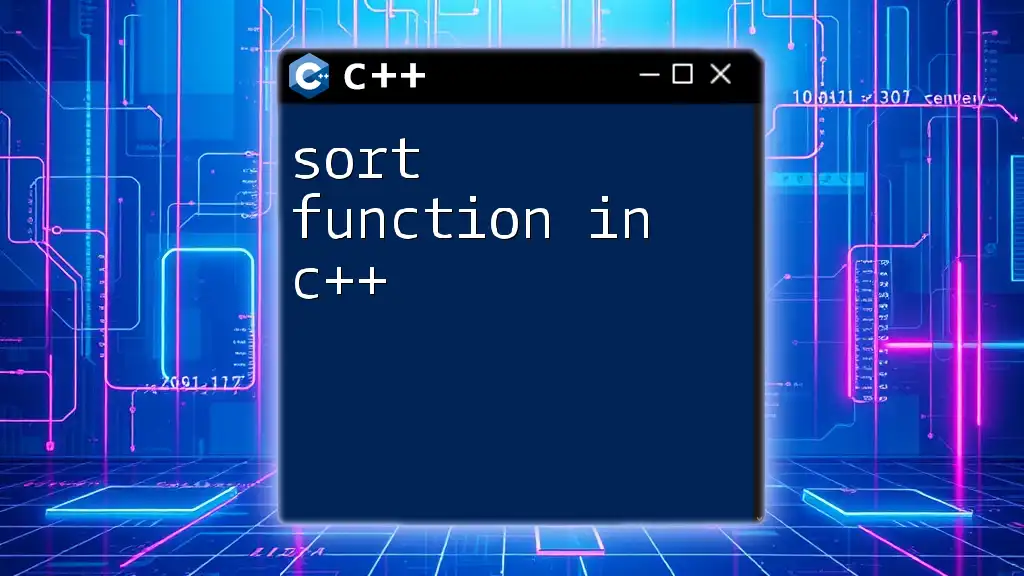
Best Practices for User-Defined Functions
To ensure that your user-defined functions are effective and maintainable, adhere to some best practices:
-
Keep Functions Focused: Each function should have a single responsibility. This focus makes functions easier to read and test. A function handling multiple tasks can become convoluted.
-
Use Meaningful Names: Naming functions descriptively is vital. It should be clear what each function does, enhancing code readability. For instance, prefer `calculateArea()` over a vague name like `doThing()`.
-
Document Your Functions: Use comments to explain the purpose and usage of your functions. A well-documented function is easier for others (and future you) to understand.
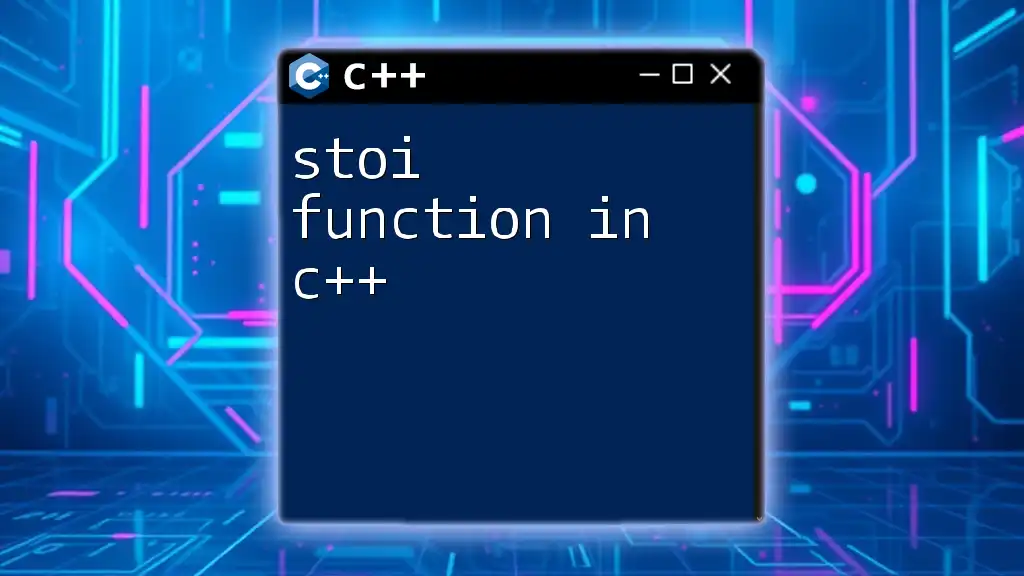
Conclusion
User-defined functions in C++ are a crucial aspect of software development that promotes modularity, reusability, and clarity. Understanding how to declare, define, and call functions, as well as best practices such as readability and documentation, equips programmers with essential skills. By incorporating user-defined functions into your code, you can significantly enhance its structure and maintainability, ultimately leading to more efficient programming experiences. Happy coding!
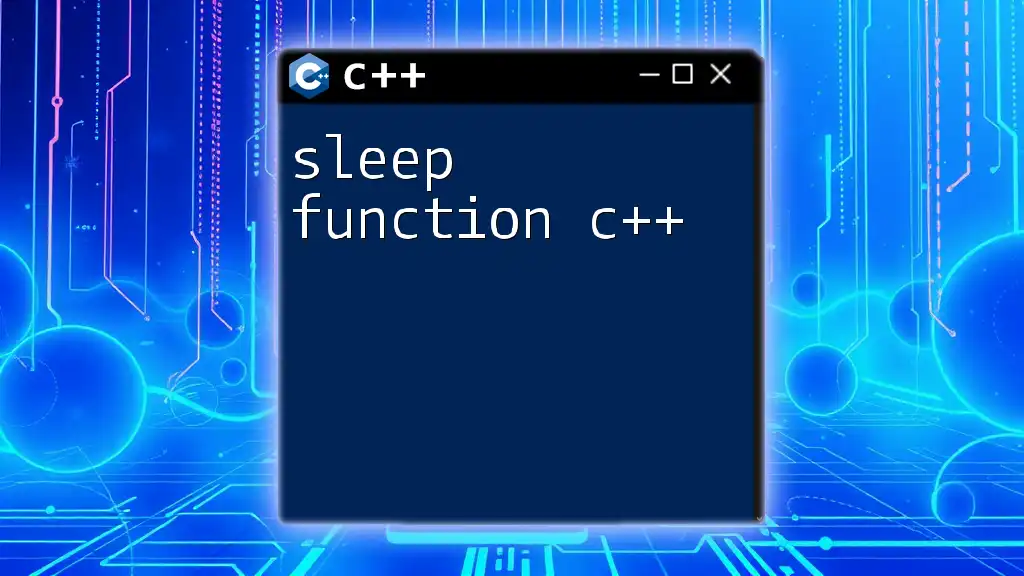
Additional Resources
To further explore user-defined functions in C++, consider referencing reputable books and online tutorials. Engaging with community forums can also provide support and insights as you continue to develop your C++ proficiency.