In C++, a function can be defined using the `return_type function_name(parameters)` syntax, allowing you to encapsulate code for reuse with specified input and output types.
Here's a simple example of a C++ function definition:
int add(int a, int b) {
return a + b;
}
Understanding Functions in C++
The Purpose of Functions
Functions are essential building blocks in C++ programming. They allow programmers to encapsulate code into reusable modules, significantly improving code organization and efficiency. By using functions, one can reduce code redundancy and enhance maintainability, leading to cleaner, more manageable codebases.
Defining a Function
Functions in C++ can be defined or declared using a specific syntax that separates the logical elements of a function. The basic syntax consists of several components:
return_type function_name(parameter_list) {
// function body
}
- Return type indicates what type of value the function will return (e.g., `int`, `float`, `void`).
- Function name is a unique identifier for the function.
- Parameter list is optional, consisting of values that the function can receive.
- The function body contains the code that performs the function's task.
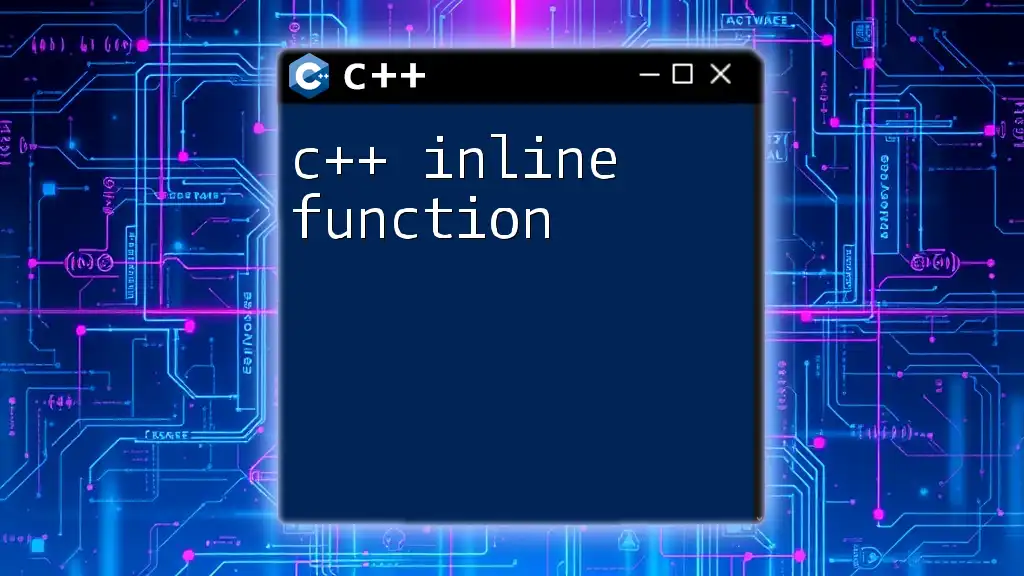
Components of a C++ Function
Return Type
The return type specifies the type of value the function will produce. Common return types include `int`, `float`, and `void`. For example, a simple function that adds two integers might look like this:
int add(int a, int b) {
return a + b;
}
In this case, `int` is the return type, indicating that the function will return an integer value.
Function Name
The function name should be descriptive, reflecting the action the function performs. This is critical for code readability and maintainability. For example:
void displayMessage() {
std::cout << "Hello, World!" << std::endl;
}
Here, the name `displayMessage` clearly indicates what the function does.
Parameters
Parameters allow functions to receive input values. They are specified in the function definition and can come in various forms, such as required or optional parameters. Here’s an example of a function that takes a `string` parameter:
void greet(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
In this case, the function `greet` requires one argument, `name`, which it uses to generate a greeting message.
Function Body
The function body contains the code that defines what the function does. It is crucial for executing the intended logic. For instance, here's how you would calculate the area of a circle:
void calculateArea(float radius) {
float area = 3.14 * radius * radius;
std::cout << "Area: " << area << std::endl;
}
In this example, the body computes the area using the provided radius.
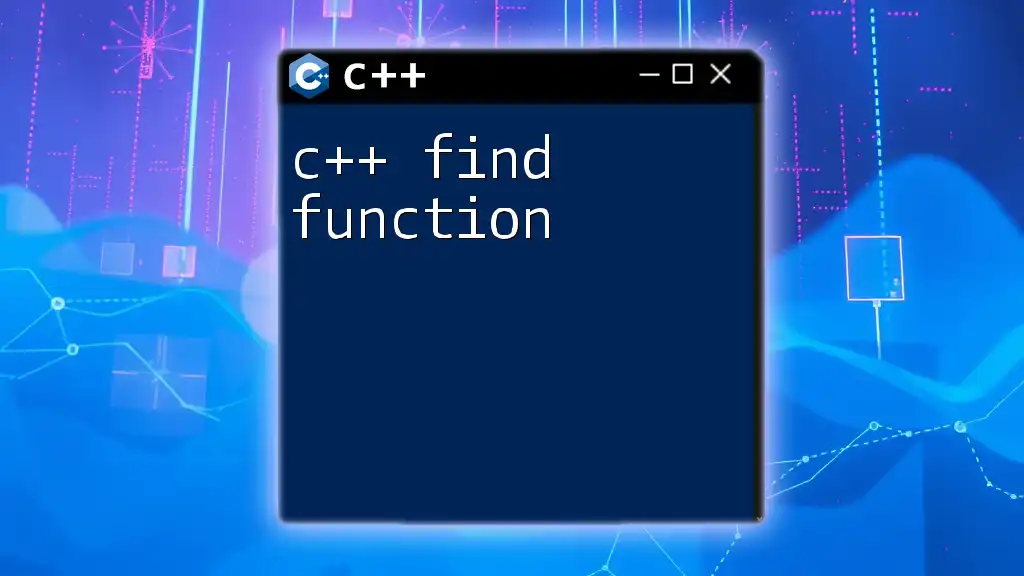
Defining Different Types of Functions
Standard Functions
Standard functions are essential to any program. They receive input and perform actions. Here’s a basic multiplication function:
int multiply(int x, int y) {
return x * y;
}
This function accepts two integers and returns their product.
Function Overloading
C++ supports function overloading, allowing multiple functions to share the same name but have different parameter types or numbers. Here’s how two `add` functions can coexist:
int add(int a, int b) {
return a + b;
}
float add(float a, float b) {
return a + b;
}
The C++ compiler can differentiate between these functions based on the argument types that are passed when they are called.
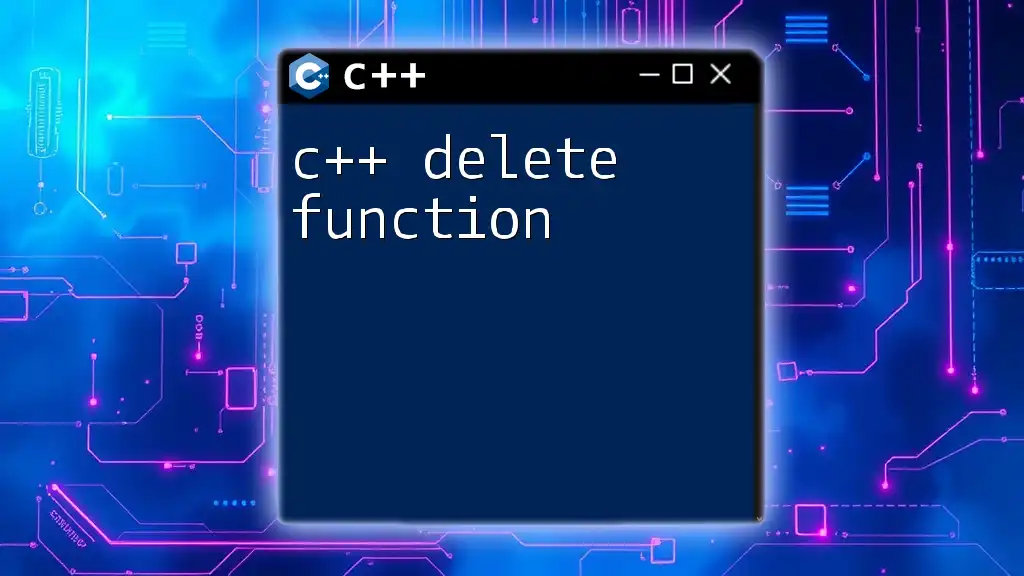
Calling Functions in C++
How to Call a Function
To use a function, it must be called in your code. The syntax for calling a function involves specifying the function name along with any required arguments, if applicable. For example:
int result = add(5, 10);
This line calls the `add` function with arguments `5` and `10`, storing the return value in the variable `result`.
Passing Arguments
When calling functions, you can pass arguments in two primary ways: pass by value and pass by reference.
- Pass by value: A copy of the actual parameter is made, and changes to the parameter within the function do not affect the original variable.
void passByValue(int x) {
x = x + 10; // This change does not affect the passed argument.
}
- Pass by reference: The function receives a reference to the actual parameter, allowing modifications to affect the original variable.
void passByReference(int& x) {
x = x + 10; // This change affects the original argument.
}
Choosing the appropriate method depends on the desired functionality.
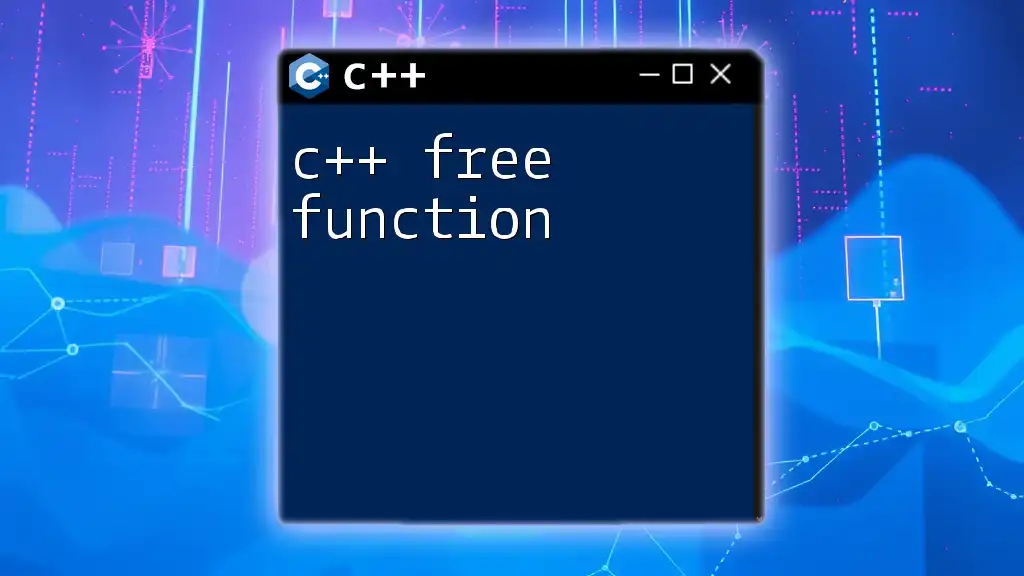
Scope and Lifetime of Functions
Local vs Global Functions
Understanding function scope is vital. Local functions are defined within another function and are only accessible within that function's context. Global functions, by contrast, can be called from anywhere in the program.
void localFunction() {
int y = 5; // 'y' is local to this function
}
Static Functions
Static functions in C++ retain their value between function calls. This is useful for tracking the number of calls or maintaining state.
static int countCalls() {
static int count = 0;
return ++count; // Increments count each time the function is called
}
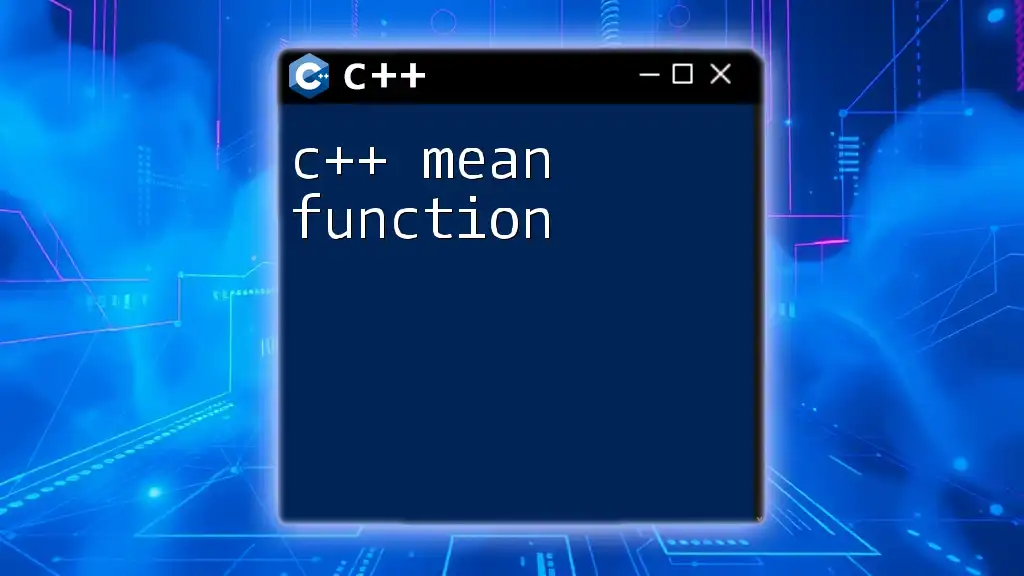
Best Practices for Defining Functions
Naming Conventions
Effective naming is critical. A good function name should be descriptive and convey its purpose clearly. Utilize camelCase or snake_case consistently for readability.
Function Documentation
Documenting your functions helps others (and your future self) understand their purpose and behavior. Comments should include the function's purpose, parameters, and return values.
// This function adds two integers and returns the result.
int add(int a, int b);
Clear documentation is a hallmark of professional code.
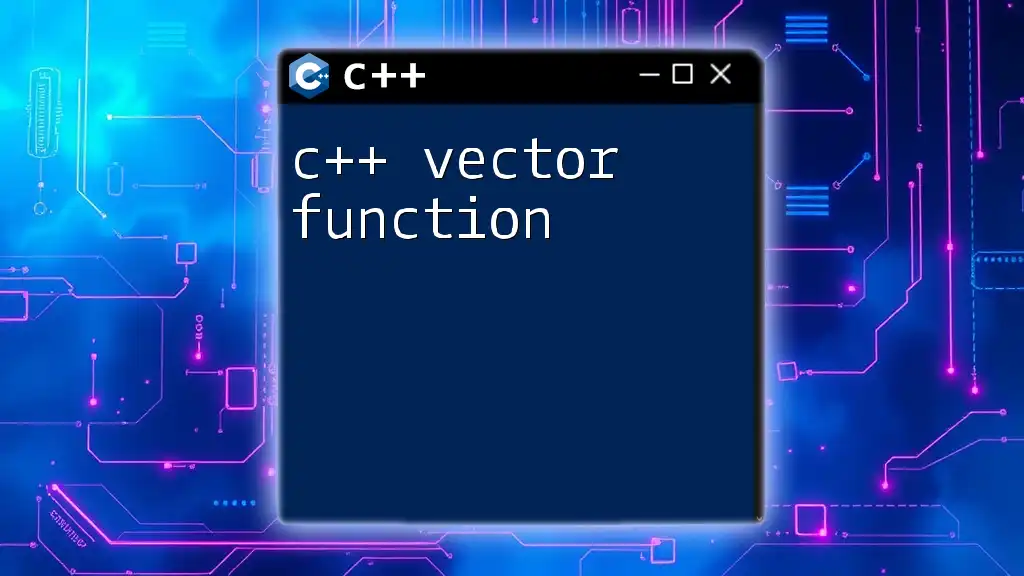
Conclusion
Defining functions in C++ is a fundamental skill that enhances programming efficiency and clarity. By mastering the art of function definition—including their components, various types, and best practices—you'll be well-equipped to write clean and maintainable code. Practicing how to define and utilize functions is crucial for both beginners and advanced programmers alike. For those eager to dive deeper into the realm of C++, countless resources await your exploration.