The XOR operator (^) in C++ performs a bitwise exclusive OR operation on two integer values, returning a result where each bit is set to 1 only if the corresponding bits of the operands are different.
Here’s a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a ^ b; // result is 6 (0110 in binary)
std::cout << "The result of a XOR b is: " << result << std::endl;
return 0;
}
Understanding XOR in C++
The XOR (exclusive or) operator is a fundamental bitwise operator in C++. It plays a vital role in binary arithmetic, logical operations, and various algorithms.
The XOR operator evaluates two bits and returns true (or `1`) only if the bits are different. Contrast this with logical AND and OR operators, where:
- AND returns true only if both bits are true (1).
- OR returns true if at least one bit is true.
Here's the truth table for the XOR operator:
A | B | A XOR B |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Key properties of the XOR operation include:
- Commutative: `A ^ B = B ^ A`
- Associative: `A ^ (B ^ C) = (A ^ B) ^ C`
- Identity: `A ^ 0 = A`
- Self-inverse: `A ^ A = 0`
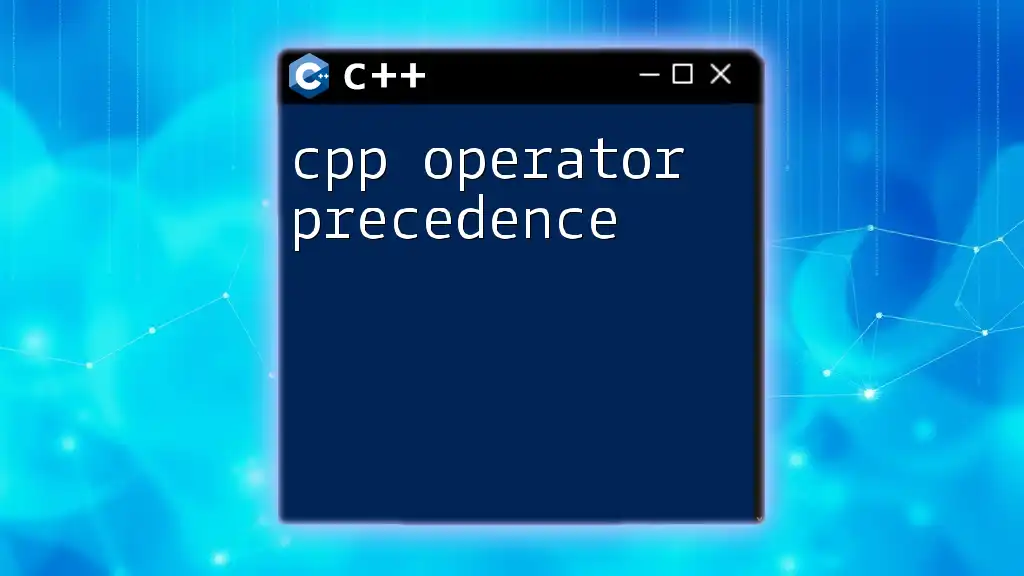
Syntax of XOR Operator in C++
In C++, the XOR operator is represented with the caret symbol (`^`).
The basic syntax is as follows:
result = a ^ b; // where a and b are operands
Explanation of Operand Types
The XOR operator operates on integral types such as `int`, `char`, and `long`. It essentially works on the binary representation of these numbers, allowing bitwise manipulation.
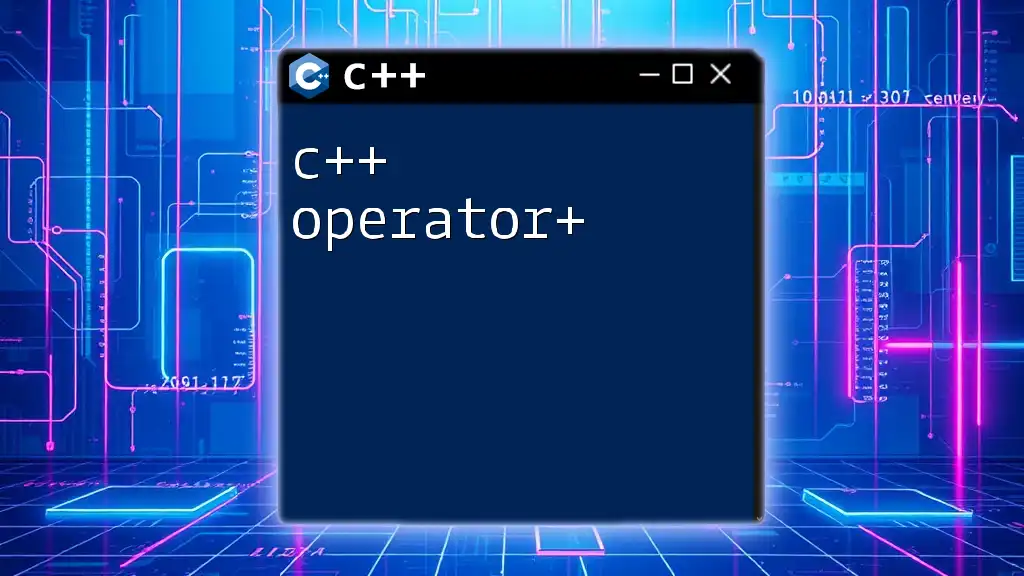
Functionality of the XOR Operator in C++
How XOR Works with Binary Numbers
When you perform an XOR operation on two integers, the operation looks at the binary representation of both numbers.
For instance, consider two integers:
- `a = 5` (binary: `0101`)
- `b = 3` (binary: `0011`)
When applying XOR:
0101 (5)
^ 0011 (3)
-------
0110 (6)
Here’s a sample code demonstrating the XOR operation and its result:
#include <iostream>
int main() {
int a = 5; // binary: 0101
int b = 3; // binary: 0011
int result = a ^ b; // result: 0110 => 6
std::cout << "Result of 5 XOR 3: " << result << std::endl;
return 0;
}
Explanation of the Result
The result, `6` (binary `0110`), demonstrates that the XOR of `5` and `3` produces a new number where each bit is a result of the XOR operation on the corresponding bits of `5` and `3`.
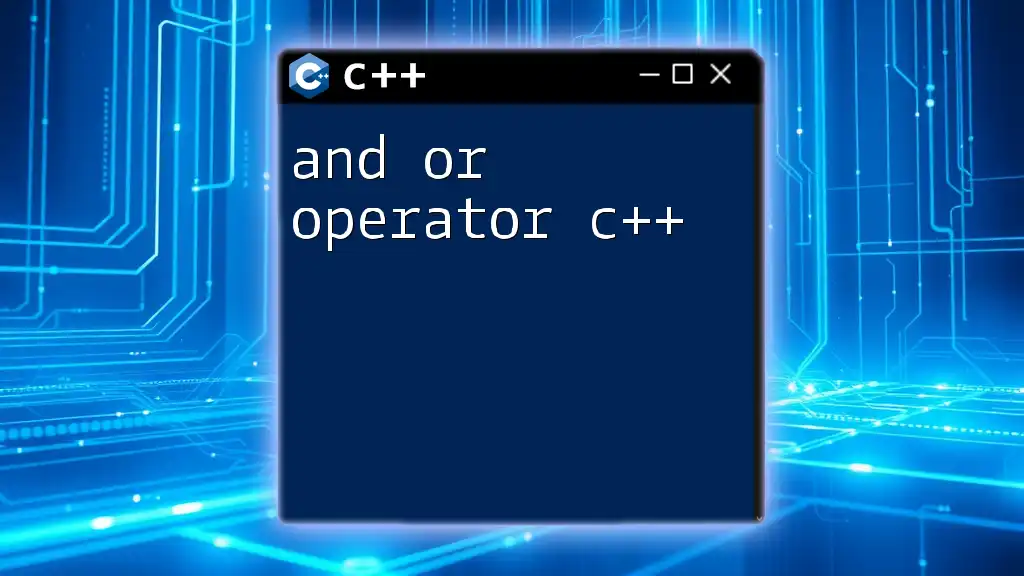
Useful Applications of the XOR Operator in C++
Cryptography and Data Security
The XOR operator has significant applications in simple encryption techniques. The encryption process can leverage the fact that a value XOR’d with a key can be decrypted by XOR’ing it again with the same key. This property makes XOR a fundamental tool in symmetric encryption algorithms.
Swapping Values Without a Temporary Variable
The XOR operator allows you to swap two variables without needing a temporary variable. This method can be particularly elegant and efficient:
#include <iostream>
int main() {
int a = 10;
int b = 5;
a = a ^ b; // Step 1
b = a ^ b; // Step 2
a = a ^ b; // Step 3
std::cout << "After swapping: a = " << a << ", b = " << b << std::endl;
return 0;
}
In this example, the final output will show the variables `a` and `b` have been successfully swapped using the XOR operation.
Parity Checking
The XOR operator can also be used for error detection in data transmission via parity checks. When sending a sequence of bits, the sender can calculate the parity bit using XOR to ensure that the total number of `1`s is even (even parity) or odd (odd parity).
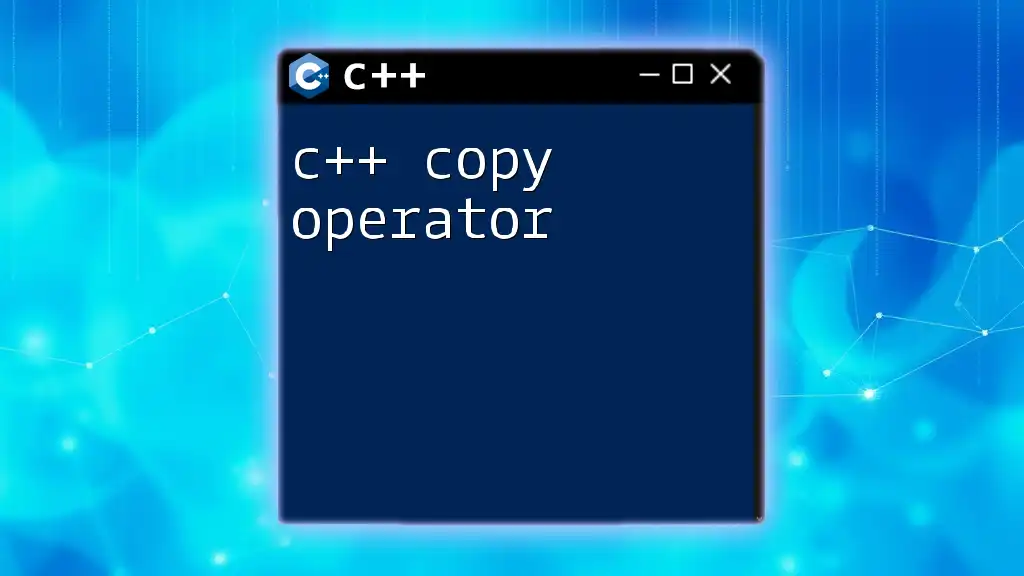
Common Mistakes and Pitfalls
One common mistake when working with the XOR operator involves misunderstanding its properties. For example, a XOR a equals 0. This can lead to errors when checking conditions or processing binary data.
Additionally, be cautious of data types; using non-integer types or mixed types can yield unexpected results or compilation errors.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
XOR Operator vs Other Operators in C++
When comparing the XOR operator with AND and OR, it is crucial to understand when to use each operator. XOR is particularly useful when you want to determine differences between two binary states or inputs.
The performance of XOR operations is generally quite high in C++, making them preferred for certain algorithms, especially those that manipulate bits directly. However, always consider readability and maintainability when deciding to use bitwise operations, as excessive use of bitwise logic can make code less understandable.
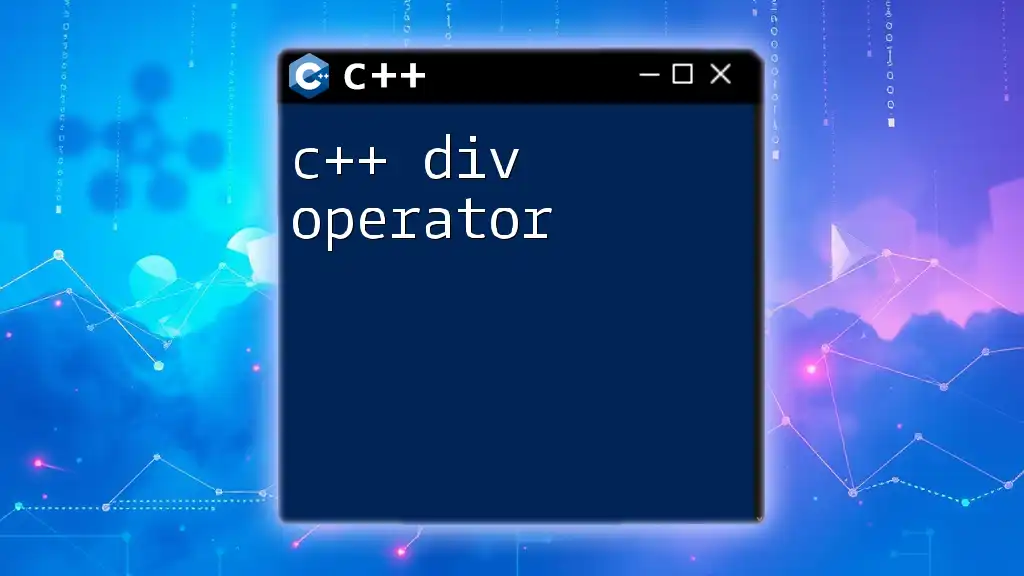
Conclusion
The C++ XOR operator is a powerful and versatile tool in programming, particularly useful in applications involving cryptography, data swaps, and parity checks. Understanding how to efficiently harness this operator can enhance your programming skills and offer elegant solutions to complex problems.
Take time to practice XOR operations with various example cases to cement your understanding. As you dive deeper into C++, you'll find that mastering operators like XOR can significantly elevate your coding proficiency.
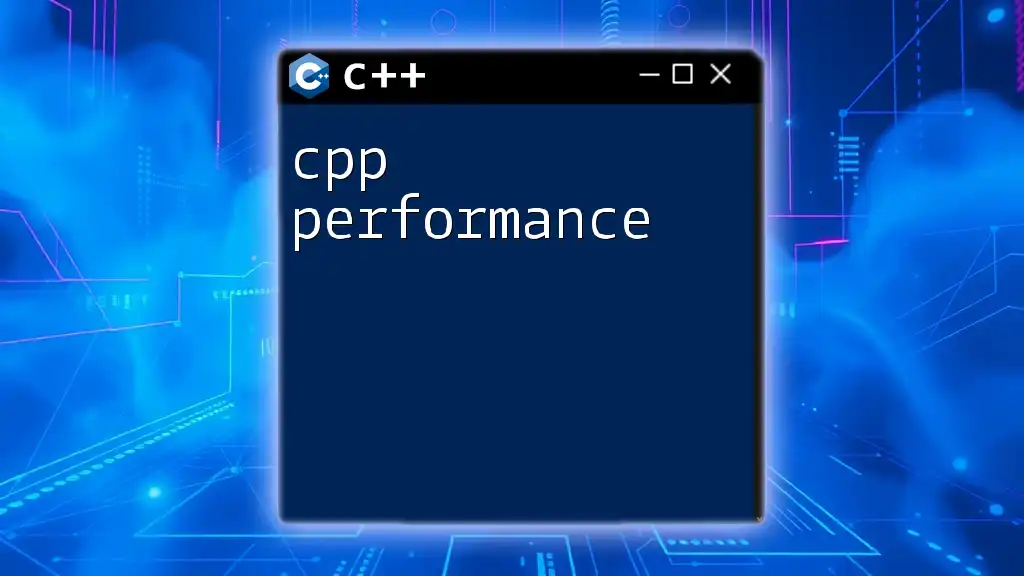
References and Further Reading
For further insights into the XOR operator and C++ programming, consider exploring online resources, tutorials, and recommended literature that focus on bitwise operations and their applications in real-world scenarios.