C++ vectors are dynamic arrays that can resize themselves automatically, allowing for efficient management of collections of data.
Here’s a simple example of using a C++ vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding the Structure of a Vector
Vector Declaration and Initialization
A vector in C++ can be declared using the `std::vector` class provided in the Standard Template Library (STL). The syntax for declaring a vector is straightforward and follows this pattern:
std::vector<int> myVector; // declares an empty vector of integers
You can also initialize vectors during declaration. There are various methods of initialization:
std::vector<int> myVector1; // empty vector
std::vector<int> myVector2(10); // vector with 10 elements initialized to 0
std::vector<int> myVector3{1, 2, 3, 4, 5}; // initialized with specific values
Each of these methods serves its purpose depending on your needs, whether you want an empty vector, a vector with predefined size, or a vector with specific contents.
Memory Management in Vectors
Vectors manage their memory dynamically, meaning they can grow or shrink during runtime. Understanding capacity and size is crucial here. The size of a vector refers to the number of elements currently stored in it, while the capacity indicates how much memory has been allocated for the vector.
To check the size and capacity, you can use:
std::cout << "Size: " << myVector.size() << std::endl;
std::cout << "Capacity: " << myVector.capacity() << std::endl;
This shows you how many elements are in the vector and how much space has been allocated for future elements, helping you to manage your memory usage more efficiently.
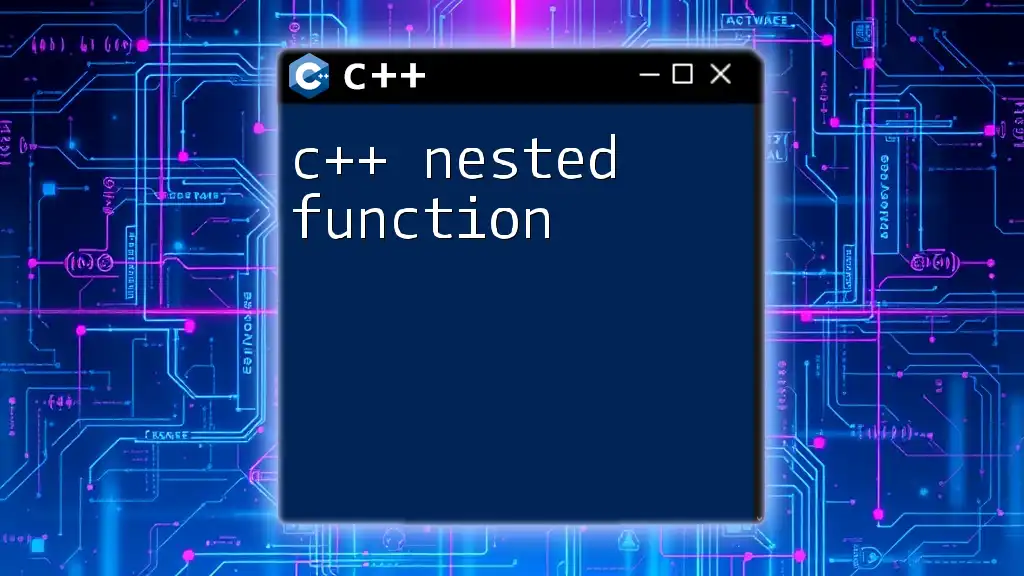
Key Functions of C++ Vectors
Access Functions
`at() Function`
The `at()` function provides safe access to elements in a vector. If the index is out of range, it throws an out-of-range exception. This makes it safer than using the `operator[]`.
Example:
try {
std::cout << myVector.at(2) << std::endl; // prints the element at index 2
} catch (std::out_of_range& e) {
std::cerr << e.what() << std::endl; // handles out-of-range access
}
`operator[]`
On the other hand, the `operator[]` does not check bounds. While it is faster, it's important to ensure that the index provided is valid.
Example:
std::cout << myVector[2] << std::endl; // prints the element at index 2
`front()` and `back()`
The `front()` and `back()` functions are used to access the first and last elements of the vector, respectively.
Example:
std::cout << "First element: " << myVector.front() << std::endl; // prints the first element
std::cout << "Last element: " << myVector.back() << std::endl; // prints the last element
Modifier Functions
`push_back()`
The `push_back()` function adds an element to the end of the vector. It automatically resizes the vector as needed.
Example:
myVector.push_back(10); // Adds 10 to the end of the vector
`pop_back()`
The `pop_back()` function removes the last element from the vector, shrinking its size.
Example:
myVector.pop_back(); // Removes the last element
`insert()`
To insert elements in the middle of a vector, you can use the `insert()` method. This also automatically manages resizing.
Example:
myVector.insert(myVector.begin() + 1, 20); // Adds 20 at index 1
`erase()`
Use the `erase()` function to remove elements at specific positions.
Example:
myVector.erase(myVector.begin() + 1); // Removes the element at index 1
`clear()`
The `clear()` function is used to remove all elements from the vector, effectively emptying it.
Example:
myVector.clear(); // Clears all elements
Capacity Management
`resize()`
The `resize()` method changes the size of the vector. If the new size is greater, it's filled with default-constructed values.
Example:
myVector.resize(5); // Resizes the vector to contain 5 elements
`reserve()`
If you anticipate needing more space, `reserve()` allocates memory without changing the size, which can enhance performance.
Example:
myVector.reserve(100); // Reserves memory for 100 elements
`shrink_to_fit()`
This function reduces the capacity of the vector to fit its current size, potentially freeing up unused memory.
Example:
myVector.shrink_to_fit(); // Adjusts capacity to match size
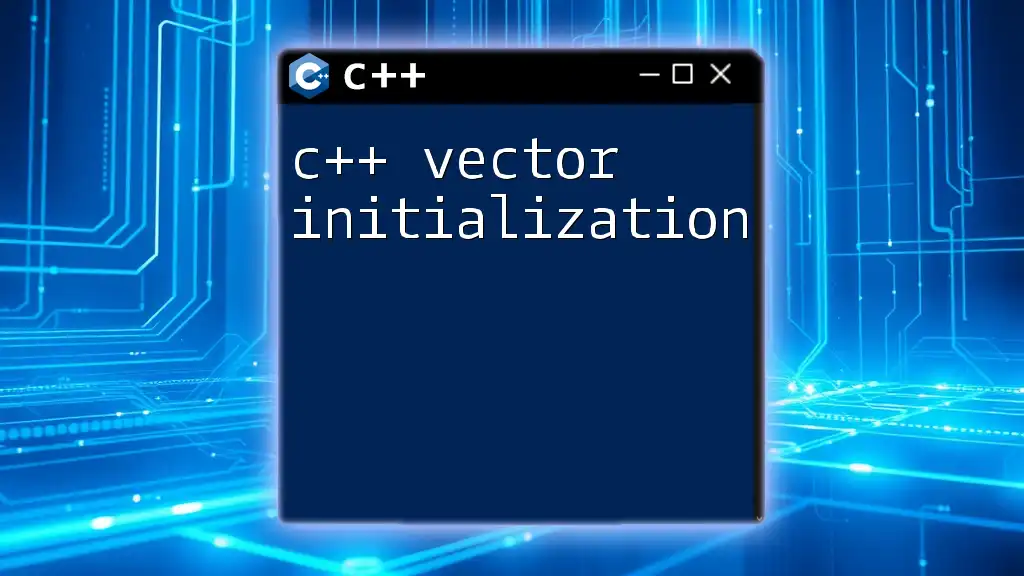
Advanced Vector Functions
Sorting and Searching in Vectors
Vectors often require sorting or searching. The STL provides powerful algorithms for such tasks.
Using `std::sort()`
Sorting a vector is easy with `std::sort()`. To sort a vector of integers, you would do:
#include <algorithm>
std::sort(myVector.begin(), myVector.end()); // Sorts myVector in ascending order
Using `std::find()`
To search for an element, you can use `std::find()`. This function examines elements in the vector to find the target.
Example:
auto it = std::find(myVector.begin(), myVector.end(), 20);
if (it != myVector.end()) {
std::cout << "Element found at index: " << std::distance(myVector.begin(), it) << std::endl;
}
Iterators with Vectors
Iterator functionality in vectors is crucial for effectively traversing or manipulating the elements. The `begin()` and `end()` functions provide iterators to the start and the end of the vector, respectively.
You can iterate over a vector using:
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
std::cout << *it << " "; // Dereferencing the iterator to get the element
}
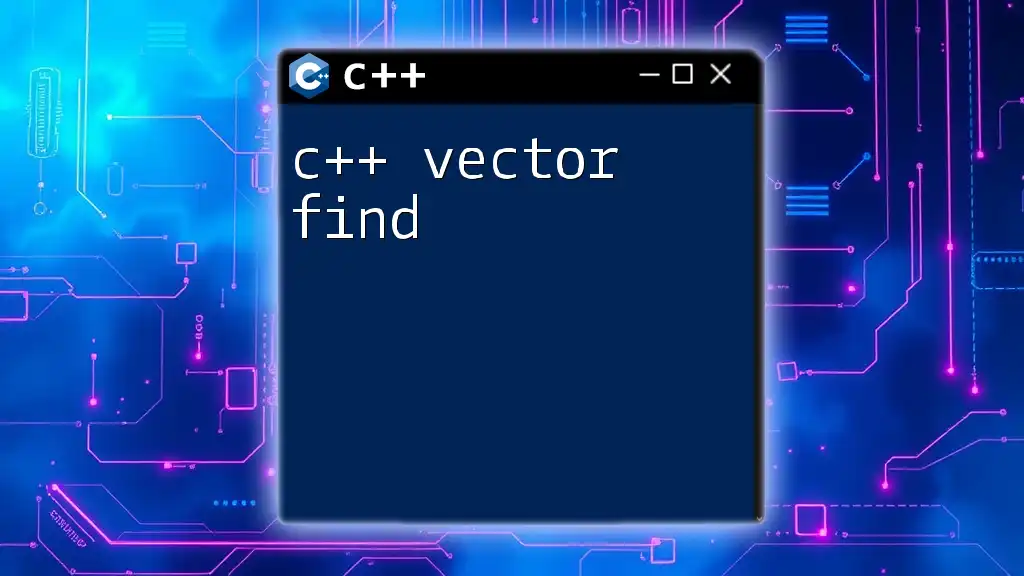
Common Use Cases of Vectors
Vectors of Vectors
A common pattern in C++ is the use of a vector of vectors, which can represent multi-dimensional data structures, like matrices.
Example of creating a 2D vector:
std::vector<std::vector<int>> matrix(3, std::vector<int>(3, 0)); // 3x3 matrix initialized to 0
matrix[1][1] = 5; // Sets the element at the second row second column to 5
Use Cases in Algorithms
Vectors simplify various complex data structures in algorithms. For example, they can be employed to represent graphs, where each vector can hold a list of edges.
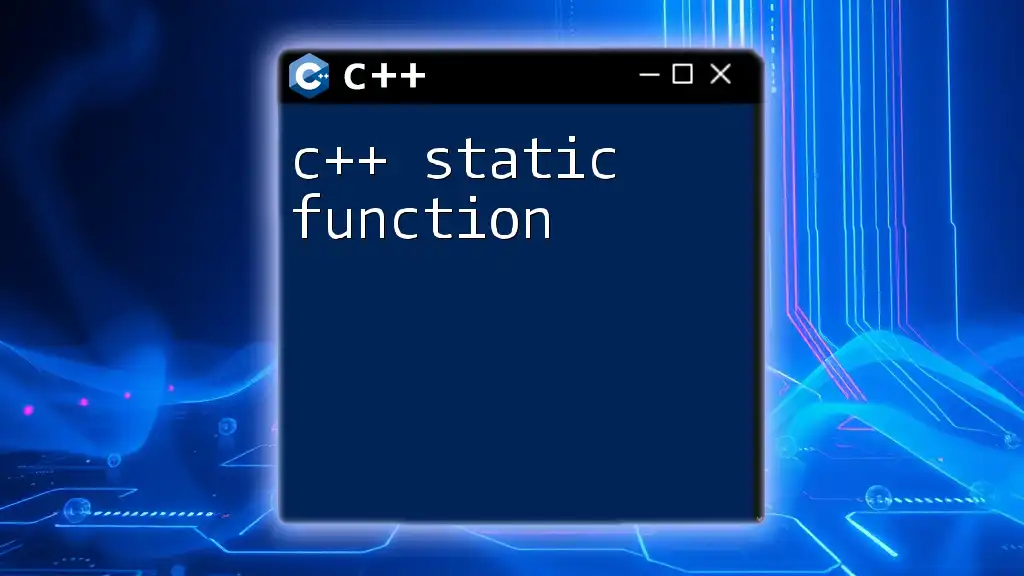
Best Practices for Using Vectors
Understanding when to use vectors versus arrays is an important decision in C++. Generally speaking, vectors are preferred due to their dynamic sizing and ease of management. Arrays have a fixed size and require more manual memory management, making them less flexible.
Memory Management Tips
To optimize vector usage and avoid memory overhead, you can use `reserve()` to preallocate memory when needed. This approach minimizes resizing operations that can slow down your program.
Performance Considerations
Resizing vectors or copying large vectors can have performance implications. When performance is critical, consider using `shrink_to_fit()` after removing elements or using `reserve()` upfront when possible.
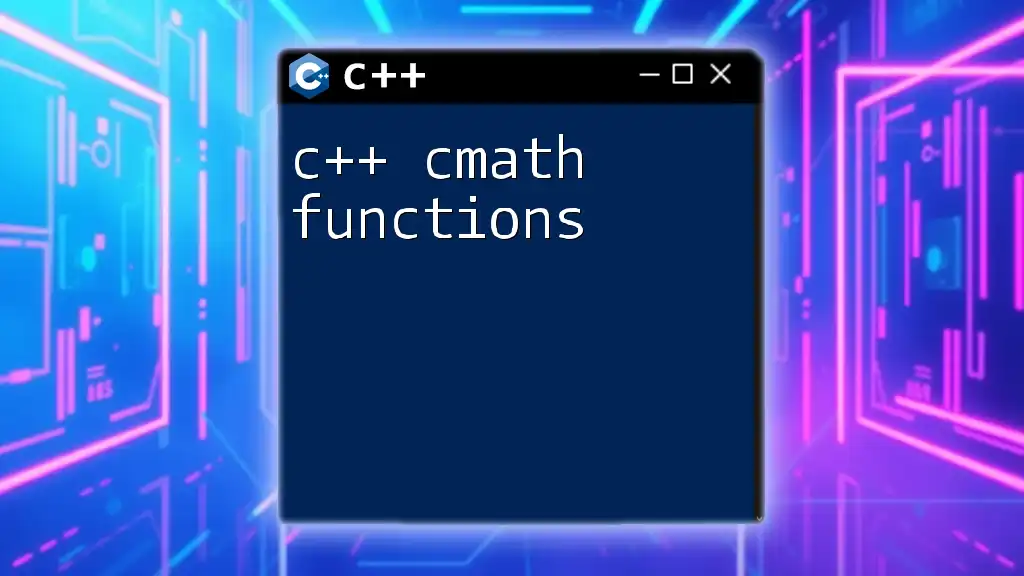
Conclusion
In this guide, we've explored the C++ vector function, covering everything from its basic structure and key functionalities to advanced topics such as sorting, searching, and complex use cases. Understanding vectors empowers you to manage dynamic collections efficiently and opens the door to writing more elegant and performant C++ code. Practice using vectors and their functions, and consider joining our community for more tips and resources to enhance your C++ programming journey.