User-defined functions in C++ allow programmers to create reusable blocks of code that perform specific tasks, defined by the user, enhancing code modularity and organization.
#include <iostream>
using namespace std;
// User-defined function
int add(int a, int b) {
return a + b;
}
int main() {
cout << "The sum is: " << add(5, 3) << endl; // Output: The sum is: 8
return 0;
}
What Are User Defined Functions?
User defined functions are custom functions that programmers create to perform specific tasks within their C++ programs. These functions allow for code modularization, enabling developers to break down complex problems into simpler, manageable pieces. By defining functions tailored to the program's needs, developers can leverage reuse and maintainability, fostering an organized coding culture.
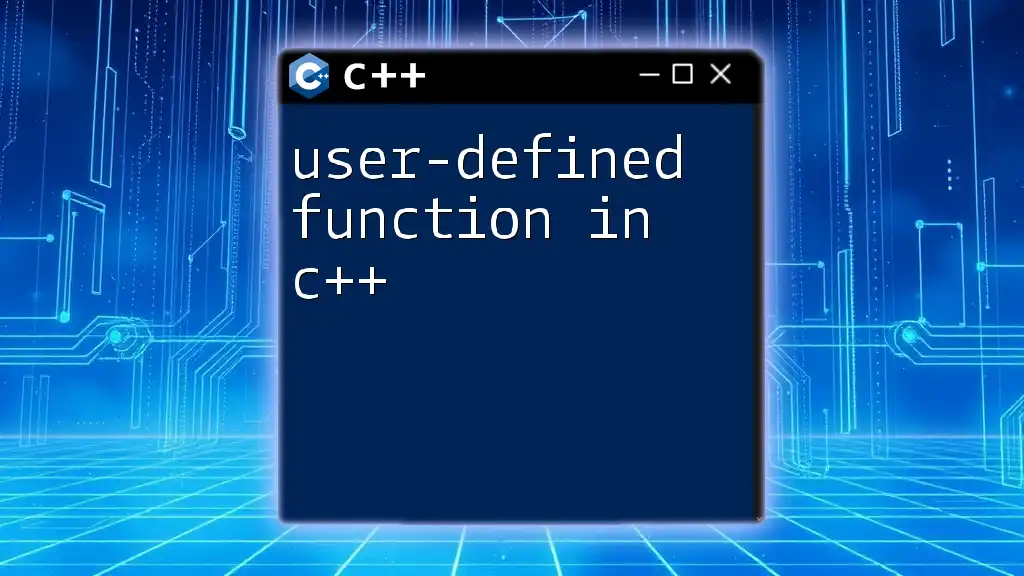
Why Use User Defined Functions?
Utilizing user defined functions offers multiple advantages:
- Modularity: Functions segregate code into isolated sections, which can be developed and tested independently.
- Code Reusability: Once a function is written, it can be used multiple times throughout the program, reducing redundancy and potential errors.
- Improved Readability: A well-structured function with a clear purpose and meaningful name enhances the readability of the code, enabling others (or yourself in the future) to understand it with ease.
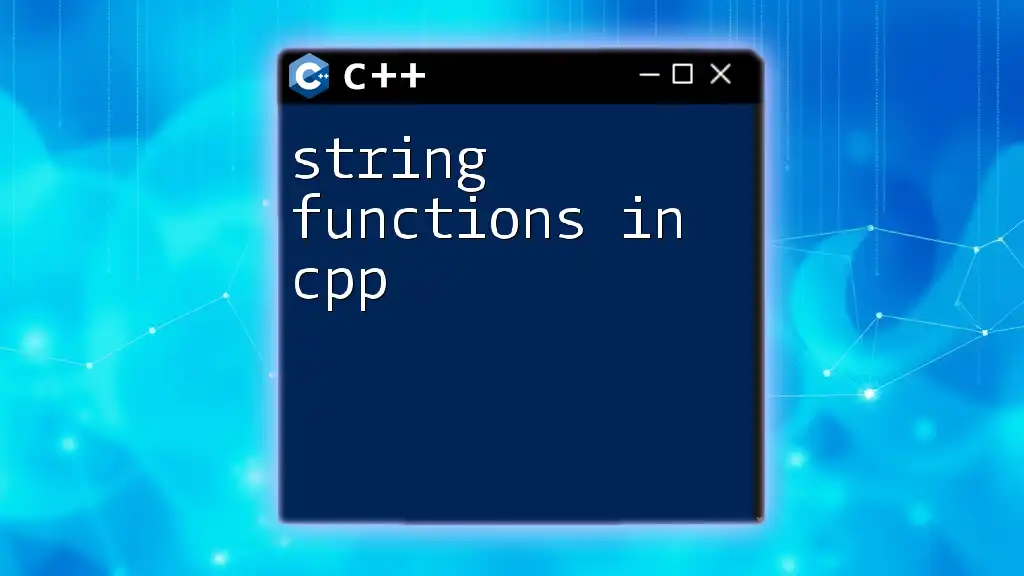
Components of a Function
Function Declaration
Every function in C++ starts with a declaration, which informs the compiler about the function's name, return type, and parameters. The syntax for declaring a function is as follows:
return_type function_name(parameter_type parameter_name);
For instance:
int add(int a, int b);
In this example, `add` is declared as a function that takes two integers and returns their sum as an integer.
Function Definition
After declaring a function, you must define its behavior. The function definition includes the function body where you specify what the function does. The general syntax is:
return_type function_name(parameter_type parameter_name) {
// Function body
}
Example of a function definition:
int add(int a, int b) {
return a + b;
}
Function Call
To execute a function, you need to make a function call. This is done by using the function's name followed by parentheses containing any necessary arguments.
Example of calling the `add` function:
int result = add(5, 3);
In this case, `5` and `3` are passed to the `add` function, and the result is stored in `result`.
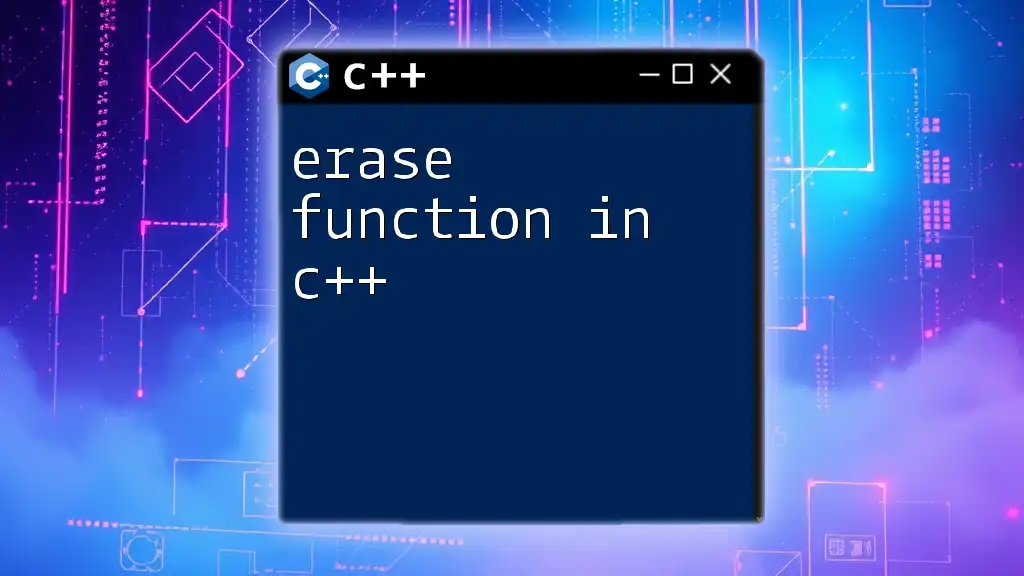
Types of Functions
Value-returning Functions
These functions return a value after execution. The basic structure involves a return statement within the function body.
Example:
int add(int a, int b) {
return a + b;
}
In this code, a function named `add` computes the sum of two integers and returns it.
Void Functions
Void functions are those that do not return any value. They perform an operation but provide no output back to the caller.
Example:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
When `greet` is called, it simply displays "Hello, World!" on the screen.
Functions with Parameters
Functions can also accept arguments, which are inputs passed to the function.
Example:
void display(int num) {
std::cout << "Number: " << num << std::endl;
}
In the above case, the `display` function takes an integer parameter and displays it.
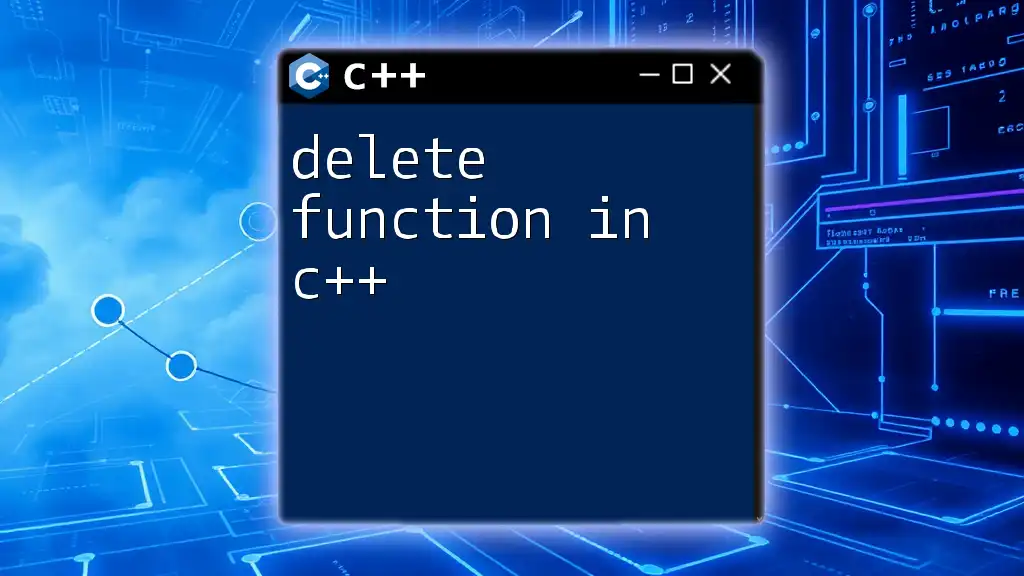
Function Overloading
What is Function Overloading?
Function overloading allows you to define multiple functions with the same name but different parameter types or numbers. C++ distinguishes which function to execute based on the number or type of arguments used in the function call.
Example of Function Overloading
void display(int num) {
std::cout << "Integer: " << num << std::endl;
}
void display(double num) {
std::cout << "Double: " << num << std::endl;
}
In this example, the `display` function is overloaded to handle both integers and doubles, showcasing the flexibility of user defined functions.
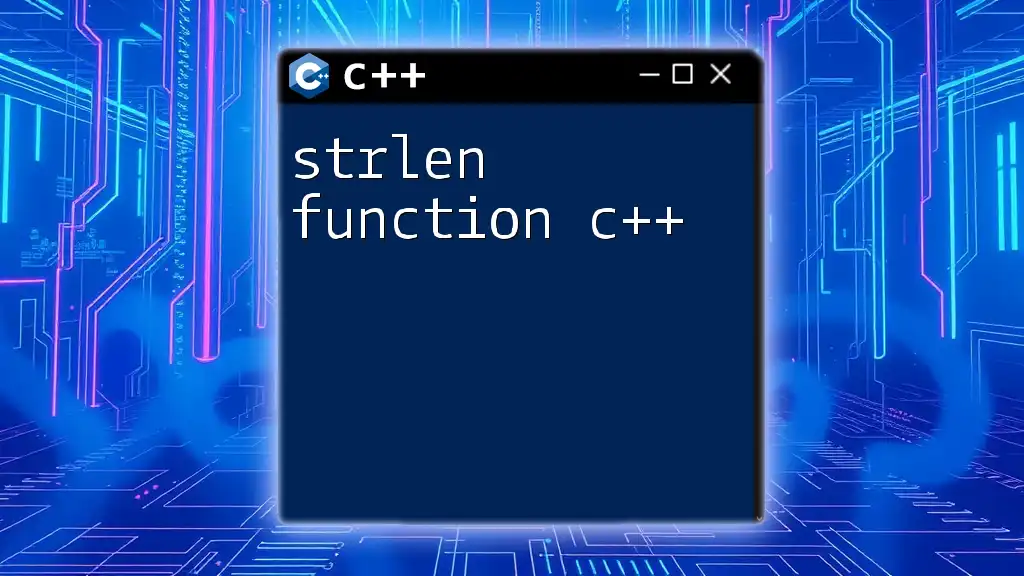
Default Arguments
What Are Default Arguments?
Default arguments allow you to initialize parameters of a function with default values. When an argument is not provided during a function call, the predefined default value is used.
Example of Default Arguments
void display(int num = 42) {
std::cout << "Number: " << num << std::endl;
}
In this case, calling `display()` without an argument will output "Number: 42."
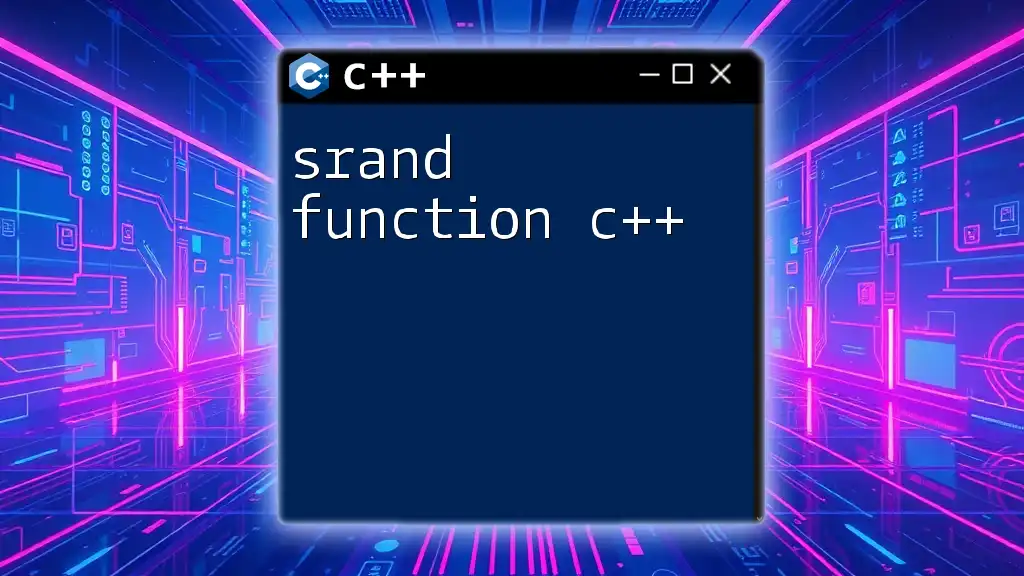
Inline Functions
Understanding Inline Functions
Inline functions can be defined using the `inline` keyword, suggesting to the compiler that it should insert the function's body directly instead of making a traditional function call. This saves the overhead of function calls, potentially improving execution speed but at the risk of increased binary size.
Example of Inline Function
inline int square(int x) { return x * x; }
Utilizing `square(5)` computes the square of `5` by inline substitution, enhancing performance for frequently called simple functions.
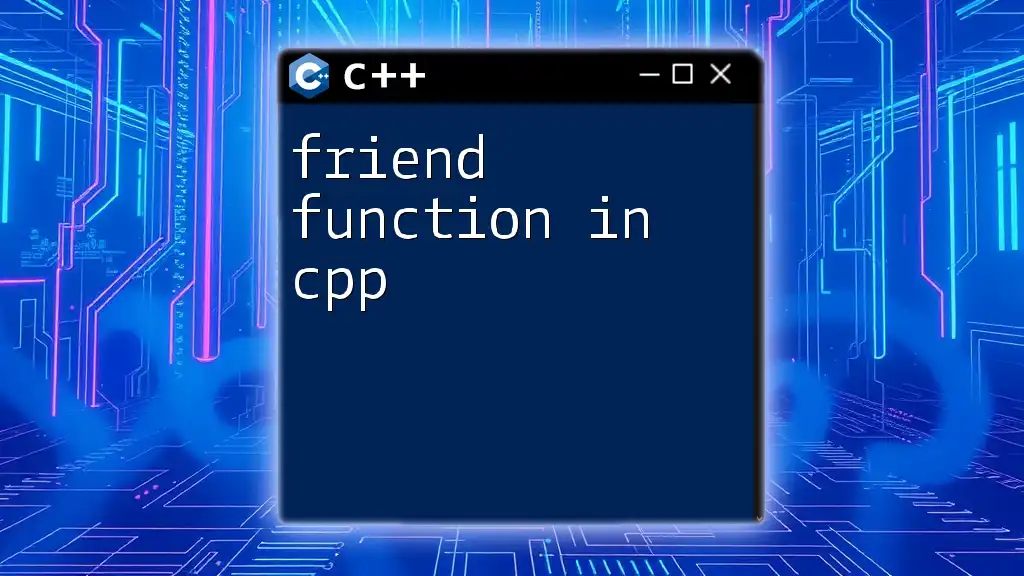
Recursion
What is Recursion?
A recursive function is one that calls itself to solve a problem. This process continues until a base condition is met. It is particularly useful for problems naturally defined in terms of smaller subproblems, such as calculating factorials or Fibonacci numbers.
Example of Recursive Function
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
In this example, the `factorial` function calls itself, breaking down the problem until reaching the base case at `n <= 1`.
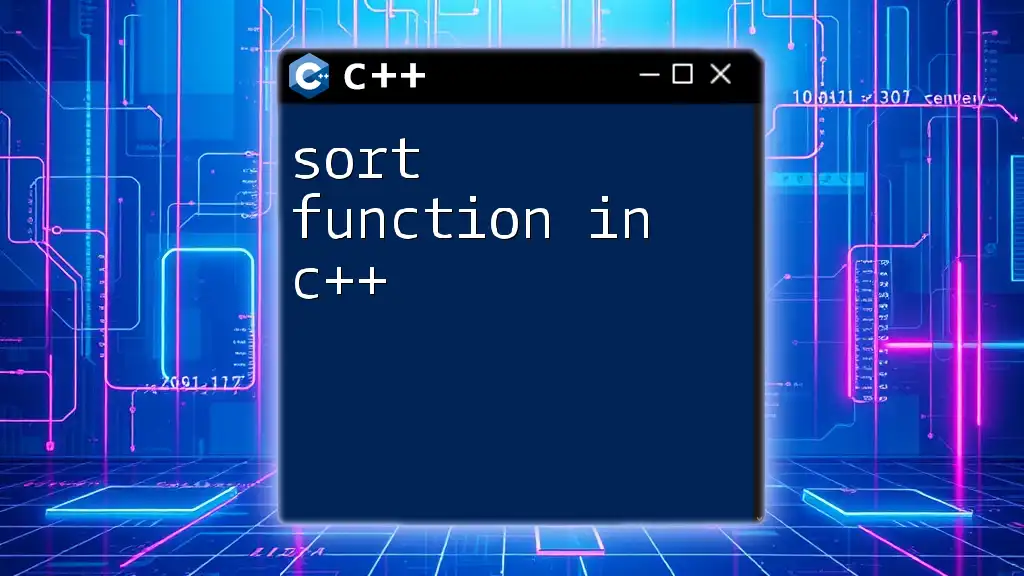
Best Practices for User Defined Functions
Keep Functions Short and Focused
Aim to design functions with a single responsibility. Avoid creating lengthy functions that perform multiple operations, as this can lead to confusion and make debugging difficult.
Use Meaningful Names
Function names should be descriptive enough to convey their purpose. Clear naming conventions greatly enhance the maintainability of the code and make it easier for others (or future you) to understand the code.
Comment Your Code
Adding comments within your functions, especially for complex code, aids in clarifying what each part of the function accomplishes. This practice is essential for future reference and collaborative projects.
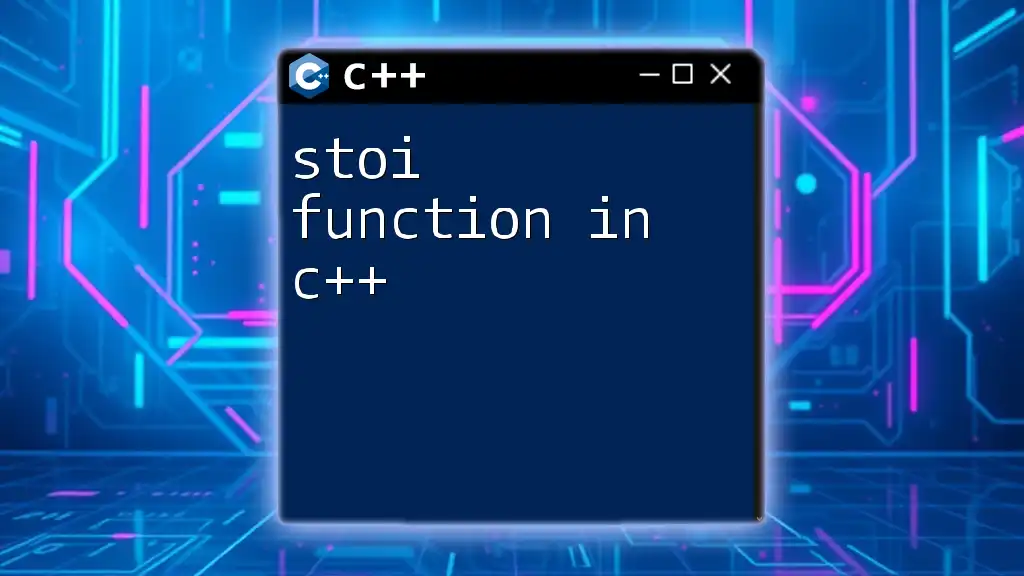
Conclusion
User defined functions in C++ provide key advantages, including better structure, enhanced readability, and increased reusability. By mastering the art of defining and utilizing functions, you’ll harness the full potential of C++, making your code more efficient and manageable. Remember to keep experimenting with writing your own functions to become proficient in their application.
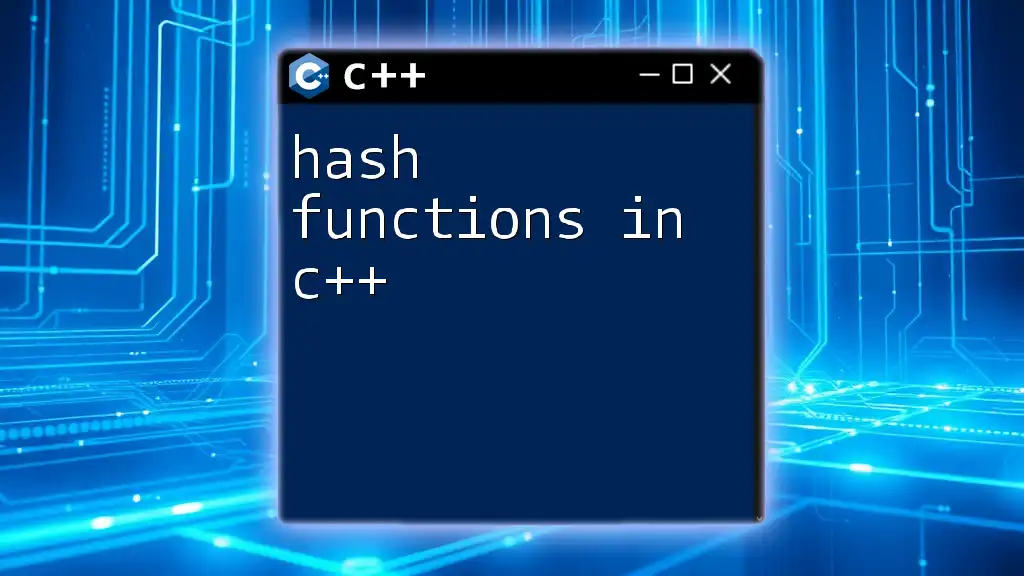
Additional Resources
For those eager to dive deeper into user defined functions, consider exploring classic C++ programming books, engaging with online tutorials, or enrolling in specialized coding courses. Each resource will further strengthen your understanding and skills in manipulating functions within C++.
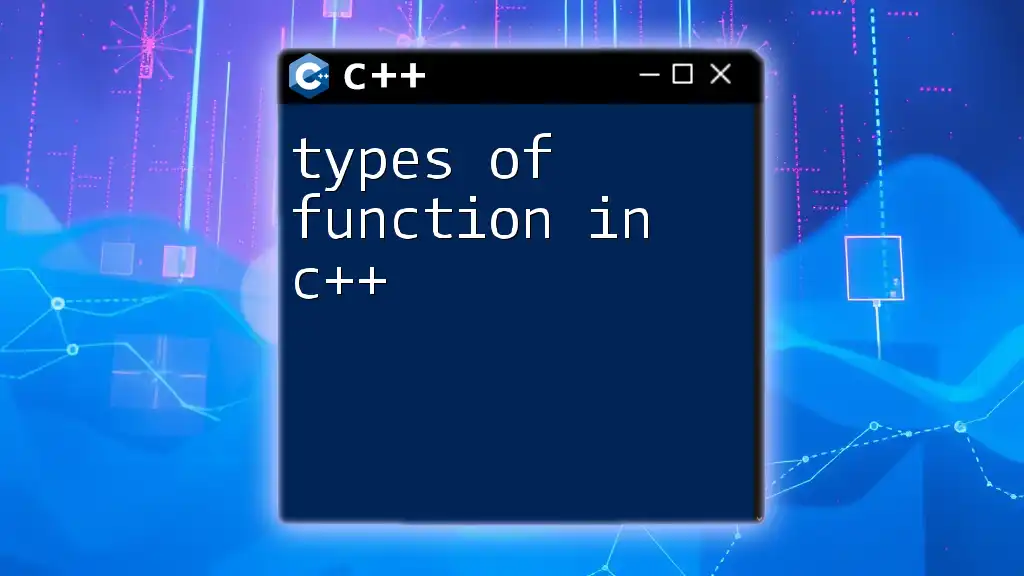
Call to Action
Start practicing today! Experiment with creating, calling, and utilizing user defined functions in your C++ programs. Don't forget to subscribe for updates and more insights into mastering C++. Happy coding!