In C++, the order of operations, or operator precedence, determines the sequence in which operators are evaluated in expressions, ensuring correct calculation results; for example, multiplication and division are performed before addition and subtraction.
Here's a code snippet demonstrating this concept:
#include <iostream>
int main() {
int result = 5 + 2 * 3; // Here, 2 * 3 is evaluated first
std::cout << "The result is: " << result << std::endl; // Outputs: The result is: 11
return 0;
}
What is Order of Operations?
Order of operations in programming defines the rules that determine the sequence in which different parts of an expression are evaluated. In C++, understanding this order is crucial for achieving the expected results, as the language employs a specific hierarchy of operations. Much like mathematics, where operations like multiplication take precedence over addition, C++ follows its own set of rules that control how expressions are computed.
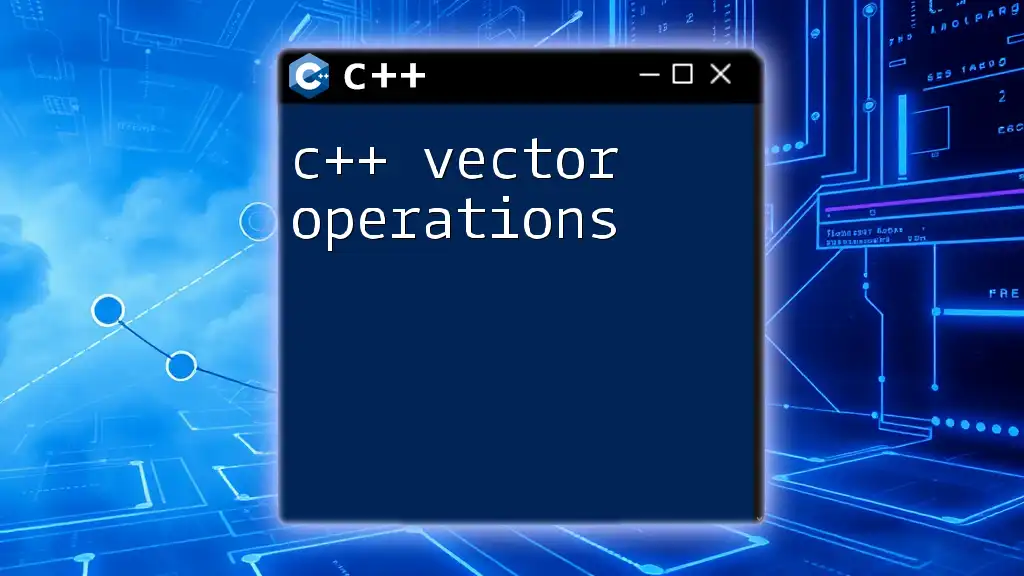
Operators in C++
C++ features a wide variety of operators that perform specific operations on variables and values. A solid understanding of these operators and their interactions is pivotal in mastering the C++ order of operations.
Arithmetic Operators
Arithmetic operators perform basic mathematical functions:
- Addition (`+`)
- Subtraction (`-`)
- Multiplication (`*`)
- Division (`/`)
- Modulus (`%`)
Relational Operators
Relational operators handle comparisons between values:
- Greater than (`>`)
- Less than (`<`)
- Equal to (`==`)
Logical Operators
Logical operators deal with boolean logic:
- AND (`&&`)
- OR (`||`)
- NOT (`!`)
Bitwise Operators
These operators operate on the binary representations of integers:
- AND (`&`)
- OR (`|`)
- XOR (`^`)
- Complement (`~`)
- Left shift (`<<`)
- Right shift (`>>`)
Assignment Operators
Assignment operators are used to assign values to variables:
- Simple assignment (`=`)
- Compound assignment operators (e.g., `+=`, `-=`).
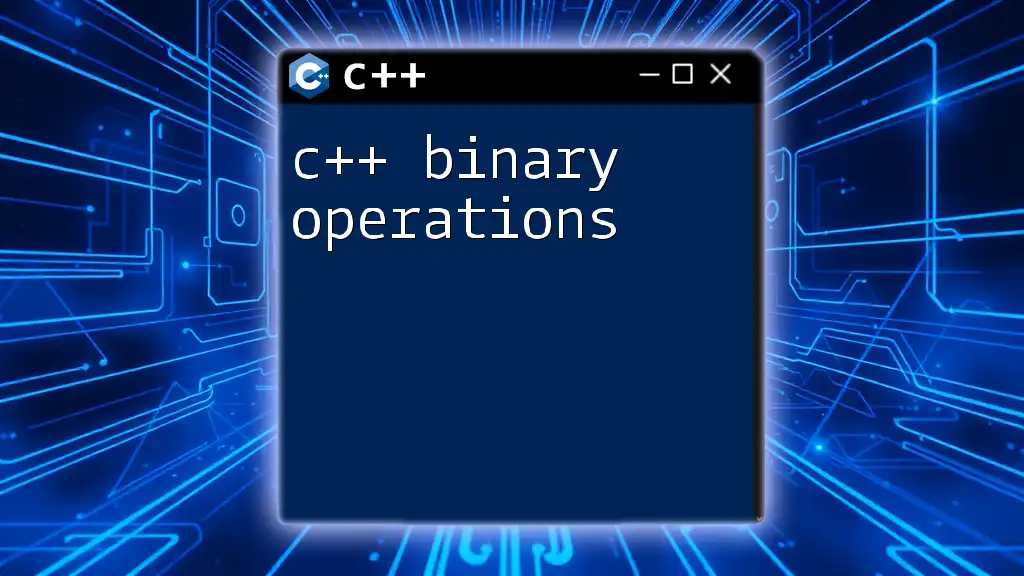
Precedence of Operators
Operator precedence defines the priority of different operators within an expression. Operators with higher precedence are executed first. Understanding this precedence can prevent logical errors in code.
Table of Operator Precedence
Below is a simplified version of operator precedence in C++ from highest to lowest:
Operator Type | Operators |
---|---|
Function Calls | `()` |
Member Dereference | `.` |
Arithmetic | `*`, `/`, `%` |
Addition/Subtraction | `+`, `-` |
Relational | `<`, `<=`, `>`, `>=` |
Equality | `==`, `!=` |
Logical | `&&`, ` |
Assignment | `=`, `+=`, `-=` |
Understanding Precedence Levels
It is essential to recognize how operator precedence impacts expression evaluations.
For example, consider the expression:
3 + 4 * 5
Here, multiplication has a higher precedence than addition, so `4 * 5` is evaluated first, yielding `20`. The overall expression becomes `3 + 20`, resulting in `23`.
For a more complex example:
20 / 4 - 2 * 3
The division and multiplication are evaluated before subtraction as they share higher precedence. Thus, `20 / 4` evaluates to `5` and `2 * 3` to `6`. The final computation processes `5 - 6`, resulting in `-1`.
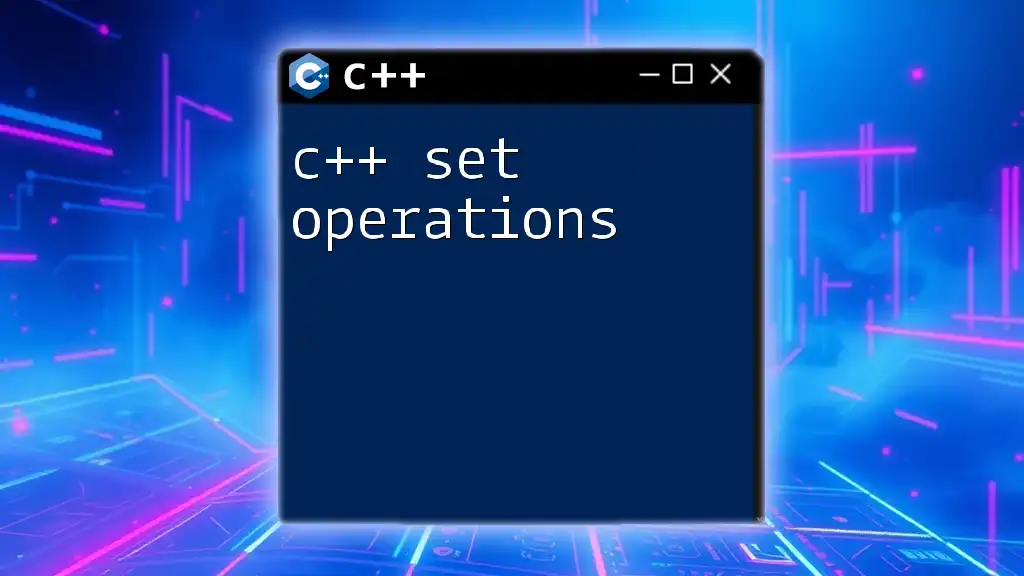
Associativity of Operators
While precedence determines the order of operations, associativity resolves situations where operators of the same precedence level appear. Associativity indicates the direction in which operations are carried out, either left-to-right or right-to-left.
Types of Associativity
- Left-to-Right: Most operators (like `+`, `-`, `*`, `/`) follow this rule.
- Right-to-Left: Certain cases like assignment (`=`) follow this direction.
Examples of Associativity
For instance, consider the expression:
10 - 5 + 2
This expression evaluates using left-to-right associativity: `(10 - 5) + 2`, first giving `5`, then adding `2` to yield `7`.
Another example demonstrating right-to-left associativity:
x = y = z = 10
Here, the assignments are processed from right to left. Thus, `z` takes `10`, then `y` takes `10`, and finally `x` is assigned the value of `10`.
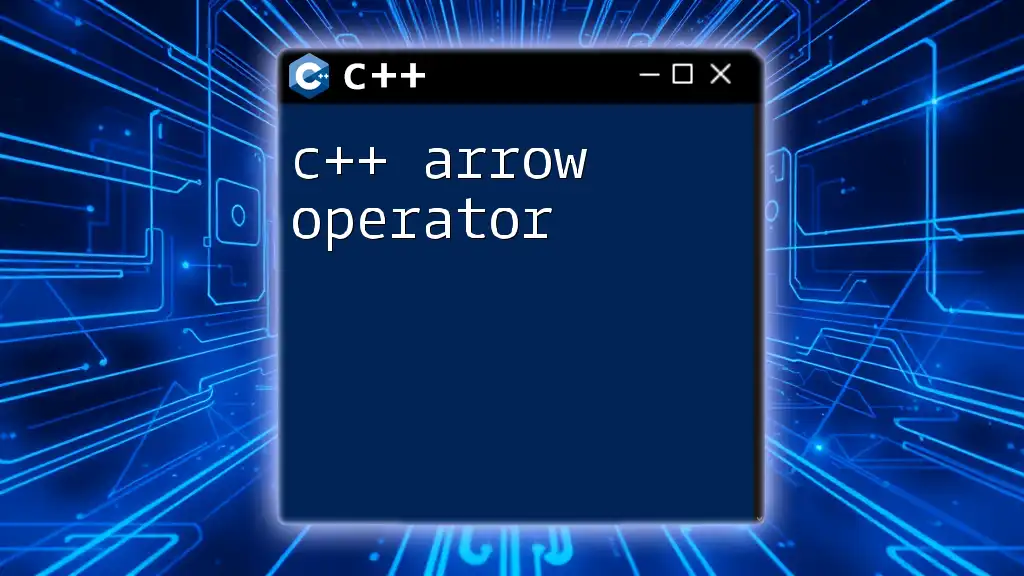
Parentheses and Their Role
Using parentheses can drastically change the outcome of an expression, allowing developers to override default precedence rules. They clarify and explicitly dictate the order in which calculations should occur.
Best Practices for Using Parentheses
- Always use parentheses for complex expressions to enhance readability.
- When in doubt, parenthesize your expressions to avoid unwanted precedence logic.
Examples with Parentheses
Consider the following expression without parentheses:
2 + 3 * 5
According to operator precedence, this evaluates first as `3 * 5`, then adds `2`, yielding `17`.
Now, with parentheses:
(2 + 3) * 5
In this case, the expression within parentheses evaluates first, resulting in `5 * 5` and producing `25`.
For a more complex example involving nested parentheses:
((1 + 2) * (3 + 4))
The two sets of parentheses evaluate first, yielding `3 * 7`, which results in `21`.
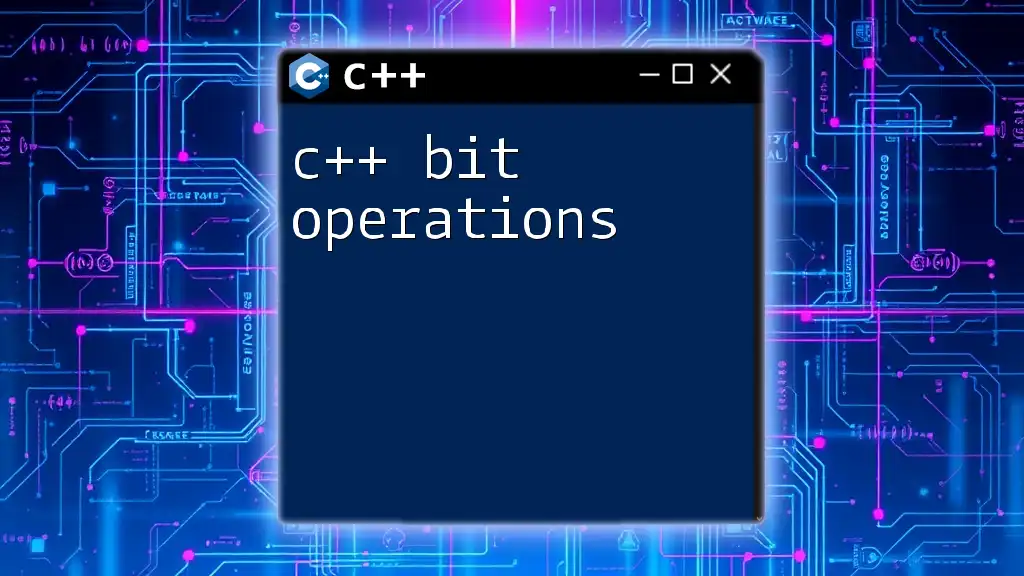
Practical Examples of Order of Operations in C++
Understanding the C++ order of operations is critical in real-world applications. Consider creating a simple calculator function encapsulating various arithmetic operations while following the correct order of operations:
double calculate(double a, double b, double c) {
return a + b * c / (a - b);
}
In this function, the multiplication and division will precede the addition, and the result will reflect proper precedence.
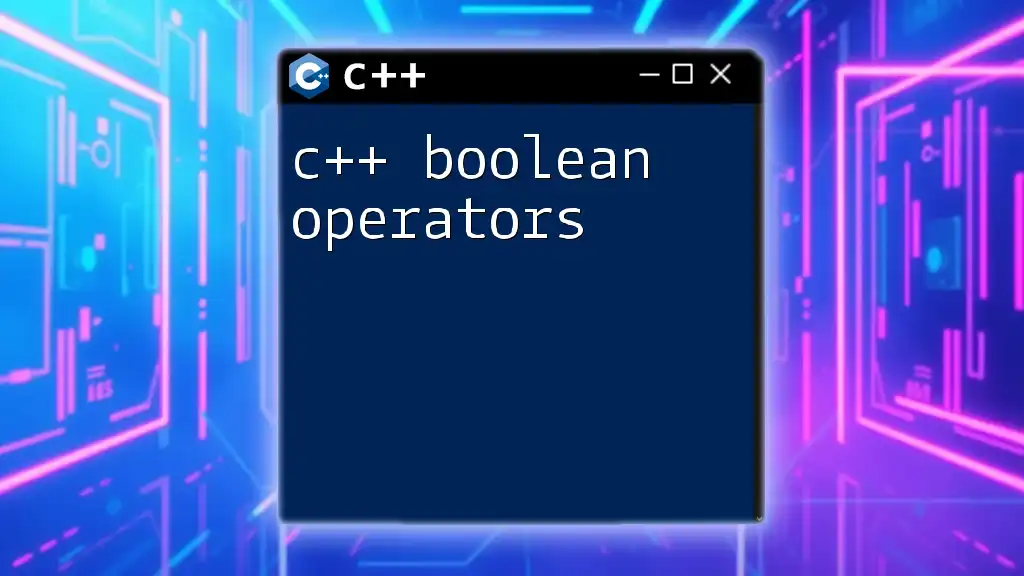
Common Errors Related to Order of Operations
Many new programmers encounter errors stemming from misconceptions about operator precedence. Common mistakes include:
- Misplacing parentheses, leading to unintended consequences.
- Assuming default precedence without careful evaluation, which can yield incorrect results.
Resolving Order of Operation Mistakes
To correct these errors, regularly debug complex expressions and clarify the intended order of operations using parentheses.
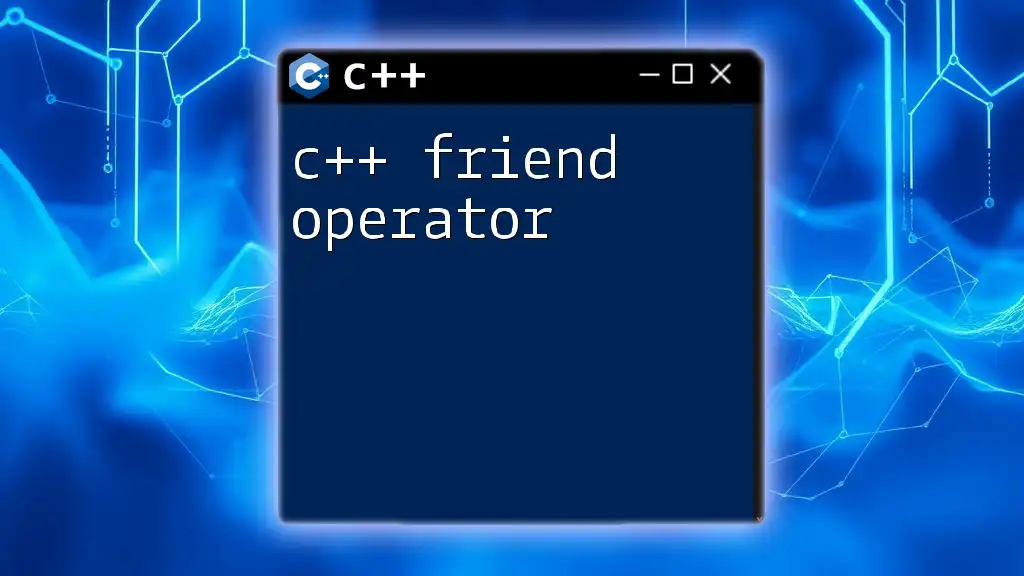
Conclusion
Understanding the C++ order of operations is fundamental for anyone looking to write reliable code. By mastering operator precedence, associativity, and the strategic use of parentheses, programmers can avoid common pitfalls and ensure their expressions yield the desired outcomes.
As you continue to explore C++, practice with various examples to solidify your understanding of this vital concept.