C++ boolean operators (`&&`, `||`, and `!`) are used to perform logical operations between boolean expressions, allowing you to evaluate conditions in control statements.
Here’s a simple code snippet demonstrating their usage:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
cout << "a && b: " << (a && b) << endl; // Outputs 0 (false)
cout << "a || b: " << (a || b) << endl; // Outputs 1 (true)
cout << "!a: " << !a << endl; // Outputs 0 (false)
return 0;
}
Understanding Boolean Data Types
What is Boolean Type?
The boolean type is a fundamental data type in C++ that represents truth values. It can hold only two possible values: `true` and `false`. This simplicity is what makes boolean types invaluable in programming, particularly in control flow and conditional logic.
Declaring Boolean Variables
Declaring a boolean variable in C++ is straightforward. The syntax involves using the keyword `bool`, followed by the variable name and its initial value. For instance, you can declare a boolean variable like this:
bool isActive = true;
Here, `isActive` will be `true`. This capability to store true or false values becomes critical when you implement decision-making structures in your code.
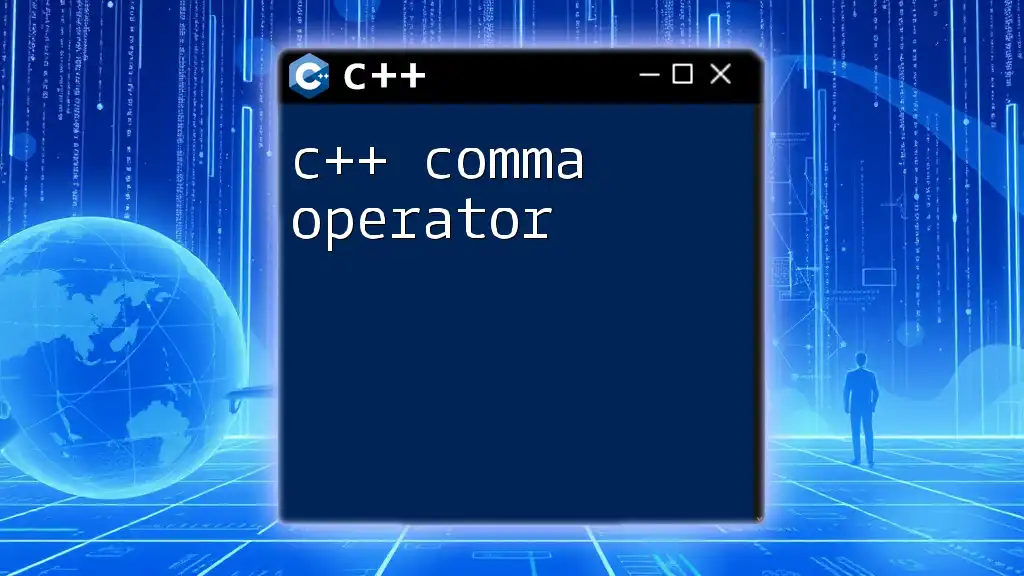
Overview of Boolean Operators
Definition of Boolean Operators
Boolean operators are used to perform logical operations on boolean values. When you combine different conditions, the results can influence the flow of your program. These operators enable programmers to create complex conditional statements that can take multiple paths based on various criteria.
Types of Boolean Operators
Logical AND (&&)
The logical AND operator (&&) checks whether both operands are true. If both conditions evaluate to `true`, the result is `true`; otherwise, it is `false`. This operator is particularly useful when you want multiple conditions to be true before executing a particular block of code.
For example:
if (a > 0 && b > 0) {
// Code to execute if both a and b are positive
}
In the example above, the code block runs only if both `a` and `b` are greater than zero.
Logical OR (||)
The logical OR operator (||), on the other hand, checks if at least one of the operands is true. If either operand evaluates to true, the whole expression returns true. This operator is used when you want to execute a code block if one or more conditions are satisfied.
For example:
if (a > 0 || b > 0) {
// Code to execute if either a or b is positive
}
Here, the code will run if either `a` or `b` is greater than zero.
Logical NOT (!)
The logical NOT operator (!) negates the boolean value of its operand. If the operand is true, using the NOT operator will turn it into false, and vice versa. This operator is useful for flipping or inverting conditions.
For example:
if (!isActive) {
// Executes if isActive is false
}
In this case, the code within the block will execute only if `isActive` is `false`.
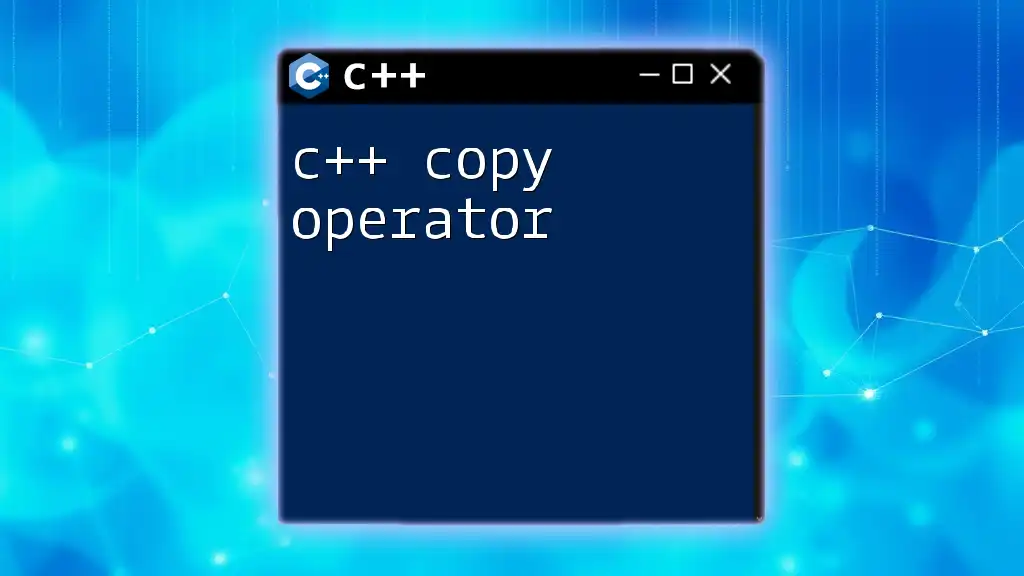
Combining Boolean Operators
Chaining Conditions
You have the flexibility to combine multiple boolean expressions using the AND, OR, and NOT operators. This capability allows for more complex checks.
Consider the following example:
if ((a > 0 && b > 0) || c == 10) {
// Executes if either both a and b are positive
// or c equals 10
}
Operator Precedence
It is essential to understand the precedence of boolean operators. The logical NOT (`!`) operator has the highest precedence, followed by logical AND (`&&`), and finally logical OR (`||`), which holds the lowest precedence.
Here’s how precedence looks visually:
Operator | Description |
---|---|
! | Highest precedence |
&& | Middle precedence |
Using parentheses to group conditions can clarify the order of evaluation and enhance code readability.
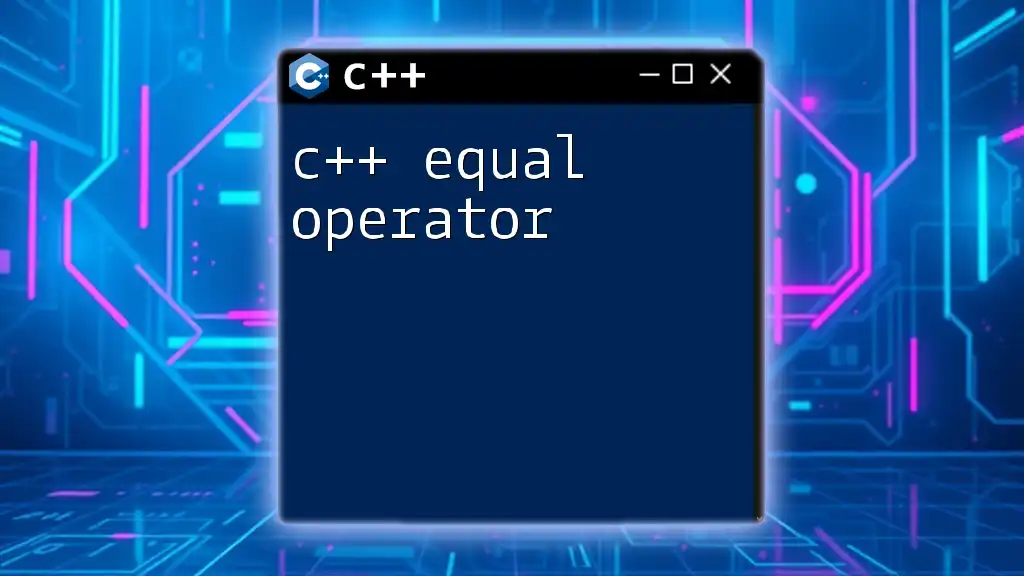
Practical Applications of Boolean Operators
Control Flow in Conditional Statements
Boolean operators play a vital role in governing the flow of conditional statements such as `if`, `else if`, and `else`. Here’s a simple example:
if (age >= 18 && hasLicense) {
cout << "You can drive!";
}
In this case, the program checks whether a person is both 18 years or older and has a driver’s license. Only when both conditions are fulfilled will the message print.
Loops and Boolean Conditionals
Boolean operators can also control the flow of loops. Consider a `while` loop that runs as long as a certain condition remains true:
while (isRunning && userInput != "exit") {
// The loop continues until isRunning is false
// or userInput is "exit"
}
This loop will keep executing until either `isRunning` is `false` or `userInput` becomes "exit".
Boolean Operators in Function Return Values
Boolean expressions are often at play when returning values from functions. Functions can return simple true/false results effectively using boolean logic.
For example, consider the function below:
bool isEligible(int age) {
return (age >= 18);
}
In this function, `true` is returned if the age is 18 or above, illustrating the use of boolean operators to evaluate conditions succinctly.
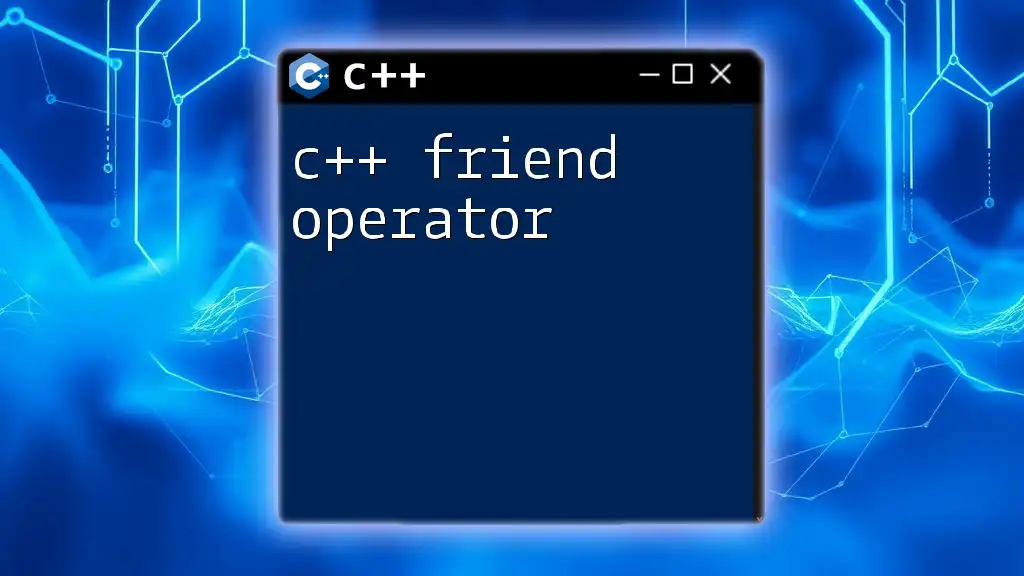
Best Practices when Using Boolean Operators
Readability Matters
When working with boolean logic, the readability of your expressions is crucial. Use parentheses around conditions to ensure clarity and make it easier for yourself and others to understand what each condition represents. For instance, consider using:
if ((age >= 18 && hasLicense) || isSupervised) {
// Clear understanding of conditions
}
Avoiding Common Pitfalls
Common mistakes include overcomplicating expressions, which can lead to logical errors and confusion. Double-check your boolean logic to ensure you aren't missing critical condition checks. Testing conditions and including debug statements can aid in identifying logical errors during program execution.
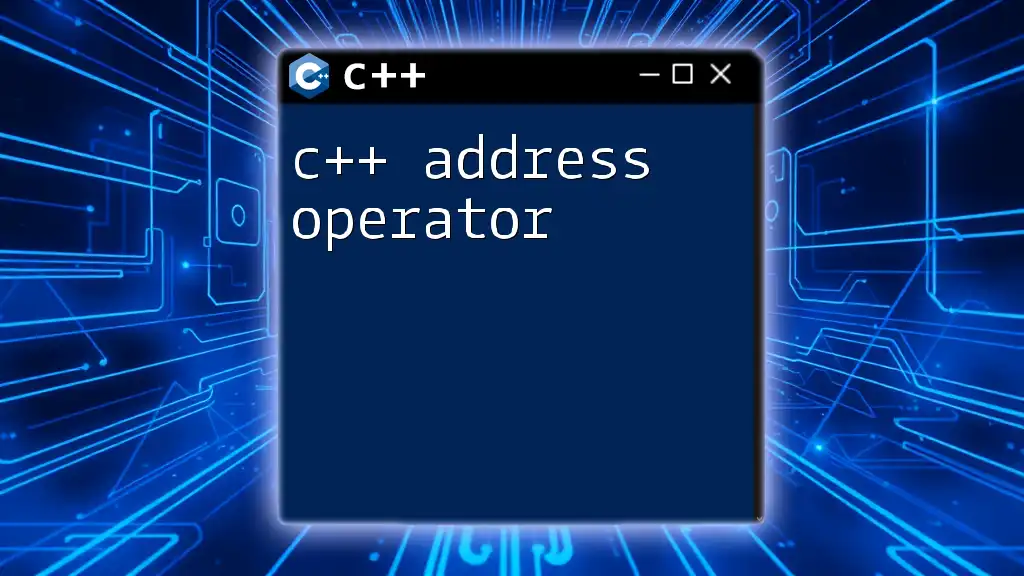
Conclusion
Mastering C++ boolean operators is vital for every programmer looking to utilize C++ effectively. They form the bedrock of logical constructs that guide decision-making within any program. Continually practicing these principles will empower you to craft programs that operate efficiently and logically.
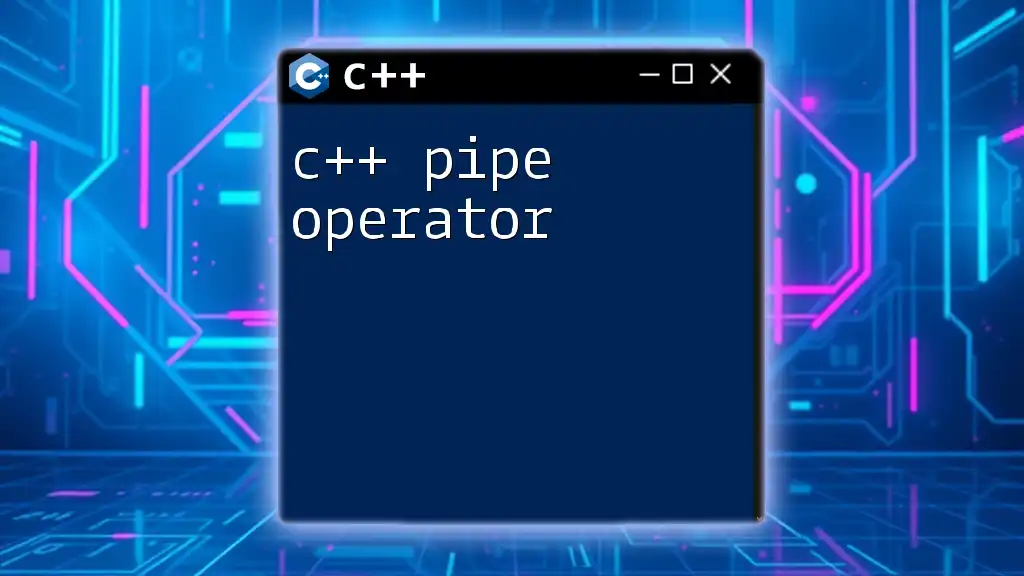
Additional Resources
Recommended Reading
To further your understanding of C++ and boolean operators, consider exploring various books, authoritative websites, and online courses that dive into advanced programming concepts.
Community and Support
Joining online communities or forums can provide ongoing support and enable knowledge sharing among C++ learners and professionals. Networking with others can enrich your learning experience and introduce you to various perspectives on programming challenges.