C++ vectors are dynamic arrays that allow for efficient element insertion, deletion, and access while automatically managing memory.
Here is a simple example demonstrating basic vector operations:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec; // Initialize a vector
// Adding elements
vec.push_back(10);
vec.push_back(20);
vec.push_back(30);
// Accessing elements
std::cout << "First element: " << vec[0] << std::endl;
// Removing the last element
vec.pop_back();
// Size of the vector
std::cout << "Size after pop: " << vec.size() << std::endl;
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a sequence container that encapsulates dynamic size arrays. Unlike traditional arrays, which have a fixed size, vectors can grow and shrink in size as needed, making them a powerful tool in C++ programming. They are part of the Standard Template Library (STL), which provides a range of algorithms and data structures.
Vectors offer several advantages over arrays:
- They automatically manage memory.
- You can easily add or remove elements without having to manually handle memory allocation.
- They provide a rich set of member functions that simplify many operations.
Key Features of the C++ Vector Library
The vector library in C++ provides several key features:
- Dynamic sizing: Vectors can grow as more elements are added, which means you don’t have to define a size upfront.
- Automatic memory management: Vectors handle their own memory allocation and deallocation, reducing the risk of memory leaks.
- Integration with STL: Vectors work seamlessly with the STL algorithms, allowing you to apply various algorithms directly to vector elements.

Common C++ Vector Operations
Creating and Initializing Vectors
Creating a vector is simple. You can use the default constructor or initialize it with specific values:
std::vector<int> numbers; // default constructor
std::vector<int> numbers = {1, 2, 3, 4}; // initialization
The above examples show how to create a vector called `numbers`. The first line creates an empty vector, while the second initializes it with values.
Adding Elements to a Vector
Using `push_back`
One of the most common operations is adding elements to a vector using the `push_back` method. This function appends a new element to the end of the vector.
std::vector<int> numbers;
numbers.push_back(5);
In this example, `5` is added to the end of the vector. This is an efficient way to build a vector at runtime.
Using `emplace_back`
`emplace_back` is similar to `push_back`, but it constructs the object in place rather than copying or moving it. This can lead to performance improvements, especially for complex types.
numbers.emplace_back(10); // constructs in place
Using `emplace_back` can be particularly beneficial when working with objects that require complex construction.
Removing Elements from a Vector
Using `pop_back`
To remove the last element of a vector, you can use the `pop_back` method. This function decreases the vector's size by one.
numbers.pop_back(); // removes the last element
This method is straightforward and efficient since no elements need to be moved.
Using `erase`
The `erase` function allows for the removal of elements from specific positions. This can be useful when you need to remove an element that is not the last in the vector.
numbers.erase(numbers.begin() + 1); // removes the second element
In this example, the second element of the vector is removed, and the remaining elements are shifted accordingly.
Accessing Elements in a Vector
Using the `[]` Operator
You can access individual elements in a vector using the subscript operator:
int firstElement = numbers[0]; // access first element
This method allows direct access, but it does not perform bounds checking, so you need to be careful not to access elements with an out-of-range index.
Using `at()`
For safer access, C++ provides the `at()` function, which checks for valid indices.
int firstElement = numbers.at(0); // safer access
If you try to access an index that is out of range, `at()` will throw an `std::out_of_range` exception, which helps prevent runtime errors.
Checking the Size and Capacity of a Vector
Using `size()`
To get the number of elements in a vector, use the `size()` function.
size_t size = numbers.size(); // returns the number of elements
Knowing the size of a vector is crucial for iterations and ensuring you don’t exceed its bounds.
Using `capacity()`
Vectors have a capacity, which indicates the number of elements they can hold before needing to resize. The `capacity()` method returns the current capacity.
size_t capacity = numbers.capacity(); // returns current capacity
When the size exceeds capacity, the vector reallocates memory to accommodate more elements, which can be an expensive operation.
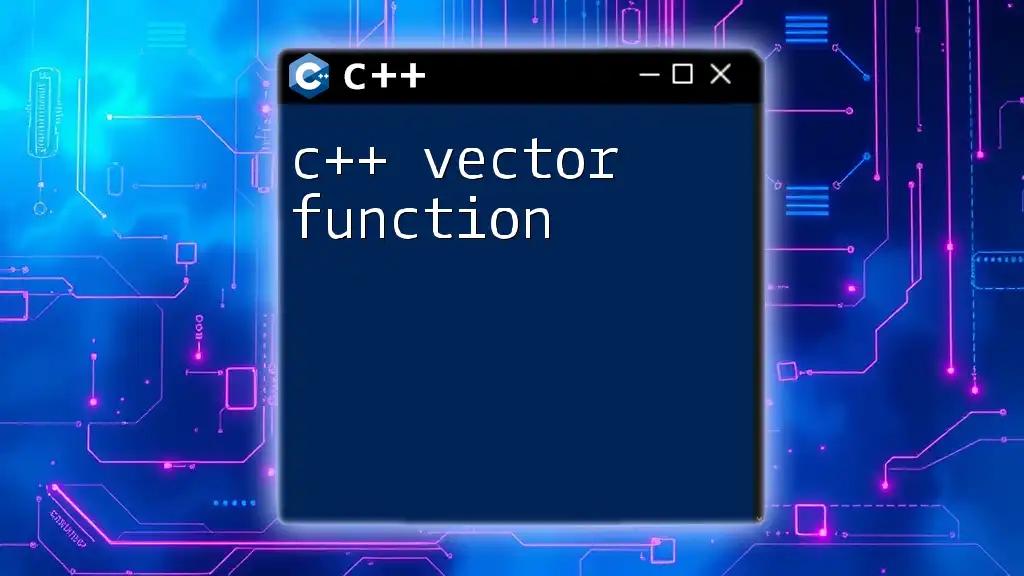
Advanced Vector Operations
Sorting Vectors
The `std::sort` function allows you to sort the contents of a vector easily. However, the vector must be of a type that supports comparison.
std::sort(numbers.begin(), numbers.end());
This example sorts the `numbers` vector in ascending order. Using `std::sort` is efficient and utilizes quicksort or heapsort, depending on the STL implementation.
Searching in Vectors
Linear Search
In cases where the vector is not sorted, a linear search is straightforward but not the most efficient.
bool found = false;
int searchValue = 3;
for (auto num : numbers) {
if (num == searchValue) {
found = true;
break;
}
}
This method checks each element until it finds a match or reaches the end of the vector.
Binary Search
For sorted vectors, use `std::binary_search`, which is much more efficient.
bool found = std::binary_search(numbers.begin(), numbers.end(), 5);
This function will only work correctly if the vector is sorted, as it relies on the order of elements to find the target.
Iterating Over a Vector
Using a Range-based For Loop
One of the easiest ways to traverse a vector is by using a range-based for loop. This approach is clean and less error-prone.
for (auto num : numbers) {
// Process num
}
This method allows you to effectively iterate over elements without worrying about index management.
Using Iterators
You can also use iterators to navigate through a vector. This method is particularly useful when modifying elements during iteration.
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
// Process *it
}
Iterators provide a powerful way to access and manipulate elements within a vector, supporting various STL algorithms.

Best Practices for Using C++ Vectors
When to Use Vectors
Vectors are ideal when you need a dynamic array that can grow and shrink as necessary. They are particularly useful when the number of elements is unpredictable. However, if you need random access to a fixed amount of data without resizing, standard arrays might be more efficient.
Common Pitfalls to Avoid
While vectors offer many advantages, there are some common pitfalls that developers should avoid:
- Memory management issues: Although vectors manage memory automatically, excessive resizing can lead to performance issues. Consider reserving capacity in advance if you know the number of elements you'll need.
- Misunderstanding vector resizing: When a vector resizes, previous elements may be copied to a new memory location, which can lead to increased overhead. Be mindful of this when performing many push operations.
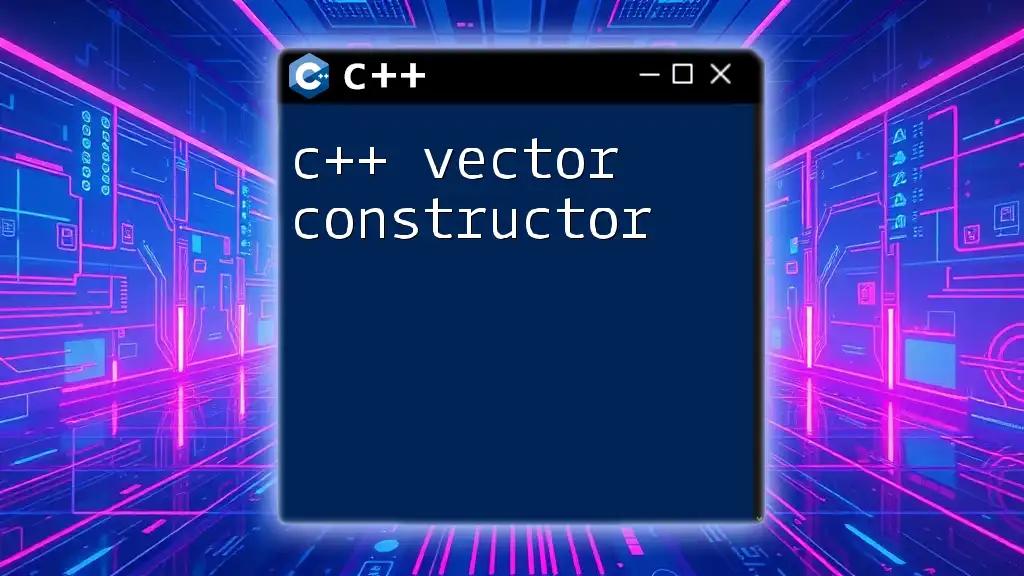
Conclusion
Mastering C++ vector operations is crucial for efficient programming. Vectors provide a dynamic, flexible data structure that can simplify many tasks in C++. By gaining a thorough understanding of vectors and their operations, you can write more effective, maintainable, and efficient C++ code.
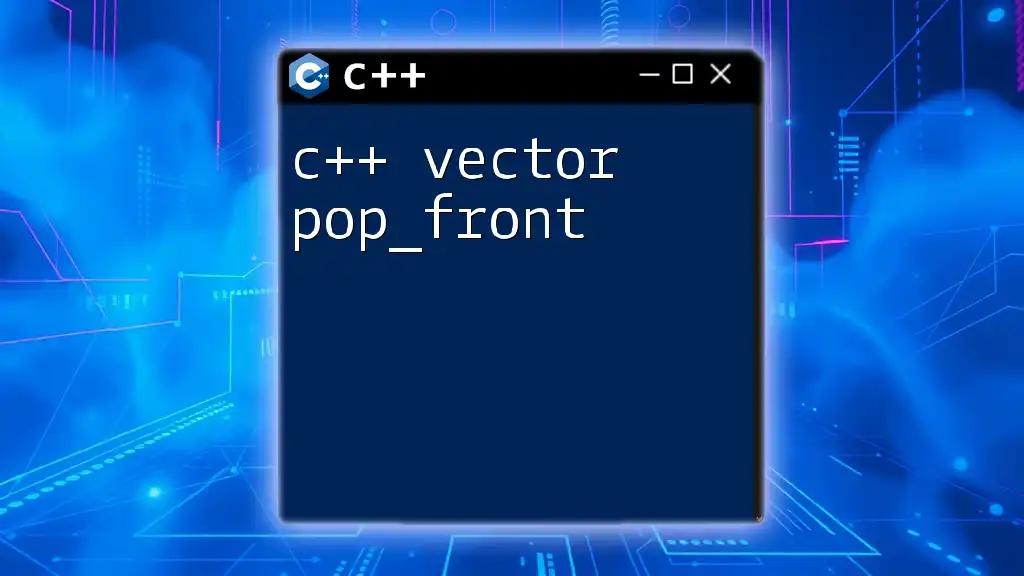
Additional Resources
For more in-depth learning, explore the official C++ documentation on vectors and recommended C++ programming books and resources that can further enhance your understanding of this powerful data structure.