The C++ comma operator evaluates its first operand and discards the result, then evaluates and returns the second operand, allowing for the execution of multiple expressions in a single statement.
#include <iostream>
int main() {
int a = 1, b = 2;
int result = (a += 3, b += 4); // a becomes 4, b becomes 6, result is 6
std::cout << "a: " << a << ", b: " << b << ", result: " << result << std::endl;
return 0;
}
Understanding the Basics of the Comma Operator
What is the Comma Operator?
The C++ comma operator (`,`) is a binary operator that allows you to evaluate two expressions and returns the value of the second expression. Its primary function is to separate multiple expressions within a single statement. The syntax is simple: it consists of two operands separated by a comma:
expr1, expr2
As you can see, `expr1` is evaluated first, and then the result of `expr2` is returned. This might sound straightforward, but understanding where and when to employ this operator effectively is crucial for writing clean and efficient C++ code.
When to Use the Comma Operator
The comma operator proves useful in various scenarios. Here are some common use cases:
- In loops: where multiple variables need to be updated in a single iteration.
- In function calls: when you need to execute several operations before passing parameters.
- Within conditional statements: allowing you to make concise decisions based on multiple expressions.

How the Comma Operator Works in C++
Evaluating Multiple Expressions
When you utilize the comma operator, each expression is evaluated in sequence from left to right. However, it’s essential to note that only the value of the second expression is returned. This sequential evaluation allows for executing different side effects in each expression.
Return Value of the Comma Operator
The whole expression returns the final value of `expr2`; any value calculated in `expr1` is discarded. Here's an example that illustrates this behavior:
int result = (1 + 2, 3 + 4);
In this case, the value of `result` will be 7, as the first expression (1 + 2) is evaluated but not returned.
Example: Using the Comma Operator in a Loop
A common use case of the C++ comma operator can be illustrated within a `for` loop, where multiple variables might be updated in a single statement:
for (int i = 0, j = 10; i < j; ++i, --j) {
// Loop will execute while i < j, i increments, j decrements
std::cout << "i: " << i << ", j: " << j << std::endl;
}
In this loop, both `i` and `j` are updated during each iteration using the comma operator, producing a cleaner and more compact loop structure.

Practical Applications of the Comma Operator
Improving Readability in Code
The comma operator can significantly enhance code readability, allowing you to condense multiple assignments or expressions into a single line. For instance, consider the transformation of redundant statements:
x = 5;
y = 10;
z = x + y;
This can be simplified using the comma operator:
int z = (x = 5, y = 10, x + y);
In this snippet, the use of the comma operator clearly indicates the series of actions leading to the result stored in `z`.
Applications in Function Calls
You can also leverage the comma operator when calling functions, especially when the function accepts multiple parameters.
void display(int x, int y) {
std::cout << "X: " << x << ", Y: " << y << std::endl;
}
display((a = 3, b = 4), (c = 5, d = 6)); // uses comma operator for assignment
In this example, multiple assignments are made in function calls, showcasing how the comma operator streamlines coding practices.
Using the Comma Operator in Conditional Statements
The comma operator can be employed in conditional statements, albeit with caution. It allows several expressions to be executed before the control flow continues:
if ((a = 1, b = 2, a < b)) {
std::cout << "Condition met: a is less than b" << std::endl;
}
In this example, you can see that `a` and `b` are assigned values, and the result of `a < b` determines the flow. However, this usage can obfuscate code readability, so it should be used judiciously.
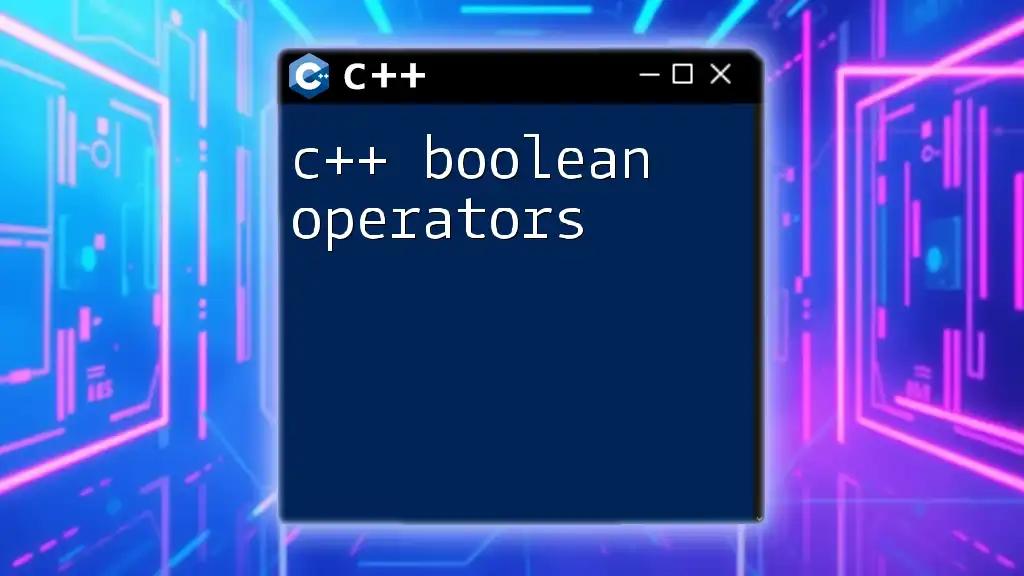
Common Pitfalls and Best Practices
Avoiding Confusion with Operator Precedence
One of the most significant pitfalls of using the C++ comma operator arises from confusion regarding operator precedence. Since the comma operator has a lower precedence than most other operators, unexpected behaviors may occur if not handled carefully. Always group expressions with parentheses when using the comma operator within larger expressions.
Best Practices for Using the Comma Operator
To use the comma operator effectively, consider the following best practices:
- Maintain clarity: While the comma operator can condense code, using it excessively may lead to confusion. Always prioritize readability.
- Limit usage in complex structures: Employ the comma operator judiciously in nested expressions to avoid ambiguity.
- Use comments: Annotate your code where the comma operator is employed so that others can understand your intent easily.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/_next/image?url=%2Fimages%2Fposts%2Fc%2Fcpp-operator.webp&w=1080&q=75)
Conclusion
In summary, the C++ comma operator provides a powerful mechanism for evaluating multiple expressions within a single statement. Its applications can simplify code, enhance readability, and improve efficiency when used appropriately. However, it’s essential to understand its syntax, behavior, and potential pitfalls to use it effectively.
As you continue to explore the nuances of C++, be encouraged to experiment with various operators, including the comma operator in different scenarios, to gain deeper insights into its capabilities and limitations.

Additional Resources
To delve further into advanced C++ features and operators, consider exploring external articles and tutorials dedicated to enhancing your understanding of C++ programming.

Frequently Asked Questions
What are the limitations of the comma operator?
The C++ comma operator has limitations primarily in terms of readability and potential for confusion. It should not be overused, especially in places where clarity is paramount.
Can I use the comma operator in class members?
While technically possible, using the comma operator within class members is generally discouraged. It can lead to less readable code and should be avoided in favor of clearer approaches.